Comprehensive Guide to Apache Dubbo - Building a Provider-Consumer Demo
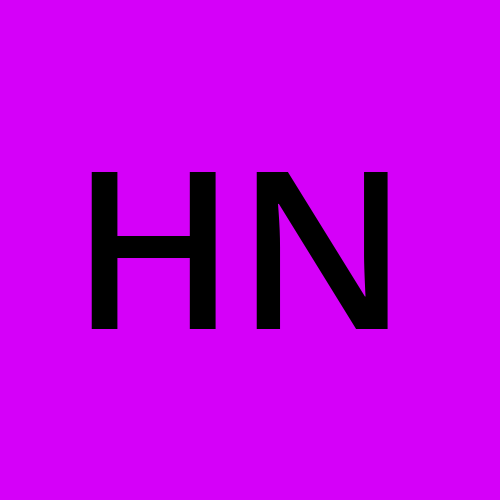
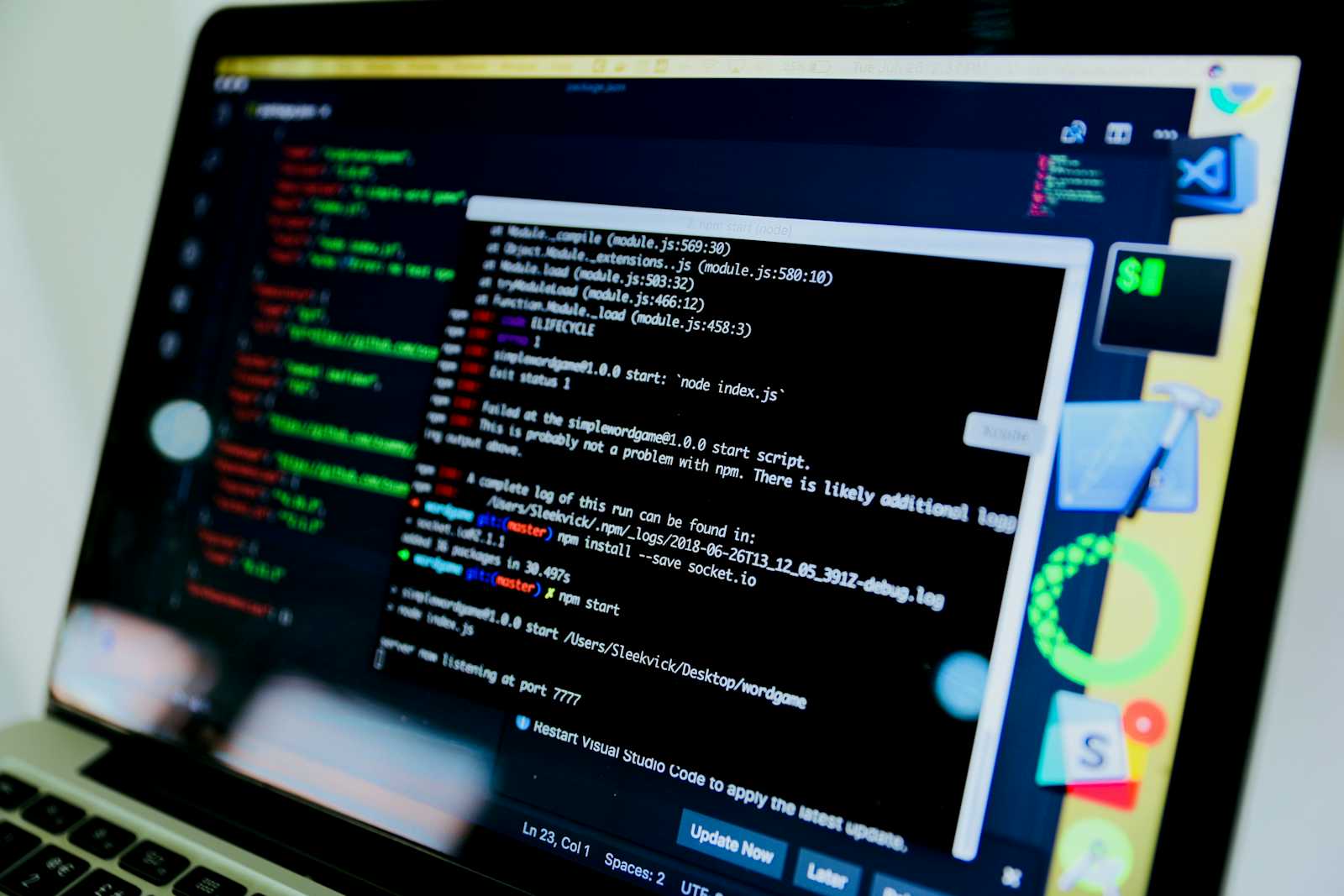
This guide will take you through the step-by-step process of creating a simple Apache Dubbo demo with a provider and a consumer. It is designed for beginners, providing detailed explanations for each step. By the end of this guide, you should have a clear understanding of how to set up, configure, and run a basic Dubbo application.
Prerequisites:
Java Development Kit (JDK): Ensure that you have Java installed on your machine. Dubbo is a Java-based framework, and you need the JDK to compile and run Java applications. You can download it here.
