One-Hot Encoding vs. Ordinal Encoding: Which Is Best for Machine Learning?
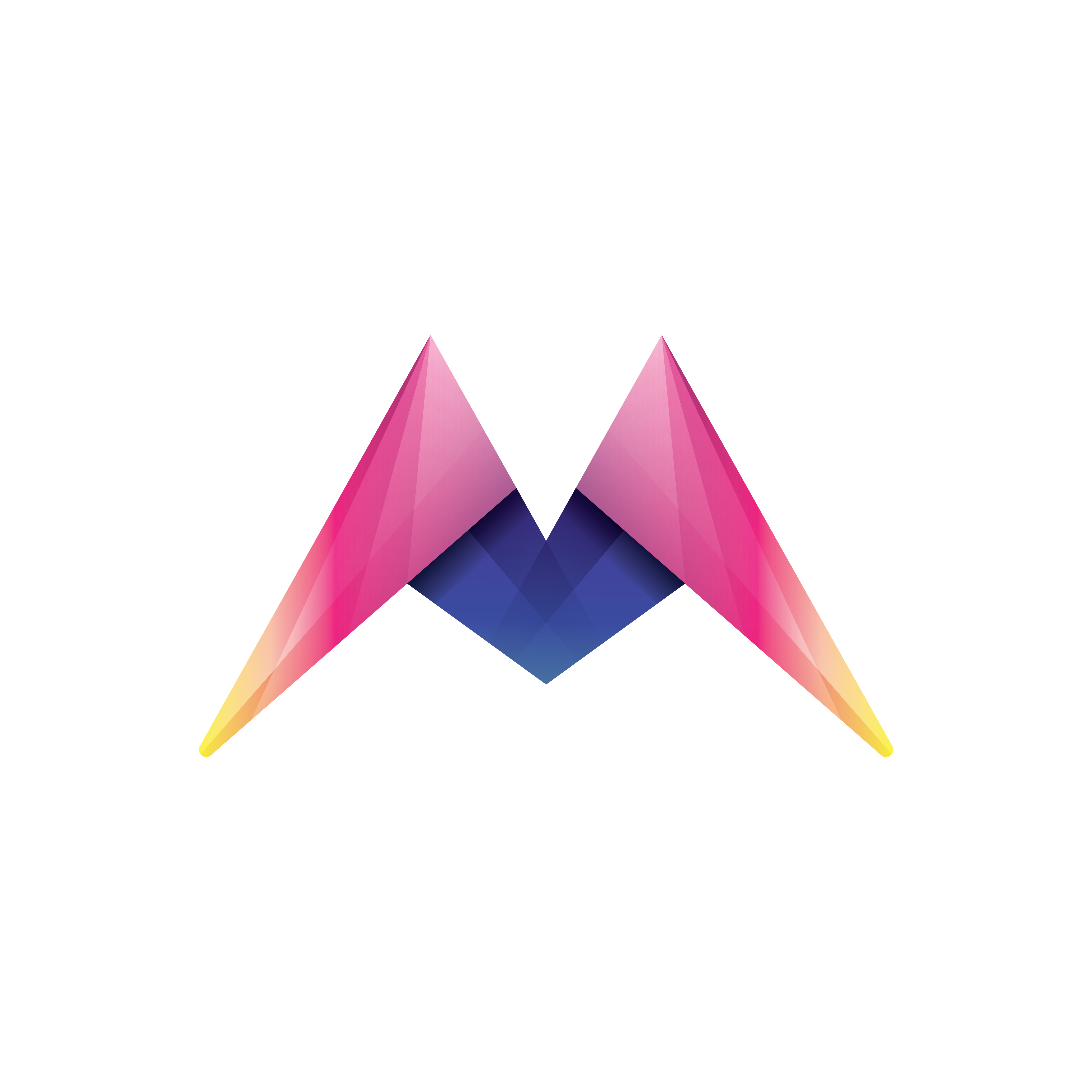
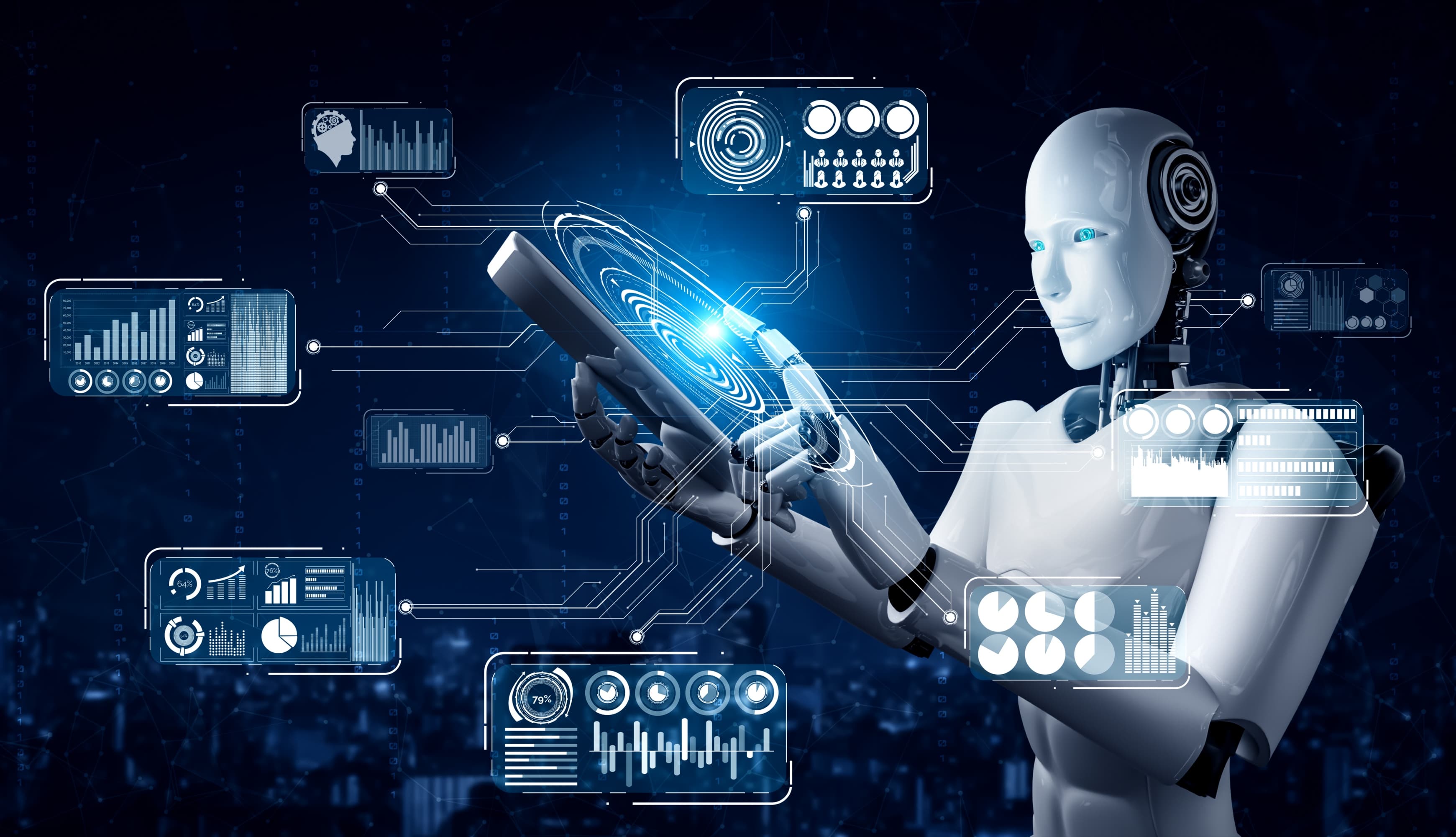
When working with machine learning models, one of the crucial steps in data preprocessing is encoding categorical variables. Categorical data, which represents categories or groups, needs to be converted into a numerical format that algorithms can process. Two popular methods for this conversion are one-hot encoding and ordinal encoding. In this blog post, we will explore these techniques in detail and demonstrate how to implement them using Scikit-Learn in Python, with a special focus on an example involving army rankings.
What is Categorical Data?
Categorical data refers to variables that represent distinct categories or groups. Examples include colors (e.g., red, green, blue), countries (e.g., USA, Canada, Mexico), or customer feedback (e.g., satisfied, neutral, dissatisfied). Machine learning models typically require numerical input, so categorical data must be transformed.
1. One-Hot Encoding
What is One-Hot Encoding?
One-hot encoding is a method where each category is converted into a binary vector. Each category is represented by a column, and the presence of a category is indicated by a 1, while other categories are marked with 0s. This method is ideal for nominal data where there is no inherent order among the categories.
