Solving SEO Problems in React Applications
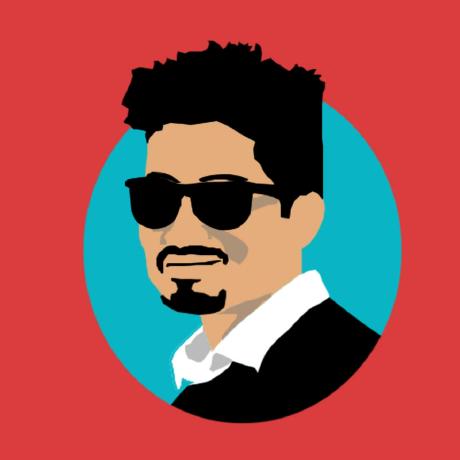
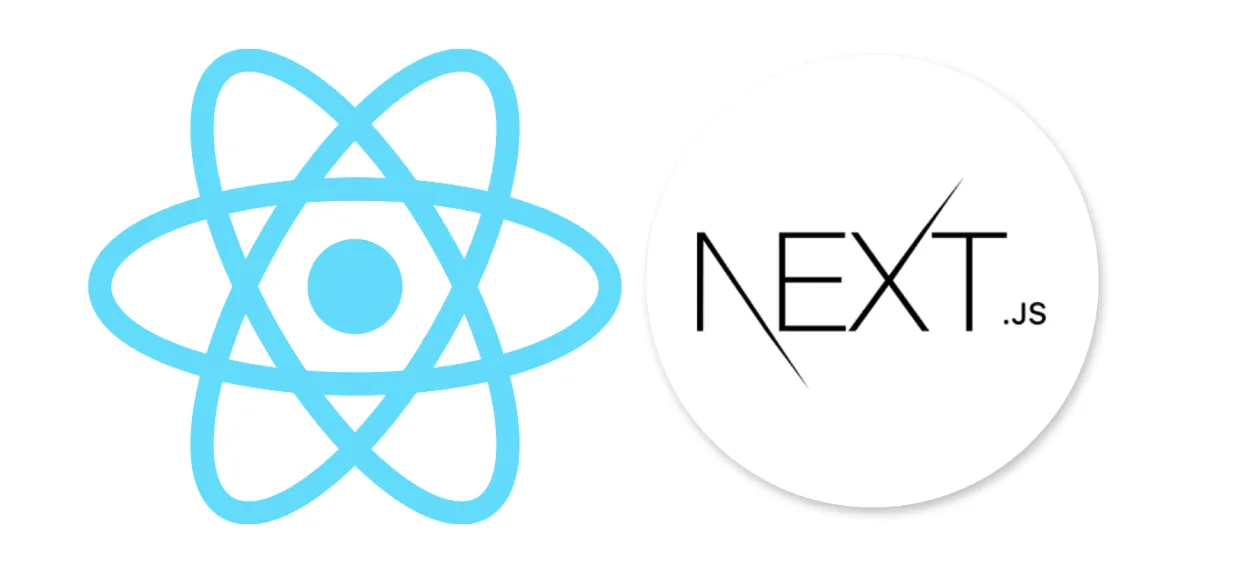
React has revolutionized front-end development with its component-based architecture and virtual DOM, offering a highly interactive user experience. However, when it comes to Search Engine Optimization (SEO), React applications face significant challenges. The primary issue arises from React’s default reliance on Client-Side Rendering (CSR), which can hinder search engine indexing and affect page performance. This post explores how combining Next.js, a React framework, with React in the same repository can address these SEO challenges effectively. We will dive into the intricacies of SSR (Server-Side Rendering) and SSG (Static Site Generation) provided by Next.js, and illustrate how this approach can enhance the performance and SEO of dynamic public dashboards, such as a listing page.
Understanding SEO Challenges in React Applications
1. Client-Side Rendering (CSR) and Its Implications for SEO
Client-Side Rendering (CSR) is a rendering technique where JavaScript code runs in the browser to generate the HTML content dynamically. While CSR provides a fluid and interactive user experience, it poses several challenges for SEO:
Delayed Content Visibility: In CSR, content is rendered on the client-side after the JavaScript code executes. Search engine bots that crawl and index your site might struggle to see and understand this content, leading to suboptimal search engine rankings.
JavaScript Execution: Search engines like Google have made significant advancements in executing JavaScript, but not all bots are as efficient. This discrepancy can result in incomplete indexing of your content.
Page Load Time: CSR can lead to slower initial page load times because the browser needs to download and execute JavaScript before rendering the content. Slow load times negatively impact user experience and SEO.
2. Google’s Ranking Algorithm and Its Requirements
Google’s ranking algorithm evaluates numerous factors to determine the quality and relevance of a webpage. Key factors include:
Crawlability: Googlebot must be able to access and index the content on your page effectively. Pages rendered entirely on the client-side may be difficult for bots to crawl, affecting your rankings.
Page Speed: Faster-loading pages contribute to a better user experience and are favored by Google. Slow pages can lead to higher bounce rates and lower rankings.
Content Relevance: High-quality, relevant content that is easily accessible and indexable is crucial for ranking well in search results.
Next.js and React: A Strategic Combination for SEO
1. Server-Side Rendering (SSR) with Next.js
Next.js, a React framework, provides the capability of Server-Side Rendering (SSR), which significantly improves SEO and performance:
Pre-rendering: With SSR, HTML is generated on the server for each request, ensuring that search engines receive fully-rendered content. This makes it easier for bots to index your pages effectively.
