Building a Mixture of Agents (MoA) Chatbot
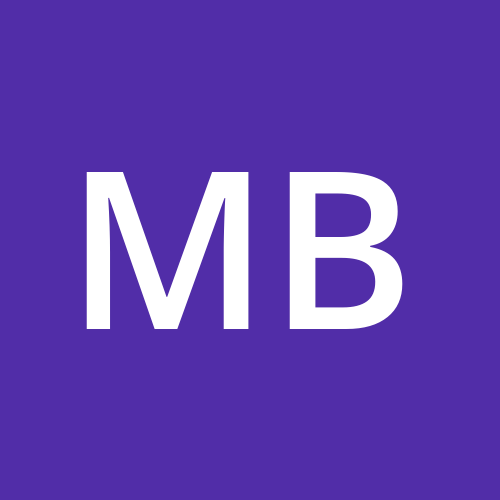
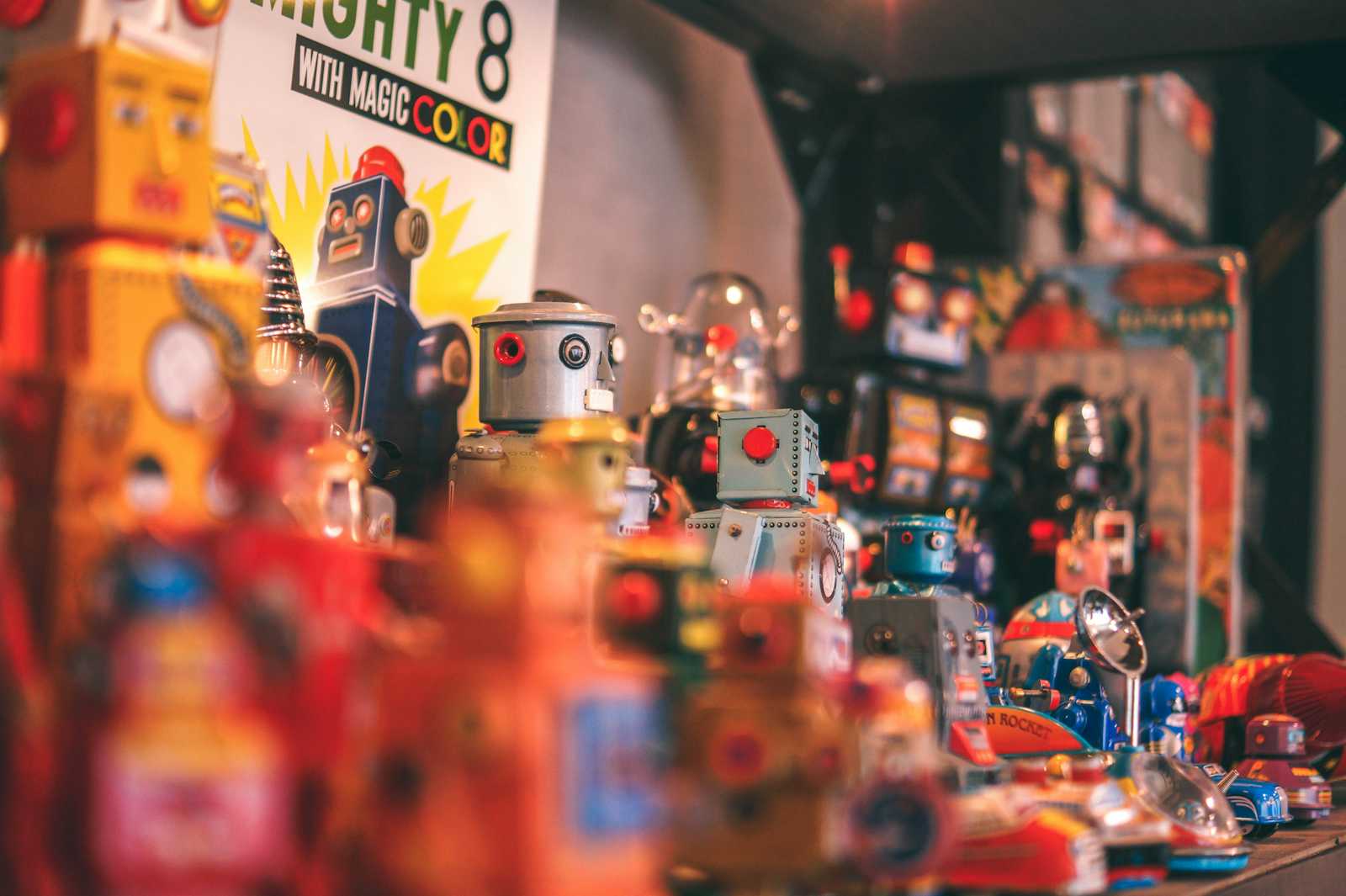
I'm excited to share with you a project I've been working on that combines my love for AI, rapid prototyping, and pushing the boundaries of what's possible with current language models. Let's dive into the world of Mixture of Agents (MoA) chatbots!
The Concept: Why Settle for One AI When You Can Have Four?
The idea behind this project is simple yet powerful: instead of relying on a single AI model to answer user queries, why not leverage the strengths of multiple models? This approach allows us to tap into the diverse knowledge and capabilities of different AI architectures, potentially leading to more comprehensive and nuanced responses.
