How to Convert Voice to Text: A Comprehensive Guide
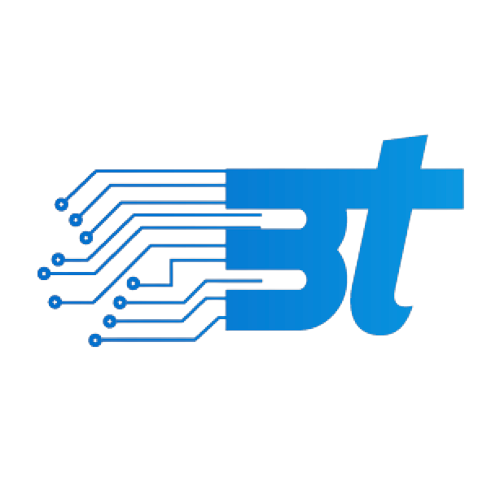
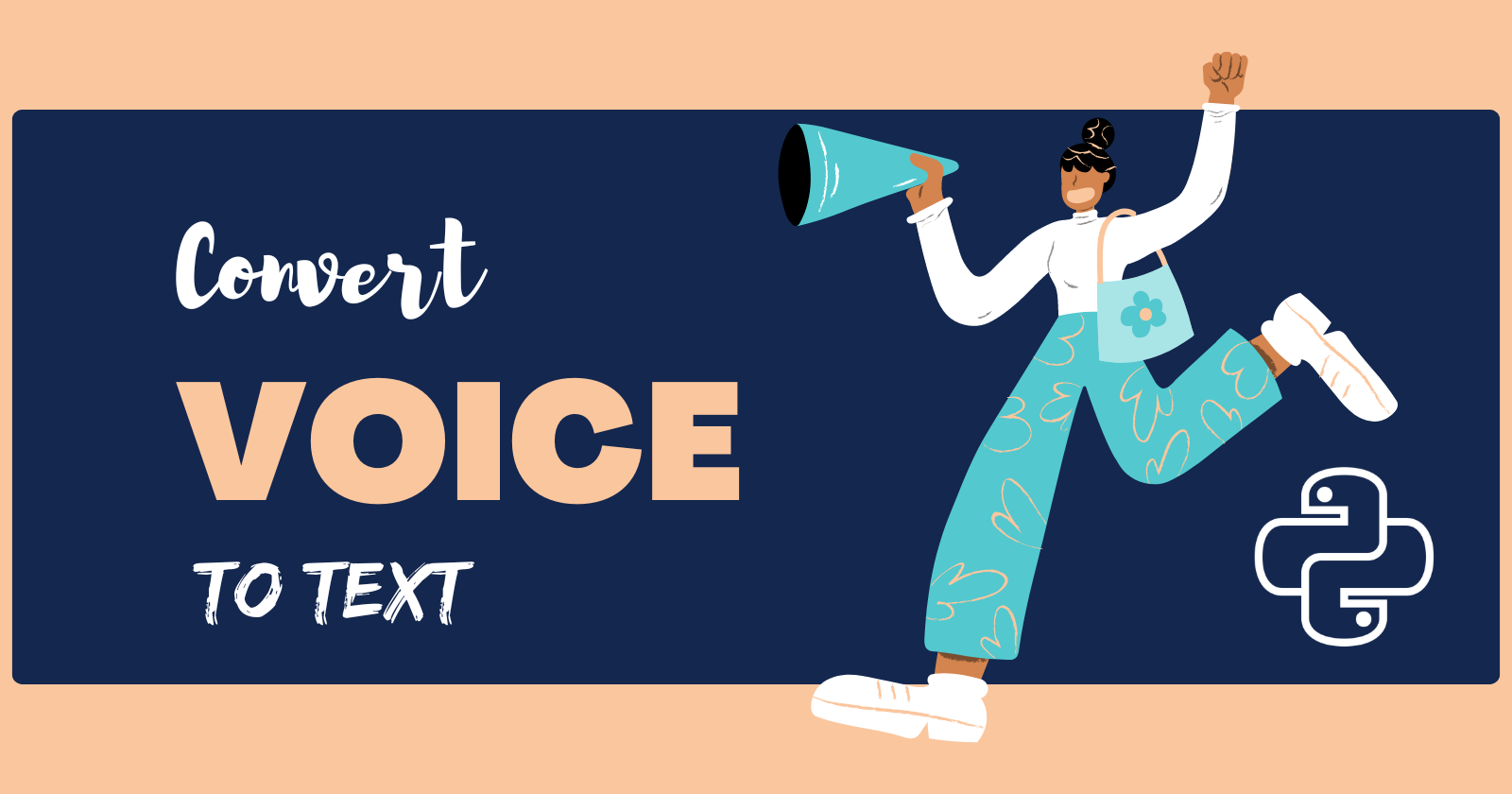
In today's digital world, converting voice into text has become an essential task for various applications, from transcription services to voice-controlled applications. Whether you're building a voice assistant or simply transcribing audio recordings, there are several ways to achieve this. In this blog, we'll explore various methods to convert voice to text using different libraries and APIs in Python.
1. Using the SpeechRecognition
Library
The SpeechRecognition
library is one of the most popular and straightforward tools for converting speech to text in Python. It supports several APIs and engines, including Google's Web Speech API, which is used by default.
