6 Beginner Projects to Master React's useState Hook
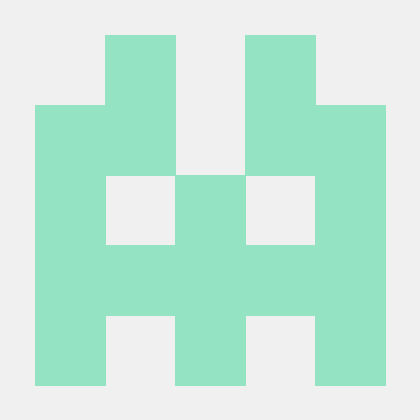
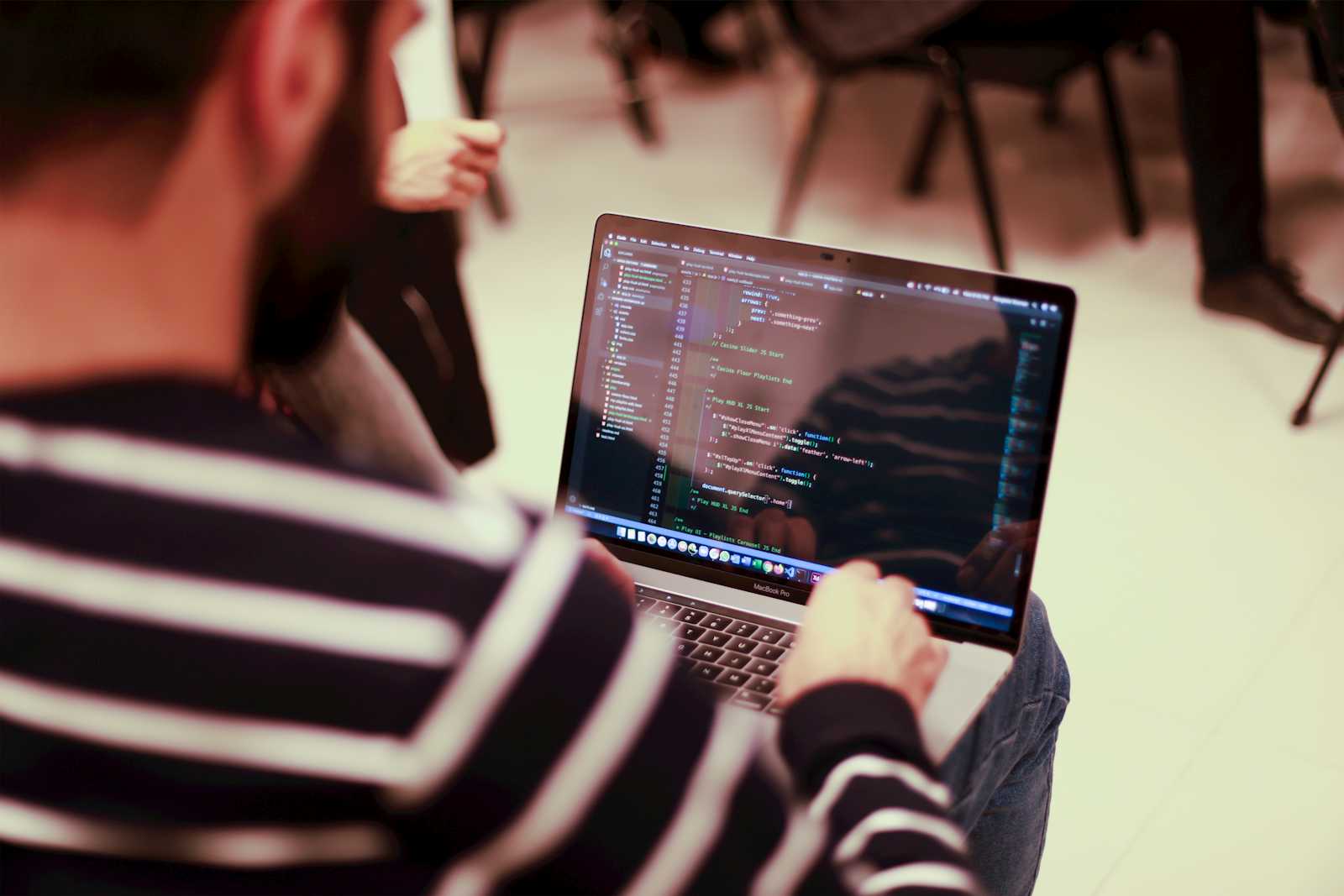
Introduction
When you’re learning React, one of the first things you’ll come across is the useState
hook. It’s super important for managing state in your components, and getting the hang of it will really boost your React skills. But instead of just reading about it, why not dive in with some hands-on projects?
