How to Use If-Else Conditions in Python
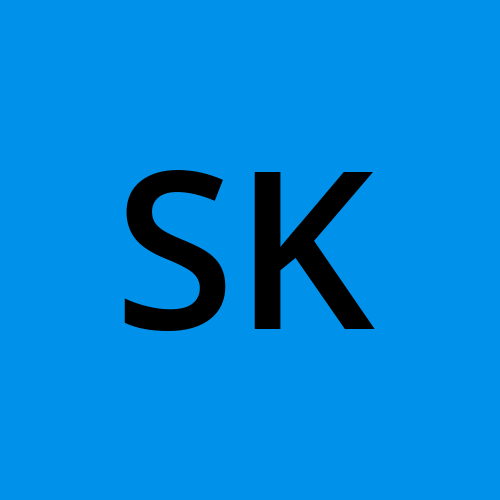
Python ek aisi programming language hai jo beginners ke liye kaafi friendly hai. Agar aap programming mein naye hain aur Python seekh rahe hain, toh aapko if-else statements ke baare mein zaroor pata hona chahiye. Is blog post mein, hum Python ke if-else statements ko bilkul simple tarike se samjhenge, taaki aap asaani se inko apne code mein use kar sakein.
Contents
If-Else Statement Kya Hota Hai?
If-Else Statement Ka Use Kyu Karein?
Basic Syntax: If-Else Statement Kaise Likhe?
If-Else Statement Ke Alag-Alag Types
If Statement
If-Else Statement
If-Elif-Else Statement
Nested If-Else Statement
If-Else Statement Mein Kaunse Operators Use Hote Hain?
Comparison Operators
Logical Operators
If-Else Statement Ke Simple Examples
Real-World Mein If-Else Statement Ka Use
Best Practices
Conclusion
1. If-Else Statement Kya Hota Hai?
If-Else statement Python mein decision lene ka ek tareeka hai. Matlab, aapko agar apne code mein kuch condition ke basis par decision lena hai, toh aap if-else ka use karte hain.
Example:
age = 18
if age >= 18:
print("Aap vote kar sakte hain.")
else:
print("Aap vote nahi kar sakte.")
Yahaan, hum check kar rahe hain ki age
18 ya usse zyada hai ya nahi. Agar age
18 ya usse zyada hai, toh "Aap vote kar sakte hain." print hoga, warna "Aap vote nahi kar sakte."
2. If-Else Statement Ka Use Kyu Karein?
If-else statements ka use aap tab karte hain jab:
Decision Making: Aapko kuch condition ke basis par alag-alag actions lene hote hain.
Input Validation: User se input lene ke baad usko check karna hota hai.
Program Control: Aap apne program ka flow control karna chahte hain.
For example, agar aapko check karna hai ki kisi student ke marks passing marks se zyada hain ya nahi, toh aap if-else statement use kar sakte hain.
3. Basic Syntax: If-Else Statement Kaise Likhe?
If-Else statement likhne ka tareeka bilkul simple hai. Aap pehle if
keyword use karte hain, fir condition likhte hain, aur uske baad else
block likhte hain jo tab execute hota hai jab condition false ho.
Syntax:
if condition:
# Yeh code tab chalega jab condition true hogi
statement(s)
else:
# Yeh code tab chalega jab condition false hogi
statement(s)
Example:
temperature = 20
if temperature > 25:
print("Bahut garmi hai.")
else:
print("Mausam thik hai.")
Is example mein, agar temperature 25 se zyada hota, toh "Bahut garmi hai." print hota. Agar temperature 25 ya usse kam hota, toh "Mausam thik hai." print hota.
4. If-Else Statement Ke Alag-Alag Types
If Statement
If statement ka use tab kiya jaata hai jab aapko sirf ek condition check karni ho.
Example:
number = 10
if number > 0:
print("Number positive hai.")
If-Else Statement
Agar condition true hai toh ek block chalega, aur agar false hai toh doosra block.
Example:
number = -5
if number > 0:
print("Number positive hai.")
else:
print("Number negative hai.")
If-Elif-Else Statement
Jab aapko multiple conditions check karni hoti hain, toh "elif" ka use karte hain.
Example:
marks = 85
if marks >= 90:
print("Aapko grade A mila hai.")
elif marks >= 75:
print("Aapko grade B mila hai.")
else:
print("Aapko grade C mila hai.")
Nested If-Else Statement
Ek if-else block ke andar doosra if-else block likha ja sakta hai.