Here's how it works:
We take the user's question and send it to four different language models.
Each model processes the query and generates its own response.
A fifth model, acting as an "aggregator," synthesizes these responses into a single, coherent answer.
The result? A chatbot that provides multi-perspective, well-rounded answers to user queries.
The Tech Stack: Groq, Replit, and Gradio
For this project, I've leveraged some cutting-edge tools:
Groq: This incredible inference API allows us to run multiple AI models with lightning-fast speed. It's the secret sauce that makes real-time processing of multiple models feasible.
Replit: I've set up the project as a Replit template, making it super easy for anyone to clone and run their own instance of the chatbot.
Gradio: This library provides a simple yet powerful interface for our chatbot, allowing users to interact with it through a clean, web-based UI.
The Code: Let's Break It Down
Let's walk through some key parts of the code to understand how this MoA chatbot works.
Setting Up the Models
reference_models = [
"gemma2-9b-it", "llama3-70b-8192", "llama3-8b-8192", "mixtral-8x7b-32768"
]
aggregator_model = "llama3-70b-8192"
Here, we define our four reference models and the aggregator model. I've chosen a mix of different architectures and sizes to get a diverse range of responses.
The Aggregator's System Prompt
The aggregator model needs to know its job. We provide it with a detailed system prompt:
aggregator_system_prompt = """As an advanced language model, your task is to synthesize responses from multiple AI models into a single, coherent, and high-quality answer. Follow these guidelines:
1. Analyze and compare: Carefully examine the responses from different models, noting similarities, differences, and unique insights.
2. Evaluate accuracy: Critically assess the information provided, identifying and discarding any inaccuracies or biases.
...
"""
This prompt is crucial as it guides the aggregator in combining the various model outputs effectively.
Running the Models
The run_llm
function handles individual model calls:
async def run_llm(model, message, history):
messages = [{
"role": "user" if i % 2 == 0 else "assistant",
"content": str(msg)
} for i, msg in enumerate(history + [message])]
response = await client.chat.completions.create(
model=model,
messages=messages,
temperature=0.7,
max_tokens=512,
)
return response.choices[0].message.content
We use async calls to Groq's API for optimal performance.
The Main Chat Function
The heart of our chatbot is the chat_with_mixture_of_agents
function:
async def chat_with_mixture_of_agents(message, history):
# Run reference models
tasks = [run_llm(model, message, history) for model in reference_models]
results = await asyncio.gather(*tasks)
# Prepare aggregator input
aggregator_input = "\n\n".join([
f"{model}:\n{response}"
for model, response in zip(reference_models, results)
])
# Run aggregator model
aggregator_messages = [
{
"role": "system",
"content": aggregator_system_prompt
},
{
"role": "user",
"content": f"User query: {message}\n\n{aggregator_input}"
},
]
response_content = ""
stream = await client.chat.completions.create(
model=aggregator_model,
messages=aggregator_messages,
temperature=0.7,
max_tokens=1024,
top_p=1,
stop=None,
stream=True,
)
async for chunk in stream:
content = chunk.choices[0].delta.content
if content:
response_content += content
yield response_content
This function orchestrates the entire process:
It runs all reference models concurrently.
It prepares the input for the aggregator model.
It streams the aggregator's response back to the user in real-time.
The User Interface
We use Gradio to create a simple chat interface:
with gr.Blocks(fill_height=True, head=js) as demo:
gr.ChatInterface(
chat_with_mixture_of_agents,
clear_btn=None,
undo_btn=None,
retry_btn=None,
fill_height=True,
examples=[
"Explain the concept of quantum entanglement in simple terms.",
"What are the potential implications of artificial general intelligence on society?",
# ... more example questions ...
])
This creates a clean, user-friendly interface with some example questions to get users started.
Conclusion and Future Directions
This MoA chatbot is just the beginning. There are so many exciting directions we could take this:
Experimenting with different combinations of models
Implementing more sophisticated aggregation techniques
Adding user controls for model selection or weighting
Integrating external knowledge sources
The possibilities are endless, and I can't wait to see what the community does with this concept. Remember, the project is open-source, so feel free to fork it, modify it, and make it your own!
If you want to try it out yourself, head over to the Replit template and start chatting with our AI panel. And don't forget to let me know what you think or if you have any cool ideas for improvements.
Happy coding, and may your AI adventures be ever exciting! ๐๐ค
Subscribe to my newsletter
Read articles from Martin Bowling directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
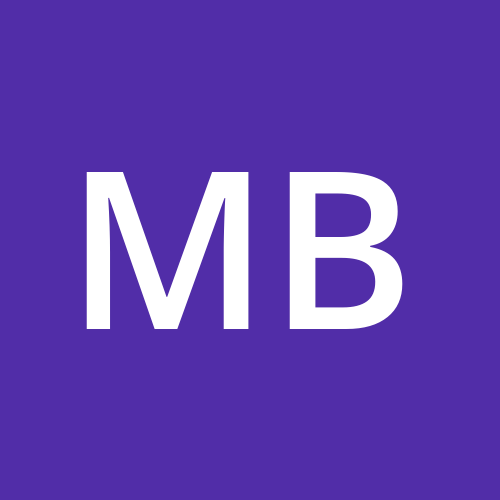