Jenkins Important Interview Questions
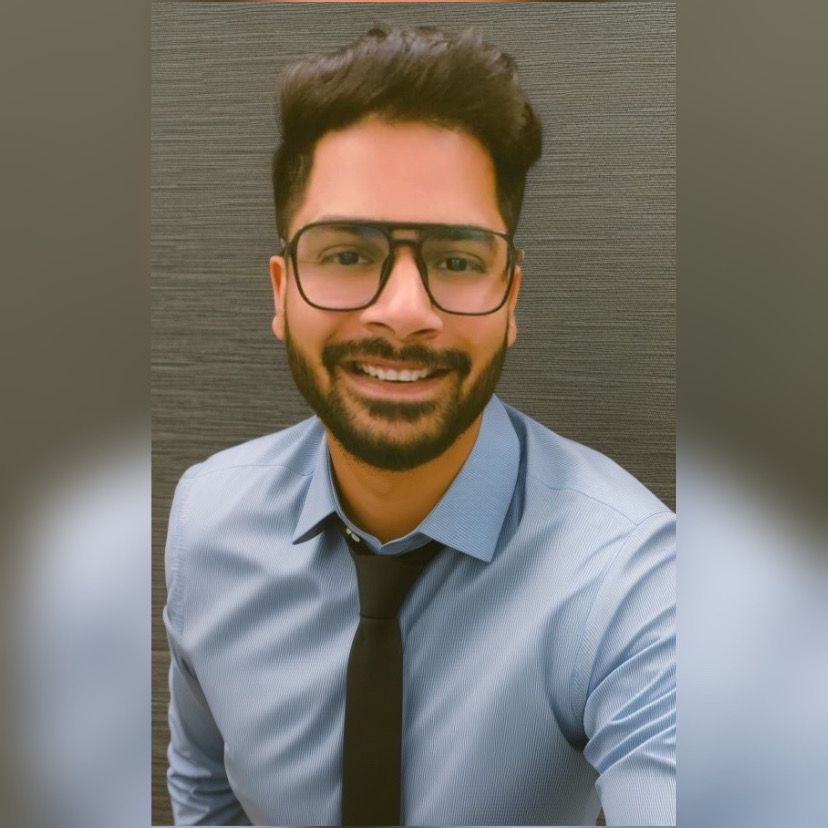
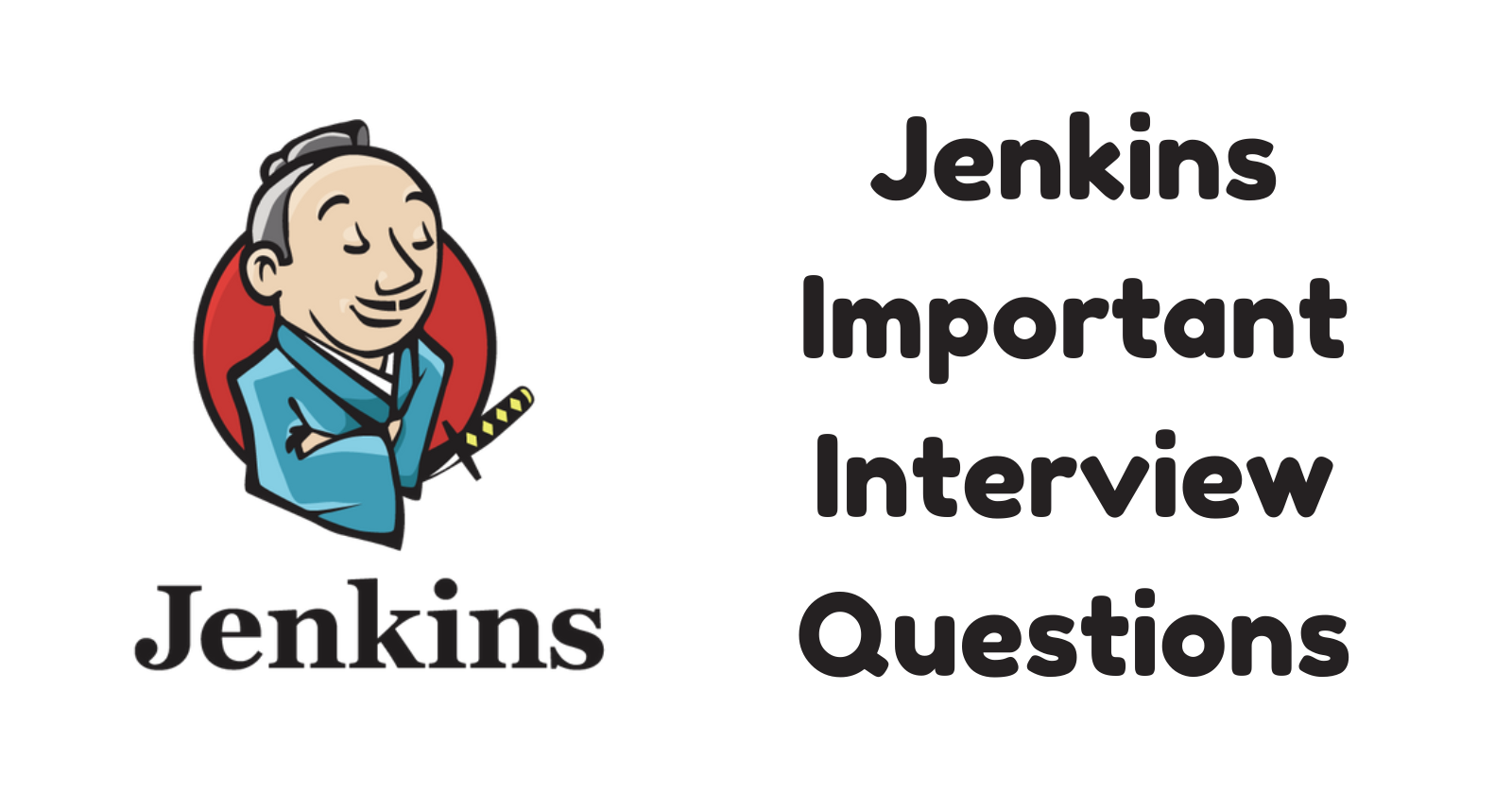
1. What’s the difference between continuous integration, continuous delivery, and continuous deployment?
Continuous Integration (CI): CI involves the frequent integration of code changes into a shared repository. Developers regularly commit code to the version control system, and automated builds and tests run to ensure that the new code integrates smoothly with the existing codebase. The main goal of CI is to detect errors early by integrating work frequently.
Continuous Delivery (CD): Continuous Delivery extends CI by ensuring that code changes can be automatically deployed to a production-like environment. The code is always in a deployable state, but the deployment is typically triggered manually. This ensures that the application can be released to production at any time with minimal effort.
Continuous Deployment: Continuous Deployment is the next step after Continuous Delivery, where every code change that passes automated tests is automatically deployed to production. This removes the need for manual approval, ensuring that the software is always up-to-date and available to users.
2. Benefits of CI/CD
Faster Time to Market: Automating the integration, testing, and deployment processes allows for faster and more frequent releases.
Improved Code Quality: Regular integration and automated testing catch bugs early, improving overall code quality.
Reduced Manual Effort: Automation reduces the manual workload, allowing developers to focus on more critical tasks.
Better Collaboration: CI/CD encourages frequent collaboration between development, operations, and QA teams, fostering a more cohesive workflow.
Lower Risk: Smaller, incremental changes reduce the risk of major issues, as problems are identified and resolved early.
Increased Flexibility: The ability to release at any time allows teams to respond quickly to changes in market demands or customer needs.