Example:
number = 15
if number > 0:
if number % 2 == 0:
print("Number positive aur even hai.")
else:
print("Number positive aur odd hai.")
else:
print("Number negative hai.")
5. If-Else Statement Mein Kaunse Operators Use Hote Hain?
If-Else statements ke saath commonly kuch operators use hote hain, jinke zariye aap conditions define karte hain.
Comparison Operators
Yeh operators do values ko compare karte hain.
==
(Equal to):a == b
!=
(Not equal to):a != b
>
(Greater than):a > b
<
(Less than):a < b
>=
(Greater than or equal to):a >= b
<=
(Less than or equal to):a <= b
Example:
age = 18
if age >= 18:
print("Aap vote kar sakte hain.")
else:
print("Aap vote nahi kar sakte.")
Logical Operators
Yeh operators multiple conditions ko combine karne ke liye use hote hain.
and
: Dono conditions true honi chahiye.or
: Koi ek condition true honi chahiye.not
: Condition ko opposite kar deta hai.
Example:
age = 20
is_registered = True
if age >= 18 and is_registered:
print("Aap vote kar sakte hain.")
else:
print("Aap vote nahi kar sakte.")
6. If-Else Statement Ke Simple Examples
Aapke liye kuch aur simple examples yaha diye gaye hain taaki aap better samajh sakein.
Simple Example
num = int(input("Koi number daaliye: "))
if num % 2 == 0:
print(f"{num} ek even number hai.")
else:
print(f"{num} ek odd number hai.")
Example with Logical Operators
age = int(input("Apni age daaliye: "))
has_voter_id = input("Kya aapke paas voter ID hai? (yes/no): ").lower()
if age >= 18 and has_voter_id == 'yes':
print("Aap vote kar sakte hain.")
else:
print("Aap vote nahi kar sakte.")
Example with Nested If-Else
marks = int(input("Apne marks daaliye: "))
if marks >= 40:
if marks >= 75:
print("Aapko distinction mila hai.")
else:
print("Aap pass ho gaye hain.")
else:
print("Aap fail ho gaye hain.")
7. Real-World Mein If-Else Statement Ka Use
If-Else statements ka real-world mein bahut hi zyada use hota hai. Yeh kuch examples hain:
Form Validation: Agar aapko user se input lena hai aur usko check karna hai, toh if-else ka use hota hai.
Business Logic: Agar aapko kisi business rule ke basis par decision lena hai, toh if-else use hota hai.
Game Development: Games mein player ke actions par alag-alag responses generate karne ke liye if-else use hota hai.
8. Best Practices
Code ko Readable Banayein: Apne if-else blocks ko properly indent karein taaki code readable rahe.
Comments Likhe: Code mein comments add karne se samajhna asaan hota hai ki kya ho raha hai.
Avoid Nested If-Else: Jitna ho sake, nested if-else ko avoid karein. Isse code complex ho jaata hai.
Conditions Combine Karein: Logical operators ka use karke conditions ko combine karein.
9. Conclusion
If-Else statements Python mein decision making ke liye kaafi useful hote hain. Is post mein humne if-else statements ko simple aur asaan tarike se samjha. Ab aap inko apne code mein confidently use kar sakte hain. Practice karte rahein aur apne coding skills ko improve karte rahein!
Subscribe to my newsletter
Read articles from Sandhya Kondmare directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
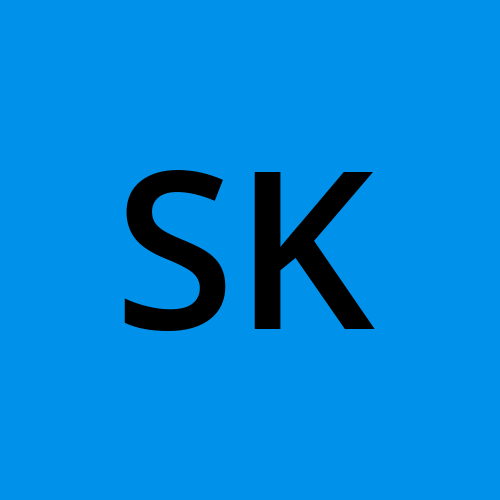
Sandhya Kondmare
Sandhya Kondmare
Aspiring DevOps Engineer with 2 years of hands-on experience in designing, implementing, and managing AWS infrastructure. Proven expertise in Terraform for infrastructure as code, automation tools, and scripting languages. Adept at collaborating with development and security teams to create scalable and secure architectures. Hands-on in AWS, GCP, Azure and Terraform best practices.