Design Patterns Explained: Improve Your Software Crafting Skills

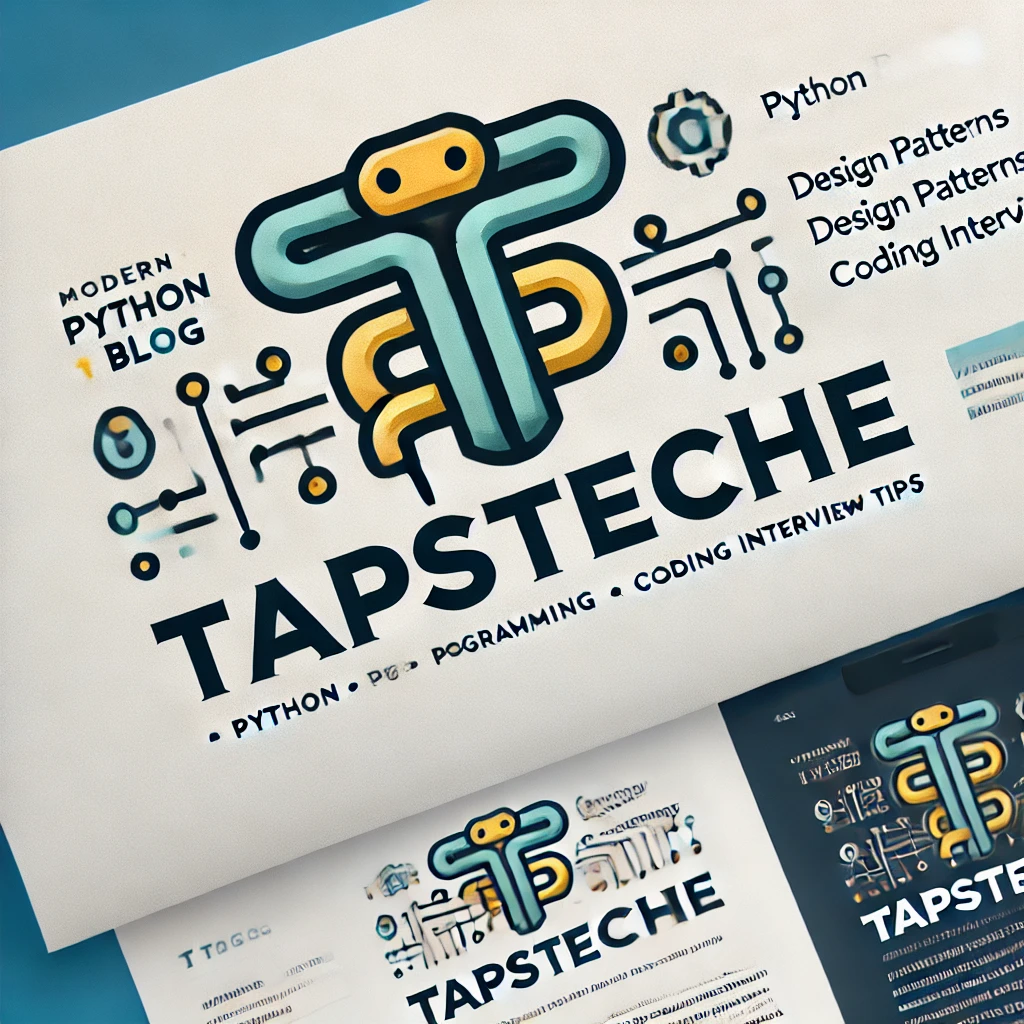
In the world of software development, the term "Design Patterns" holds a special place. Whether you're a seasoned programmer or just starting your journey, understanding design patterns can be a game-changer in how you approach problem-solving and software architecture. In this series, we will dive deep into the most essential design patterns, exploring their importance, application, and how they can help you become a more effective and efficient developer.
What are Design Patterns?
Design patterns are proven solutions to common problems that software developers face. They are like blueprints or templates that you can apply to solve specific design issues in your code. Think of them as best practices distilled into a repeatable, teachable format. Design patterns are not specific to any programming language; rather, they are concepts that can be implemented in any language, including Python, Java, C#, and more.
These patterns emerged from the collective experience of software developers who encountered and solved similar problems repeatedly. By understanding and using these patterns, you can leverage their experience to avoid pitfalls, write more maintainable code, and communicate your design decisions more clearly with other developers.
Why Should You Learn Design Patterns?
Improved Code Quality:
- Design patterns encourage best practices, leading to code that is more readable, maintainable, and scalable.
Better Communication:
- Design patterns provide a shared language for developers. When you say "I'm using a Singleton here," other developers instantly understand the pattern and its implications.
