Harnessing the Power of Generators and Iterators for Optimal Iteration in Python

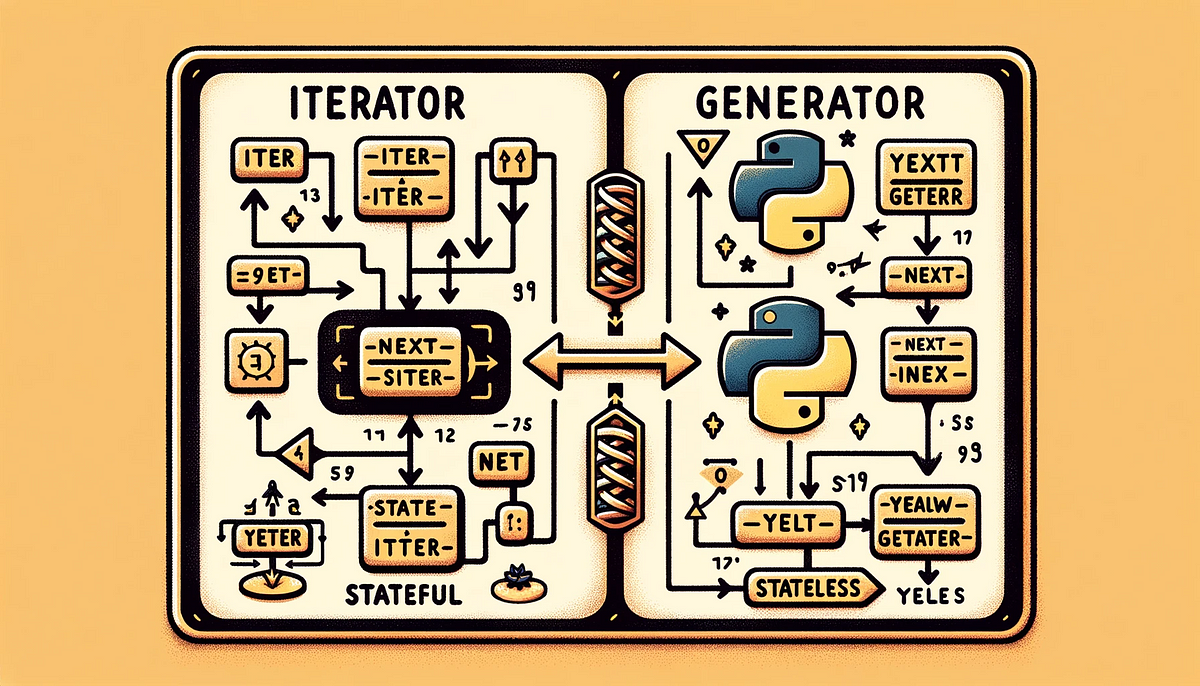
In Python, generators and iterators are key concepts that enable efficient iteration over data. These tools are invaluable for handling large datasets, streaming data, and implementing custom iteration patterns. By understanding how they work and when to use them, you can optimize your code for both performance and readability.
Understanding Iterators
An iterator is an object that allows you to traverse through all the elements in a collection, such as a list or tuple. The iterator protocol requires the implementation of two methods:
__iter__(self)
: Returns the iterator object itself and is implicitly called at the start of loops.__next__(self)
: Returns the next item from the collection. When there are no more items, it raises theStopIteration
exception.
Here’s an example of a simple iterator:
This will output the numbers from 1 to 4, demonstrating the basic workings of an iterator.
Generators: A Step Beyond Iterators
Generators are a special type of iterator that are defined using a function rather than a class. They allow you to iterate over a sequence of values lazily, meaning values are generated on-the-fly and only when needed. This makes generators ideal for working with large datasets or streams of data.
A generator is created using the yield
keyword:
This example produces the same output as the iterator example, but the generator function is much simpler and more concise.
Generator Expressions
Python also allows you to create generators using a syntax similar to list comprehensions. These are called generator expressions:
Generator expressions are a memory-efficient way to handle large computations since they yield values one at a time rather than storing them all in memory.
When to Use Generators and Iterators
Generators and iterators are particularly useful when:
You’re working with large datasets that don’t fit into memory.
You need a custom iteration logic that isn’t covered by Python’s built-in iterables.
You want to implement an algorithm that yields results incrementally, such as reading lines from a large file or streaming data from an API.
Subscribe to my newsletter
Read articles from Shrey Dikshant directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Shrey Dikshant
Shrey Dikshant
Aspiring data scientist with a strong foundation in adaptive quality techniques. Gained valuable experience through internships at YT Views, focusing on operation handling. Proficient in Python and passionate about data visualization, aiming to turn complex data into actionable insights.