The Essential Guide to HTML and CSS: Building Blocks of Web Development

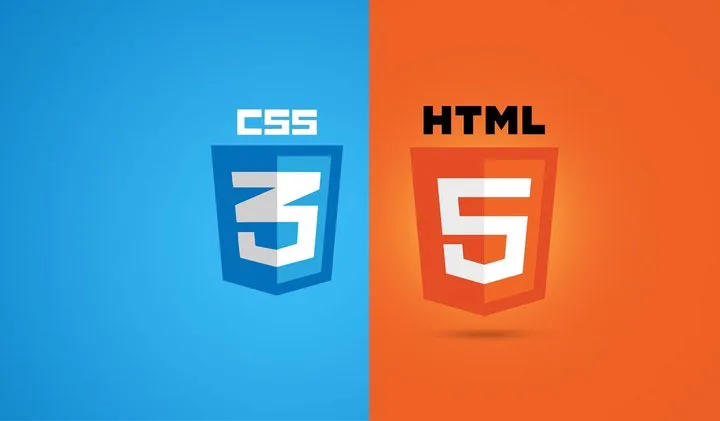
Introduction
In web development, HTML (Hypertext Markup Language) and CSS (Cascading Style Sheets) are the fundamental technologies that form the backbone of every website. Whether you’re a beginner stepping into coding or a seasoned developer looking to brush up on your skills, understanding HTML and CSS is crucial. In this blog, we'll dive into the essentials of these technologies, exploring their key concepts, and how they work together to create stunning websites.
1. What is HTML?
HTML is the standard markup language used to create the structure of web pages. It defines the content and layout of a webpage by using a series of elements and tags. Each element represents a different part of the webpage, such as headings, paragraphs, links, images, and more.
Key HTML Concepts:
Tags and Elements: HTML uses tags to define elements. Tags are usually enclosed in angle brackets, like
<p>
for a paragraph or<h1>
for a heading. Most elements have opening and closing tags, like<p>content</p>
.<h1>This is a Heading</h1> <p>This is a paragraph.</p> <a href="https://example.com">This is a link</a>
Attributes: Attributes provide additional information about elements. They are always included in the opening tag and usually appear in key-value pairs, like
class="classname"
orhref="URL"
.<img src="image.jpg" alt="Description of image"> <a href="https://example.com" target="_blank">Visit Example</a>
Document Structure: An HTML document has a specific structure, starting with the
<!DOCTYPE html>
declaration, followed by<html>
,<head>
, and<body>
tags. It<head>
contains metadata and<body>
includes visible content.<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>My First Webpage</title> </head> <body> <h1>Welcome to My Website</h1> <p>This is a simple HTML document.</p> </body> </html>
<meta>
Tag: The<meta>
tag provides metadata about the HTML document, which is data about data. This metadata is typically used by browsers and search engines.charset
Attribute: Thecharset
attribute defines the character encoding, which is the set of characters and symbols that can be displayed in the document.UTF-8
: UTF-8 (Unicode Transformation Format - 8-bit) is a widely used character encoding that can represent almost all characters from all written languages. It includes ASCII (which is limited to English characters) and supports characters from many languages like Arabic, Chinese, and symbols like emojis.Forms: Forms are crucial for user interaction, allowing data submission through input fields, buttons, and other controls. Elements like
<input>
,<textarea>
, and<button>
are key components of forms.<form action="/submit" method="post"> <label for="name">Name:</label> <input type="text" id="name" name="name"> <button type="submit">Submit</button> </form>
Common Header Meta Tags: Meta tags in the
<head>
section are crucial for setting up the page's behavior and ensuring compatibility across different devices and search engines.<meta name="description" content="A brief description of the webpage"> <meta name="keywords" content="HTML, CSS, JavaScript"> <meta name="author" content="Your Name"> <meta name="viewport" content="width=device-width, initial-scale=1.0">
2. What is CSS?
CSS is the language used to style HTML documents. It allows developers to apply design elements like colors, fonts, layouts, and spacing to the HTML structure, making the webpage visually appealing and user-friendly.
Key CSS Concepts:
Selectors: Selectors are used to target HTML elements that you want to style. They can be simple (like targeting an element by tag, class, or ID) or complex (like combining selectors).
h1 { color: blue; font-size: 24px; } .container { width: 80%; margin: 0 auto; } #header { background-color: #f4f4f4; padding: 10px; }
Box Model: The box model is a fundamental concept in CSS that defines how elements are displayed on a webpage. It consists of margins, borders, padding, and the content itself.
