Simplifying API Client Development with Automated Pagination Links in REST APIs
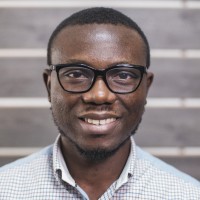
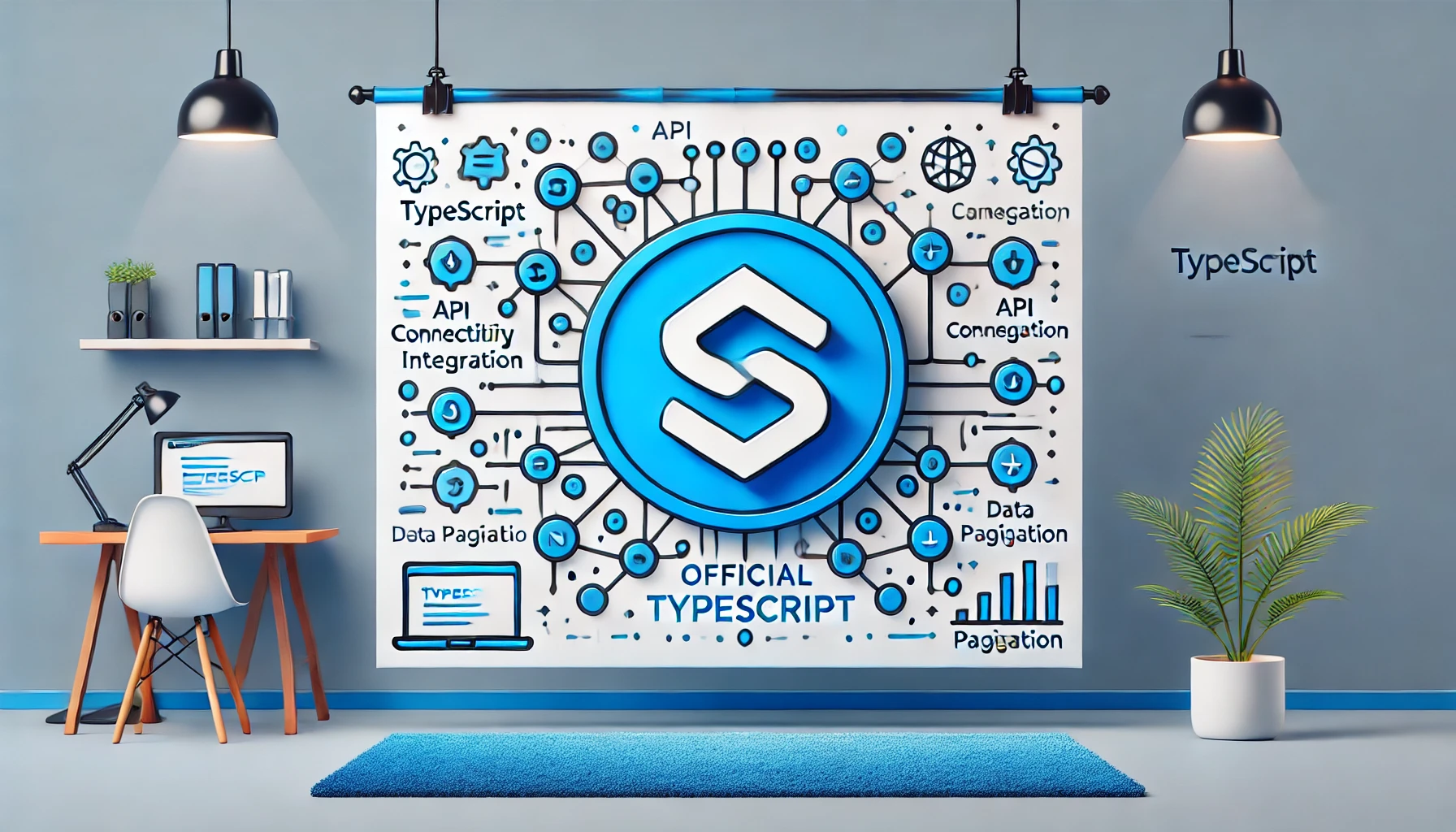
In today’s data-driven and AI world, REST APIs that return paginated data are a common necessity, especially when dealing with large datasets that can't reasonably fit into a single HTTP response body. However, there’s an often-overlooked aspect of API development that can significantly improve APIs and their developer experience (DX): generating navigation links (like next and previous relative links) for paginated responses directly within the API.
