Building a Custom Packet Sniffer: Hands-On Guide with Code and Tools Overview
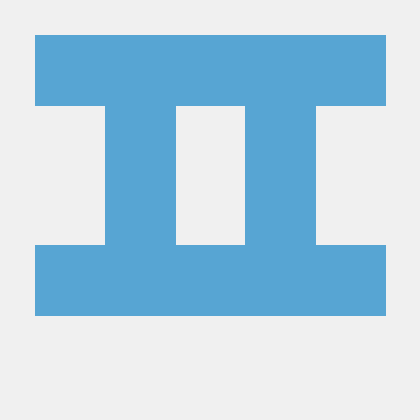
Table of contents
- Article Title: Building a Custom Packet Sniffer: Hands-On Guide with Code and Tools Overview
- 1. What is Packet Sniffing?
- 2. Existing Packet Sniffing Tools
- 3. Why Build a Custom Packet Sniffer?
- 4. Hands-On: Creating a Custom Packet Sniffer in Python
- Running the Code: Ethical Considerations
- Output
- 5. Advantages of Using a Custom Sniffer
- 6. Conclusion
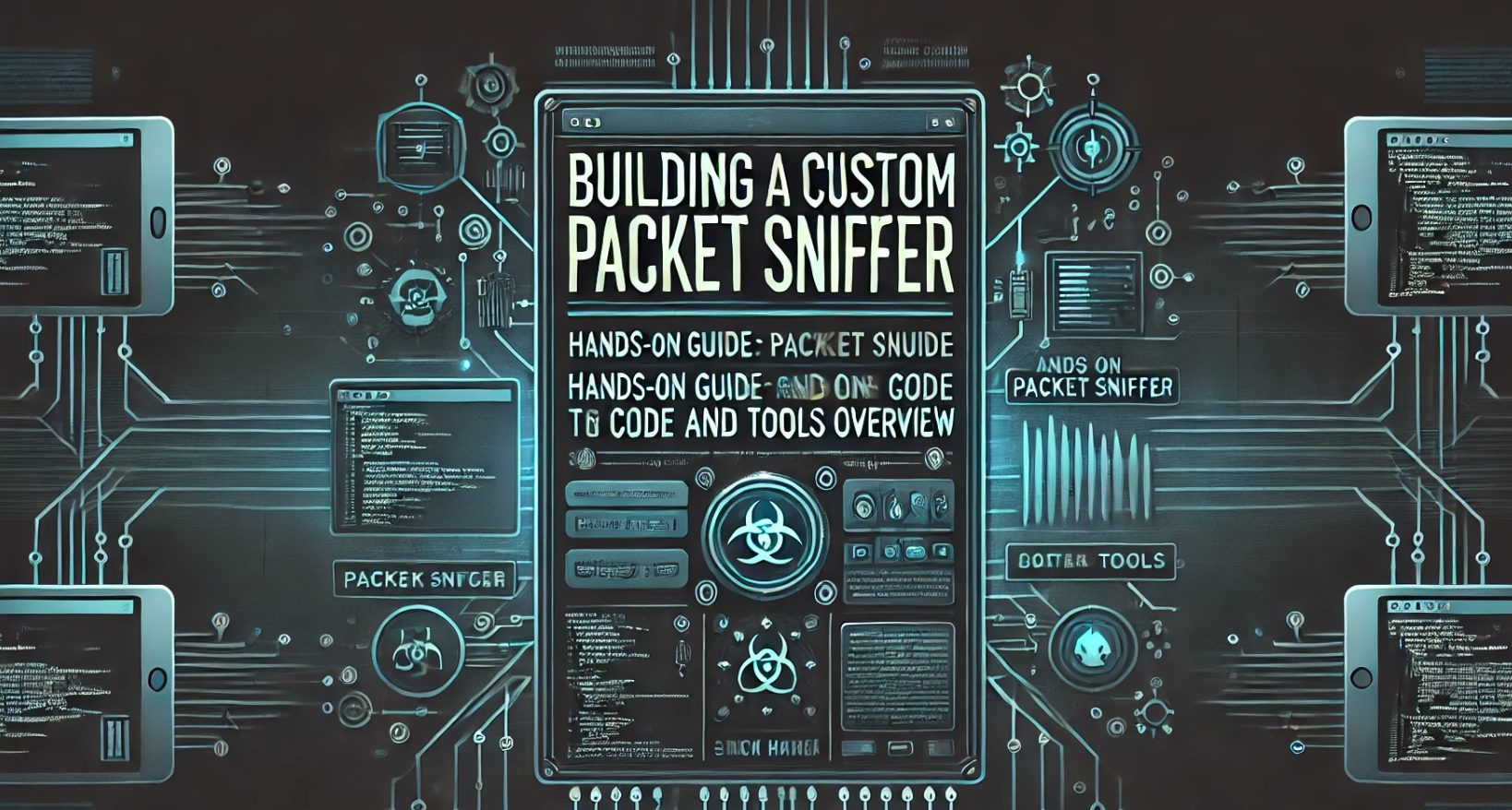
Packet sniffing is a crucial skill in cybersecurity, allowing network administrators and security professionals to monitor network traffic and diagnose issues. While tools like Wireshark provide comprehensive features, building a custom packet sniffer offers unique advantages, such as the ability to tailor it for specific tasks and gain deeper insights into network protocols.
In this article, we'll explore the concept of packet sniffing, review existing tools, and guide you through creating your own custom packet sniffer in Python. We’ll also discuss the advantages of using a custom-built sniffer and provide a hands-on example with code that you can run to see the packet length, protocol, source, and destination addresses.
Article Title: Building a Custom Packet Sniffer: Hands-On Guide with Code and Tools Overview
Packet sniffing is a crucial skill in cybersecurity, allowing network administrators and security professionals to monitor network traffic and diagnose issues. While tools like Wireshark provide comprehensive features, building a custom packet sniffer offers unique advantages, such as the ability to tailor it for specific tasks and gain deeper insights into network protocols.
In this article, we'll explore the concept of packet sniffing, review existing tools, and guide you through creating your own custom packet sniffer in Python. We’ll also discuss the advantages of using a custom-built sniffer and provide a hands-on example with code that you can run to see the packet length, protocol, source, and destination addresses.
1. What is Packet Sniffing?
Packet sniffing involves capturing and analyzing data packets as they travel across a network. This can be useful for:
Troubleshooting Network Issues: Identify bottlenecks or errors in network communication.
Security Monitoring: Detect unusual or malicious activity on a network.
Learning and Research: Understand how network protocols work by observing real traffic.
2. Existing Packet Sniffing Tools
Several tools are widely used for packet sniffing:
Wireshark: The most popular packet analyzer, Wireshark offers a graphical interface and extensive filtering options. It supports real-time packet capture and deep inspection of hundreds of protocols.
tcpdump: A command-line tool that allows users to capture and display packets being transmitted or received over a network. It’s lightweight and powerful, but less user-friendly than Wireshark.
Nmap: While primarily a network scanner, Nmap also has packet-sniffing capabilities. It’s useful for network discovery and security auditing.
3. Why Build a Custom Packet Sniffer?
While existing tools are powerful, there are several advantages to building your own packet sniffer:
Customization: Tailor the sniffer to specific network conditions or protocols. For instance, you can focus on only capturing HTTP traffic or packets from a particular source.
Performance: A custom sniffer can be optimized for specific tasks, reducing overhead and improving performance in targeted scenarios.
Learning Experience: Building your own sniffer deepens your understanding of networking protocols and data flow, enhancing your cybersecurity skills.
4. Hands-On: Creating a Custom Packet Sniffer in Python
Let’s dive into creating a simple packet sniffer using Python. This sniffer will capture packets, and for each packet, it will display the packet length, protocol, source address, and destination address.
Step 1: Setting Up the Environment
Running the Code: Ethical Considerations
Before you run this packet sniffer, it's crucial to understand that capturing network traffic can raise significant privacy and legal concerns. Packet sniffing should only be performed on networks you own, have permission to monitor, or in a controlled lab environment specifically set up for learning purposes, such as SEED Labs.
