Kotlin Flow and Flow Builders
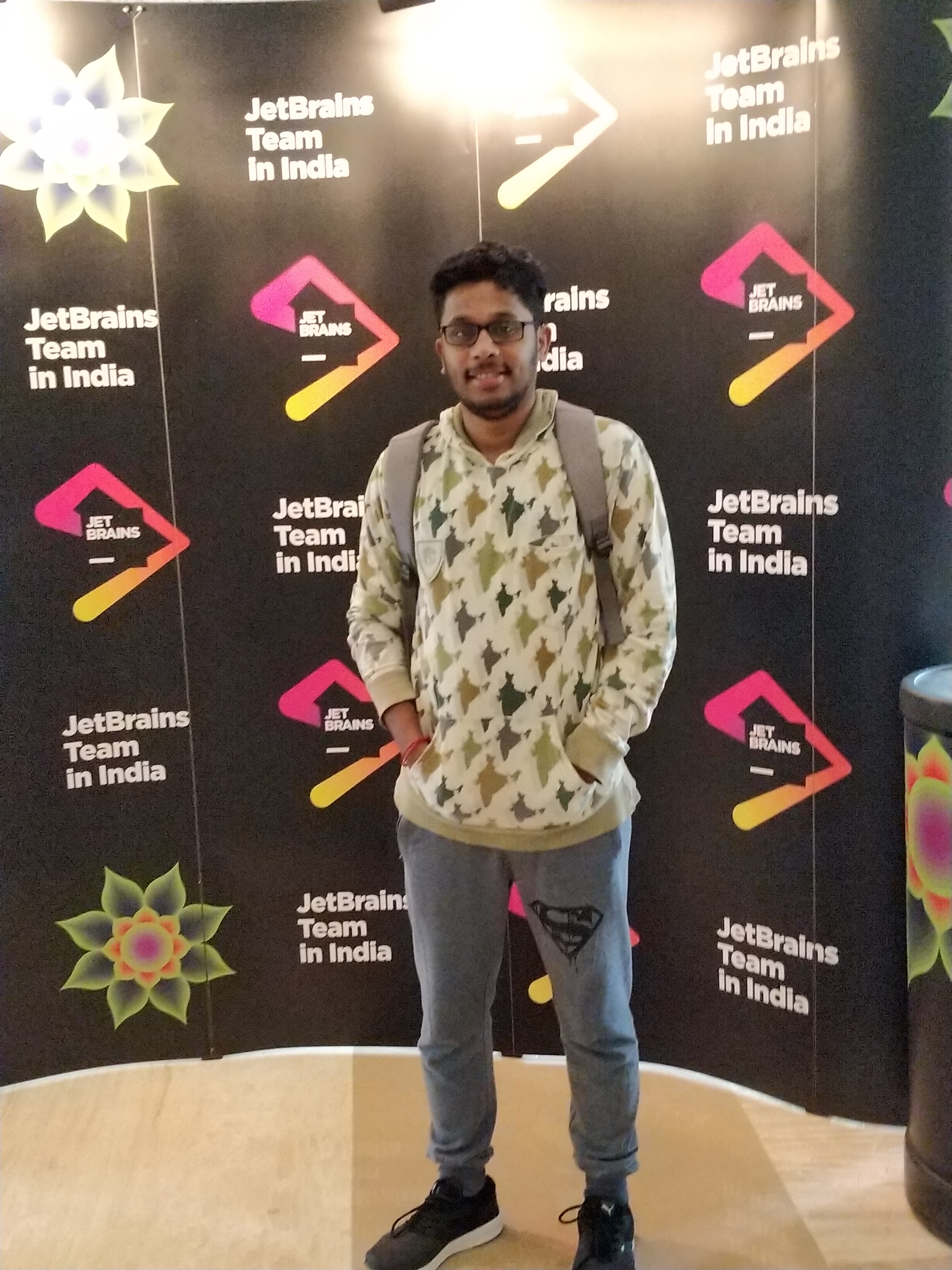
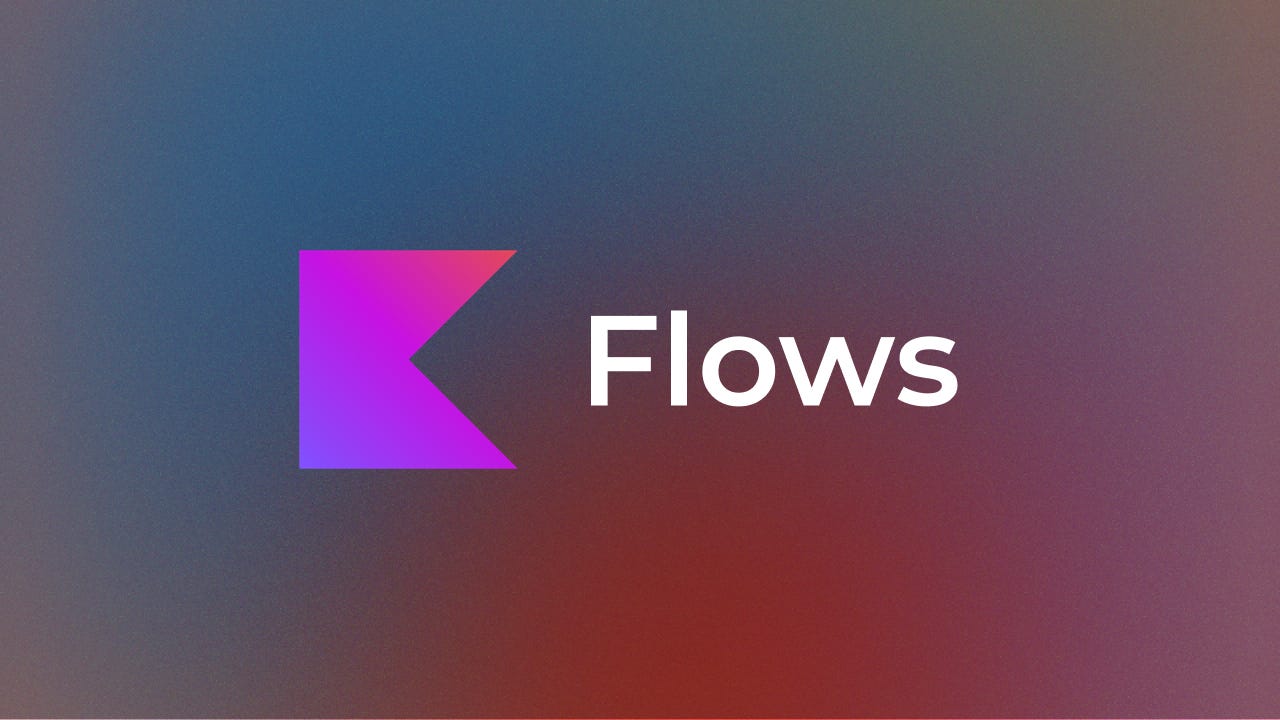
In this series of articles , we will discuss about Kotlin flows and in this article we will discuss about Flows and Flow Builders
Code gist can be found in https://gist.github.com/vprabhu/cf5f6407516f0bc97d764c7a9961e3cd
Whats is Flow ?
Already we know that suspend function asynchronously returns a single value .
Flows can return multiple asynchronously computed values
Flow Builders
we can build a flow using one of the following ways
<T> flowOf(value : T)
Creates a flow from fixed set of any values which is it can emit both a single type value and multiple data type values as follows
/**
* flowOf() -> Create a flow from fixed set of values
*/
val singleValueFlow = flowOf<Int>(578943).collect { value ->
println("Emitted Value : $value") // output : 578943
}
/*
* flowOf() -> creating a flow with multiple data types
*/
val multiValueFlows = flowOf<Any>(23, 2.90, false, "Android 1.0", 09.2008)
multiValueFlows.collect { value ->
println("multiValueFlows -> Emitted Value : $value")
//output :
//multiValueFlows -> Emitted Value : 23
//multiValueFlows -> Emitted Value : 2.9
//multiValueFlows -> Emitted Value : false
