Merge Sort
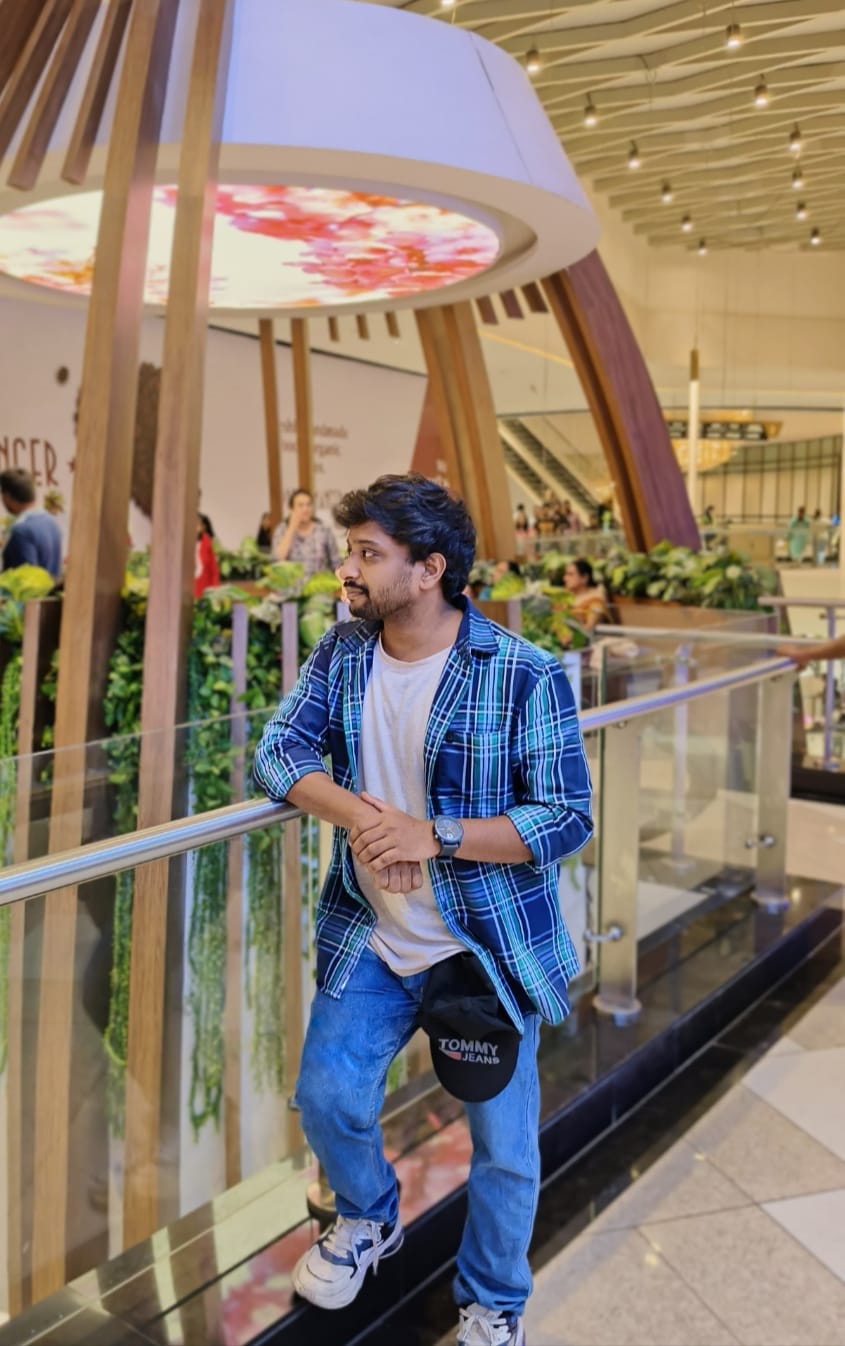
1 min read
Table of contents
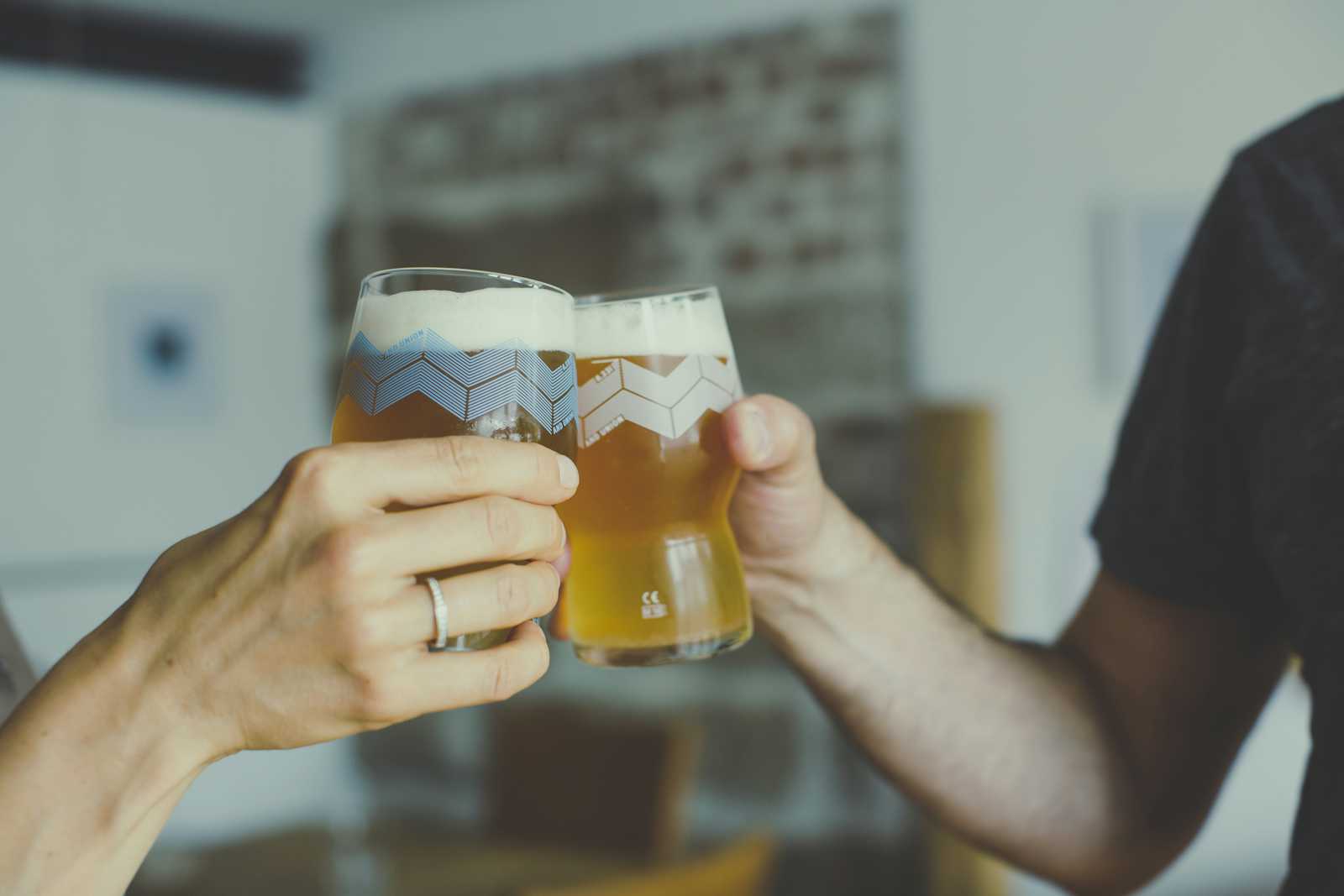
Merge sort divides the array into two halves, recursively sorts each half, and then merges them back together in sorted order.
It follows the divide-and-conquer approach, achieving a time complexity of
O(nlogn)
.
