How to Save Custom Data from WooCommerce Custom Checkout Fields: A Step-by-Step Guide
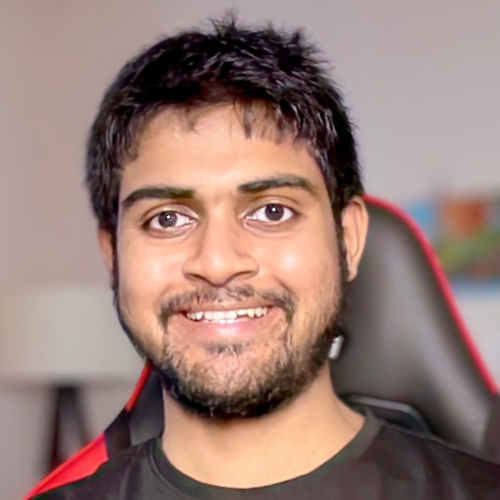
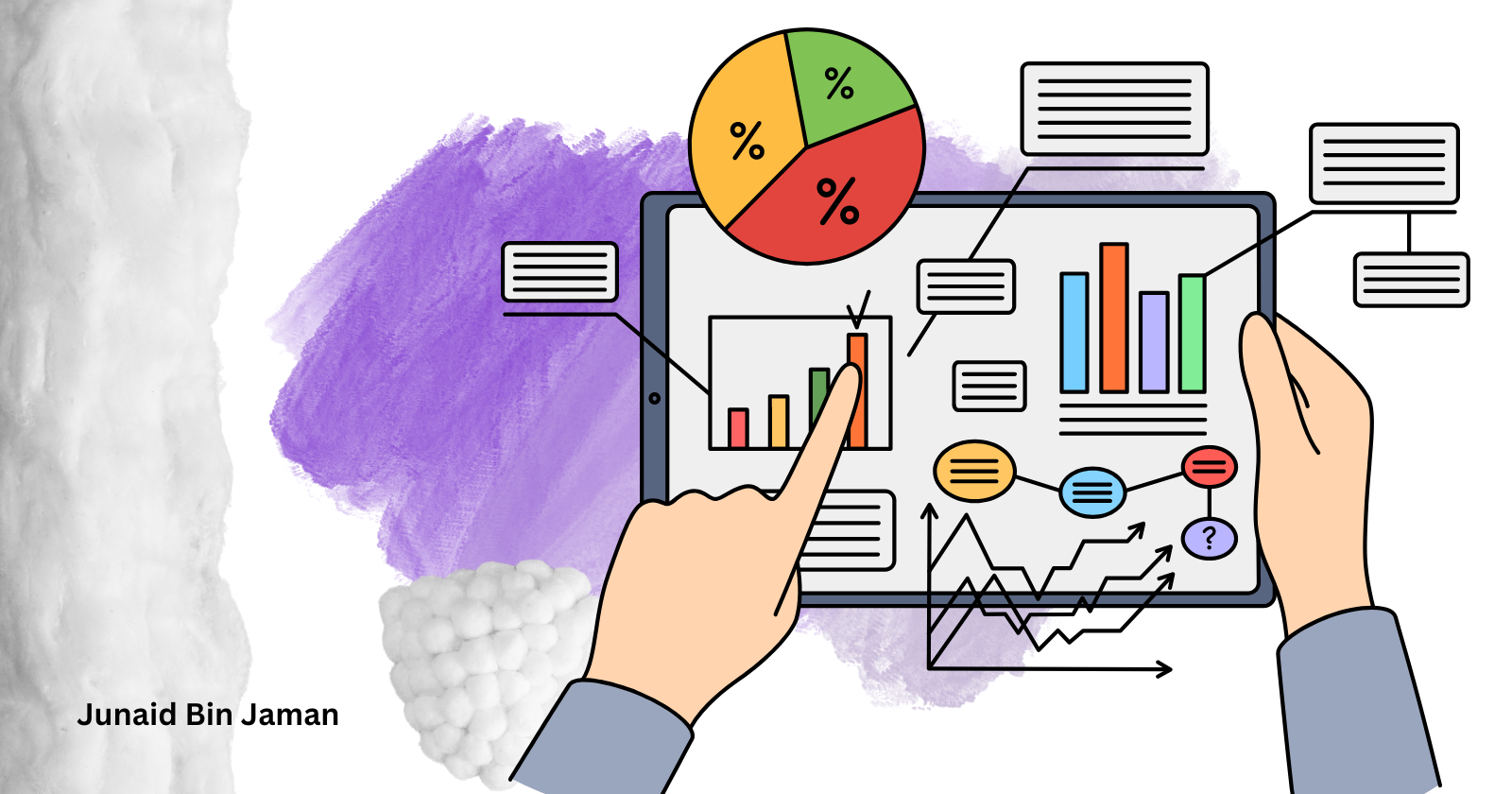
Adding custom checkout fields in WooCommerce is a powerful way to enhance the checkout experience and collect additional information from your customers. However, once you've added these fields, you'll need to save the data entered by users so it can be accessed later. This blog will guide you through the process of saving custom data from WooCommerce custom checkout fields, complete with examples and explanations.
