Pandas Series and DataFrames: A Comprehensive Guide

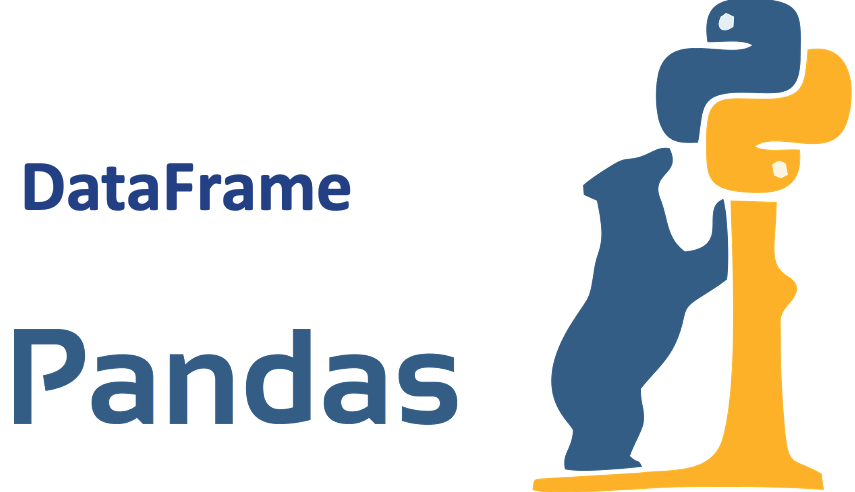
Introduction
Pandas is a powerful Python library for data manipulation and analysis. Two fundamental data structures in Pandas are Series and DataFrames. Mastering these data structures is essential for effective data analysis. In this blog, we will delve into Series and DataFrames, covering their creation, features, and key operations.
Pandas Series
A Pandas Series is a one-dimensional labeled array of values. It's similar to a list or a NumPy array, but with additional features:
Indexing: Series has an index, which is a set of labels for each value.
Data Type: Series can hold any data type, including integers, floats, strings, and more.
Creating a Series
You can create a Series from a list, array, or dictionary.
import pandas as pd
s = pd.Series([1, 2, 3, 4, 5], index=['a', 'b', 'c', 'd', 'e'])
Series Features
Indexing and Selecting: Select specific values using indexing or label-based selection.
Data Type: Series can hold different data types.
Missing Data: Series can handle missing data using NaN (Not a Number) values.
Pandas DataFrames
A Pandas DataFrame is a two-dimensional labeled data structure with columns of potentially different types. Think of it as a spreadsheet or a table:
Indexing: DataFrame has an index (rows) and columns.
Data Type: DataFrame can hold different data types in each column.
Creating a DataFrame
You can create a DataFrame from a dictionary, list of dictionaries, or other data structures.
data = {'Name': ['John', 'Anna', 'Peter'], 'Age': [28, 24, 35]}
df = pd.DataFrame(data, index=['a', 'b', 'c'])
DataFrame Features
Indexing and Selecting: Select specific rows or columns using indexing or label-based selection.
Data Type: DataFrame can hold different data types in each column.
Missing Data: DataFrame can handle missing data using NaN values.
Key Operations
Selection: Select specific rows or columns using indexing or conditional statements.
Filtering: Filter data using conditional statements.
Grouping: Group data by one or more columns and perform aggregation operations.
Merging: Merge two or more DataFrames based on a common column.
Conclusion
Pandas Series and DataFrames are powerful data structures for data analysis. Understanding their features and operations is essential for working with data in Python. Practice and explore more to become proficient in using these data structures.
Additional Tips and Tricks
Use
pd.set_option
to customize display options.Use
df.head()
anddf.tail()
to view the first and last few rows.Use
df.info
()
anddf.describe()
to view summary statistics
Stay tuned for the next part of this series, where we will explore more advanced topics in Machine Learning. If you have any questions or feedback, feel free to leave a comment below.
Happy learning!
About the Author: Sreemathibala P is a final-year computer science student specializing in Artificial Intelligence and Machine Learning. Passionate about data science and coding, I share insights on various machine learning topics.
Follow Me: -
[X] (x.com/SreemathibalaP)
[GitHub](github.com/sreemathibalapalpandian).
Subscribe to my newsletter
Read articles from Sreemathibala Palpandian directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Sreemathibala Palpandian
Sreemathibala Palpandian
I am a Final year student doing my Bachelor's in Computer Science and Engineering in specialization with Artificial Intelligence and Machine Learning