In this article, we'll explore why this practice is important and how you can implement it in your API. By offloading this task to the backend, you can make your API more ergonomic and user-friendly, sparing your clients from unnecessary work.
Why You Should Generate Pagination Links for Your API Clients
Let's start with the "Why". Here are two compelling reasons to handle pagination links for your API clients :
Eliminate Unnecessary Work for Clients:
When you provide pagination links in your API responses, you save your clients from having to compute these links themselves. This is particularly important in large or distributed systems where consistency and efficiency are key. By constructing these links on the server side, you ensure that all clients have a consistent, reliable way to navigate through pages of datasets.Influence How Your API is Used:
By controlling pagination behavior through your API, you can influence how clients interact with your data. Whether it’s by adjusting how pages are navigated or by controlling the format of pagination links, you can ensure that your API is used in the most efficient and intended manner. Additionally, if you offer an SDK, this approach allows you to offload some of the computational work to the client side, thereby optimizing server resources.
How to Implement Pagination Links in Your Backend API
Now that we've covered the importance of this feature, let’s dive into the implementation. The key is to augment your API controllers so that they return more than just the actual paged data—they should also return URLs to the next and previous pages of the data.
We will look at an example of searching for flights in a Nodejs backend application built with Express and TypeScript, but the principles should generally apply in a language agnostic way.
Step 1: Refactor Your API Controllers
The first step is to modify your API controllers to construct and include pagination links in the response. Rather than simply returning a list of data, you’ll augment the response with URLs pointing to the next and previous pages relative to the current page the user has requested for.
Step 2: Implement Your buildPaginationLinks(...)
The core of this functionality lies in a variation of this buildPaginationLinks(...)
function which the API controller will delegate to. Here’s how it works:
Extract Query Parameters: Start by extracting query parameters from the incoming HTTP request, using sensible defaults where appropriate. The value of the
page
parameter will help determine whether a previous or next link should be generated.Conditionally Set The Previous and Next Links: If the current page is greater than 1, generate a previous link. Conversely, if there are more pages of matching data to be retrieved (e.g
hasMore
isTRUE
), then generate a next link.
Step 3: Use a Query Param Setter Factory
To manage the construction of these links in a simple, robust, and portable way, our buildPaginationLinks(...)
function also delegates to another setQueryParams({...})
function. This function is provided by a getQueryParamSetter(...)
factory function, which captures the HTTP request argument and uses it to generate URLs, including the scheme and host.
This setup allows you to dynamically update the endpoint URL with the necessary query parameters (like the page number or sorting direction). The modified URL is then returned as the next or previous link, which is included in the response sent back to the API client.
Step 4: Offer Flexibility with a pagenav
Query Parameter
To give clients control over where pagination links are placed, introduce a pagenav
(or similar) query parameter. This parameter is an enum and can have values like head
or body
, with a default of body
if that makes sense to your API.
Based on its value, you can set the pagination links either in the response headers (if pagenav=head
) or within the response body (if pagenav=body
).
Caveat: The Responsibility of Maintaining API Contract
While adding pagination links directly in your API responses can greatly enhance the usability and developer experience, it’s crucial to remember that any structure or data you expose to clients becomes a formal part of your API contract. Once these pagination links are included in your responses, clients will likely depend on them, which makes them difficult to change or remove without potentially breaking existing integrations.
This is why it’s important to carefully consider the structure and format of these links before implementing them. Make sure the URLs are future-proof and that they follow consistent patterns that can be maintained as your API evolves. If changes to the pagination structure are necessary in the future, consider versioning your API or providing adequate deprecation notices and migration paths to ensure a smooth transition for your clients. Always approach API design with the mindset that whatever you send to clients may need to be supported indefinitely.
Conclusion
Generating pagination links directly in your API responses is a simple yet powerful way to enhance the usability and DX of your APIs. By taking this responsibility off the client’s shoulders, you make your API more ergonomic and ensure a more consistent and reliable pagination experience.
If you ship an SDK, consider building this functionality into the client side to further optimize server resources. Over time, these enhancements can lead to a smoother, more efficient development process for everyone involved.
Subscribe to my newsletter
Read articles from Charles Opute Odili directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
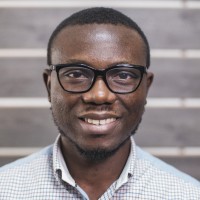
Charles Opute Odili
Charles Opute Odili
I am a Senior Software Engineer, Engineering Manager, and Mentor. I love building experiences that empower people at scale, helping businesses drive more value in measurable ways.