Code:
Let’s see how to perform one-hot encoding using Scikit-Learn:
import numpy as np
from sklearn.preprocessing import OneHotEncoder
import pandas as pd
# Sample data
data = np.array(['Red', 'Green', 'Blue', 'Green', 'Red']).reshape(-1, 1)
# Initialize the OneHotEncoder
encoder = OneHotEncoder(sparse=False)
# Fit and transform the data
one_hot_encoded = encoder.fit_transform(data)
# Convert the result to a DataFrame for better readability
df_one_hot = pd.DataFrame(one_hot_encoded, columns=encoder.get_feature_names_out(['Color']))
print("Original Data:")
print(data)
print("\nOne-Hot Encoded Data:")
print(df_one_hot)
Output:
Original Data:
[['Red']
['Green']
['Blue']
['Green']
['Red']]
One-Hot Encoded Data:
Color_Blue Color_Green Color_Red
0 0.0 0.0 1.0
1 0.0 1.0 0.0
2 1.0 0.0 0.0
3 0.0 1.0 0.0
4 0.0 0.0 1.0
Advantages and Disadvantages
Advantages:
No Assumed Ordinal Relationship: Suitable for nominal data where categories don’t have a meaningful order.
Clear Representation: Provides a straightforward, clear representation of categorical data.
Disadvantages:
High Dimensionality: Can lead to a high-dimensional feature space if the categorical variable has many categories.
Sparse Data: The result is a sparse matrix, which may be computationally expensive.
2. Ordinal Encoding
What is Ordinal Encoding?
Ordinal encoding assigns each category a unique integer value based on an inherent order. This method is suitable for categorical data where there is a meaningful ranking or order among the categories.
Army Ranking Example
Consider a variable "Army Rank" with categories representing military ranks. The ranks have a meaningful order: Private, Sergeant, Lieutenant, Captain, Major. We’ll use ordinal encoding to assign numerical values to these ranks.
Code:
import numpy as np
from sklearn.preprocessing import OrdinalEncoder
import pandas as pd
# Sample data
data = np.array(['Private', 'Captain', 'Lieutenant', 'Sergeant', 'Major', 'Private']).reshape(-1, 1)
# Define the order of ranks
categories = [['Private', 'Sergeant', 'Lieutenant', 'Captain', 'Major']]
# Initialize the OrdinalEncoder with the defined order
encoder = OrdinalEncoder(categories=categories)
# Fit and transform the data
ordinal_encoded = encoder.fit_transform(data)
# Convert the result to a DataFrame for better readability
df_ordinal = pd.DataFrame(ordinal_encoded, columns=['Army_Rank'])
print("Original Data:")
print(data)
print("\nOrdinal Encoded Data:")
print(df_ordinal)
Output:
Original Data:
[['Private']
['Captain']
['Lieutenant']
['Sergeant']
['Major']
['Private']]
Ordinal Encoded Data:
Army_Rank
0 0.0
1 3.0
2 2.0
3 1.0
4 4.0
5 0.0
Advantages and Disadvantages
Advantages:
Efficient Representation: Uses a single column for encoding, which reduces dimensionality.
Order Preservation: Captures the ordinal nature of the data, making it suitable when order matters.
Disadvantages:
Assumption of Ordinality: Assumes that the numerical values reflect the order, which might not always be appropriate.
Potential Misinterpretation: Algorithms may interpret numerical values as having arithmetic relationships, which could be misleading.
Choosing the Right Encoding Method
One-Hot Encoding is best for nominal data where there is no intrinsic ordering among categories. It’s ideal when working with algorithms that can handle high dimensionality and sparse data.
Ordinal Encoding is suitable for ordinal data where the order of categories is important. It’s useful for reducing dimensionality but should be used cautiously to avoid misleading the model with numerical values.
Conclusion
Understanding and correctly implementing encoding methods like one-hot and ordinal encoding are essential for preprocessing categorical data in machine learning. By using Scikit-Learn’s OneHotEncoder
and OrdinalEncoder
, you can transform categorical variables into numerical formats that enhance your model’s performance and accuracy.
In this blog, we demonstrated how to apply these techniques with practical examples, including a special case with army rankings. Feel free to experiment with these techniques and adjust them according to the needs of your dataset and machine learning model.
Happy encoding!
