Optimizing Prompts from Llama 3.1 70B to 8B
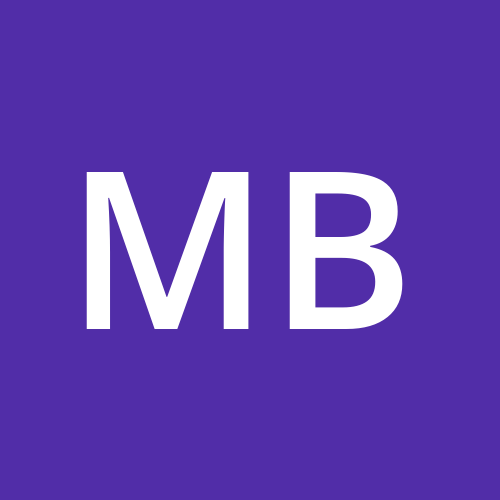
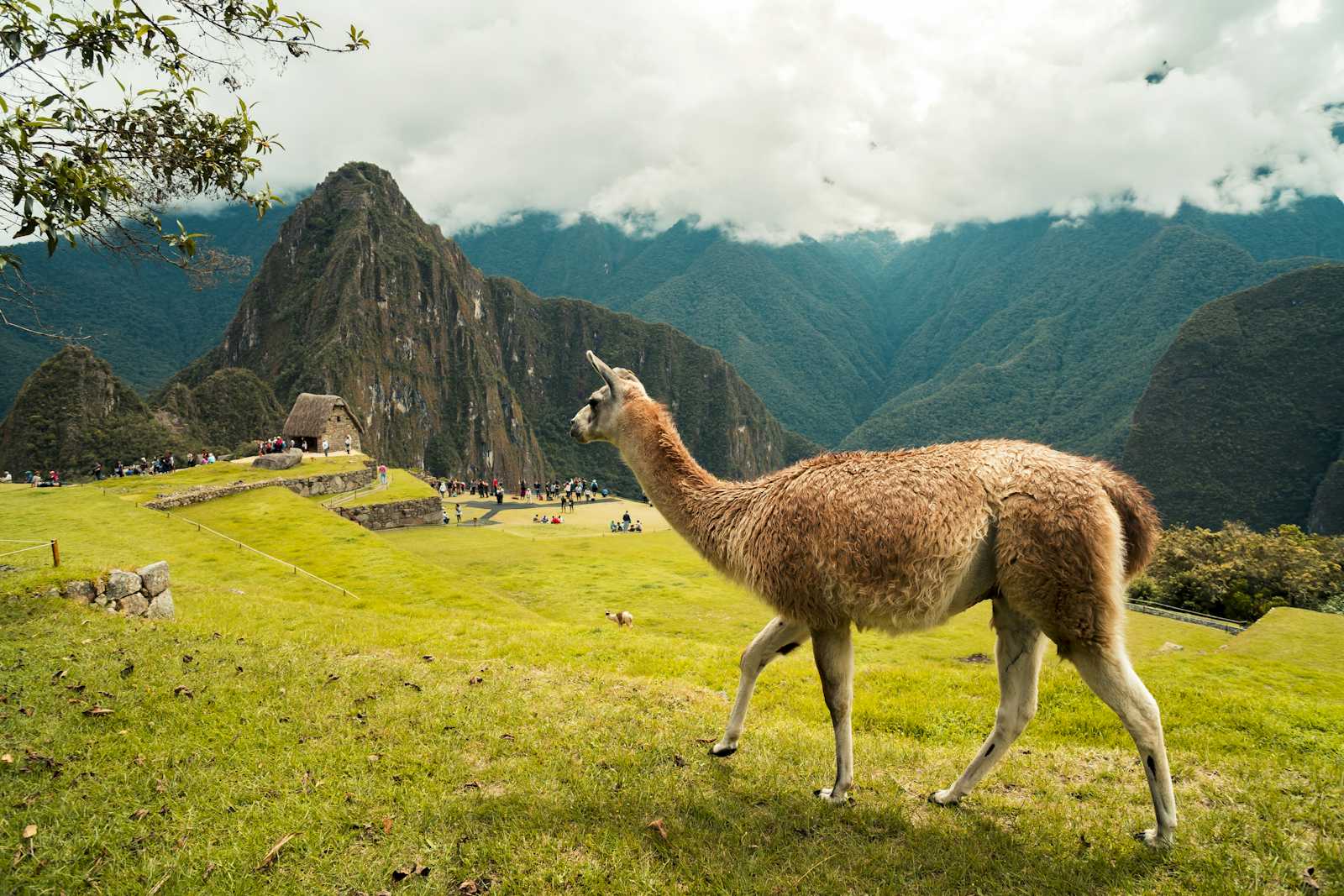
As an AI developer and enthusiast, I'm always looking for ways to push the boundaries of what's possible with language models. Today, I'm thrilled to share my latest project: a tool that optimizes prompts from Groq's Llama 3.1 70B model to work efficiently with their 8B model. Built on Replit and inspired by Matt Shumer's gpt-prompt-engineer, this tool is designed to make advanced prompt optimization accessible to everyone.
The Challenge: Balancing Power and Efficiency
Large language models like Llama 3.1 70B are incredibly powerful, but they come with significant computational costs. On the other hand, smaller models like Llama 3.1 8B are more efficient but may lack some of the capabilities of their larger counterparts. The challenge lies in bridging this gap – how can we leverage the power of larger models to optimize prompts for smaller, more efficient ones?
The Solution: A Prompt Optimization Pipeline
My tool addresses this challenge through a sophisticated prompt optimization pipeline. Here's how it works:
Task Description: Users input a description of the task they want to accomplish.
