Today I Learned About Promises in JavaScript: A Beginner's Guide

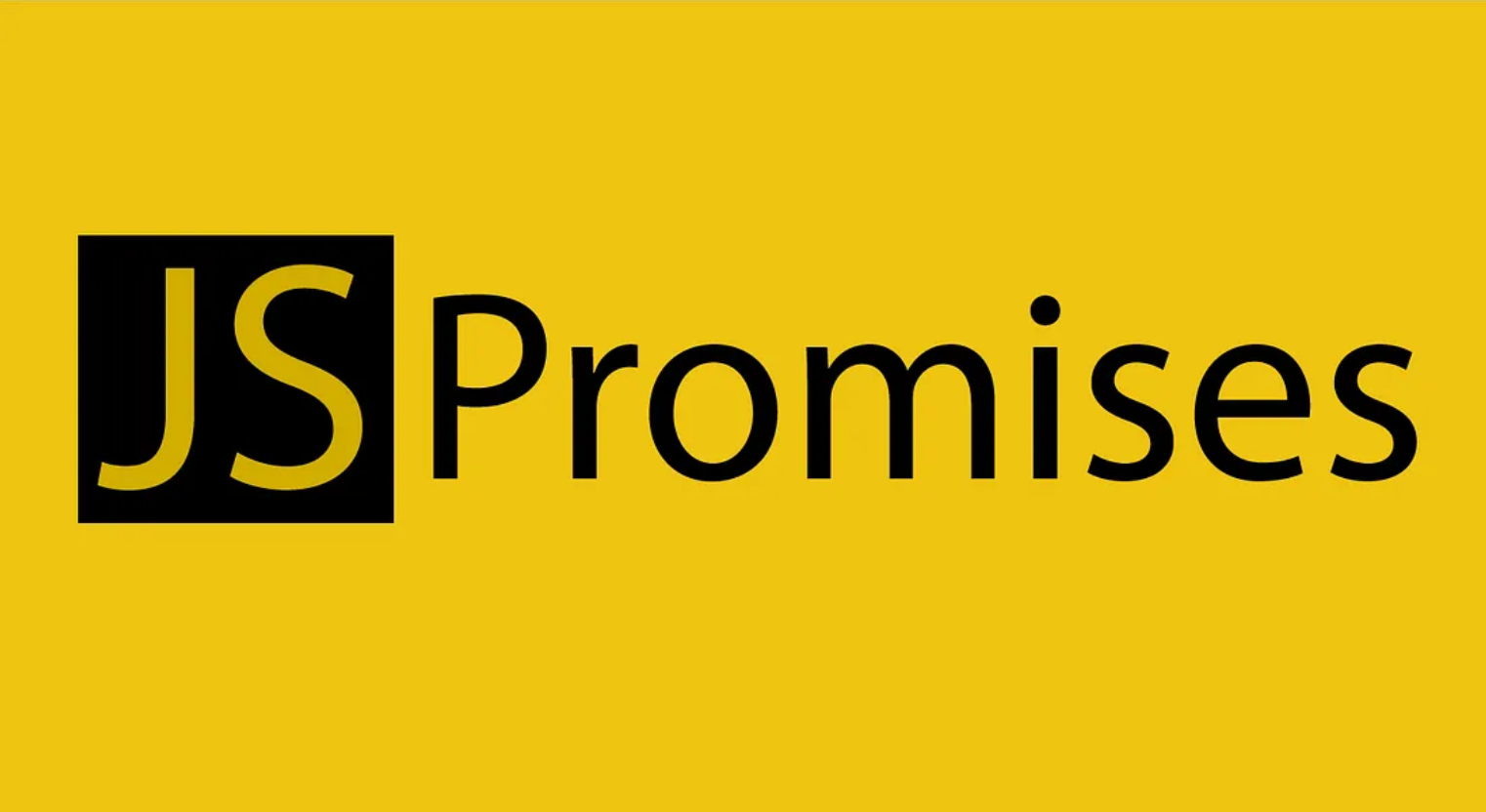
Introduction:
Hey everyone! Today, I had the chance to learn about something super important in JavaScript—promises. If you've been working with asynchronous code and have found yourself tangled in a mess of callbacks, promises are going to be your new best friend.
