A simple regular expression example
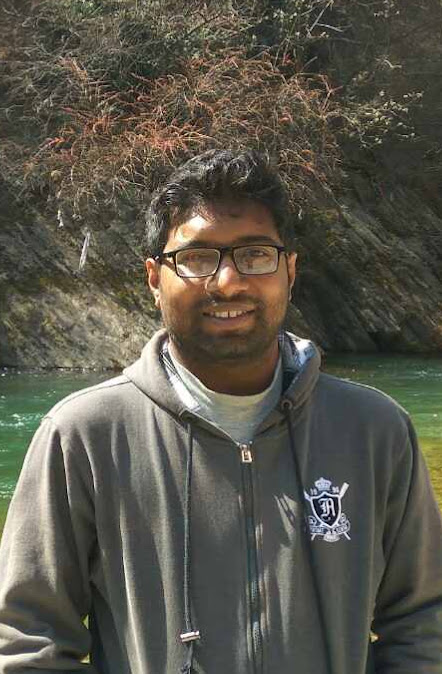
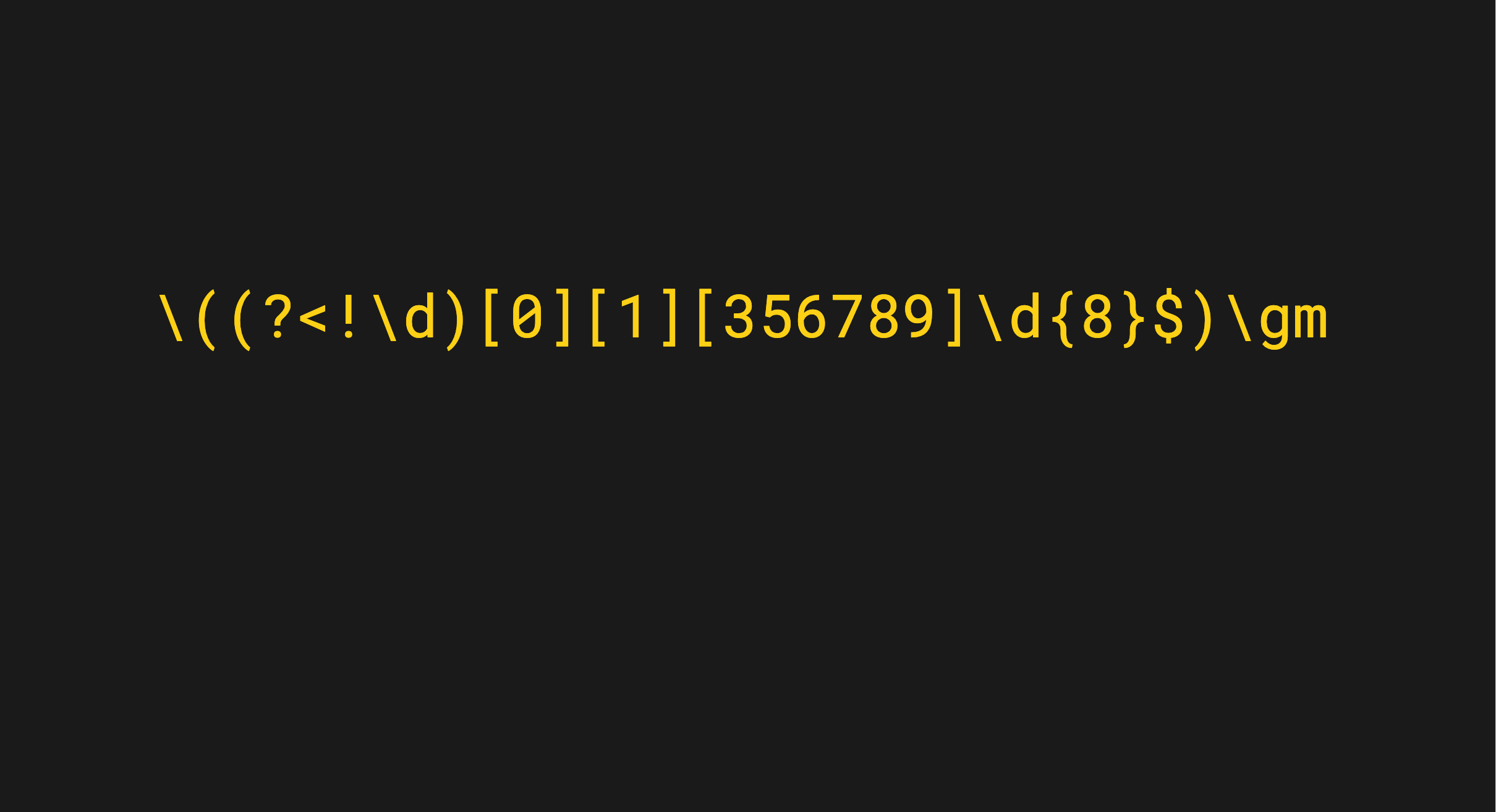
Once upon a time, simple regex patterns are really hard to understand to me. But now-a-days, I can write simple regex patterns comfortably. Few weeks ago, I also have written a complex one. Today I'm going to share how I approach a regex problem.