Installation
To get started, you'll need to install the necessary libraries:
pip install SpeechRecognition pyaudio
Basic Implementation
Here's a simple example of how to use the SpeechRecognition
library to convert voice to text:
import speech_recognition as sr
# Initialize recognizer
recognizer = sr.Recognizer()
# Capture voice from microphone
with sr.Microphone() as source:
print("Please say something...")
audio = recognizer.listen(source)
# Convert voice to text
try:
text = recognizer.recognize_google(audio)
print("You said: " + text)
except sr.UnknownValueError:
print("Sorry, I could not understand the audio")
except sr.RequestError as e:
print("Could not request results; {0}".format(e))
Troubleshooting PyAudio
Issues
If you encounter the AttributeError: Could not find PyAudio; check installation
, it usually indicates that the PyAudio
module is not installed correctly. Here's how you can fix it:
For Windows:
Install it using pip:
pip install PyAudio‑0.2.11‑cp39‑cp39‑win_amd64.whl
For macOS:
Install using Homebrew:
brew install portaudio pip install pyaudio
For Linux:
Install the dependencies:
sudo apt-get install python3-pyaudio pip install pyaudio
2. Using Vosk: Offline Speech Recognition
If you need an offline solution, Vosk is a fantastic choice. Vosk supports multiple languages and is highly efficient, making it suitable for a wide range of applications, even on embedded systems.
Installation
To use Vosk, you'll need to install the Vosk library along with sounddevice
for audio capture:
pip install vosk sounddevice
Using Vosk for Speech Recognition
Here's how you can use Vosk to convert voice to text:
import sounddevice as sd
import vosk
import queue
import json
# Load the Vosk model
model = vosk.Model("path_to_vosk_model")
q = queue.Queue()
def callback(indata, frames, time, status):
q.put(bytes(indata))
# Initialize microphone input
with sd.RawInputStream(samplerate=16000, blocksize=8000, dtype='int16',
channels=1, callback=callback):
rec = vosk.KaldiRecognizer(model, 16000)
print("Please say something...")
while True:
data = q.get()
if rec.AcceptWaveform(data):
result = rec.Result()
text = json.loads(result).get("text", "")
print("You said:", text)
break
Vosk is particularly useful when you need to perform speech recognition without relying on internet connectivity.
3. Using Whisper by OpenAI
Whisper is a powerful speech recognition model developed by OpenAI. It's known for its accuracy and versatility, but it may be slower compared to other models due to its deep learning architecture.
Installation
To use Whisper, you’ll need to install the library and FFmpeg:
pip install git+https://github.com/openai/whisper.git
Additionally, you need to install FFmpeg:
On macOS:
brew install ffmpeg
On Ubuntu:
sudo apt-get install ffmpeg
Using Whisper for Speech Recognition
Here's how you can use Whisper to convert voice to text:
import whisper
model = whisper.load_model("base")
# Transcribe directly from a file
result = model.transcribe("path_to_audio_file.wav")
print("You said:", result["text"])
Whisper is ideal for scenarios where you need a highly accurate transcription, even in challenging audio conditions.
4. Using AssemblyAI API
AssemblyAI is an easy-to-use API that offers advanced speech recognition features like speaker diarization, sentiment analysis, and more. It's a great option for developers looking for a robust, cloud-based solution.
Setup
Get an API Key from the AssemblyAI website.
Install requests library if you haven't already:
pip install requests
Using AssemblyAI for Speech Recognition
Here's how you can use AssemblyAI to convert voice to text:
import requests
# Upload your audio file
headers = {
"authorization": "YOUR_API_KEY",
"content-type": "application/json"
}
audio_url = "https://storage.googleapis.com/path_to_your_audio_file.wav"
response = requests.post("https://api.assemblyai.com/v2/transcript", json={"audio_url": audio_url}, headers=headers)
transcript_id = response.json()['id']
# Retrieve the transcription
response = requests.get(f"https://api.assemblyai.com/v2/transcript/{transcript_id}", headers=headers)
print("You said:", response.json()['text'])
AssemblyAI’s API is a powerful tool for developers who need more than just basic speech recognition. It supports a wide range of features, making it a versatile choice for complex applications.
5. External Tools
For those looking for more advanced or specialized tools, commercial options like Dragon NaturallySpeaking or IBM Watson Speech to Text are available. These tools are often used in professional settings for their accuracy and additional features, such as speaker identification and language model customization.
IBM Watson Speech to Text
IBM Watson offers a robust speech-to-text service with capabilities such as real-time transcription, speaker diarization, and custom language models.
Dragon NaturallySpeaking
Dragon NaturallySpeaking is a premium tool designed for high-accuracy dictation and transcription, widely used in medical and legal industries.
Conclusion
SpeechRecognition
library to advanced APIs like AssemblyAI and Whisper, there's a solution for every use case.Whether you're building a voice-controlled application, transcribing interviews, or simply experimenting with voice-to-text technology, the methods discussed in this blog will help you get started quickly and effectively.
By exploring these various methods, you can choose the one that best fits your needs and start integrating voice-to-text capabilities into your projects today.
Subscribe to my newsletter
Read articles from ByteScrum Technologies directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
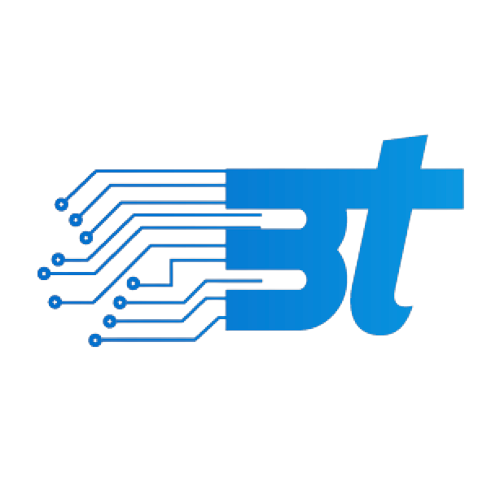
ByteScrum Technologies
ByteScrum Technologies
Our company comprises seasoned professionals, each an expert in their field. Customer satisfaction is our top priority, exceeding clients' needs. We ensure competitive pricing and quality in web and mobile development without compromise.