Efficiency in Problem-Solving:
- By recognizing problems that align with known patterns, you can quickly apply a solution that has been tested and proven, saving time and effort.
Avoiding Common Pitfalls:
- Many design patterns are crafted specifically to avoid common problems, such as code duplication, tight coupling, and other bad practices.
Types of Design Patterns
Design patterns are generally categorized into three types:
Creational Patterns:
- These patterns deal with object creation mechanisms, trying to create objects in a manner suitable to the situation. Creational patterns help make a system independent of how its objects are created, composed, and represented.
Structural Patterns:
- Structural patterns deal with object composition or the structure of classes. They help ensure that if one part of the system changes, the entire structure does not need to change as well.
Behavioral Patterns:
- Behavioral patterns focus on communication between objects. They help in coordinating how objects interact with each other.
Design Patterns We Will Cover in This Series
Over the next few articles, we will explore a variety of design patterns that fall under these categories. Here's a sneak peek at the patterns we'll be discussing:
Creational Patterns:
Singleton Pattern:
- Ensures a class has only one instance and provides a global point of access to it.
Factory Method Pattern:
- Provides an interface for creating objects but allows subclasses to alter the type of objects that will be created.
Abstract Factory Pattern:
- Provides an interface for creating families of related or dependent objects without specifying their concrete classes.
Builder Pattern:
- Separates the construction of a complex object from its representation, allowing the same construction process to create different representations.
Prototype Pattern:
- Creates new objects by copying an existing object, known as the prototype.
Structural Patterns:
Adapter Pattern:
- Allows incompatible interfaces to work together by wrapping an existing class with a new interface.
Bridge Pattern:
- Decouples an abstraction from its implementation so that the two can vary independently.
Composite Pattern:
- Composes objects into tree structures to represent part-whole hierarchies. It allows clients to treat individual objects and compositions of objects uniformly.
Decorator Pattern:
- Allows behavior to be added to individual objects, either statically or dynamically, without affecting the behavior of other objects from the same class.
Facade Pattern:
- Provides a simplified interface to a complex subsystem, making it easier to use.
Flyweight Pattern:
- Reduces the cost of creating and manipulating a large number of similar objects.
Proxy Pattern:
- Provides a surrogate or placeholder for another object to control access to it.
Behavioral Patterns:
Chain of Responsibility Pattern:
- Passes a request along a chain of handlers. Each handler can either process the request or pass it to the next handler in the chain.
Command Pattern:
- Encapsulates a request as an object, thereby allowing for parameterization of clients with queues, requests, and operations.
Interpreter Pattern:
- Implements a specialized language and interprets sentences in that language.
Iterator Pattern:
- Provides a way to access elements of a collection sequentially without exposing its underlying representation.
Mediator Pattern:
- Defines an object that encapsulates how a set of objects interact. This pattern promotes loose coupling by keeping objects from referring to each other explicitly.
Memento Pattern:
- Captures and externalizes an object’s internal state so that it can be restored later, without violating encapsulation.
Observer Pattern:
- Defines a one-to-many dependency between objects, so that when one object changes state, all its dependents are notified and updated automatically.
State Pattern:
- Allows an object to alter its behavior when its internal state changes. The object will appear to change its class.
Strategy Pattern:
- Defines a family of algorithms, encapsulates each one, and makes them interchangeable. Strategy lets the algorithm vary independently from clients that use it.
Template Method Pattern:
- Defines the skeleton of an algorithm in an operation, deferring some steps to subclasses.
- Visitor Pattern:
- Represents an operation to be performed on the elements of an object structure. Visitor lets you define a new operation without changing the classes of the elements on which it operates.
Conclusion
Design patterns are powerful tools in a developer’s toolkit. Understanding and applying them can significantly improve the quality of your code, making it more modular, flexible, and easy to maintain. Throughout this series, we will demystify these patterns with simple explanations and practical examples, ensuring that you not only understand the patterns but also know when and how to apply them in your projects.
Stay tuned as we embark on this journey to master design patterns, starting with our first deep dive into the Singleton Pattern in the next article. Let's build better software, one pattern at a time!
Subscribe to my newsletter
Read articles from Tarun Sharma directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Tarun Sharma
Tarun Sharma
Hi there! I’m Tarun, a Senior Software Engineer with a passion for technology and coding. With experience in Python, Java, and various backend development practices, I’ve spent years honing my skills and working on exciting projects. On this blog, you’ll find insights, tips, and tutorials on topics ranging from object-oriented programming to tech trends and interview prep. My goal is to share valuable knowledge and practical advice to help fellow developers grow and succeed. When I’m not coding, you can find me exploring new tech trends, working on personal projects, or enjoying a good cup of coffee. Thanks for stopping by, and I hope you find my content helpful!