In this blog post, I’ll guide you through seven beginner-friendly projects that will help you get comfortable with useState
. Whether you’re just starting out or want to practice more, these projects are a great way to learn by doing.
What is UseState?
Before we start with the projects, let’s quickly go over what useState
is. In React, "state" is just a fancy word for variables that tell your component how to behave or look. The useState
hook lets you add state to your function components, which wasn’t possible before React introduced hooks.
Here’s a simple example to show you how it works:
import React, { useState } from 'react';
function Counter() {
const [count, setCount] = useState(0);
return (
<div>
<p>You clicked {count} times</p>
<button onClick={() => setCount(count + 1)}>
Click me
</button>
</div>
);
}
In this example, useState(0)
sets up a state variable called count
with an initial value of 0
. When you click the button, setCount
updates the count, and React re-renders the component with the new count.
Project 1: Simple Counter
Project Overview
This is a basic project where you’ll create a simple counter. It’s a great way to start using the useState
hook.
How to Build It
Create a React component called
Counter
.Use
useState
to set up a state variable calledcount
with a starting value of0
.Add a button that increases the count by 1 when clicked.
Add a button that decreases the count by 1
Add a Reset button to change the count to 0
Display the current count on the screen.
Code Example
import React, { useState } from 'react'
function CounterApp() {
const [count, setCount] = useState(0);
return (
<div>
<h1>{count}</h1>
<button onClick={() => {setCount(count+1)}}>+</button>
<button onClick={() => setCount(count-1)}>-</button>
<button onClick={() => setCount(0)}>Reset</button>
</div>
)
}
export default CounterApp
What’s Happening Here?
In this project, the useState
hook is used to create and manage a count
state variable. The setCount
function is used to update the count
state whenever a button is clicked. The UI automatically reflects these changes because React re-renders the component whenever the state changes. The "+" button increments the count, the "-" button decrements it, and the "Reset" button resets the count back to 0.
Project 2: Toggle Button
Project Overview
Next, let’s create a toggle button that switches between "ON" and "OFF." This project will show you how to use useState
to toggle between two states.
How to Build It
Create a React component called
ToggleButton
.Use
useState
to set up a state variable calledisOn
, starting withfalse
.Add a button that toggles between
true
andfalse
when clicked.Display "ON" when
isOn
is true and "OFF" whenisOn
is false.
Code Example
import React, { useState } from 'react';
function ToggleButton() {
const [isOn, setIsOn] = useState(false);
return (
<button onClick={() => setIsOn(!isOn)}>
{isOn ? 'ON' : 'OFF'}
</button>
);
}
What’s Happening Here?
The isOn
state keeps track of whether the button is "ON" or "OFF." Clicking the button flips the state between true
and false
, and the button text updates accordingly.
Project 3: Simple To-Do List
Project Overview
In this project, you'll create a simple to-do list where users can add and remove tasks. This introduces managing an array with useState
.
How to Build It
Create a React component called
ToDoList
.Use
useState
to manage an array of tasks.Add an input field and a button to add new tasks to the list.
Display the list of tasks with a button to remove each task.