3. What is meant by CI/CD?
CI/CD stands for Continuous Integration and Continuous Delivery (or Continuous Deployment). It is a set of practices that automate the software development lifecycle (SDLC), aiming to improve the delivery speed and quality of software. CI/CD enables teams to integrate code frequently, run automated tests, and deploy code to production or staging environments, ensuring that the software is always in a deployable state.
4. What is Jenkins Pipeline?
A Jenkins Pipeline is a suite of plugins that supports implementing and integrating continuous delivery pipelines into Jenkins. It allows defining a build process, from simple build-and-test to a complex multi-step process, in code using the Pipeline DSL (Domain-Specific Language). Pipelines can be defined in two syntaxes: Declarative and Scripted.
Declarative Pipeline: Provides a more straightforward syntax that is easier to understand and use.
Scripted Pipeline: Offers more flexibility, allowing for complex logic, but is more verbose and harder to manage.
5. How do you configure a job in Jenkins?
Step 1: Log in to Jenkins and click on "New Item."
Step 2: Enter the job name and select the type of project (e.g., Freestyle project, Pipeline).
Step 3: Configure the Source Code Management (SCM) to point to the version control system (e.g., Git).
Step 4: Configure the build triggers (e.g., Poll SCM, Build periodically).
Step 5: Define the build steps (e.g., Shell commands, Invoke Ant, Maven).
Step 6: Optionally, set up post-build actions (e.g., email notifications, publish artifacts).
Step 7: Save the job configuration.
6. Where do you find errors in Jenkins?
Errors in Jenkins can be found in various places:
Console Output: When a build fails, the console output provides detailed logs of what went wrong during the build process.
Build History: The build history section displays the status of previous builds and any associated error messages.
System Log: Jenkins’ system logs provide more detailed information about errors and issues related to the Jenkins server itself.
Job-Specific Logs: Some plugins and configurations might write additional logs that can be found in the job's workspace or specific directories on the Jenkins server.
7. In Jenkins, how can you find log files?
Jenkins log files are typically located in the following directories:
Jenkins Home Directory: The primary log file (
jenkins.log
) can be found in the Jenkins home directory. This log contains information about Jenkins operations.Job Workspaces: Each Jenkins job has a workspace directory where specific build logs can be found (
$JENKINS_HOME/jobs/<job_name>/builds/
). The logs for each build are stored here.System Log: Accessible from the Jenkins UI under "Manage Jenkins" > "System Log," this provides real-time logs of Jenkins operations.
8. Jenkins workflow and write a script for this workflow?
Jenkins workflow refers to the series of steps defined in a Jenkins pipeline that governs how code is built, tested, and deployed. A sample Declarative Pipeline script is shown below:
pipeline {
agent any
stages {
stage('Checkout') {
steps {
// Checkout code from the repository
git url: 'https://github.com/example/repo.git'
}
}
stage('Build') {
steps {
// Compile or build the code
sh 'mvn clean install'
}
}
stage('Test') {
steps {
// Run unit tests
sh 'mvn test'
}
}
stage('Deploy') {
steps {
// Deploy the application
sh 'scp target/app.war user@server:/path/to/deploy'
}
}
}
post {
always {
// Notify on completion
mail to: 'team@example.com',
subject: "Build ${currentBuild.fullDisplayName}",
body: "Build completed with status: ${currentBuild.currentResult}"
}
}
}
9. How to create continuous deployment in Jenkins?
To create Continuous Deployment in Jenkins:
Step 1: Define a pipeline that includes build, test, and deployment stages.
Step 2: Set up the deployment stage to automatically deploy the code to the production environment after successful tests.
Step 3: Ensure the deployment is automated by using tools like Ansible, Docker, or Kubernetes, depending on your infrastructure.
Step 4: Remove manual approvals from the pipeline configuration.
Step 5: Test the pipeline thoroughly in a staging environment before enabling it for production.
10. How to build a job in Jenkins?
Step 1: Create a new job by clicking on "New Item."
