Mastering Git: A Beginner's Journey to Seamless Version Control
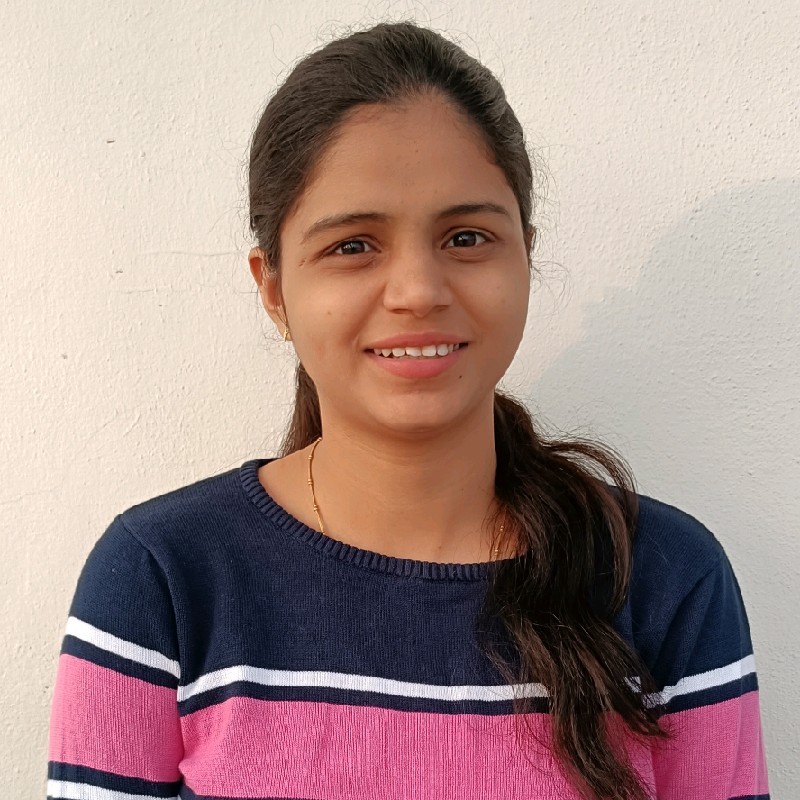
Git is an essential tool for managing and tracking changes in your code. This guide will introduce you to Git’s core concepts using a straightforward example: managing a personal project called "MyRecipeBook." We’ll walk through practical examples to help you grasp Git’s functionality and understand how it can streamline your development workflow.
Why Git? Understanding the Need
Imagine you're working on a project like "MyRecipeBook," which involves keeping track of various recipes and changes over time. Without a version control system like Git, managing updates, collaborating with others, and keeping track of different versions can become chaotic. Git helps solve these problems by:
• Tracking Changes: Git keeps a history of all changes, allowing you to review past modifications and revert to previous states if needed.
• Branching: Git allows you to create separate branches for different features or fixes, so you can work on updates without affecting the main project.
• Merging: Git helps integrate changes from different branches, ensuring that all updates are combined seamlessly.
For instance, if you and a friend are both working on different recipes, Git ensures that both of your changes are tracked and merged properly.
Why Git is Popular?
Git’s popularity stems from its speed, flexibility, and robustness. Unlike older version control systems, Git is designed to handle large projects efficiently and supports distributed development. Here’s why Git stands out:
• Speed: Git performs operations like committing changes and switching branches quickly.
• Distributed Nature: Each user has a full copy of the repository, allowing for offline work and faster access to history.
• Branching and Merging: Git’s powerful branching and merging capabilities enable complex workflows and collaborative development.
For example, with "MyRecipeBook," Git allows you to experiment with new recipes or formatting changes on separate branches without disrupting the main collection.
Git vs. GitHub
While Git manages versions and tracks changes on your local machine, GitHub is a platform that hosts Git repositories online. GitHub provides additional features like:
• Collaborative Tools: Issue tracking, pull requests, and code reviews.
• Remote Access: Share your project with others and collaborate online.
For instance, you use Git to manage "MyRecipeBook" locally, while GitHub allows you to publish it, collaborate with others, and track progress from anywhere.
Master, Feature, and Release Branches
Git organizes development through branches, each serving a specific purpose:
• Master Branch: The main (or master) branch contains the stable, production-ready code. In "MyRecipeBook," this branch holds the finalized version of your recipes.
• Feature Branch: Used to develop new features or make changes. For example, you might create a feature-new-recipe branch to add a new recipe format.
• Release Branch: Prepares the project for a new release. For "MyRecipeBook," a release-v1.0 branch would be used to finalize and test all changes before publishing.
Setting Up Your Git Repository
To start using Git with your "MyRecipeBook" project, follow these steps:
• Initialize Git: Open your terminal, navigate to your project folder, and run:
-git init
This command creates a hidden. git directory, marking your project as a Git repository.
Basic Git Commands Overview
Here’s how to use Git commands to manage your project:
• Check Status:
-git status
This command shows which files are staged for commit, modified, or untracked.