Code
function mergeSort(arr) {
const length = arr.length;
if (length <= 1) {
return arr;
}
const mid = Math.floor(length / 2);
const leftArr = arr.slice(0, mid);
const rightArr = arr.slice(mid);
return merge(mergeSort(leftArr), mergeSort(rightArr));
}
function merge(leftArr, rightArr) {
const sortedArr = [];
while (leftArr.length && rightArr.length) {
if (leftArr[0] <= rightArr[0]) {
sortedArr.push(leftArr.shift());
} else {
sortedArr.push(rightArr.shift());
}
}
return [...sortedArr, ...leftArr, ...rightArr];
}
console.log(mergeSort([6, 5, 12, 10, 9, 1]));
0
Subscribe to my newsletter
Read articles from Akshaya Biswal directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
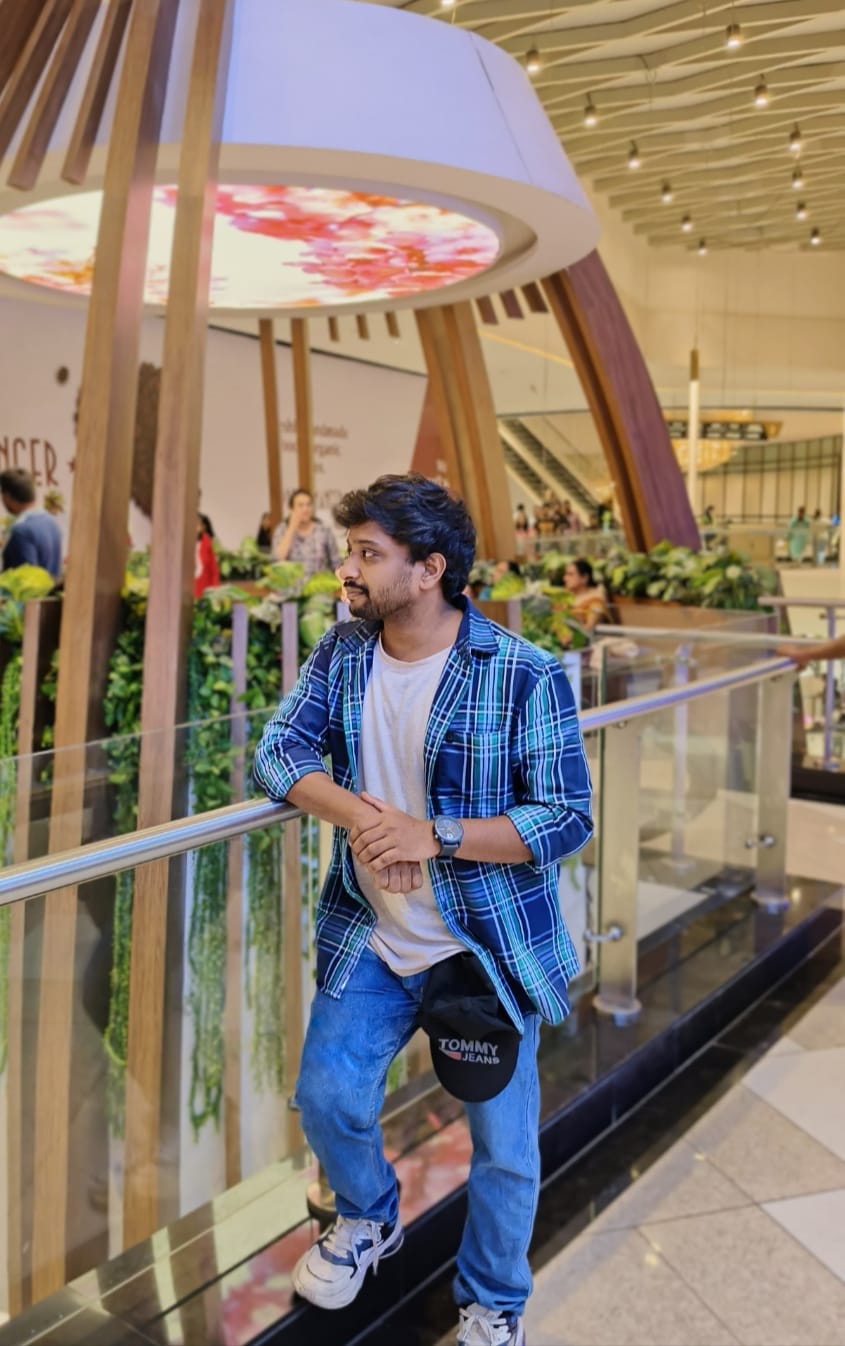
Akshaya Biswal
Akshaya Biswal
Developer by day, globe-trotter by choice. Whether fixing bugs in the mountains or optimizing on the beach, each journey enhances both my code and life.๐โ๏ธ