Note: Running a packet sniffer on a network without explicit permission is not only unethical but can also be illegal in many jurisdictions. Even though the data you capture might be encrypted, you are still accessing potentially sensitive information.
Ensure you have Python installed. You’ll also need the socket
module, which is included in the Python standard library,
import socket
import struct
# Function to unpack Ethernet frame
def ethernet_frame(data):
dest_mac, src_mac, proto = struct.unpack('! 6s 6s H', data[:14])
return get_mac_addr(dest_mac), get_mac_addr(src_mac), socket.htons(proto), data[14:]
# Function to format MAC addresses
def get_mac_addr(bytes_addr):
return ':'.join(format(byte, '02x') for byte in bytes_addr)
# Function to unpack IPv4 packets
def ipv4_packet(data):
version_header_length = data[0]
version = version_header_length >> 4
header_length = (version_header_length & 15) * 4
ttl, proto, src, target = struct.unpack('! 8x B B 2x 4s 4s', data[:20])
return version, header_length, ttl, proto, ipv4(src), ipv4(target), data[header_length:]
# Function to format IPv4 addresses
def ipv4(addr):
return '.'.join(map(str, addr))
# Function to identify the protocol name
def protocol_name(proto):
if proto == 1:
return "ICMP"
elif proto == 6:
return "TCP"
elif proto == 17:
return "UDP"
else:
return str(proto)
# Function to capture and parse packets
def sniff_packets():
# Create a raw socket
conn = socket.socket(socket.AF_PACKET, socket.SOCK_RAW, socket.ntohs(3))
while True:
# Capture a packet
raw_data, addr = conn.recvfrom(65536)
packet_length = len(raw_data)
# Unpack Ethernet frame
dest_mac, src_mac, eth_proto, data = ethernet_frame(raw_data)
print(f'\nEthernet Frame:')
print(f'\t- Destination: {dest_mac}, Source: {src_mac}, Protocol: {eth_proto}')
# Unpack IPv4 packets
if eth_proto == 8:
(version, header_length, ttl, proto, src, target, data) = ipv4_packet(data)
proto_name = protocol_name(proto)
print(f'\t- IPv4 Packet:')
print(f'\t\t- Version: {version}, Header Length: {header_length}, TTL: {ttl}')
print(f'\t\t- Protocol: {proto_name}, Source: {src}, Target: {target}')
print(f'\t\t- Packet Length: {packet_length} bytes')
if __name__ == "__main__":
sniff_packets()
Step 2: Running the Sniffer
Run the script and the sniffer will start capturing packets on your network. It will display details like the Ethernet frame, IPv4 packet details, including the protocol, source, and destination addresses.
Output
5. Advantages of Using a Custom Sniffer
Precision: Capture only the data you need, reducing clutter and improving focus.
Efficiency: Optimized for specific tasks, your custom sniffer can be faster and use fewer resources.
Enhanced Security: By building your own tool, you eliminate reliance on third-party software, which might introduce vulnerabilities.
6. Conclusion
Creating your own packet sniffer not only provides a deeper understanding of how network traffic is structured but also gives you the flexibility to customize the tool to your specific needs. While tools like Wireshark and tcpdump are incredibly powerful, a custom-built sniffer offers unique advantages in performance, precision, and learning.
With the provided code, you can now start capturing and analyzing packets on your network. Experiment with different filters and modifications to see what else you can discover about your network traffic.
Subscribe to my newsletter
Read articles from Chitraksh Gupta directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
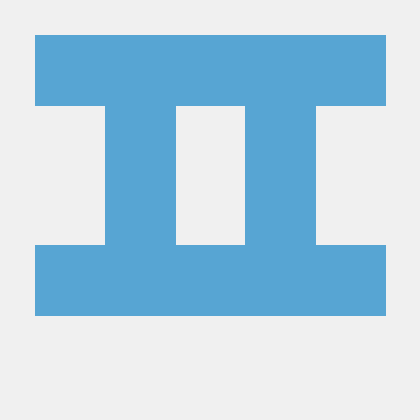