1. Introduction to Saving Custom Checkout Data
When customers fill out custom fields during the checkout process, their input needs to be stored in the order meta data. This ensures that the information is saved with the order and can be accessed later by administrators or used in order processing.
Example Scenario
Let's say you've added a custom field to collect the customer's preferred delivery date. You want to ensure that this data is saved and displayed in the order details.
2. Adding Custom Checkout Fields
Before we can save the custom data, you must have a custom checkout field in place. If you haven't added one yet, here's a quick example:
add_action('woocommerce_after_order_notes', 'custom_checkout_field');
function custom_checkout_field($checkout) {
echo '<div id="custom_checkout_field">';
woocommerce_form_field('preferred_delivery_date', array(
'type' => 'date',
'class' => array('form-row-wide'),
'label' => __('Preferred Delivery Date'),
'placeholder' => __('Select your preferred delivery date'),
'required' => true,
), $checkout->get_value('preferred_delivery_date'));
echo '</div>';
}
This code adds a date picker field to the checkout form for customers to choose their preferred delivery date.
3. Validating the Custom Checkout Field
Before saving the custom data, it's essential to validate the field to ensure the input is correct. For example, you may want to check that the date is not in the past.
Here's how to validate the custom field:
add_action('woocommerce_checkout_process', 'validate_custom_checkout_field');
function validate_custom_checkout_field() {
if (!$_POST['preferred_delivery_date']) {
wc_add_notice(__('Please select a preferred delivery date.'), 'error');
}
}
This code checks if the preferred delivery date field is empty and adds an error notice if it is.
4. Saving the Custom Checkout Field Data
Now that the custom field has been added and validated, it's time to save the data when the order is processed. This is done using the woocommerce_checkout_update_order_meta
action hook.
Example Code:
add_action('woocommerce_checkout_update_order_meta', 'save_custom_checkout_field');
function save_custom_checkout_field($order_id) {
if (!empty($_POST['preferred_delivery_date'])) {
update_post_meta($order_id, 'preferred_delivery_date', sanitize_text_field($_POST['preferred_delivery_date']));
}
}
Explanation:
Action Hook: The
woocommerce_checkout_update_order_meta
hook is triggered when the order is being processed, allowing you to save custom data to the order.update_post_meta: This function saves the data to the order’s meta data. The
sanitize_text_field
function is used to sanitize the input before saving it to the database.
5. Displaying the Custom Data in the Admin Order Page
To make the saved data visible on the order detail page in the WordPress admin, you can use the following code:
add_action('woocommerce_admin_order_data_after_billing_address', 'display_custom_checkout_field_data_in_admin', 10, 1);
function display_custom_checkout_field_data_in_admin($order) {
$preferred_delivery_date = get_post_meta($order->get_id(), 'preferred_delivery_date', true);
if ($preferred_delivery_date) {
echo '<p><strong>' . __('Preferred Delivery Date') . ':</strong> ' . esc_html($preferred_delivery_date) . '</p>';
}
}
Explanation:
Action Hook:
woocommerce_admin_order_data_after_billing_address
is used to add custom content after the billing address in the order details.get_post_meta: Retrieves the saved custom data from the order’s meta data.
6. Conclusion
Saving custom data from WooCommerce custom checkout fields is a crucial part of customizing the checkout process. By following the steps outlined in this guide, you can ensure that any custom information entered by your customers is validated, saved, and accessible for future use. Whether you're collecting delivery preferences, special instructions, or any other custom data, this approach will help you effectively manage and utilize the information within WooCommerce.
With these techniques, you can take full control of your WooCommerce checkout process, providing a more personalized and efficient experience for both you and your customers.
Subscribe to my newsletter
Read articles from Junaid Bin Jaman directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
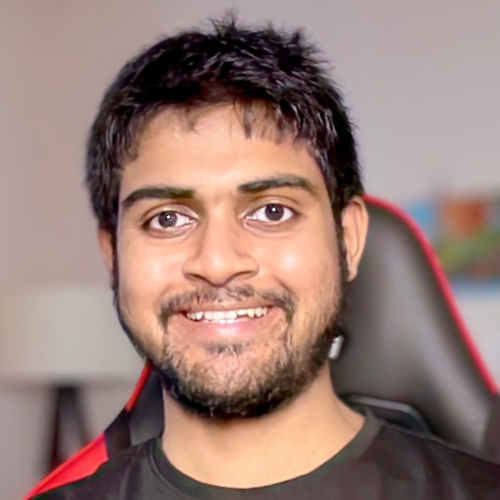
Junaid Bin Jaman
Junaid Bin Jaman
Hello! I'm a software developer with over 6 years of experience, specializing in React and WordPress plugin development. My passion lies in crafting seamless, user-friendly web applications that not only meet but exceed client expectations. I thrive on solving complex problems and am always eager to embrace new challenges. Whether it's building robust WordPress plugins or dynamic React applications, I bring a blend of creativity and technical expertise to every project.