Server-side rendering in React JS
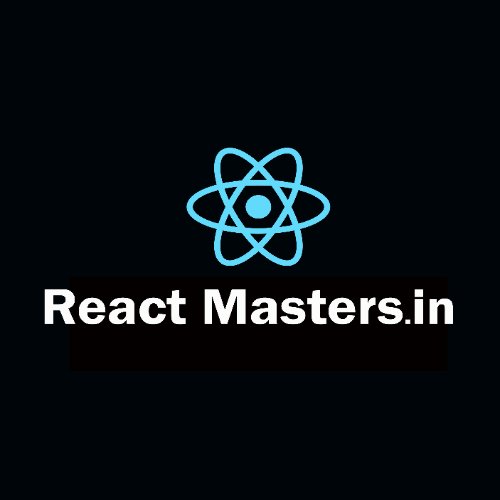
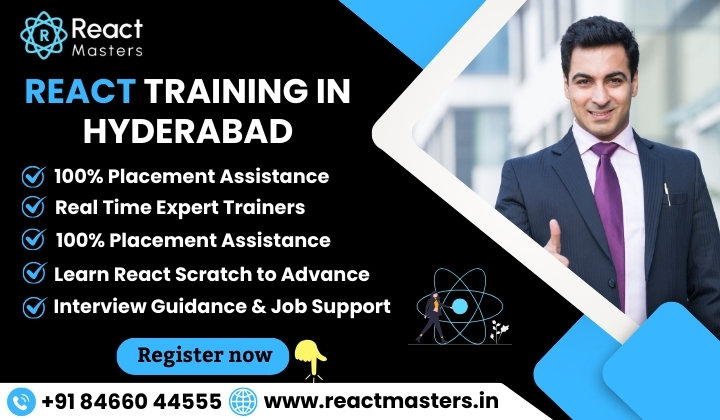
Introduction
In the world of modern web development, performance and user experience are paramount. As web applications become more dynamic and interactive, ensuring they load quickly and efficiently on a variety of devices is crucial. This is where Server-Side Rendering (SSR) comes into play, particularly in the context of React JS. SSR offers a way to improve the performance, SEO, and overall user experience of React applications. In this article, we'll delve into what SSR is, how it works in React, its benefits, and challenges, and how to implement it.
What is Server-Side Rendering (SSR)?
Server-side rendering is the process of rendering web pages on the server instead of the client. In a typical React application, rendering happens on the client side. The browser downloads a minimal HTML page, along with the necessary JavaScript files, and the React components are rendered in the browser. With SSR, the server pre-renders the React components into HTML before sending them to the client. The browser receives a fully rendered HTML page, which can be displayed immediately while the JavaScript files are downloaded and executed.
How Does SSR Work in React?
In React, SSR involves rendering the components on the server and sending the fully rendered HTML to the client. When the client receives this HTML, it displays the content immediately. Once the JavaScript files are loaded, React takes over the application, making it interactive. This process is known as hydration.
Here's a step-by-step overview of how SSR works in React:
Request to the Server:
- When a user requests a page, the server receives the request and starts processing it.
Rendering on the Server:
- The server renders the React components into HTML. This involves running the same React code that would normally run on the client but executing it on the server instead.
Sending HTML to the Client:
- The server sends the fully rendered HTML to the client. This HTML includes the content that was rendered by React components.
Hydration:
- Once the client receives the HTML, it displays it immediately. Meanwhile, the JavaScript files are downloaded, and React takes over the application, attaching event listeners and making the page interactive. This process is known as hydration because React "hydrates" the server-rendered HTML with interactive functionality.
Benefits of Server-Side Rendering in React
Improved Performance:
- SSR can significantly improve the perceived performance of a web application. Since the server sends a fully rendered HTML page to the client, users see the content faster, even on slow network connections. This leads to a better user experience, especially on mobile devices.
Better SEO:
- Search engines like Google can crawl and index server-rendered HTML more effectively than client-rendered content. With SSR, the content is available in the initial HTML response, improving the chances of better SEO rankings.
Faster Time to First Byte (TTFB):
- SSR reduces the time to the first byte, as the server sends a fully rendered HTML page instead of an empty shell that requires additional JavaScript to render content. This can lead to a faster initial load time.
Improved User Experience:
- By delivering content quickly, SSR reduces the time users spend waiting for the page to load, leading to a more seamless and enjoyable experience. This is especially important for users on slower networks or devices.
