Bitwise vs Logical Operators in Python: An Easy Explanation
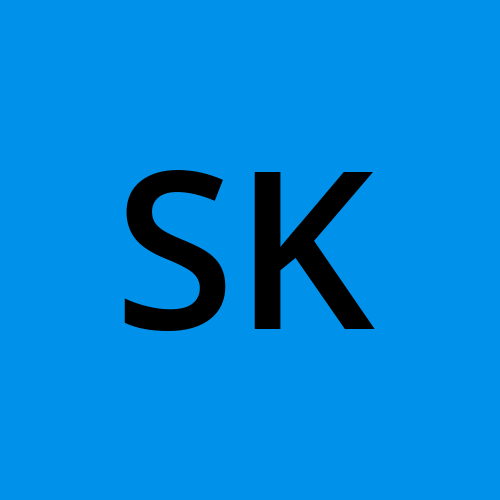
Introduction
Python programming mein operators ek important role play karte hain. Yeh operators data ko manipulate karne ke liye use kiye jaate hain. Python mein mukhya do tarah ke operators hain: Bitwise Operators aur Logical Operators. Is blog post mein hum in dono ke beech ke differences, boolean aur non-boolean types, aur kuch useful tips aur tricks discuss karenge. Toh chaliye shuru karte hain!
1. Bitwise Operators:
Bitwise operators direct binary bits ke sath kaam karte hain. Yeh operators bits ko manipulate karne ke liye use kiye jaate hain. Yahan kuch commonly used bitwise operators hain:
& (Bitwise AND): Yeh operator dono operands ke corresponding bits ko compare karta hai. Agar dono bits 1 hain, tab result bit 1 hota hai.
Example:
a = 12 # Binary: 1100 b = 7 # Binary: 0111 result = a & b # Result: 0100 (4 in decimal) print(result)
| (Bitwise OR): Yeh operator dono operands ke corresponding bits ko compare karta hai. Agar koi bhi bit 1 hai, tab result bit 1 hota hai.
Example:
a = 12 # Binary: 1100 b = 7 # Binary: 0111 result = a | b # Result: 1111 (15 in decimal) print(result)
^ (Bitwise XOR): Yeh operator dono operands ke corresponding bits ko compare karta hai. Agar bits alag hain, tab result bit 1 hota hai.
Example:
a = 12 # Binary: 1100 b = 7 # Binary: 0111 result = a ^ b # Result: 1011 (11 in decimal) print(result)
~ (Bitwise NOT): Yeh operator operand ke sabhi bits ko invert karta hai.
Example:
a = 12 # Binary: 1100 result = ~a # Result: -13 (Inverted binary bits) print(result)
<< (Left Shift): Yeh operator bits ko left shift karta hai aur right side pe zeroes add karta hai.
Example:
a = 5 # Binary: 0101 result = a << 1 # Result: 1010 (10 in decimal) print(result)
\>> (Right Shift): Yeh operator bits ko right shift karta hai aur left side pe sign bit add karta hai.
Example:
a = 5 # Binary: 0101 result = a >> 1 # Result: 0010 (2 in decimal) print(result)
2. Logical Operators:
Logical operators truth values ke sath kaam karte hain aur conditional expressions ko evaluate karne ke liye use kiye jaate hain. Yeh operators boolean logic ka part hain aur basic comparisons ko perform karte hain.
and
Operator: Yeh operator tab true return karta hai jab dono conditions true hoti hain. Agar koi bhi condition false hai, toh result false hota hai.Example:
a = True b = False result = a and b # Result: False print(result)
False Output Example:
x = 10 y = 20 if x > 15 and y > 15: print("Both conditions are true.") else: print("At least one condition is false.")
or
Operator: Yeh operator tab true return karta hai jab kisi bhi ek condition true hoti hai. Agar dono conditions false hain, tab result false hota hai.Example:
a = True b = False result = a or b # Result: True print(result)
False Output Example:
x = 10 y = 5 if x > 15 or y > 15: print("At least one condition is true.") else: print("Both conditions are false.")
not
Operator: Yeh operator condition ka opposite result return karta hai. Agar condition true hai, tohnot
usse false banata hai aur vice versa.Example:
a = True result = not a # Result: False print(result)
False Output Example:
x = 10 if not (x > 15): print("Condition is false.") else: print("Condition is true.")