Today I'm going to build a regular expression pattern to match bangladeshi mobile phone numbers. I'll test with different numbers and also extract to a list in JavaScript.
N.B: The regex pattern I've shown here can be different from you. There are many ways to write regex.
Some valid Bangladeshi phone numbers
Before finding some patterns, let's look some generated random valid phone numbers. Feel free to ignore this.
01600000000
+8801611111111
8801622222222
1633333333
01312312312
01501201201
01777777777
01800000000
01912341234
Rules
Here are the rules for any bangladeshi mobile phone number.
Country code +880
with +, the length is 15
without + but starts with 880, the length is 14
with 0, the length is 11
without 0, the length is 10
after 0, start with 1
after 1, only 3,4,5,6,7,8,9 till 2024
after +, only digits
For simplicity, let's skip 2 rules
Country code +880
start with 0 not 1
Regex step by step
[0]
for matching 0[1]
for matching 1[356789]
for matching only any of 3,5,6,7,8,9\d
for matching any digits after\d{8}
for only 8 next digits only.there is a case if there are more than 8 digits, we put
$
for end of string.But some invalid cases will also be captured like
+8801611111111
8801622222222
So, we need to start from 0 not any other digits. We can easily do this using negative lookbehind (?<!\d). So, the final regex is
(?<!\d)[0][1][356789]\d{8}$
N.B: This is a possible way. There are different ways to build patterns.
Testing regex in online
There are many online tools for building, matching or testing regex. Currently I'm using regex101
In this website's middle, put your expression in REGULAR EXPRESSION
section and test data in TEST STRING
section.
In left side, there are 3 valuable sections
EXPLANATION
: This section describes the given expression step by step.MATCH INFORMATION
: This sction shows the resultQUICK REFERENCE
: If we need any regex pattern reference, this is the place.
Play with others rules
Now let's try to add some other rules.
A valid number can start with
+8801
or8801
or01
or even only1
here 1 is common and only 1 is valid then use quantifier like this
1{1}
Now for
+880
,880
,0
we can use the alternate(OR) operator like this(\+880|880|0)
Now this condition
(\+880|880|0)
can be present or not so, we can use this ? operator.
So, a possible pattern can be
(\+880|880|0)?1{1}[356789]\d{8}$
Capturing groups
Now if we want to extract the numbers then we can use single braces ()
((?<!\d)[0][1][356789]\d{8}$)
Let's write some code and extract some phone numbers
const expression = /((?<!\d)[0][1][356789]\d{8}$)/gm
const testString =
`* 0167561999789900
* 5896988016769696
* 112589696
* 01475619997
* 01600000000
* +8801611111111
* 8801622222222
* 1238801622222222
* 1633333333
* 01312312312
* 46467401600000000
* 345589+8801611111111
* 01501201201
* 01777777777
* 01800000000
* 01912341234`
const phoneNumberList = testString.match(expression)
phoneNumberList.forEach(phoneNumber => console.log(`Extracted number: ${phoneNumber}\n`))
Like bash, in JavaScript, the regular expression should be written inside slashes(\
). After second slash there is gm
. Here g means global and m means multiline. Let's keep those explanation for another day. If you run this code, the output may like this
Output:
Extracted number: 01600000000
Extracted number: 01312312312
Extracted number: 01501201201
Extracted number: 01777777777
Extracted number: 01800000000
Extracted number: 01912341234
Conclusion
I hope you enjoyed this regex example. If you have any doubt please let me know in the comment section. If you like this content don't forget to give a thumb. Happy coding.
Subscribe to my newsletter
Read articles from Asfaq Leeon directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
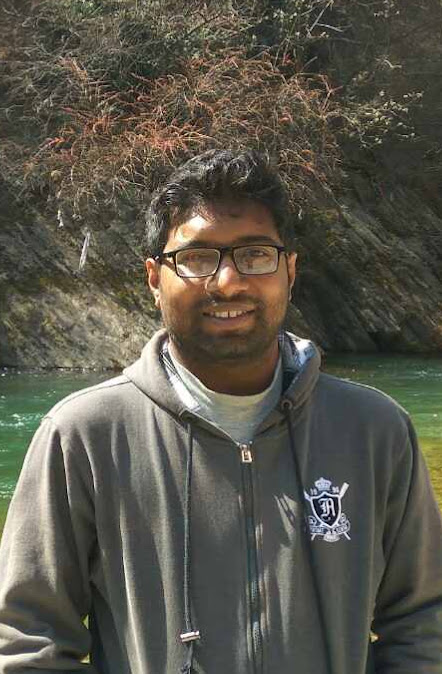