Maven: Maven is a powerful build tool for Java projects. You can download and install Maven from here.
Step 1: Create a Dubbo Provider Service
Open a terminal and navigate to the directory where you want to create the provider project. Run the following command:
mvn archetype:generate -DgroupId=com.example -DartifactId=dubbo-demo-provider -DarchetypeArtifactId=maven-archetype-quickstart -DinteractiveMode=false
This command creates a new Maven project with the specified group and artifact IDs.
1.2 Add Dubbo Dependency
Navigate into the newly created dubbo-demo-provider
directory. Open the pom.xml
file with your preferred text editor. Add the Dubbo dependency under the <dependencies>
section:
<dependencies>
<dependency>
<groupId>org.apache.dubbo</groupId>
<artifactId>dubbo</artifactId>
<version>2.7.14</version> <!-- Use the latest version available -->
</dependency>
</dependencies>
1.3 Create a Service Interface
Inside the src/main/java/com/example
directory, create an interface for the service you want to expose. For example, create DemoService.java
:
package com.example;
public interface DemoService {
String sayHello(String name);
}
1.4 Implement the Service
Create a class that implements the DemoService
interface. For example, create DemoServiceImpl.java
:
package com.example;
public class DemoServiceImpl implements DemoService {
@Override
public String sayHello(String name) {
return "Hello, " + name + "!";
}
}
1.5 Configure Dubbo
Create a configuration file named dubbo.xml
inside the src/main/resources
directory with the following content:
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://www.springframework.org/schema/beans
http://www.springframework.org/schema/beans/spring-beans.xsd">
<!-- Define the service -->
<dubbo:service interface="com.example.DemoService" ref="demoService" />
<!-- Reference to the service implementation -->
<bean id="demoService" class="com.example.DemoServiceImpl" />
</beans>
Step 2: Create a Dubbo Consumer
2.1 Create a New Maven Project for Consumer
Open a new terminal window and navigate to the directory where you want to create the consumer project. Run the following command:
mvn archetype:generate -DgroupId=com.example -DartifactId=dubbo-demo-consumer -DarchetypeArtifactId=maven-archetype-quickstart -DinteractiveMode=false
Navigate into the newly created dubbo-demo-consumer
directory.
2.2 Add Dubbo Dependency
Open the pom.xml file in the dubbo-demo-consumer directory. Add the Dubbo dependency under the <dependencies> section:
<dependencies>
<dependency>
<groupId>org.apache.dubbo</groupId>
<artifactId>dubbo</artifactId>
<version>2.7.14</version> <!-- Use the latest version available -->
</dependency>
</dependencies>
2.3 Configure Dubbo
Create a configuration file named dubbo.xml
inside the src/main/resources
directory with the following content:
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://www.springframework.org/schema/beans
http://www.springframework.org/schema/beans/spring-beans.xsd">
<!-- Reference to the remote service -->
<dubbo:reference id="demoService" interface="com.example.DemoService" />
</beans>
2.4 Invoke the Service
Create a class to invoke the service. For example, create ConsumerApplication.java
inside the src/main/java/com/example
directory:
package com.example;
import org.springframework.context.support.ClassPathXmlApplicationContext;
public class ConsumerApplication {
public static void main(String[] args) {
ClassPathXmlApplicationContext context = new ClassPathXmlApplicationContext("dubbo.xml");
context.start();
DemoService demoService = (DemoService) context.getBean("demoService");
String result = demoService.sayHello("Dubbo");
System.out.println("Result: " + result);
context.close();
}
}
Step 3: Run the Demo
3.1 Build the Projects
In each project directory (dubbo-demo-provider
and dubbo-demo-consumer
), run:
mvn clean install
3.2 Run the Provider
Navigate to the dubbo-demo-provider
directory and run:
java -jar target/dubbo-demo-provider-1.0-SNAPSHOT.jar
3.3 Run the Consumer
Navigate to the dubbo-demo-consumer
directory and run:
java -jar target/dubbo-demo-consumer-1.0-SNAPSHOT.jar
You should see the output: "Result: Hello, Dubbo!"
Congratulations! You've successfully created and run a simple Apache Dubbo demo. This guide provides a detailed explanation of each step, ensuring a comprehensive understanding. For more advanced configurations and features, refer to the official Dubbo Documentation.
Subscribe to my newsletter
Read articles from Harshit Nagpal directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
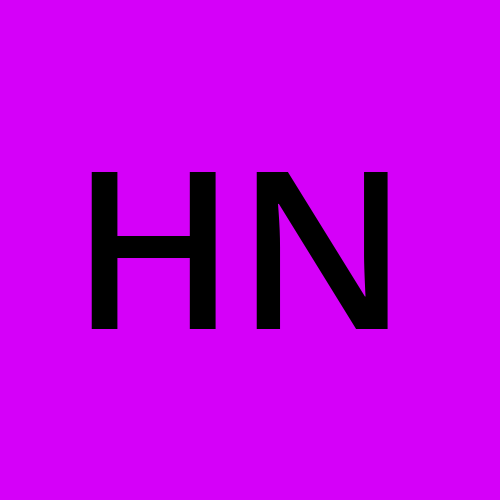