In this post, I'll break down what promises are, why they're so important, and how they can make your code cleaner and easier to manage. But first, let me give a shoutout to the amazing resources that helped me grasp this concept.
Where I Learned About Promises:
Akshay Saini's Namaste JavaScript Season 2 - Promises Video: This video is a fantastic introduction to promises. Akshay breaks down the concept in a way that's easy to understand, especially if you're new to the topic. Check it out here.
Hitesh Choudhary's Chai Aur JavaScript - Promises: Hitesh's casual and relatable style makes learning about promises fun. He explains everything while sipping on chai, making it a relaxed and informative session. Watch it here.
MDN Web Docs: No learning journey is complete without checking out the official documentation. The MDN Web Docs on promises provide a detailed and thorough explanation of how promises work in JavaScript. Read the docs here.
The combination of these three resources is absolutely lajawab (fantastic)! If you're looking to get a solid understanding of promises, I highly recommend checking them all out.
What is a Promise?
So, what exactly is a promise? In JavaScript, a promise is an object that represents the eventual completion (or failure) of an asynchronous operation. Think of it like a promise in real life—something that will be fulfilled or rejected in the future.
Why Do We Need Promises?
JavaScript is single-threaded, meaning it can only do one thing at a time. But what happens when you need to perform a task that takes time, like fetching data from an API? You don't want to block the whole program while waiting for that task to finish. This is where asynchronous operations come into play, allowing the rest of the code to keep running while waiting for the task to complete.
But managing these asynchronous operations with callbacks can get messy quickly. You might end up with deeply nested callbacks, also known as "callback hell." That's why we need promises—they make it easier to work with asynchronous code and keep things neat and tidy.
How Promises Work
A promise can be in one of three states:
Pending: The initial state, where the promise is neither fulfilled nor rejected.
Fulfilled: The operation completed successfully, and the promise is resolved with a value.
Rejected: The operation failed, and the promise is rejected with a reason (error).
Why Use Promises?
Here are some reasons why promises are a better choice for handling asynchronous operations:
Avoid Callback Hell: Promises help you avoid the deeply nested callbacks that can make your code hard to read and maintain.
// Callback Hell Example asyncOperation1(function(result1) { asyncOperation2(result1, function(result2) { asyncOperation3(result2, function(result3) { // More nested callbacks... }); }); });
Chaining: Promises allow you to chain operations together, making your code more linear and easier to follow.
// Promise Chaining asyncOperation1() .then(result1 => asyncOperation2(result1)) .then(result2 => asyncOperation3(result2)) .then(result3 => { // Final result }) .catch(error => { // Handle any error that occurred in the chain });
Error Handling: With promises, you can handle errors in a single
.catch()
block, which makes your error handling logic cleaner.Cleaner Syntax with Async/Await: Promises work seamlessly with
async/await
syntax, making your asynchronous code look and behave more like synchronous code.
Conclusion: Learning about promises has been a game-changer for me, and I'm excited to keep exploring this concept further. If you're working with asynchronous code in JavaScript, understanding promises is essential. I hope this post helps you get started, and I highly recommend checking out the resources I mentioned earlier—they're absolutely top-notch!
Happy coding! 🚀
Subscribe to my newsletter
Read articles from Bhavesh Jadhav directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Bhavesh Jadhav
Bhavesh Jadhav
I am a passionate web developer from India, currently pursuing an MCA from Government College of Engineering, Aurangabad. With a strong foundation in HTML, CSS, JavaScript, and expertise in frameworks like React and Node.js, I create dynamic and responsive web applications. My skill set extends to backend development with PHP, MySQL, and MongoDB. I also have experience with AJAX, jQuery, and I am proficient in Python and Java. As an AI enthusiast, I enjoy leveraging AI tools and LLMs to enhance my projects. Eager to expand my horizons, I am delving into DevOps to optimize development workflows and improve deployment processes. Always keen to learn and adapt, I strive to integrate cutting-edge technologies into my work. Let's connect and explore the world of technology together!