3. Boolean vs Non-Boolean Types:
Boolean Types: Boolean values sirf do hi values ko represent karte hain:
True
aurFalse
. Yeh values logical operations ke results ko represent karti hain.Example:
a = True b = False print(a and b) # Output: False
Non-Boolean Types: Yeh types woh values hain jo boolean context mein convert hoti hain, jaise integers aur strings. Non-zero numbers aur non-empty strings
True
ke roop mein convert hote hain, jabki zero aur empty stringsFalse
ke roop mein convert hote hain.Example:
number = 10 if number: print("Number is not zero.") # Output: Number is not zero.
string = "" if string: print("String is not empty.") else: print("String is empty.") # Output: String is empty.
Boolean vs Non-Boolean Functions:
Boolean Functions: Functions jo boolean values return karte hain. Yeh functions conditions ko check karte hain aur
True
yaFalse
return karte hain.Example:
def is_even(num): return num % 2 == 0
Non-Boolean Functions: Functions jo integers, strings, lists, etc. return karte hain. Yeh functions complex operations perform karte hain.
Example:
def add_numbers(a, b): return a + b
4. Tips and Tricks for Learning Operators:
Understand Operator Precedence: Python mein operators ki precedence important hoti hai. Arithmetic operators ki precedence logical aur bitwise operators se zyada hoti hai.
Use Parentheses for Clarity: Complex expressions ko clear aur readable banane ke liye parentheses ka use karein.
Practice with Examples: Regular practice aur real-life examples se aap operators ke usage ko acche se samajh sakte hain.
Refer to Python Documentation: Official Python documentation ko refer karna bhi useful hota hai. Yeh aapko operators ke behavior aur syntax ke bare mein detailed information provide karta hai.
Conclusion
Is blog post mein humne Python ke bitwise aur logical operators, boolean aur non-boolean types, aur kuch useful tips aur tricks discuss kiye hain. Bitwise operators binary data ke saath kaam karte hain jabki logical operators boolean expressions ko evaluate karte hain. Boolean aur non-boolean types ke beech ka difference samajhna programming mein accuracy aur efficiency ko improve kar sakta hai.
Aapko agar yeh blog useful laga, toh ise share karna na bhoolen aur kisi bhi query ke liye comments mein likhein. Happy coding!
:Python operators, bitwise operators, logical operators, boolean vs non-boolean, Python programming, Python tips and tricks, bitwise vs logical operators, Python blog, programming basics.
Subscribe to my newsletter
Read articles from Sandhya Kondmare directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
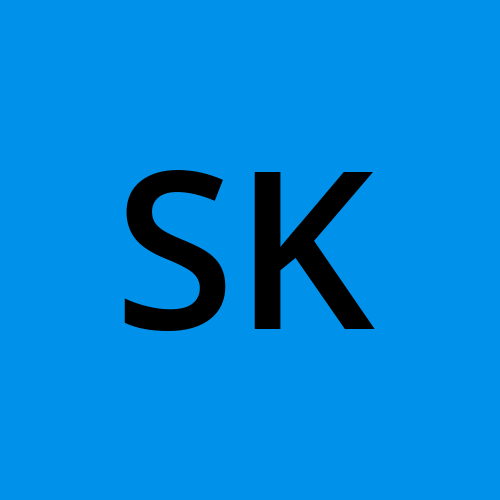
Sandhya Kondmare
Sandhya Kondmare
Aspiring DevOps Engineer with 2 years of hands-on experience in designing, implementing, and managing AWS infrastructure. Proven expertise in Terraform for infrastructure as code, automation tools, and scripting languages. Adept at collaborating with development and security teams to create scalable and secure architectures. Hands-on in AWS, GCP, Azure and Terraform best practices.