Code Example
import React, { useState } from 'react';
function ToDoList() {
// State to manage the list of todos
const [todos, setTodos] = useState([]);
// State to manage the value of the input field
const [inputValue, setInputValue] = useState('');
// Function to add a new todo item to the list
const addTodos = () => {
// Prevent adding an empty todo
if(inputValue.trim() === '') return;
// Create a new todo object
const newTodo = {
todo: inputValue, // The text of the todo
id: todos.length + 1 // Unique ID for the todo
};
// Update the todos state with the new todo
setTodos([...todos, newTodo]);
// Clear the input field after adding the todo
setInputValue('');
};
// Function to delete a todo item by its ID
const deleteTodo = (id) => {
// Filter out the todo that matches the provided ID and update the state
setTodos(todos.filter((todo) => todo.id !== id));
};
return (
<div>
<h1>Todo App</h1>
{/* Input field to enter a new todo */}
<input
type="text"
value={inputValue}
onChange={(e) => setInputValue(e.target.value)}
/>
{/* Button to add the todo to the list */}
<button onClick={addTodos}>ADD</button>
{/* Display the list of todos */}
<div>
<ul>
{/* Loop through the todos and render each one */}
{todos.map((t) => (
<li key={t.id}>
{/* Display the text of the todo */}
{t.todo}
{/* Button to delete the todo */}
<button onClick={() => deleteTodo(t.id)}>delete</button>
</li>
))}
</ul>
</div>
</div>
);
}
What’s Happening Here?
Here, useState
is used to manage the list of tasks and the current input value. When you add a new task, it’s added to the todos
array. You can also remove tasks from the list.
Project 4: Form Input Handler
Project Overview
In this project, you’ll build a simple form that captures user input, like a name and email, and displays it on the screen.
How to Build It
Create a React component called
FormInput
.Use
useState
to manage the state for each input field.Add input fields for name and email.
Display the input values below the form.
Code Example
import React, { useState } from 'react';
function FormInput() {
// State variables to store the name and email values
const [name, setName] = useState('');
const [email, setEmail] = useState('');
return (
<div>
{/* Input field for the user's name */}
<input
type="text"
value={name}
onChange={(e) => setName(e.target.value)} // Updates the name state on input change
placeholder="Name"
/>
{/* Input field for the user's email */}
<input
type="email"
value={email}
onChange={(e) => setEmail(e.target.value)} // Updates the email state on input change
placeholder="Email"
/>
{/* Displaying the entered name */}
<p>Name: {name}</p>
{/* Displaying the entered email */}
<p>Email: {email}</p>
</div>
);
}
export default FormInput;
What’s Happening Here?useState
manages the state of the name and email input fields. As you type, the state updates, and the current values are displayed below the input fields.
Project 5: Image Carousel
Project Overview
In this project, you’ll create an image carousel that allows users to navigate through a set of images. This is a great way to practice using useState
to manage which image is currently being displayed.
How to Build It
Create a React component called
ImageCarousel
.Use
useState
to manage the index of the currently displayed image.Create an array of image URLs that will be displayed in the carousel.
Add buttons to navigate to the previous and next images.
Display the image based on the current index.
Code Example
import React, { useState } from 'react';
function ImageCarousel() {
// Array of image URLs for the carousel
const images = [
'https://images.unsplash.com/photo-1723098232760-379e763a3f9b?q=80&w=1931&auto=format&fit=crop&ixlib=rb-4.0.3&ixid=M3wxMjA3fDB8MHxwaG90by1wYWdlfHx8fGVufDB8fHx8fA%3D%3D',
'https://images.unsplash.com/photo-1722218424133-30c28ad4fd42?q=80&w=2070&auto=format&fit=crop&ixlib=rb-4.0.3&ixid=M3wxMjA3fDB8MHxwaG90by1wYWdlfHx8fGVufDB8fHx8fA%3D%3D',
'https://images.unsplash.com/photo-1723053425944-838d7501ebfb?q=80&w=2070&auto=format&fit=crop&ixlib=rb-4.0.3&ixid=M3wxMjA3fDB8MHxwaG90by1wYWdlfHx8fGVufDB8fHx8fA%3D%3D',
'https://images.unsplash.com/photo-1722218424232-14a9d128524f?q=80&w=2071&auto=format&fit=crop&ixlib=rb-4.0.3&ixid=M3wxMjA3fDB8MHxwaG90by1wYWdlfHx8fGVufDB8fHx8fA%3D%3D',
];
// State to keep track of the current image index
const [index, setIndex] = useState(0);
// Function to go to the next image in the carousel
const nextImage = () => {
// If we're at the last image, wrap around to the first image
if(index + 1 > images.length - 1) {
setIndex(0);
} else {
// Otherwise, go to the next image
setIndex(index + 1);
}
};
// Function to go to the previous image in the carousel
const prevImage = () => {
// If we're at the first image, wrap around to the last image
if(index - 1 < 0) {
setIndex(images.length - 1);
} else {
// Otherwise, go to the previous image
setIndex(index - 1);
}
};
return (
<div>
{/* Display the current image */}
<img
src={images[index]}
alt="Carousel Image"
style={{ width: '600px', height: '300px' }}
/>
{/* Navigation buttons */}
<div style={{ marginTop: '20px' }}>
<button onClick={prevImage}>Previous</button>
<button onClick={nextImage} style={{ marginLeft: '10px' }}>Next</button>
</div>
</div>
);
}
export default ImageCarousel;
What’s Happening Here?
