๐ Simplifying Web Development with Lit Components: JavaScript Web Components Made Easier
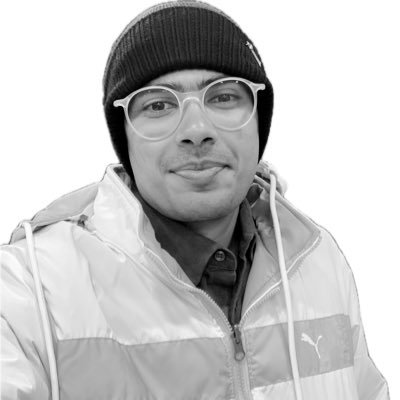

In the evolving landscape of web development, the need for reusable, modular, and efficient components has become paramount. While JavaScript Web Components provide a powerful solution to these needs, they often come with a steep learning curve. This is where Lit Components step in, simplifying the creation and use of Web Components with a lightweight and easy-to-use library. In this blog post, weโll explore what Lit Components are, why theyโre gaining popularity, and how you can start using them to simplify your web development projects.
๐ What Are Lit Components?
Lit Components are a modern library that builds on top of the Web Components standard. They make it easier to create custom HTML elements with a simplified API. Lit Components help developers avoid some of the boilerplate code and complexity associated with traditional Web Components, offering a more streamlined development experience.
๐ Key Features of Lit Components
โจ Lightweight: Lit Components are incredibly lightweight, ensuring fast load times and minimal performance overhead.
๐ Declarative Rendering: Lit uses a template-based rendering approach, making your code more readable and maintainable.
โก Reactivity: Built-in reactive properties automatically update your UI when data changes.
๐ Compatibility: Since Lit builds on the Web Components standard, components are fully compatible with all modern browsers and can be used with other libraries and frameworks.
๐ Why Choose Lit Components?
๐ฏ 1. Ease of Use
Lit Components simplify the process of creating Web Components by removing much of the boilerplate code. This means you can get up and running faster, with less code to write and maintain.
Example: Hereโs how you can create a simple counter component with Lit:
import { LitElement, html, css } from 'lit';
class MyCounter extends LitElement {
static properties = {
count: { type: Number }
};
static styles = css`
button {
font-size: 18px;
padding: 10px;
margin: 5px;
}
`;
constructor() {
super();
this.count = 0;
}
render() {
return html`
<div>
<button @click="${this._increment}">Increment</button>
<p>Count: ${this.count}</p>
</div>
`;
}
_increment() {
this.count += 1;
}
}
customElements.define('my-counter', MyCounter);
In just a few lines of code, you have a fully functional, interactive counter component!
โ๏ธ 2. Reactivity Made Simple
With Litโs built-in reactivity, your components automatically update when properties change. This reduces the need for complex state management and makes it easier to keep your UI in sync with your data.
Example: Reactive properties in Lit:
static properties = {
count: { type: Number }
};
Whenever count
is updated, the UI automatically re-renders, ensuring that your componentโs state is always reflected in the DOM.
๐ 3. Declarative and Readable Code
Lit Components use tagged template literals to define your componentโs HTML structure. This makes your code more declarative, easier to read, and less prone to errors.
Example: The html
template tag in Lit:
render() {
return html`
<p>Hello, Lit Components!</p>
`;
}
๐ 4. Compatibility with Modern Frameworks
Since Lit Components adhere to the Web Components standard, theyโre compatible with popular frameworks like React, Angular, and Vue. This allows you to integrate Lit Components into your existing projects without any hassle.
๐ 5. Performance Optimization
Lit Components are optimized for performance out of the box. The library is lightweight, and its fine-grained reactivity ensures that only the parts of the DOM that need to change are updated, leading to faster rendering and better performance.
๐ Getting Started with Lit Components
๐ ๏ธ Step 1: Installation
You can easily add Lit Components to your project using npm:
npm install lit
๐งโ๐ป Step 2: Creating Your First Lit Component
Start by creating a new JavaScript file for your component. Import the necessary modules from Lit and define your custom component:
import { LitElement, html, css } from 'lit';
class MyFirstComponent extends LitElement {
render() {
return html`<p>Welcome to my first Lit component!</p>`;
}
}
customElements.define('my-first-component', MyFirstComponent);
๐ Step 3: Using Your Component in HTML
Once your component is defined, you can use it just like any other HTML element:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Lit Components Example</title>
<script type="module" src="my-first-component.js"></script>
</head>
<body>
<my-first-component></my-first-component>
</body>
</html>
๐ ๏ธ Step 4: Enhancing Your Component
From here, you can start adding styles, properties, and methods to your Lit Component, making it as simple or as complex as needed for your application.
๐ค
Lit Components offer a powerful yet simplified way to create custom web components. By reducing the boilerplate code and complexity typically associated with Web Components, Lit makes it easier for developers of all levels to create reusable, modular, and performant components.
Whether youโre building a simple widget or a complex application, Lit Components can help you achieve your goals more efficiently. Start exploring Lit Components today and see how they can simplify your web development process!
Happy coding! ๐
Feel free to leave comments or questions below! ๐ฌ
Subscribe to my newsletter
Read articles from Vivek directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
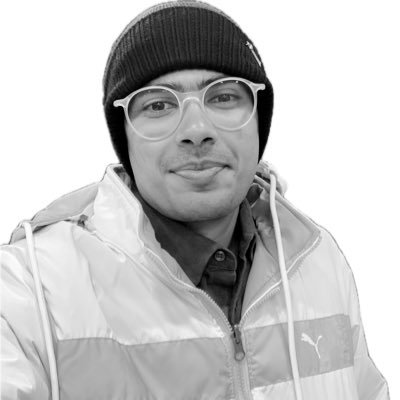
Vivek
Vivek
Curious Full Stack Developer wanting to try hands on โจ๏ธ new technologies and frameworks. More leaning towards React these days - Next, Blitz, Remix ๐จ๐ปโ๐ป