Best Practices for Writing Clean and Maintainable Code
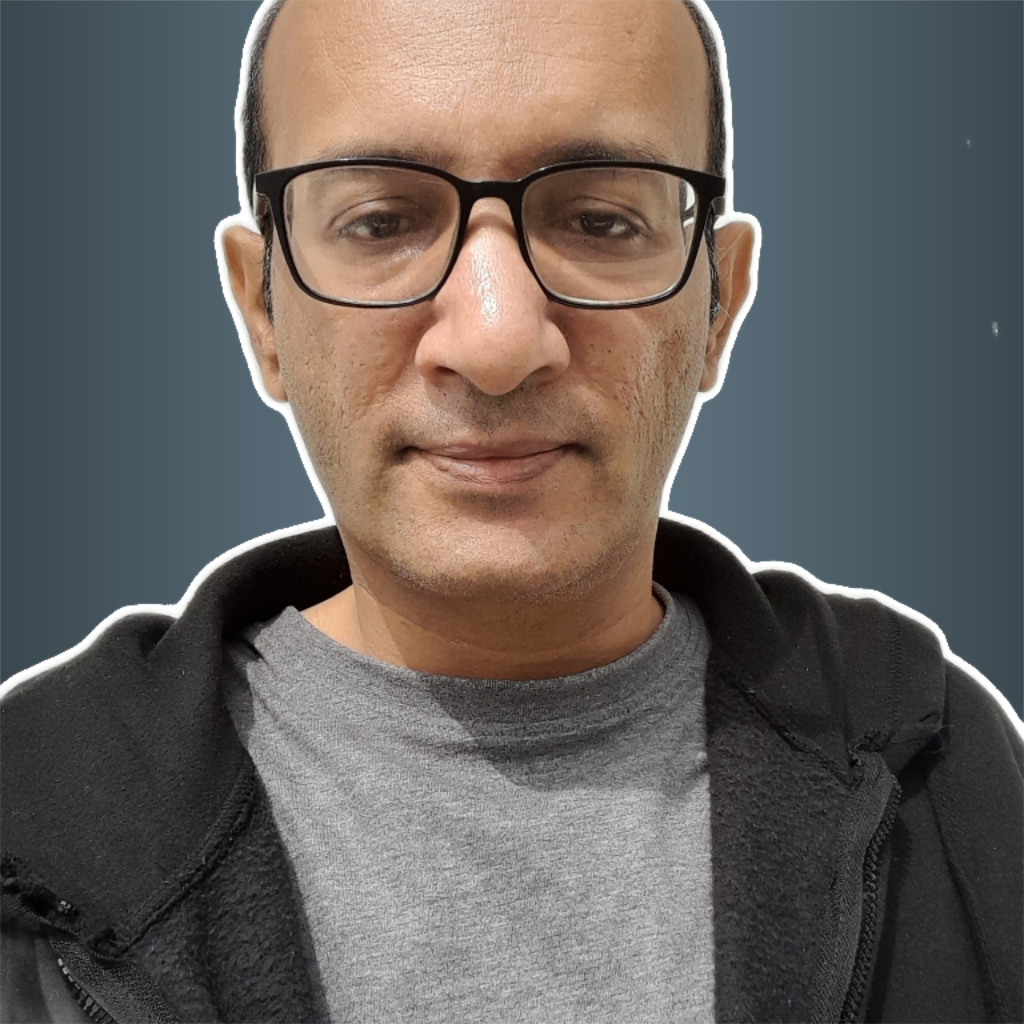
Table of contents
- 1. Follow a Consistent Coding Style
- 2. Write Meaningful Comments
- 3. Keep It Simple (KISS Principle)
- 4. DRY (Don’t Repeat Yourself)
- 5. Write Modular Code
- 6. Prioritize Readability
- 7. Write Unit Tests
- 8. Use Version Control
- 9. Refactor Regularly
- 10. Document Your Code
- Conclusion: Clean Code Leads to Better Software
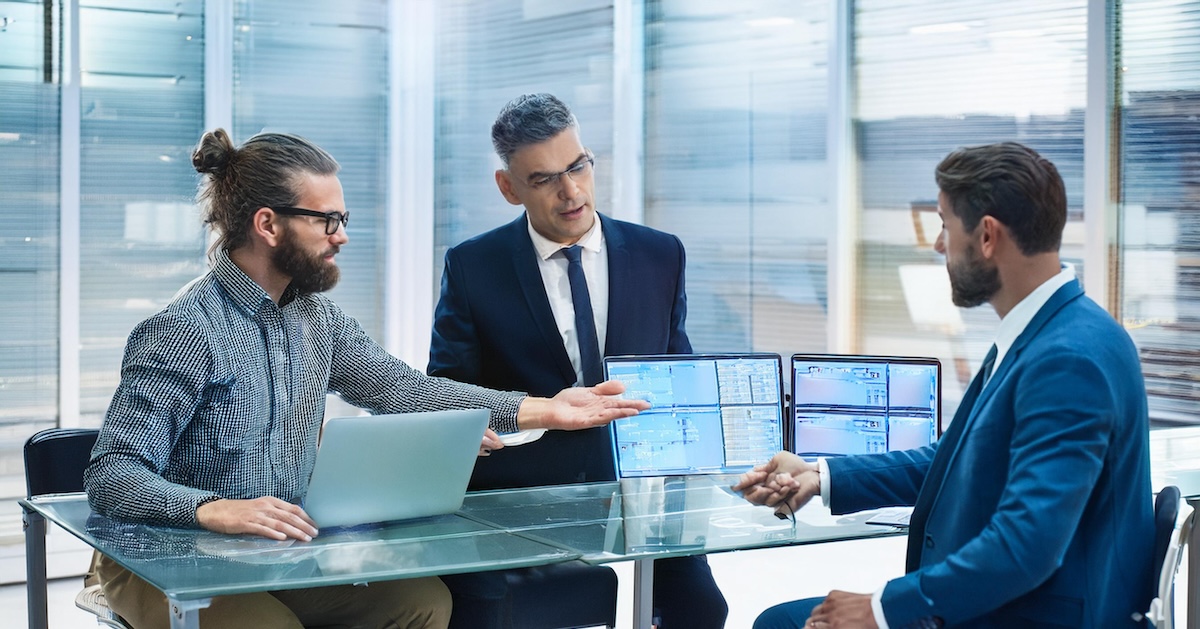
Writing clean and maintainable code is essential for any software engineer. Code that is well-organized, easy to understand, and simple to modify not only reduces the risk of bugs but also makes it easier for other developers to collaborate on the project. In this article, we’ll explore the best practices for writing code that stands the test of time.
1. Follow a Consistent Coding Style
Overview: A consistent coding style ensures that your code is uniform and predictable, making it easier to read and understand.
Best Practices:
Adopt a Style Guide: Use established style guides for your programming language, such as Google’s JavaScript Style Guide or PEP 8 for Python.
Consistent Naming Conventions: Use meaningful and consistent names for variables, functions, and classes. For example, use camelCase for variables and functions, and PascalCase for classes.
Indentation: Use consistent indentation, such as 2 or 4 spaces, to structure your code visually. Avoid mixing tabs and spaces.
Line Length: Keep line lengths within a reasonable limit (e.g., 80-100 characters) to make the code easier to read on various devices.
2. Write Meaningful Comments
Overview: Comments are essential for explaining the purpose of your code, especially in complex or non-obvious sections.
Best Practices:
Comment Why, Not What: Focus on explaining why the code is written a certain way, rather than what the code does, as the code itself should be self-explanatory.
Avoid Over-Commenting: Too many comments can clutter the code and make it harder to read. Comment only when necessary.