Predictable State:
- With SSR, the server controls the initial state of the application, making it easier to manage and debug. The server sends the pre-rendered content along with the initial state, ensuring consistency between the server and the client.
Challenges and Drawbacks of SSR
Increased Server Load:
- SSR shifts the rendering process from the client to the server, which can increase the load on the server. For high-traffic applications, this can lead to performance bottlenecks and require more powerful servers.
Complexity in Development:
- Implementing SSR adds complexity to the development process. Developers need to consider how their code will run on both the server and client, which can lead to challenges with state management, routing, and third-party libraries that rely on the browser environment.
Slower Time to Interaction (TTI):
- While SSR improves the initial load time, it can delay the time it takes for the page to become interactive. This is because the client needs to download and execute the JavaScript files before React can take over and make the page interactive.
Potential for Code Duplication:
- In some cases, SSR may lead to code duplication between the server and the client. Developers need to ensure that the same logic works correctly in both environments, which can be challenging.
Limited Access to Browser APIs:
- Since SSR runs on the server, it doesn't have access to browser-specific APIs like
window
,document
, orlocalStorage
. Developers need to write code that handles these differences, which can add complexity.
- Since SSR runs on the server, it doesn't have access to browser-specific APIs like
Implementing Server-Side Rendering in React
Implementing SSR in a React application typically involves setting up a server that can render React components into HTML and serve them to the client. Here's a basic overview of how to implement SSR in React:
Setting Up a Node.js Server:
- A Node.js server is commonly used to handle SSR in React. Tools like Express.js can be used to create a server that listens for requests and renders React components.
Rendering React Components on the Server:
- The
react-dom/server
the package provides methods likerenderToString()
andrenderToStaticMarkup()
to render React components into HTML on the server.
- The
Sending the Rendered HTML to the Client:
- Once the components are rendered, the server sends the HTML to the client along with the necessary JavaScript files to handle hydration.
Hydration on the Client:
- On the client side, React hydrates the server-rendered HTML, attaching event listeners and making the page interactive.
Handling Routing and State Management:
- SSR requires careful consideration of routing and state management. Libraries like React Router and Redux can be configured to work with SSR, ensuring that the initial state and routes are consistent between the server and client.
SSR with Next.js
One of the easiest ways to implement SSR in a React application is by using Next.js, a popular React framework that provides built-in support for SSR. Next.js handles many of the complexities of SSR, including routing, code splitting, and data fetching, making it an excellent choice for developers looking to leverage SSR in their React applications.
Conclusion
Server-side rendering in React JS is a powerful technique that can significantly improve the performance, SEO, and user experience of web applications. By rendering content on the server and sending fully rendered HTML to the client, SSR ensures faster load times and better visibility in search engines. However, SSR also comes with its own set of challenges, including increased server load, complexity in development, and potential delays in interactivity. Developers need to carefully consider these factors when deciding whether to implement SSR in their React applications. Tools like Next.js can simplify the process, making it easier to reap the benefits of SSR while managing its complexities.
Hope you got full information about Server-side rendering in React. For More details about the course of free demo sessions contact Mob:+91 84660 44555
Subscribe to my newsletter
Read articles from React Masters directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
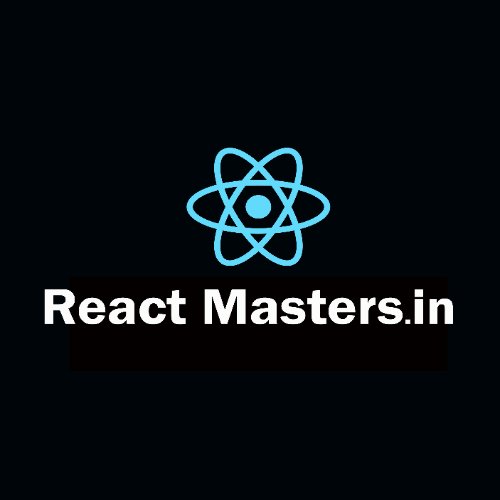
React Masters
React Masters
React Masters is one of the leading React JS training providers in Hyderabad. We strive to change the conventional training modes by bringing in a modern and forward program where our students get advanced training. We aim to empower the students with the knowledge of React JS and help them build their career in React JS development. React Masters is a professional React training institute that teaches the fundamentals of React in a way that can be applied to any web project. Industry experts with vast and varied knowledge in the industry give our React Js training. We have experienced React developers who will guide you through each aspect of React JS. You will be able to build real-time applications using React JS.