Streamlining Resource Management in Python: A Deep Dive into Context Managers and the with Statement

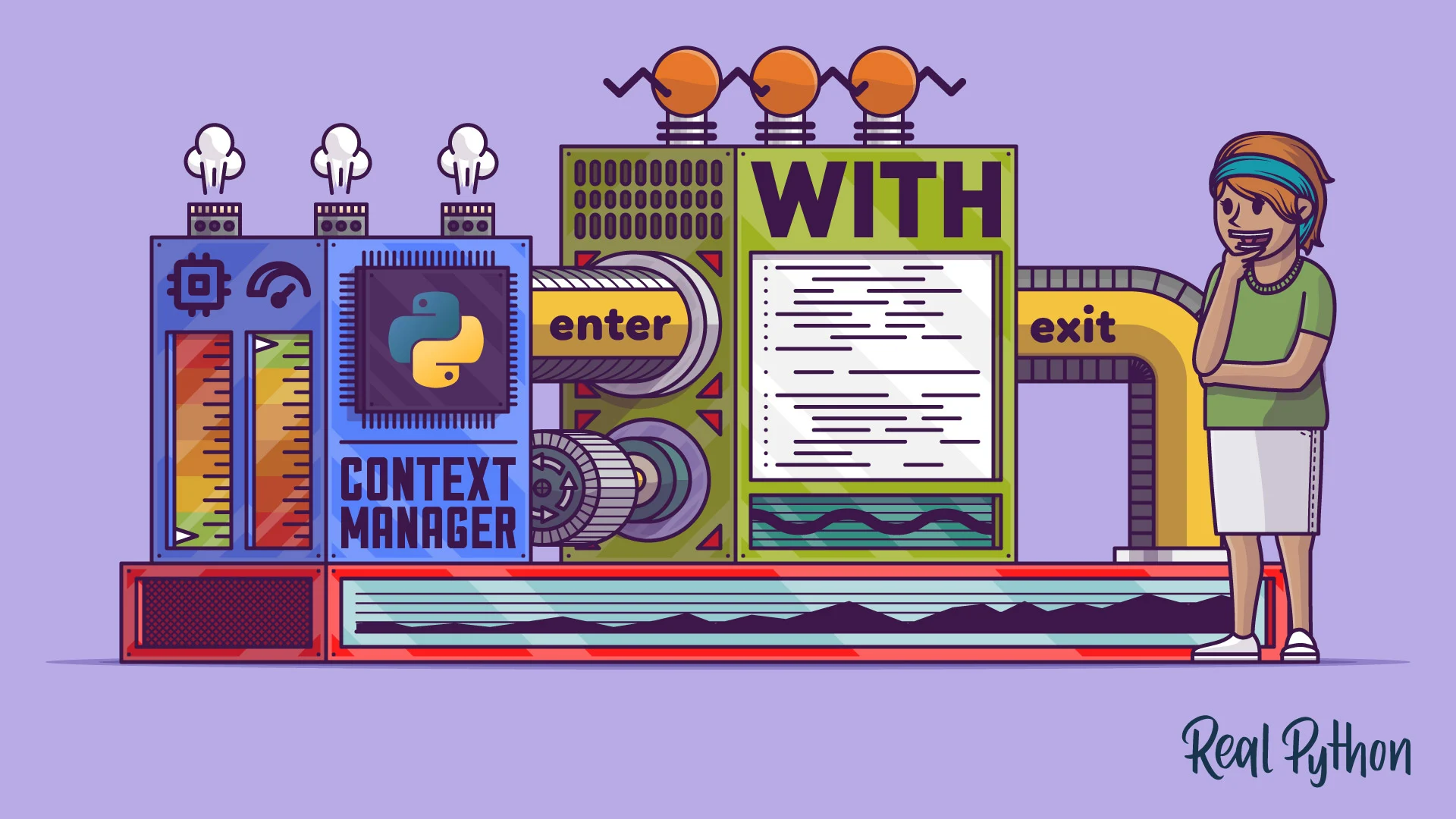
Efficient resource management is a cornerstone of robust software development. In Python, one of the most effective tools for managing resources—such as files, network connections, and database transactions—is the context manager. The with
statement, which leverages context managers, provides a clean and readable way to ensure that resources are properly acquired and released.
What is a Context Manager?
A context manager is an object that defines the runtime context to be established when executing a with
statement. It is responsible for setting up the context and then cleaning up after the block of code has been executed. The two essential methods a context manager must implement are:
__enter__(self)
: This method is called when the execution flow enters the context of thewith
statement. It can return a value that is bound to the variable following theas
keyword.__exit__(self, exc_type, exc_value, traceback)
: This method is called when the execution flow leaves the context of thewith
statement. It handles the cleanup, such as closing files or releasing locks. The parameters help handle exceptions that may have been raised within the block.
The Power of the with
Statement
The with
statement simplifies resource management by automatically handling the setup and teardown tasks defined in the context manager. Here's a basic example using a file:
In this example, the open()
function returns a file object, which acts as a context manager. The file is automatically closed when the block of code is exited, even if an error occurs.
Creating Custom Context Managers
While Python provides built-in context managers for many tasks, you can also create custom ones using either classes or the contextlib
module. Here's an example using a class:
This custom context manager opens a file for writing and ensures it is closed properly after the block is executed.
The contextlib
Module
Python's contextlib
module provides utilities to simplify the creation of context managers. For instance, the @contextmanager
decorator allows you to write a generator-based context manager, which can be more concise:
Subscribe to my newsletter
Read articles from Shrey Dikshant directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Shrey Dikshant
Shrey Dikshant
Aspiring data scientist with a strong foundation in adaptive quality techniques. Gained valuable experience through internships at YT Views, focusing on operation handling. Proficient in Python and passionate about data visualization, aiming to turn complex data into actionable insights.