Step 2: Configure the SCM to pull the latest code from the repository.
Step 3: Set up build triggers like Poll SCM or Webhooks to trigger builds automatically.
Step 4: Define the build steps, which could involve compiling the code, running tests, or packaging the application.
Step 5: Save the configuration and click "Build Now" to trigger the job manually.
11. Why do we use pipelines in Jenkins?
Pipelines in Jenkins are used for:
Automation: Automate the build, test, and deploy processes.
Version Control: Pipelines are defined as code, allowing for version control and easy changes.
Complex Workflows: Handle complex workflows with multiple stages and parallel executions.
Continuous Delivery: Enable continuous integration and continuous delivery, ensuring the application is always in a deployable state.
12. Is Jenkins alone sufficient for automation?
Jenkins is a powerful automation server, but it often works best when integrated with other tools. While Jenkins handles continuous integration and deployment, it may require additional tools for configuration management (e.g., Ansible), containerization (e.g., Docker), monitoring, and security. These integrations make the CI/CD process more robust and scalable.
13. How will you handle secrets in Jenkins?
Secrets in Jenkins can be handled using:
Jenkins Credentials Plugin: Store secrets like passwords, SSH keys, or API tokens securely in Jenkins and access them within pipelines.
Environment Variables: Inject secrets as environment variables during the build.
Third-Party Vaults: Integrate Jenkins with secret management tools like HashiCorp Vault to manage secrets outside Jenkins.
Encrypted Files: Store secrets in encrypted files and decrypt them only during the build process.
14. Explain the different stages in a CI/CD setup.
Source Stage: Code is committed to the version control system.
Build Stage: The code is compiled or packaged into an executable artifact.
Test Stage: Automated tests are run to ensure the code is functional.
Deploy Stage: The application is deployed to a staging or production environment.
Monitoring Stage: The deployed application is monitored to ensure it's running as expected.
Feedback Stage: Feedback is gathered from monitoring tools or users, and any issues are addressed in subsequent builds.
15. Name some of the plugins in Jenkins.
Git Plugin: Integrates Jenkins with Git repositories.
Maven Plugin: Allows Jenkins to build projects using Apache Maven.
Docker Plugin: Integrates Docker with Jenkins for building and deploying containers.
Pipeline Plugin: Enables defining jobs as code using a DSL.
Credentials Plugin: Manages credentials securely within Jenkins.
Blue Ocean: Provides a modern user interface for Jenkins pipelines.
Slack Notification Plugin: Sends build notifications to Slack channels.
Scenario-Based Question
1. You have a Jenkins pipeline that deploys to a staging environment. Suddenly, the deployment failed due to a missing configuration file. How would you troubleshoot and resolve this issue?
Step 1: Check the pipeline's console output for error messages related to the missing configuration file.
Step 2: Identify the stage where the failure occurred and examine the code or script responsible for that stage.
Step 3: Verify that the configuration file exists in the correct location within the repository or on the server.
Step 4: Ensure that the file is correctly referenced in the pipeline script.
Step 5: If the file is missing, restore it from version control or recreate it based on documentation.
Step 6: Rerun the pipeline to confirm the issue is resolved.
2. Imagine you have a Jenkins job that is taking significantly longer to complete than expected. What steps would you take to identify and mitigate the issue?
Step 1: Review the console output to identify stages that are taking longer than expected.
Step 2: Check for bottlenecks in the build process, such as resource-intensive tasks or network delays.
Step 3: Analyze system resource usage (CPU, memory, disk I/O) on the Jenkins server and agents.
Step 4: Consider optimizing the code or build process, such as parallelizing tasks or caching dependencies.
Step 5: Scale the Jenkins environment by adding more agents or optimizing existing ones.
Step 6: Review and adjust any timeouts or limits configured in Jenkins or related tools.
3. You need to implement a secure method to manage environment-specific secrets for different stages (development, staging, production) in your Jenkins pipeline. How would you approach this?