State Management:
useState
is used to keep track of theindex
, which determines which image is shown.Image Array: The images are stored in an array, and the
index
is used to access the appropriate image.Navigation: The "Previous" and "Next" buttons update the
index
, looping back to the start or end of the array as needed.
This project will help you understand how to use useState
to control which item in a list is displayed, and how to create dynamic, interactive components in React.
Project 6: Theme Switcher
Project Overview
In this project, you’ll build a simple theme switcher that allows users to toggle between light and dark modes. This project will help you understand how to use useState
to manage UI state and apply conditional styles based on the current theme.
How to Build it
Create a React component called
ThemeSwitcher
.Use
useState
to manage the current theme (light or dark).Add a button to toggle between the themes.
Apply different styles based on the selected theme, such as changing the background and text colors.
Code Example
import React, { useState } from 'react';
function ThemeSwitcher() {
// State to track whether the current theme is dark or light
const [isDarkTheme, setIsDarkTheme] = useState(false);
// Function to toggle between dark and light themes
const toggleTheme = () => {
// Update the state to the opposite of its current value
setIsDarkTheme((prevTheme) => !prevTheme);
};
// Styles that will be applied to the div based on the current theme
const themeStyles = {
backgroundColor: isDarkTheme ? '#333' : '#FFF', // Dark background for dark theme, light background for light theme
color: isDarkTheme ? '#FFF' : '#000', // White text for dark theme, black text for light theme
padding: '20px',
textAlign: 'center'
};
return (
<div style={themeStyles}>
{/* Display the current theme */}
<h1>{isDarkTheme ? 'Dark Theme' : 'Light Theme'}</h1>
{/* Button to switch between themes */}
<button onClick={toggleTheme}>
Switch to {isDarkTheme ? 'Light' : 'Dark'} Theme
</button>
</div>
);
}
export default ThemeSwitcher;
What’s Happening Here?
State Management: The
useState
hook is used to keep track of whether the dark theme is active (isDarkTheme
).Theme Toggle: The
toggleTheme
function switches the theme by toggling the value ofisDarkTheme
betweentrue
andfalse
.Dynamic Styling: The
themeStyles
object contains styles that change based on the value ofisDarkTheme
, adjusting the background and text colors accordingly.
This project will give you a solid understanding of how to use useState
for managing UI state and applying conditional styling in React. It's a simple but powerful way to enhance user experience by allowing theme customization.
How have these projects impacted your understanding of useState? Are there any specific use cases you'd like to explore further? Share your thoughts in the comments below!
Subscribe to my newsletter
Read articles from KodaKodama directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
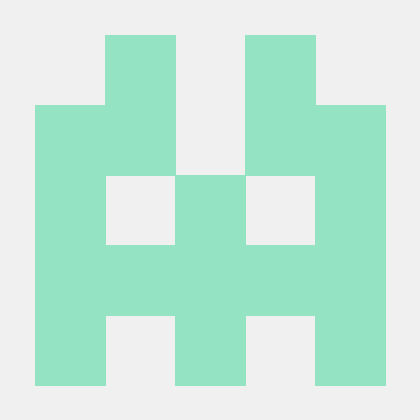