Python Special Operators Explained: Number Systems and Problem Solving Tips
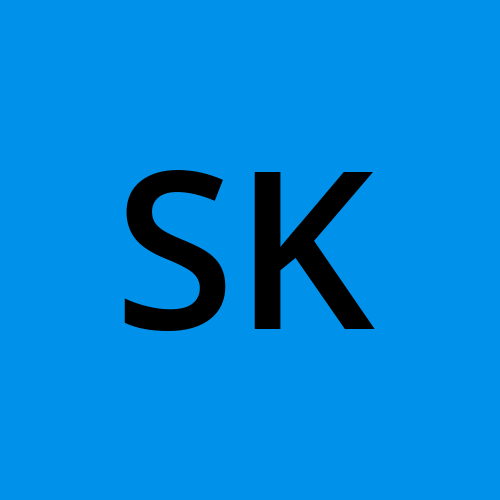
Introduction
Python programming mein operators ka role crucial hota hai. Yeh operators data ko manipulate karne aur logical operations perform karne mein madad karte hain. Python mein kuch special operators bhi hote hain jo specific tasks ke liye design kiye gaye hain. Is blog post mein hum special operators aur number systems jaise decimal aur binary ke basics ke saath, in systems ko use karke problems ko solve karne ke methods ko bhi discuss karenge.
1. Special Operators in Python:
Python mein kuch special operators hain jo data manipulation aur comparison tasks ke liye use kiye jaate hain. Yeh operators Python ki programming flexibility ko enhance karte hain.
is
Operator: Yeh operator check karta hai ki do objects ek hi reference ko point kar rahe hain ya nahi. Yeh object identity ko compare karta hai.Example:
a = [1, 2, 3] b = [1, 2, 3] c = a print(a is b) # Output: False print(a is c) # Output: True
Explanation:
a is b
false return karega kyunkia
aurb
alag list objects hain.a is c
true return karega kyunkia
aurc
same object reference ko point karte hain.is not
Operator: Yeh operatoris
operator ka negation hai. Yeh check karta hai ki do operands alag objects hain ya nahi.Example:
a = [1, 2, 3] b = [1, 2, 3] c = a print(a is not b) # Output: True print(a is not c) # Output: False
Explanation:
a is not b
true return karega kyunkia
aurb
alag objects hain, jabkia is not c
false return karega kyunkia
aurc
same object hain.in
Operator: Yeh operator check karta hai ki ek value kisi container (list, tuple, string, etc.) mein present hai ya nahi.Example:
fruits = ['apple', 'banana', 'cherry'] print('banana' in fruits) # Output: True print('grape' in fruits) # Output: False
Explanation:
'banana' in fruits
true return karega kyunki'banana'
list mein present hai, jabki'grape' in fruits
false return karega kyunki'grape'
list mein nahi hai.not in
Operator: Yeh operatorin
operator ka negation hai. Yeh check karta hai ki ek value kisi container mein nahi hai.Example:
fruits = ['apple', 'banana', 'cherry'] print('banana' not in fruits) # Output: False print('grape' not in fruits) # Output: True
Explanation:
'banana' not in fruits
false return karega kyunki'banana'
list mein hai, jabki'grape' not in fruits
true return karega kyunki'grape'
list mein nahi hai.
2. Number Systems: Decimal aur Binary Basics
Python programming aur computer science mein number systems ki understanding zaroori hai. Decimal aur binary number systems widely used hain aur programming mein unka knowledge beneficial hota hai.
Decimal Number System (Base-10):
- Definition: Decimal system mein 10 digits hote hain (0 se 9). Yeh system daily life mein use hota hai aur human-readable hota hai.
Example:
decimal_number = 255
print(f"Decimal number: {decimal_number}") # Output: Decimal number: 255
Binary Number System (Base-2):
- Definition: Binary system mein 2 digits hote hain (0 aur 1). Yeh system computers aur digital systems ke liye fundamental hai.
Example:
binary_number = 0b11111111 # Binary representation of 255
print(f"Binary number: {binary_number}") # Output: Binary number: 255
Conversion Example:
decimal_number = 255
binary_number = bin(decimal_number)
print(f"Binary representation: {binary_number}") # Output: Binary representation: 0b11111111
Explanation: bin()
function decimal number ko binary string mein convert karta hai, jisme 0b
prefix binary number ko denote karta hai.