Step 1: Use the Jenkins Credentials Plugin to store secrets securely within Jenkins.
Step 2: Create separate credentials for each environment (development, staging, production).
Step 3: Use environment-specific variables in the pipeline to access the correct credentials based on the deployment stage.
Step 4: Consider using a third-party secret management tool like HashiCorp Vault for more complex scenarios, where secrets are managed externally.
Step 5: Ensure that access to secrets is restricted to only those who need it, using appropriate role-based access controls.
4. Suppose your Jenkins master node is under heavy load and build times are increasing. What strategies can you use to distribute the load and ensure efficient build processing?
Step 1: Add more Jenkins agents to distribute the load across multiple machines.
Step 2: Implement a Jenkins master-agent architecture where the master handles orchestration, and agents handle the actual build processes.
Step 3: Optimize the build process by parallelizing tasks and using pipeline stages effectively.
Step 4: Schedule non-critical jobs during off-peak hours to reduce load during peak times.
Step 5: Consider using a cloud-based Jenkins setup with auto-scaling capabilities to handle varying loads.
5. A developer commits a code change that breaks the build. How would you set up Jenkins to automatically handle such scenarios and notify the relevant team members?
Step 1: Configure Jenkins to run automated tests on each commit using the CI pipeline.
Step 2: Set up post-build actions to send email notifications or Slack messages to the relevant team members when a build fails.
Step 3: Use tools like SonarQube or static analysis plugins to catch potential issues before they break the build.
Step 4: Implement a "fail fast" strategy where the pipeline aborts as soon as a critical error is detected.
Step 5: Consider setting up automated rollbacks or reverts for failed builds to ensure the main branch remains stable.
6. You are tasked with setting up a Jenkins pipeline for a multi-branch project. How would you handle different configurations and build steps for different branches?
Step 1: Use the Jenkins Multibranch Pipeline Plugin to automatically discover and create jobs for each branch.
Step 2: Define branch-specific configurations within the Jenkinsfile using conditionals (e.g.,
if (env.BRANCH_NAME == 'master')
).Step 3: Set up different stages and steps based on the branch name, ensuring that production branches (e.g.,
master
,release
) have more rigorous testing and deployment steps.Step 4: Use environment variables and credentials specific to each branch to manage environment-specific configurations.
Step 5: Review and test the pipeline for each branch to ensure that it behaves as expected.
7. How would you implement a rollback strategy in a Jenkins pipeline to revert to a previous stable version if the deployment fails?
Step 1: Store each successful deployment artifact (e.g., Docker image, WAR file) with a unique version or tag.
Step 2: In the pipeline, include a rollback stage that triggers when a deployment fails.
Step 3: Use the previous stable artifact to redeploy the application to the target environment.
Step 4: Test the rollback process in a staging environment to ensure it works as expected.
Step 5: Include notifications in the rollback stage to inform the team of the rollback action.
8. In a scenario where you have multiple teams working on different projects, how would you structure Jenkins jobs and pipelines to ensure efficient resource utilization and manage permissions?
Step 1: Organize jobs and pipelines into folders or views based on teams or projects to keep things organized.
Step 2: Use Jenkins roles and permissions to control access to jobs, ensuring that only authorized users can modify or execute jobs.
Step 3: Set up shared Jenkins agents that can be used by multiple teams, but use labels to control which jobs run on which agents.
Step 4: Monitor resource usage and allocate resources (e.g., CPU, memory) to ensure that no single team monopolizes the Jenkins environment.
Step 5: Implement quotas or limits on build concurrency to prevent resource contention.
9. Your Jenkins agents are running in a cloud environment, and you notice that build times fluctuate due to varying resource availability. How would you optimize the performance and cost of these agents?
Step 1: Implement auto-scaling for Jenkins agents based on demand, ensuring that the right number of agents are available when needed.