Subscribe to my newsletter
Read articles from Mohamed Abubakkar M directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
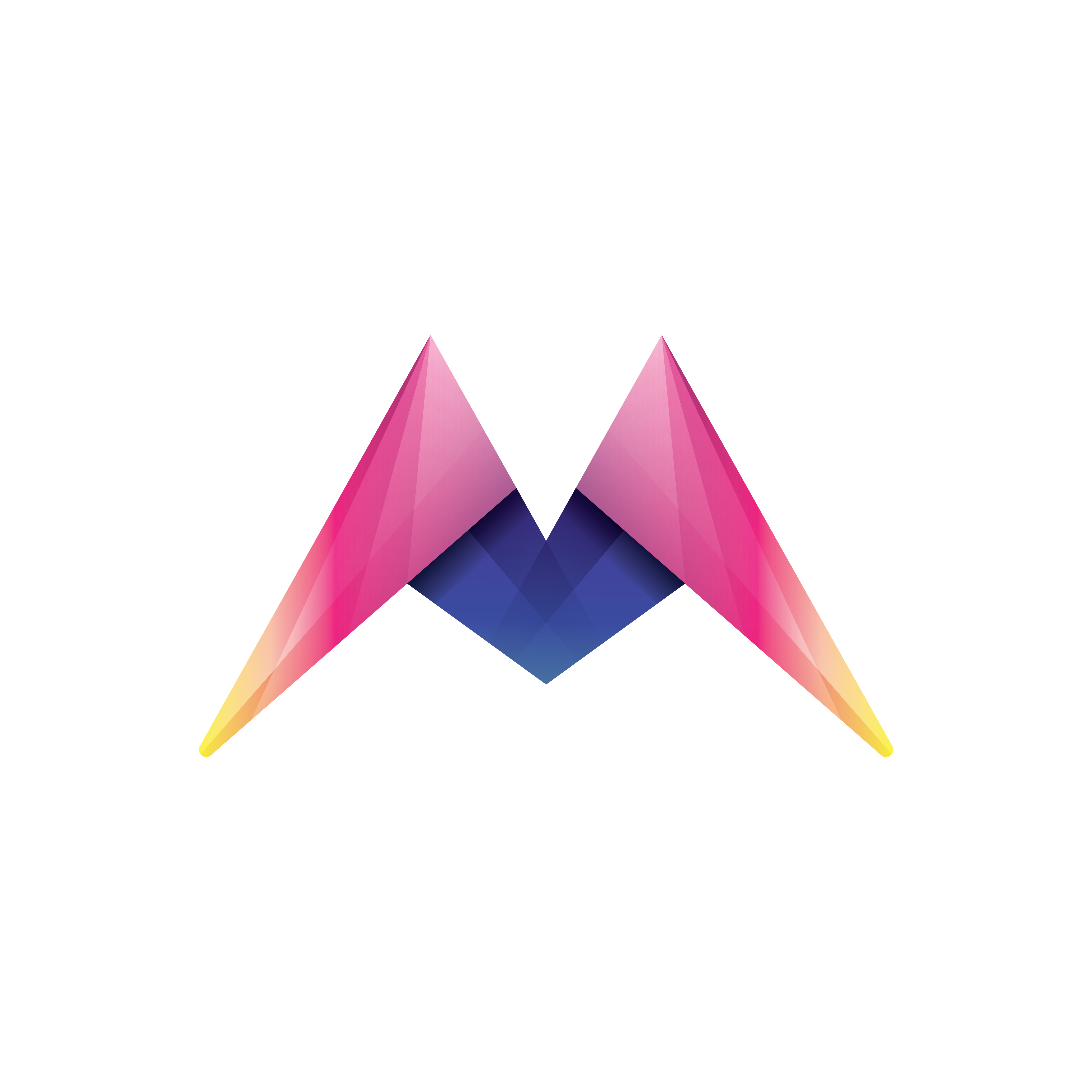
Mohamed Abubakkar M
Mohamed Abubakkar M
👋 Hello! I’m Mohamed Abubakkar I’m currently pursuing a Bachelor’s degree in Computer Science Engineering at Thiagarajar College of Engineering. My passion for technology drives me to explore a wide array of tech stacks, dive deep into data science, and tackle complex problems in data structures and algorithms (DSA). On my blog, I share insights from my academic journey, practical coding experiences, and projects that fuel my curiosity. Whether you’re interested in the latest in tech, data science tips, or strategies for mastering DSA, you’ll find a mix of tutorials, reflections, and learning resources here. I’m always open to connecting with fellow tech enthusiasts and students, so don’t hesitate to reach out! Thanks for visiting my blog. Let’s explore the world of technology together!