Initial Page Load: SSR reduces the time it takes for the content to be displayed to users. Since the server delivers pre-rendered HTML, users see content faster, which improves both SEO and user experience.
Dynamic Content Handling: Next.js can dynamically generate content on the server based on the request, making it ideal for applications with frequently updated data.
2. Static Site Generation (SSG) with Next.js
Static Site Generation (SSG) is another feature of Next.js that can be beneficial for SEO:
Static HTML Generation: SSG allows you to pre-render pages at build time. The generated HTML is served as static files, ensuring fast load times and improved SEO.
Reduced Server Load: Serving static files minimizes the load on the server, leading to faster content delivery and better scalability.
Content Freshness: Although static, SSG pages can be regenerated and updated as needed, ensuring that content remains relevant and up-to-date.
Combining Next.js with React in a Single Repository
1. Project Structure
To integrate Next.js and React in a single repository, structure your project as follows:
/pages: This directory is used for pages that will benefit from SSR or SSG. Next.js handles routing and page rendering here.
/src: Place your React application components and logic that rely on CSR in this directory. This is where you can include interactive components that do not require server-side rendering.
2. Configuration and Setup
Next.js Configuration: Set up Next.js to handle SSR and SSG. Create a
next.config.js
file to configure Next.js settings according to your needs.React Configuration: Continue to develop your React application with CSR for features that do not need server-side rendering. You can include these components in your React app and manage them separately from the Next.js setup.
Implementing a Dynamic Public Dashboard with Next.js and React
1. Use Case: Dynamic Listing Page
Consider a scenario where you need to create a dynamic listing page that displays various items. This page needs to be SEO-friendly and performant, handling frequent updates and user interactions.
Example Implementation Steps:
Create Dynamic Routes with Next.js: Use Next.js to create dynamic routes for your listing page. For instance, if you have a
listings
page, configure it to handle dynamic paths.import { useRouter } from 'next/router'; import fetch from 'node-fetch'; const ListingPage = ({ listing }) => { const router = useRouter(); if (router.isFallback) { return <div>Loading...</div>; } return ( <div> <h1>{listing.title}</h1> <p>{listing.description}</p> {/* Additional listing details */} </div> ); }; export async function getServerSideProps(context) { const res = await fetch(`https://api.example.com/listings/${context.params.id}`); const listing = await res.json(); return { props: { listing }, }; } export default ListingPage;
Implement Client-Side Interactions with React: Enhance your listing page with interactive features using React. For example, you might add filters, sorting options, or a search bar.
import { useState } from 'react'; const Filter = ({ onFilterChange }) => { const [filter, setFilter] = useState(''); const handleChange = (e) => { setFilter(e.target.value); onFilterChange(e.target.value); }; return ( <input type="text" value={filter} onChange={handleChange} placeholder="Filter listings..." /> ); }; export default Filter;
Optimize Performance and SEO: Ensure that your listing page loads quickly and is SEO-friendly by implementing best practices such as lazy loading images, minimizing JavaScript bundle sizes, and using proper meta tags.
import Document, { Html, Head, Main, NextScript } from 'next/document'; class MyDocument extends Document { render() { return ( <Html> <Head> <meta name="description" content="Find the best listings on our platform" /> <meta name="robots" content="index, follow" /> </Head> <body> <Main /> <NextScript /> </body> </Html> ); } } export default MyDocument;
Benefits of Combining Next.js and React
1. Enhanced SEO
By leveraging SSR and SSG with Next.js, you ensure that search engines receive fully-rendered HTML content, improving crawlability and indexing. This results in better search engine rankings and increased visibility.
2. Improved Performance
Combining SSR and SSG with CSR provides a balanced approach to performance. Users receive fast-loading static content while enjoying interactive features powered by React, leading to a seamless user experience.
3. Unified Development Experience
Managing both SSR and CSR in a single repository simplifies development and maintenance. You can take advantage of Next.js for SEO-critical pages while using React for dynamic and interactive components.
Conclusion
Combining Next.js with React in the same repository offers a powerful solution to the SEO challenges associated with client-side rendering. By utilizing Next.js for server-side rendering and static site generation, you can ensure that your pages are fully indexable by search engines and deliver a superior user experience. Embracing this hybrid approach not only enhances SEO but also optimizes performance, providing a robust and scalable solution for modern web development.
For more detailed guides and longer-form ebooks, visit AhmadWKhan.com.
Subscribe to my newsletter
Read articles from Ahmad W Khan directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
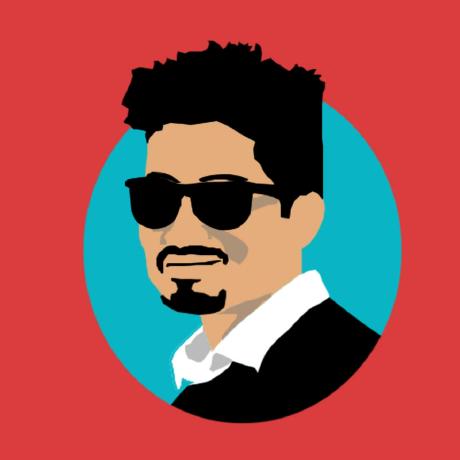