3. Solving Problems with Number Systems and Operators:
Number systems aur operators ka use karke problems ko solve karne ka approach different ho sakta hai, lekin yeh basic steps aapko help karenge:
Binary to Decimal Conversion: Agar aapko binary number ko decimal mein convert karna hai, toh aap bitwise operators aur manual conversion techniques ka use kar sakte hain.
Example:
binary_number = 0b1101 # Binary representation of 13 decimal_number = int(binary_number) # Convert binary to decimal print(f"Decimal representation: {decimal_number}") # Output: Decimal representation: 13
Decimal to Binary Conversion: Decimal number ko binary mein convert karne ke liye
bin()
function use kar sakte hain.Example:
decimal_number = 13 binary_number = bin(decimal_number) print(f"Binary representation: {binary_number}") # Output: Binary representation: 0b1101
Bitwise Operations: Binary numbers ke saath bitwise operations perform karke different results achieve kiye ja sakte hain. For example, bitwise AND, OR, aur XOR operations binary data ko manipulate karte hain.
Example:
a = 0b1010 # Binary: 10 b = 0b1100 # Binary: 12 result_and = a & b # Bitwise AND result_or = a | b # Bitwise OR result_xor = a ^ b # Bitwise XOR print(f"Bitwise AND result: {bin(result_and)}") # Output: Bitwise AND result: 0b1000 print(f"Bitwise OR result: {bin(result_or)}") # Output: Bitwise OR result: 0b1110 print(f"Bitwise XOR result: {bin(result_xor)}") # Output: Bitwise XOR result: 0b0110
Explanation: Bitwise operations binary numbers ko directly manipulate karte hain aur binary data ki processing ko optimize karte hain.
4. Tips and Tricks for Mastering Special Operators and Number Systems:
Understand Operator Precedence: Python mein operators ki precedence ko samajhna important hai. Arithmetic operators ki precedence logical aur bitwise operators se zyada hoti hai.
Practice with Examples: Regular practice aur real-life examples se aap operators aur number systems ko better samajh sakte hain.
Use Interactive Tools: Python ke interactive shell ya Jupyter Notebooks mein experiments karna aapko quickly results dekhne mein help karta hai.
Refer to Documentation: Official Python documentation aur tutorials ko refer karke operators aur number systems ke deeper insights gain karein.
Visualize Concepts: Binary aur decimal systems ko visualizing karne se aapko better understanding milti hai. Tables aur diagrams ka use karke concepts ko clear kar sakte hain.
Conclusion
Is blog post mein humne Python ke special operators aur number systems (decimal aur binary) ke basics discuss kiye hain. Special operators jaise is
, is not
, in
, aur not in
data manipulation aur comparison mein useful hote hain. Number systems ka knowledge programming aur data handling mein madadgar hota hai. Regular practice aur proper understanding ke saath, aap in concepts ko effectively use kar sakte hain.
: Python special operators, number systems, decimal vs binary, Python programming basics, Python operators tutorial, binary number system, decimal number system, Python blog, special operators in Python, programming tips, Python for beginners
Subscribe to my newsletter
Read articles from Sandhya Kondmare directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
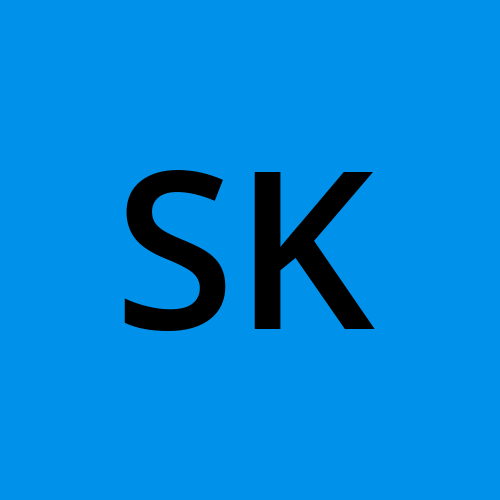
Sandhya Kondmare
Sandhya Kondmare
Aspiring DevOps Engineer with 2 years of hands-on experience in designing, implementing, and managing AWS infrastructure. Proven expertise in Terraform for infrastructure as code, automation tools, and scripting languages. Adept at collaborating with development and security teams to create scalable and secure architectures. Hands-on in AWS, GCP, Azure and Terraform best practices.