.box { width: 300px; padding: 20px; border: 5px solid black; margin: 10px; }
Inline vs. Block Elements: Inline elements (e.g.,
<span>
,<a>
) only take up as much width as necessary, while block elements (e.g.,<div>
,<p>
) take up the full width of their container.<span>This is an inline element</span> <div>This is a block element</div>
Positioning: Relative/Absolute: Positioning controls how elements are placed on the page.
relative
positioning adjusts the element's position relative to its normal location, whileabsolute
positioning places the element based on its nearest positioned ancestor..relative-box { position: relative; top: 10px; left: 20px; } .absolute-box { position: absolute; top: 50px; left: 100px; }
Common CSS Structural Classes: Structural classes like
.container
,.row
, and.col
are used to create layouts and organize content on the page..container { width: 80%; margin: 0 auto; } .row { display: flex; flex-wrap: wrap; } .col { flex: 1; padding: 10px; }
Common CSS Styling Classes: Styling classes like
.btn
,.text-center
, and.hidden
are used to apply consistent styles across elements..btn { padding: 10px 20px; background-color: blue; color: white; border-radius: 5px; } .text-center { text-align: center; } .hidden { display: none; }
CSS Specificity: Specificity determines which CSS rule applies when multiple rules target the same element. It's calculated based on the type of selectors used.
/* ID selector has higher specificity */ #unique-element { color: red; } /* Class selector has lower specificity */ .common-class { color: blue; }
CSS Responsive Queries: Media queries are used to apply different styles based on the screen size or device type, ensuring your website looks great on all devices.
@media (max-width: 600px) { .container { width: 100%; } }
Flexbox and Grid: Flexbox and CSS Grid are powerful layout models in CSS. Flexbox is great for one-dimensional layouts, while Grid excels in creating two-dimensional layouts with rows and columns.
.flex-container { display: flex; justify-content: space-between; } .grid-container { display: grid; grid-template-columns: 1fr 1fr 1fr; gap: 10px; }
Pseudo-Classes and Pseudo-Elements: These allow you to style elements based on their state (like
:hover
for when a user hovers over an element) or specific parts of an element (like::before
and::after
for adding content before or after an element).a:hover { color: red; } p::before { content: "Note: "; font-weight: bold; }
3. How HTML and CSS Work Together
HTML and CSS work in tandem to create beautiful, functional websites. HTML provides the structure, while CSS applies the style, allowing you to separate content from presentation. This separation is crucial for maintaining and scaling web projects.
Inline, Internal, and External CSS:
Inline CSS: Applies styles directly within the HTML elements using the
style
attribute. This is not recommended for larger projects as it can clutter the HTML code.<h1 style="color: blue;">This is a Blue Heading</h1>
Internal CSS: Styles are written within the
<style>
tag inside the<head>
section of an HTML document. It's useful for styling a single page.<head> <style> body { background-color: lightblue; } </style> </head>
External CSS: The most efficient method, external CSS links a separate
.css
file to the HTML document. This allows for consistent styling across multiple pages and easier maintenance.<head> <link rel="stylesheet" href="styles.css"> </head>
4. Best Practices for HTML and CSS
To ensure your websites are both user-friendly and easy to maintain, follow these best practices:
Use Semantic HTML: Semantic elements like
<header>
,<footer>
,<article>
, and<section>
provide meaning to your content, improving accessibility and SEO.<header> <h1>Website Title</h1> <nav> <ul> <li><a href="#">Home</a></li> <li><a href="#">About</a></li> <li><a href="#">Contact</a></li> </ul> </nav> </header>
Keep CSS Organized: Use a consistent naming convention for classes and IDs, and consider using methodologies like BEM (Block, Element, Modifier) to keep your stylesheets organized.
.block__element--modifier { /* Styles */ }
Optimize for Performance: Minimize and compress CSS files, avoid excessive use of inline styles, and load CSS files asynchronously to improve page load times.
<link rel="stylesheet" href="styles.min.css" async>
Test Responsiveness: Always test your design on various devices and screen sizes. Use tools like browser developer tools to simulate different screen resolutions.
@media (min-width: 768px) { .sidebar { display: block; } }
Conclusion
HTML and CSS are the building blocks of web development. Mastering these technologies allows you to create well-structured, visually appealing, and responsive websites. Whether you’re building a simple blog or a complex web application, a strong foundation in HTML and CSS will set you on the path to success. Keep practicing, experiment with new techniques, and stay updated with the latest trends in web design.
Subscribe to my newsletter
Read articles from Ravi Gupta directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