Step 2: Use spot instances or reserved instances to reduce costs, depending on the workload requirements.
Step 3: Optimize build processes to reduce resource consumption, such as caching dependencies or parallelizing tasks.
Step 4: Monitor and analyze build times to identify patterns and adjust agent configurations accordingly.
Step 5: Consider using a mix of on-demand and reserved/spot instances to balance cost and performance.
Thank you for taking the time to read! 💚
Subscribe to my newsletter
Read articles from Rajat Chauhan directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
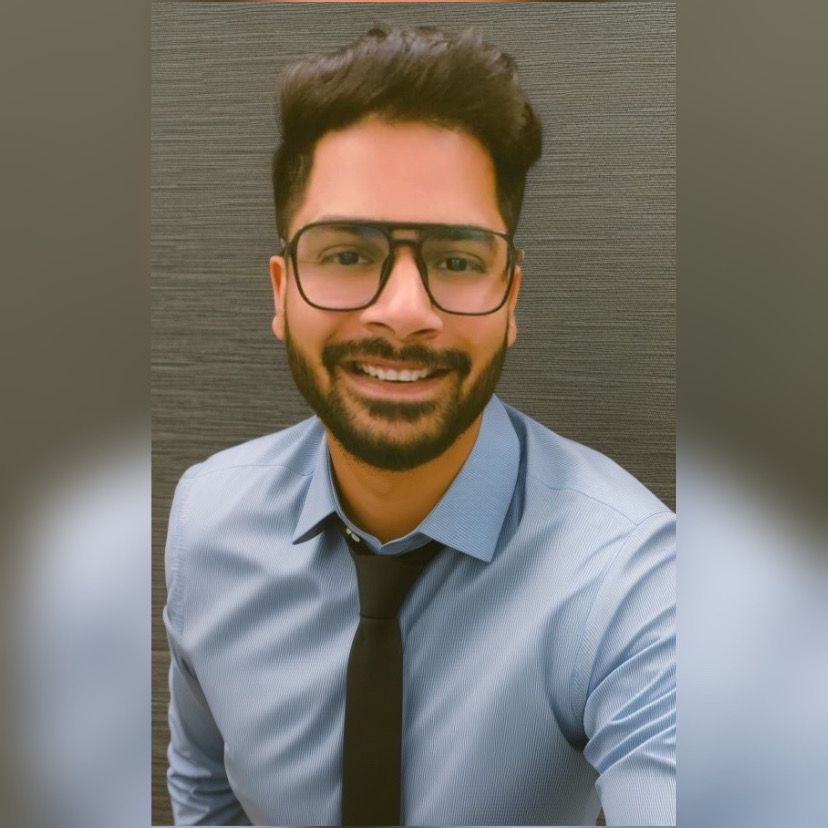
Rajat Chauhan
Rajat Chauhan
Rajat Chauhan is a skilled Devops Engineer, having experience in automating, configuring, deploying releasing and monitoring the applications on cloud environment. • Good experience in areas of DevOps, CI/CD Pipeline, Build and Release management, Hashicorp Terraform, Containerization, AWS, and Linux/Unix Administration. • As a DevOps Engineer, my objective is to strengthen the company’s applications and system features, configure servers and maintain networks to reinforce the company’s technical performance. • Ensure that environment is performing at its optimum level, manage system backups and provide infrastructure support. • Experience working on various DevOps technologies/ tools like GIT, GitHub Actions, Gitlab, Terraform, Ansible, Docker, Kubernetes, Helm, Jenkins, Prometheus and Grafana, and AWS EKS, DevOps, Jenkins. • Positive attitude, strong work ethic, and ability to work in a highly collaborative team environment. • Self-starter, Fast learner, and a Team player with strong interpersonal skills • Developed shell scripts (Bash) for automating day-to-day maintenance tasks on top of that have good python scripting skills. • Proficient in communication and project management with good experience in resolving issues.