//multiValueFlows -> Emitted Value : Android 1.0
//multiValueFlows -> Emitted Value : 9.2008
}
2.<T> Iterable<T>.asFlow()
Extension function for various types of iterators to convert them into flows and it cal also emit multiple data type values
/**
* asFlow() -> extension function on various types to convert them into Flows
*/
listOf("A", "B", "C", 4, true, 90.89).asFlow().collect { value ->
println("AsFlow Emitted Value : $value")
//output :
//AsFlow Emitted Value : A
//AsFlow Emitted Value : B
//AsFlow Emitted Value : C
//AsFlow Emitted Value : 4
//AsFlow Emitted Value : true
//AsFlow Emitted Value : 90.89
}
3.<T> flow(block: suspend <T>.() -> Unit)
Creates a flow from suspendable code block given
/**
* flow {..} -> creates a flow from the suspendable block given
*/
flow {
kotlinx.coroutines.delay(2000)
emit(890)
emit(returnSomething())
}.collect { value ->
println("flow {} Emitted Value : $value")
// output :
// flow {} Emitted Value : 890
// flow {} Emitted Value : 340
}
fun returnSomething(): Int {
return 340
}
Important Considerations:
Simply put
collect{ .. } -> Terminal flow operator that collects the given flow
emit() -> Collects the value emitted by the upstream.
we will discuss this is further articles
Please leave your comments to improve.