Keep Comments Up to Date: Ensure that comments are accurate and reflect the current state of the code. Outdated comments can be misleading.
3. Keep It Simple (KISS Principle)
Overview: Simplicity is key to writing clean and maintainable code. The simpler the code, the easier it is to understand, test, and modify.
Best Practices:
Avoid Over-Engineering: Don’t add unnecessary complexity or features. Implement only what is required.
Break Down Complex Problems: Divide complex problems into smaller, manageable functions or modules.
Use Clear and Simple Logic: Avoid convoluted logic and strive for straightforward solutions.
4. DRY (Don’t Repeat Yourself)
Overview: The DRY principle encourages the reduction of code duplication by promoting reuse.
Best Practices:
Extract Reusable Code: Identify common patterns in your code and extract them into reusable functions, classes, or modules.
Use Inheritance and Composition: In object-oriented programming, use inheritance or composition to reuse code across different classes.
Centralize Configuration: Keep configuration settings, constants, and magic numbers in one place to avoid repetition.
5. Write Modular Code
Overview: Modular code is organized into small, independent units (modules) that can be developed, tested, and maintained separately.
Best Practices:
Single Responsibility Principle: Each module or function should have one specific responsibility. This makes the code easier to understand and maintain.
Encapsulation: Encapsulate related functionality within classes or modules, exposing only what is necessary.
Loose Coupling: Minimize dependencies between modules to make them more reusable and easier to change independently.
6. Prioritize Readability
Overview: Code readability is crucial for collaboration and long-term maintenance. Code that is easy to read is also easier to debug and enhance.
Best Practices:
Descriptive Names: Use descriptive names for variables, functions, and classes that clearly convey their purpose.
Avoid Obscure Abbreviations: Use full words instead of abbreviations that may not be immediately understood by others.
Consistent Formatting: Apply consistent formatting throughout your codebase to improve readability.
7. Write Unit Tests
Overview: Unit tests validate that individual components of your code work as expected. Writing tests ensures that your code is reliable and reduces the chances of introducing bugs when making changes.
Best Practices:
Test-Driven Development (TDD): Consider writing tests before implementing the actual code to ensure it meets the requirements.
Aim for High Test Coverage: Ensure that your tests cover a significant portion of your code, especially critical and complex areas.
Automate Testing: Use continuous integration tools to automate the running of tests whenever code changes are made.
8. Use Version Control
Overview: Version control systems like Git are essential for tracking changes, collaborating with other developers, and managing code versions.
Best Practices:
Commit Frequently: Make small, frequent commits with clear, descriptive messages.
Use Branches: Use branches to work on features, bugs, or experiments independently, and merge them into the main codebase when they are ready.
Code Reviews: Regularly review code with your team to maintain quality and consistency.
9. Refactor Regularly
Overview: Refactoring involves improving the structure of your code without changing its functionality. It helps in keeping the codebase clean, efficient, and easier to maintain.
Best Practices:
Identify Code Smells: Look for signs of poor design, such as large classes, long functions, or duplicated code, and refactor them.
Incremental Refactoring: Refactor small parts of your code regularly rather than trying to overhaul the entire codebase at once.
Maintain Functionality: Ensure that refactoring doesn’t introduce bugs by running tests before and after the refactoring process.
10. Document Your Code
Overview: Documentation provides a reference for others (and yourself) to understand how to use and extend your code.
Best Practices:
API Documentation: Clearly document the interfaces, functions, and methods available in your codebase, including their expected inputs and outputs.
Installation and Setup Guides: Provide instructions for setting up the development environment, dependencies, and configuration.
Keep Documentation Updated: Ensure that documentation is kept up to date with the latest changes in the code.
Conclusion: Clean Code Leads to Better Software
Writing clean and maintainable code is an investment that pays off in the long run. It reduces the risk of bugs, makes your code easier to understand, and facilitates collaboration with other developers. By following these best practices, you’ll create a codebase that is not only functional but also a pleasure to work with.
