๐ Mastering Shell Scripting: Control Commands for Beginners

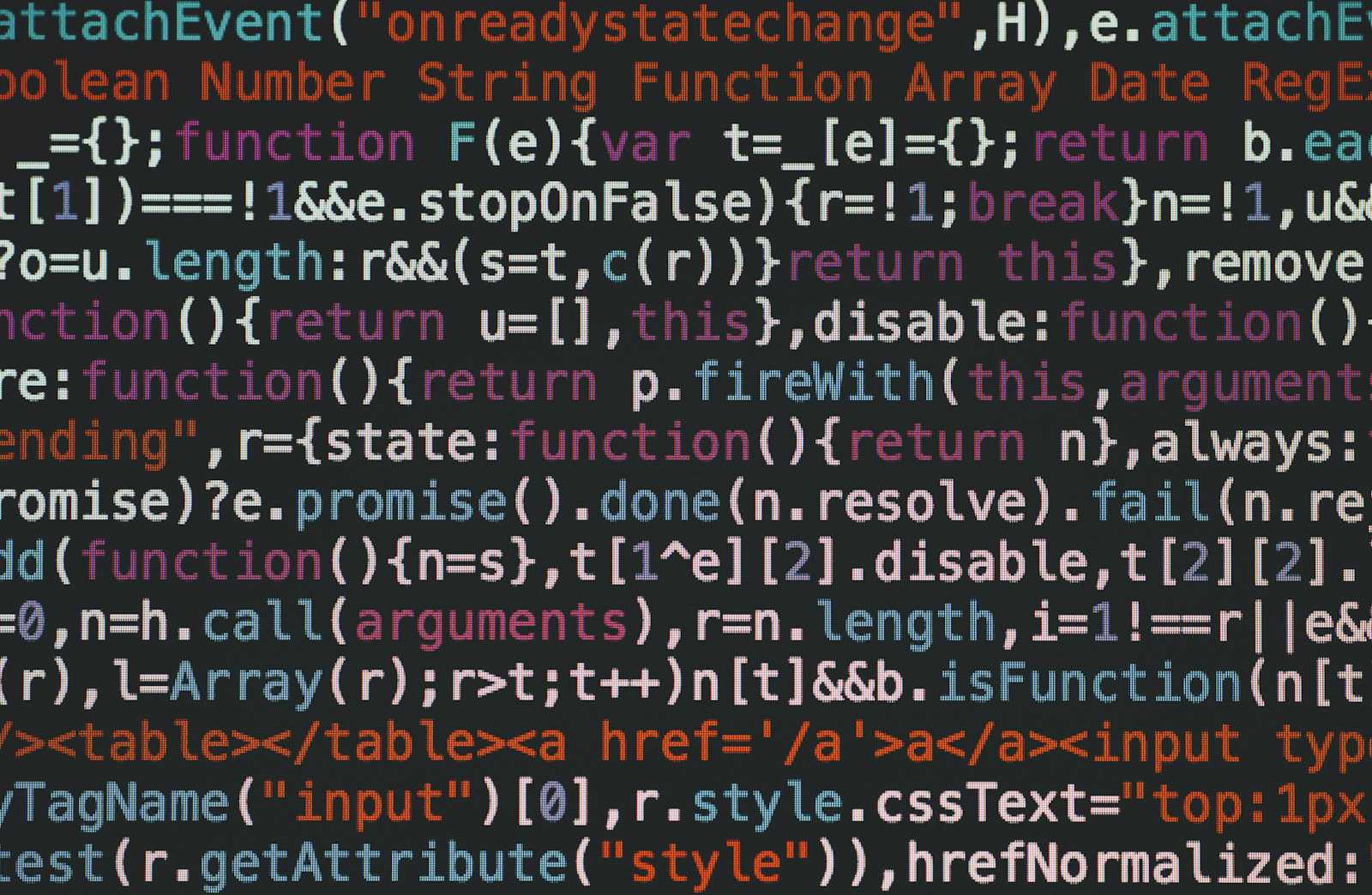
Control Commands:
if control statement:
To use multiple conditions in one if-else
block, the elif
keyword is used in shell. If expression1
is true, it executes statement 1 and 2, and this process continues. If none of the conditions are true, it processes the else part.