Happy and Enjoy coding
Subscribe to my newsletter
Read articles from Vignesh Prabhu directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
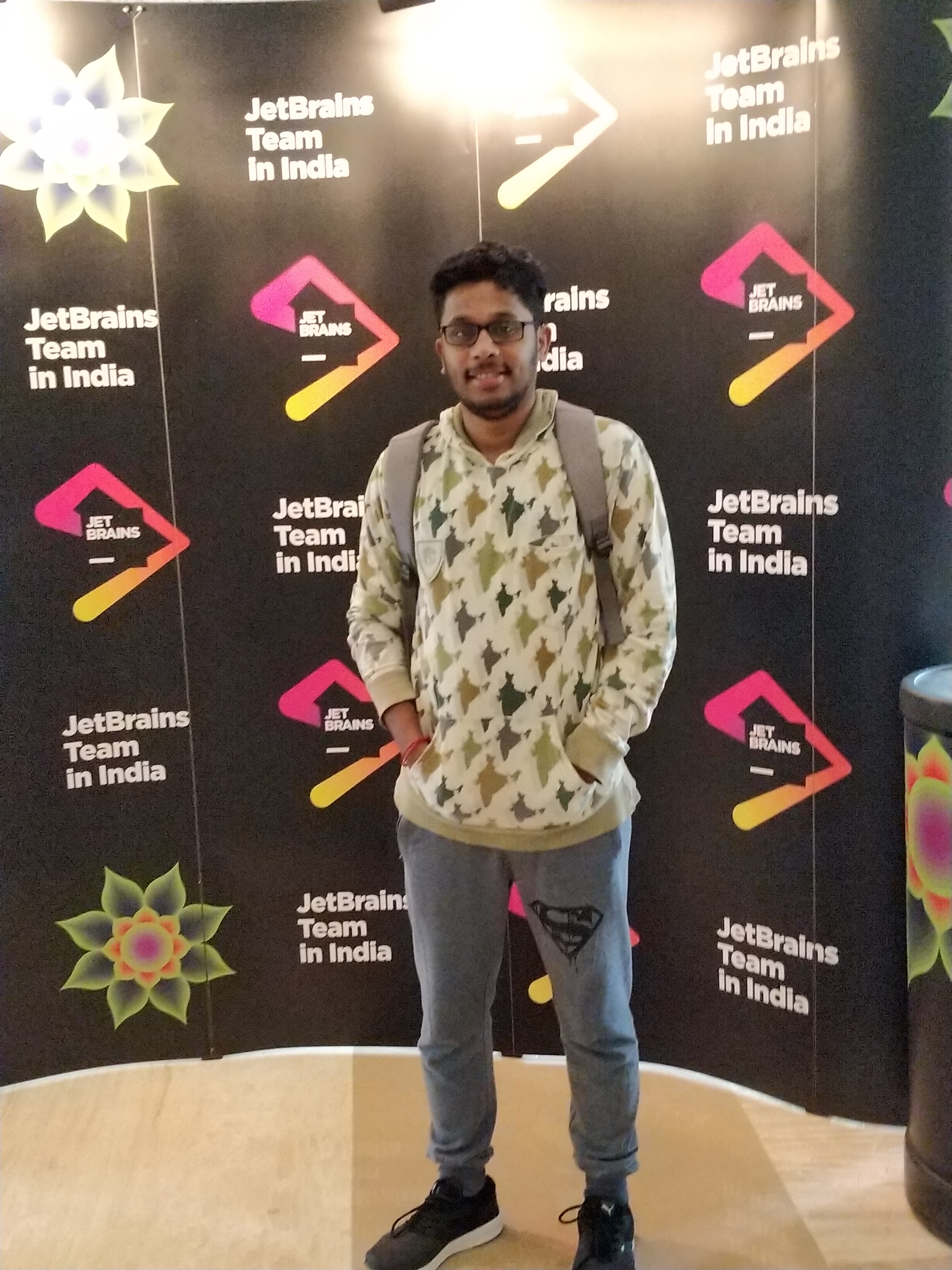
Vignesh Prabhu
Vignesh Prabhu
I am an Android application developer who is looking for new challenges to solve , love to learn and implement new things in coding