• Stage Changes:
-git add filename.txt
or to stage all changes:
-git add .
Staging prepares files for committing.
• Commit Changes:
-git commit -m "Your message"
This command saves a snapshot of your project with a descriptive message.
• Push Changes:
-git push
Uploads your local commits to a remote repository on GitHub.
• Pull Updates:
-git pull
Updates your local branch with changes from the remote repository
Git Configuration
Before using Git, configure your user details:
1. Set User Information:
git config --global
user.name
"Your Name"
git config --global
user.email
"
you@example.com
"
This ensures commits are correctly attributed to you.
Cloning a Repository
To work on an existing project, clone it:
2. Clone Repository:
git clone
This copies the repository to your local machine.
Understanding .gitignore
Exclude certain files from version control by adding patterns to a .gitignore file:
3. Add Patterns:
node_modules/
*.log
This prevents unnecessary files from being tracked.
Tagging
Label important commits with tags:
4. Create a Tag:
git tag v1.0
Tags help mark significant milestones, like version releases.
Branch Management
Manage branches for different development stages:
5. Create a Branch:
git branch new-feature
6. Switch Branches:
git checkout new-feature
7. Delete a Branch:
git branch -d old-branch
Organize and manage various lines of development effectively.
Reverting Changes
Undo modifications or roll back commits:
8. Undo Local Changes:
git checkout -- file.txt
9. Revert a Commit:
git revert <commit-id>
Reverting helps correct mistakes or unwanted changes.
Using Git Merge Tools
Graphical merge tools help resolve conflicts:
- Use a Merge Tool:
Tools like KDiff3 or Meld offer visual interfaces for managing conflicts during merges.
Git Workflow Strategies
Different workflows help manage development:
•Git Flow: Includes branches for features, releases, and hotfixes.
•GitHub Flow: Simplified workflow focusing on feature branches and pull requests.
Collaborative Workflows
Collaborate using forks and pull requests:
Fork a Repository: Create a personal copy on GitHub.
Submit a Pull Request: Propose changes to the original repository.
Review Code: Collaborate on and discuss proposed changes
Using Git with IDEs
Integrate Git with IDEs like VS Code or IntelliJ IDEA to streamline version control within your development environment.
Security Practices
Manage sensitive data and use access controls to protect your repositories.
In this guide, we’ve explored the fundamental concepts of Git through practical examples from managing a personal project, "MyRecipeBook." We covered the essentials of Git’s functionality, including how to track changes, manage branches, and collaborate with others. By understanding these concepts and applying them to your projects, you can efficiently manage code changes and streamline your development workflow.
Whether you're working alone or as part of a team, Git offers a robust solution for tracking and integrating changes. With this guide, you're now equipped to start using Git effectively, ensuring your projects are well-organized, and your development process is smooth.
Subscribe to my newsletter
Read articles from Kajal Patil directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
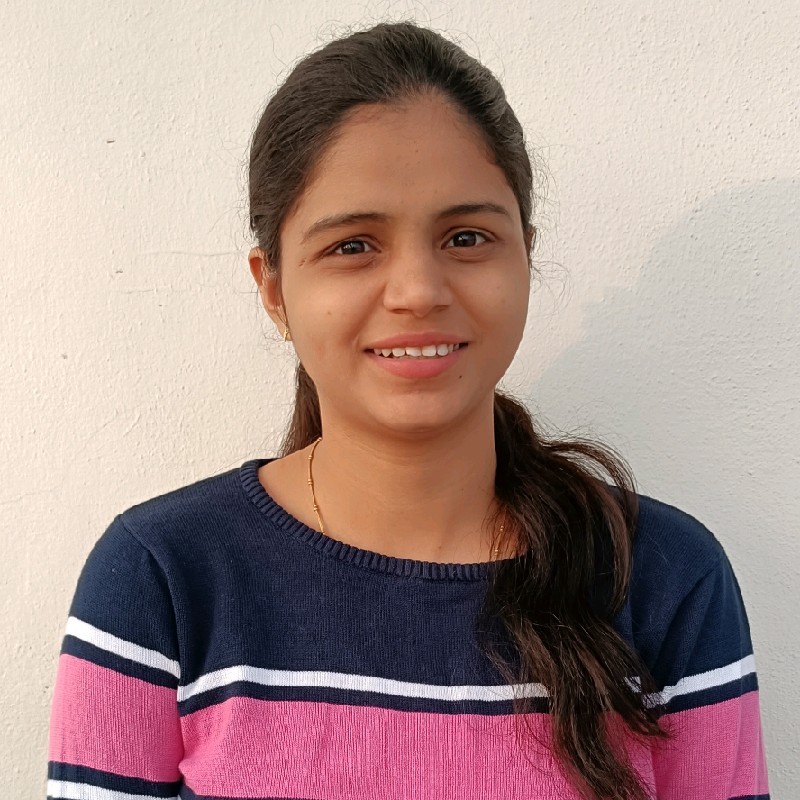