Day 15: Error Handling in Shell Scripting - A Comprehensive Guide

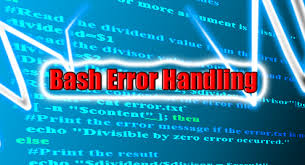
Why is Error Handling Important in Scripts? π€
Error handling is crucial because it ensures that your scripts behave predictably, even when things go wrong. Without error handling, a script might fail silently, leaving you unaware of the issue until it's too late leading to a inconsistent state. Proper error handling:
Prevents System Failures: It helps to avoid cascading failures that could bring down entire systems or services and thus lead to outages.
Improves Debugging: It provides clear information about what went wrong and where, making it easier to troubleshoot.
Enhances User Experience: It allows scripts to fail gracefully, providing meaningful feedback to users instead of cryptic error messages.
Increases Script Reliability: By handling errors appropriately, you make your scripts more robust and dependable.
Topics to Cover
Understanding Exit Status: Every command returns an exit status (0 for success and non-zero for failure). Learn how to check and use exit statuses.
Using if Statements for Error Checking: Learn how to use if statements to handle errors.
Using trap for Cleanup: Understand how to use the trap command to handle unexpected errors and perform cleanup.
Redirecting Errors: Learn how to redirect errors to a file or /dev/null.
Creating Custom Error Messages: Understand how to create meaningful error messages for debugging and user information.
Understanding Exit Status π
Every command executed in a shell script returns an exit status. This status is a numerical code that indicates whether the command was successful or not:
0: Success
Non-zero: Failure (The specific non-zero value often indicates the type of error)
You can check the exit status of the last executed command using the special variable $?
. Here's an example:
#!/bin/bash
mkdir /devops_practice/tanmayaday15
if [ $? -eq 0 ]; then #Here $? tells if mkdir ran successfully or not
echo "Directory created successfully."
else
echo "Failed to create directory."
fi
In this script, the mkdir
command attempts to create a directory. The if
statement checks the exit status of the mkdir
command. If it's 0
, the script confirms success; otherwise, it reports failure.
Using if
Statements for Error Checking β
if
statements are essential for checking the success or failure of a condition given in your script. They allow you to execute different blocks of code depending on whether a command succeeds or fails. Here's a simple example:
#!/bin/bash
cp /devops_practice/tanmayaday15 /90daysofdevops/
if [ $? -ne 0 ]; then
echo "Error: Failed to copy file."
exit 1
fi
In this example, if the cp
command fails (i.e., returns a non-zero exit status), the script outputs an error message and exits with a status of 1
, indicating that something went wrong.
Using trap
for Cleanup π§Ή
The trap
command is a powerful feature in shell scripting that allows you to specify cleanup actions when your script exits unexpectedly. This is particularly useful for releasing resources, deleting temporary files, or rolling back changes.
Hereβs how to use trap
:
#!/bin/bash
tempfile=$(mktemp)
trap "rm -f $tempfile" EXIT
# Simulate a command that might fail
cp /some/nonexistent/file $tempfile
if [ $? -ne 0 ]; then
echo "Error: Failed to copy file."
exit 1
fi
# Further processing...
# The tempfile will be automatically removed when the script exits
In this script, the trap
command ensures that the temporary file created by mktemp
is deleted when the script exits, regardless of whether it exits normally or due to an error.
Redirecting Errors π
In shell scripting, you can redirect error messages to a file or discard them entirely by redirecting them to /dev/null
. This can be useful for logging errors or keeping your scriptβs output clean.
Here's how to do it:
#!/bin/bash
command 2> error.log
# Or to ignore errors:
command 2> /dev/null
In the above example, 2>
redirects the standard error (stderr) to a file named error.log
. If you prefer to ignore errors, you can redirect them to /dev/null
, which is a special file that discards all data written to it.
Creating Custom Error Messages π οΈ
Custom error messages can significantly aid in debugging and provide clearer feedback to users. Instead of relying on default error messages, you can craft your own:
#!/bin/bash
file="/path/to/some/file"
if [ ! -f "$file" ]; then
echo "Error: $file does not exist."
exit 1
fi
# Further processing...
In this example, the script checks if a specific file exists. If not, it outputs a custom error message and exits with a non-zero status. This approach ensures that users and developers have a clear understanding of what went wrong.
Conclusion π―
Error handling is a critical aspect of shell scripting that ensures your scripts are robust, reliable, and user-friendly. By understanding exit statuses, using if
statements, employing trap
for cleanup, redirecting errors, and crafting custom error messages, you can significantly improve the quality and maintainability of your scripts.
Remember, a well-handled error is not just a problem avoidedβit's an opportunity to make your script smarter and more resilient. Happy scripting! β¨
Subscribe to my newsletter
Read articles from Tanmaya Arora directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Tanmaya Arora
Tanmaya Arora
Hey there! π π Welcome to my DevOps journey! π I'm Tanmaya Arora, an enthusiastic DevOps Engineer. Currently, on a learning adventure, I'm here to share my journey and Blogs about DevOps. I believe in fostering a culture of resilience, transparency, and shared responsibility. Embracing agility and flexibility, in this adventure let's grow together in this vibrant DevOps space. Join me in transforming software delivery through collaboration, innovation, and excellence! ππ§π‘ π Connect with me for friendly chats, group discussions, shared experiences and learning moments.