Start incorporating these practices into your daily coding routine, and you’ll notice improvements in the quality and longevity of your software projects.
Subscribe to my newsletter
Read articles from Azhar Hussain directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
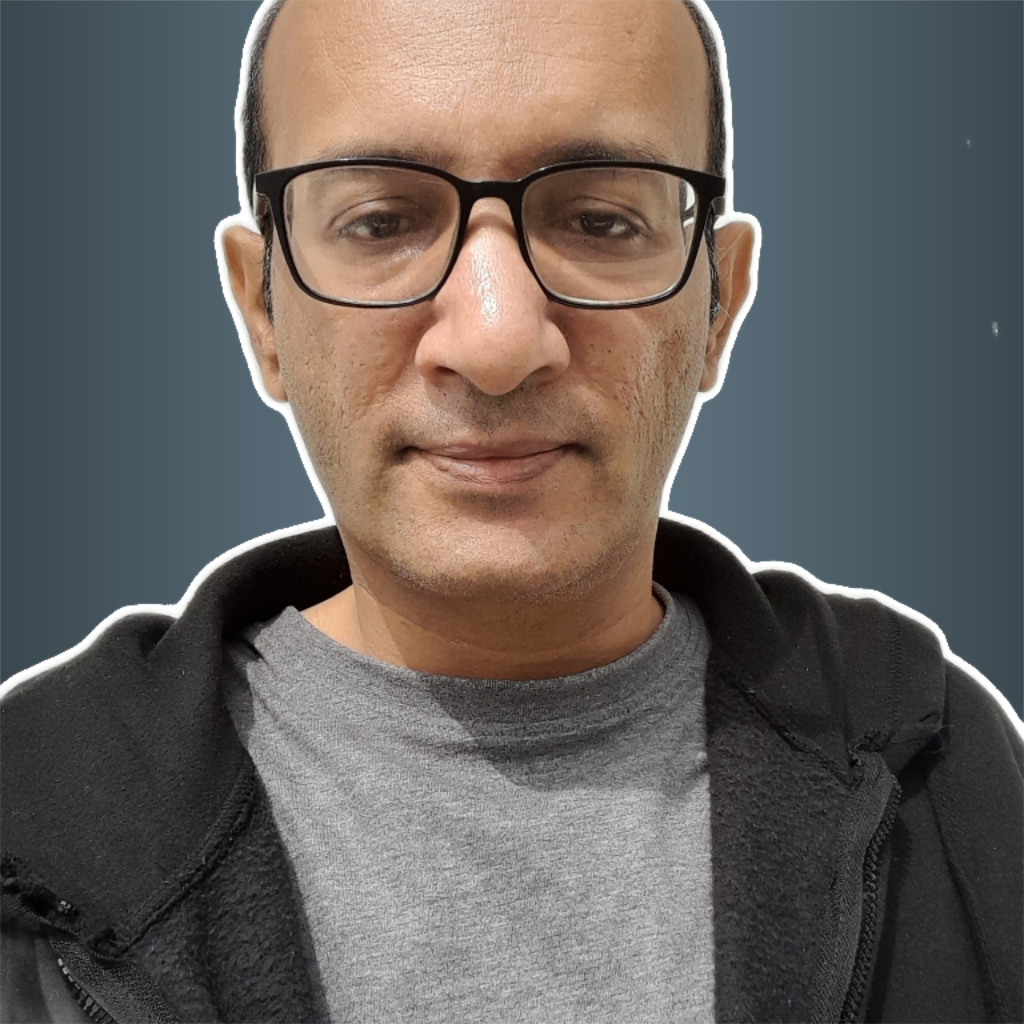
Azhar Hussain
Azhar Hussain
Over 2 decades of software engineering experience, including over a decade in building, scaling and leading engineering teams.