- Syntax-
if [ expression1 ] then statement1 statement2 . . elif [ expression2 ] then statement3 statement4 . . else statement5 fi
#!/bin/bash
# Prompt the user for input
echo "Enter Num1"
read num1
echo "Enter Num2"
read num2
echo "Enter Num3"
read num3
# Check if all numbers are equal
if [ "$num1" -eq "$num2" ] && [ "$num1" -eq "$num3" ] && [ "$num2" -eq "$num3" ]; then
echo "All numbers are equal"
# Check if num1 is the largest
elif [ "$num1" -gt "$num2" ] && [ "$num1" -gt "$num3" ]; then
echo "$num1"
# Check if num2 is the largest
elif [ "$num2" -gt "$num1" ] && [ "$num2" -gt "$num3" ]; then
echo "$num2"
# If not num1 or num2, then num3 must be the largest
else
echo "$num3"
fi
example1:
Enter Num1: 2
Enter Num2: 2
Enter Num3: 2All numbers are equal
example2:
Enter Num1: 1
Enter Num2: 2
Enter Num3: 33
Note: if and then must be separated, either with a <<>new line>> or semicolon (;). Termination of the if statement is fi.
For loop:
The for loop operates on lists of items. It repeats a set of commands for each item in a list.
Here, var
is the name of a variable, and word1
to wordN
are sequences of characters separated by spaces (words). Each time the for loop runs, the value of the variable var
is set to the next word in the list, from word1
to wordN
.
Syntax-
for <var> in <value1 value2 ... valuen> do <command 1> <command 2> <etc> done
#! /bin/bash
echo "ptinting number 1 to 10:"
for ((i=1; i<=10; i++))
do
echo "$i"
done
o/p: printing number 1 to 10:
1
2
3
4
5
6
7
8
9
10
while loop:
Here, the command is evaluated, and based on the result, the loop will execute. If the command evaluates to false, the loop will terminate.
Syntax-
while <condition> do <command 1> <command 2> <etc> done
#! /bin/bash
i=5
while [ $i -ge 0 ]
do
echo "$i"
echo " "
i=$((i - 1))
done
o/p:
5
4
3
2
1
0
Switch case:
The switch case statement is an effective alternative to the multilevel if-then-else-fi statement. It allows you to match multiple values against a single variable. This approach is easier to read and write.
- Syntax-
case word in pattern1) Statement(s) to be executed if pattern1 matches ;; pattern2) Statement(s) to be executed if pattern2 matches ;; pattern3) Statement(s) to be executed if pattern3 matches ;; \) Default condition to be executed ;; esac*
#!/bin/bash
echo "Which color do you like best?"
echo "1 - Blue"
echo "2 - Red"
echo "3 - Yellow"
echo "4 - Green"
echo "5 - Orange"
read color;
case $color in
1) echo "Blue is a primary color.";;
2) echo "Red is a primary color.";;
3) echo "Yellow is a primary color.";;
4) echo "Green is a secondary color.";;
5) echo "Orange is a secondary color.";;
*) echo "This color is not available. Please choose a different one.";;
esac
o/p: Which color do you like best?
1 - Blue
2 - Red
3 - Yellow
4 - Green
5 - Orange
3
Yellow is a primary color
Conclusion:
Mastering shell scripting is a valuable skill for anyone working in a Unix-like environment. By understanding and utilizing control commands such as if
, for
, while
, and Switch case
, you can automate tasks, manage system operations, and enhance your productivity. These foundational concepts are essential for beginners and provide a strong base for more advanced scripting techniques. Keep practicing and experimenting with different scenarios to become proficient in shell scripting.
Subscribe to my newsletter
Read articles from Anas Ansari directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Anas Ansari
Anas Ansari
A passionate DevOps Engineer who enjoys sharing his thoughts with others and learning from them!