Initial Prompt Generation: The tool uses the 70B model to generate an initial prompt based on the task description.
Prompt Variation: It then creates multiple variations of the prompt, aiming for diversity and improvement.
System Prompt Generation: A system prompt is created to guide the 8B model in understanding the task.
8B Model Testing: The tool tests the optimized prompts on the 8B model, providing detailed results.
Let's dive into the key components of this tool:
Generating the Initial Prompt
def generate_prompt(task):
completion = client.chat.completions.create(
model=MODEL_70B,
messages=[{
"role": "system",
"content": "You are an AI assistant that generates example prompts for given tasks. Respond with only the prompt, nothing else."
}, {
"role": "user",
"content": f"Generate a sample prompt for the following task: {task}"
}],
temperature=0.7,
max_tokens=500,
)
return completion.choices[0].message.content.strip()
This function uses the 70B model to generate an initial prompt based on the user's task description. It's designed to be focused and concise, providing a solid starting point for optimization.
Creating Prompt Variations
def generate_candidate_prompts(task, prompt_example):
completion = client.chat.completions.create(
model=MODEL_70B,
messages=[{
"role": "system",
"content": "Given an example prompt, create seven additional prompts for the same task that are even better. Ensure the new prompts are diverse and unique from one another."
}, {
"role": "user",
"content": f"Task: {task}\nExample prompt: {prompt_example}"
}],
temperature=0.5,
max_tokens=4000,
)
prompts = completion.choices[0].message.content.strip().split('\n')
return prompts[:7] # Ensure we only return 7 prompts
This function generates multiple variations of the initial prompt, aiming for diversity and improvement. By creating a range of options, we increase the chances of finding an optimal prompt for the 8B model.
Generating the System Prompt
def generate_system_prompt(task, prompts):
completion = client.chat.completions.create(
model=MODEL_70B,
messages=[{
"role": "system",
"content": "Given a user-description of their task and a set of prompts, write a fantastic system prompt that describes the task to be done perfectly."
}, {
"role": "user",
"content": f"Task: {task}\nPrompts: {json.dumps(prompts)}"
}],
temperature=0.5,
max_tokens=1000,
)
return completion.choices[0].message.content.strip()
This function creates a system prompt that helps guide the 8B model in understanding and executing the task. It's a crucial step in ensuring that the smaller model can perform as effectively as possible.
Testing on the 8B Model
def test_8b(system_prompt, prompt):
completion = client.chat.completions.create(
model=MODEL_8B,
messages=[{
"role": "system",
"content": system_prompt
}, {
"role": "user",
"content": prompt
}],
temperature=0.5,
max_tokens=2000,
)
return completion.choices[0].message.content
This function tests the optimized prompts on the 8B model, allowing us to evaluate the effectiveness of our optimization process.
The User Interface: Simplifying Complexity
To make this tool accessible to a wide range of users, I've implemented a Gradio interface. This provides a user-friendly way to interact with the complex underlying processes:
with gr.Blocks() as iface:
gr.Markdown("# Llama 3.1 70B to 8B Prompt Conversion")
gr.Markdown("Convert prompts from Llama 3.1 70B to Llama 3.1 8B")
with gr.Row():
task_input = gr.Textbox(label="Task Description")
generate_button = gr.Button("Generate & Test Example Prompt")
with gr.Row():
prompt_input = gr.Textbox(label="Prompt Example", lines=5)
initial_response = gr.Textbox(label="70B Model Response", lines=5)
output = gr.Textbox(label="Conversion Output", lines=30)
groq_code_output = gr.Code(
label="Groq Code with 8B System Prompt and New Prompt",
language="python")
submit_button = gr.Button("Convert and Test")
# ... (click event handlers)
This interface allows users to input their task, generate an initial prompt, and then run the full optimization process with just a few clicks.
Why This Matters
This tool has significant implications for AI development and deployment:
Efficiency: By optimizing prompts for smaller models, we can reduce computational costs without sacrificing too much capability.
Accessibility: It makes advanced prompt engineering techniques available to a wider audience, fostering innovation.
Research: It provides a platform for studying the differences between large and small language models, potentially leading to new insights in AI development.
Try It Yourself
I've made this tool available as a Replit template, so you can try it out for yourself. Just add your Groq API key, and you're ready to go! Check it out here:
Conclusion
As we continue to push the boundaries of AI, tools like this will play a crucial role in making advanced technologies more accessible and efficient. I'm excited to see how the community will use and build upon this project. Whether you're optimizing AI deployments, studying model capabilities, or developing new prompt engineering strategies, I hope this tool will be a valuable resource in your AI journey.
Feel free to fork the project, contribute, or share your results. Let's work together to unlock the full potential of AI, making it more efficient and accessible for everyone!
Subscribe to my newsletter
Read articles from Martin Bowling directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
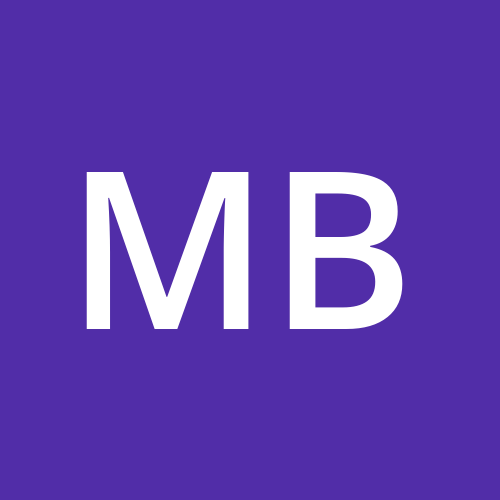