API 101 : Understanding RESTful APIs
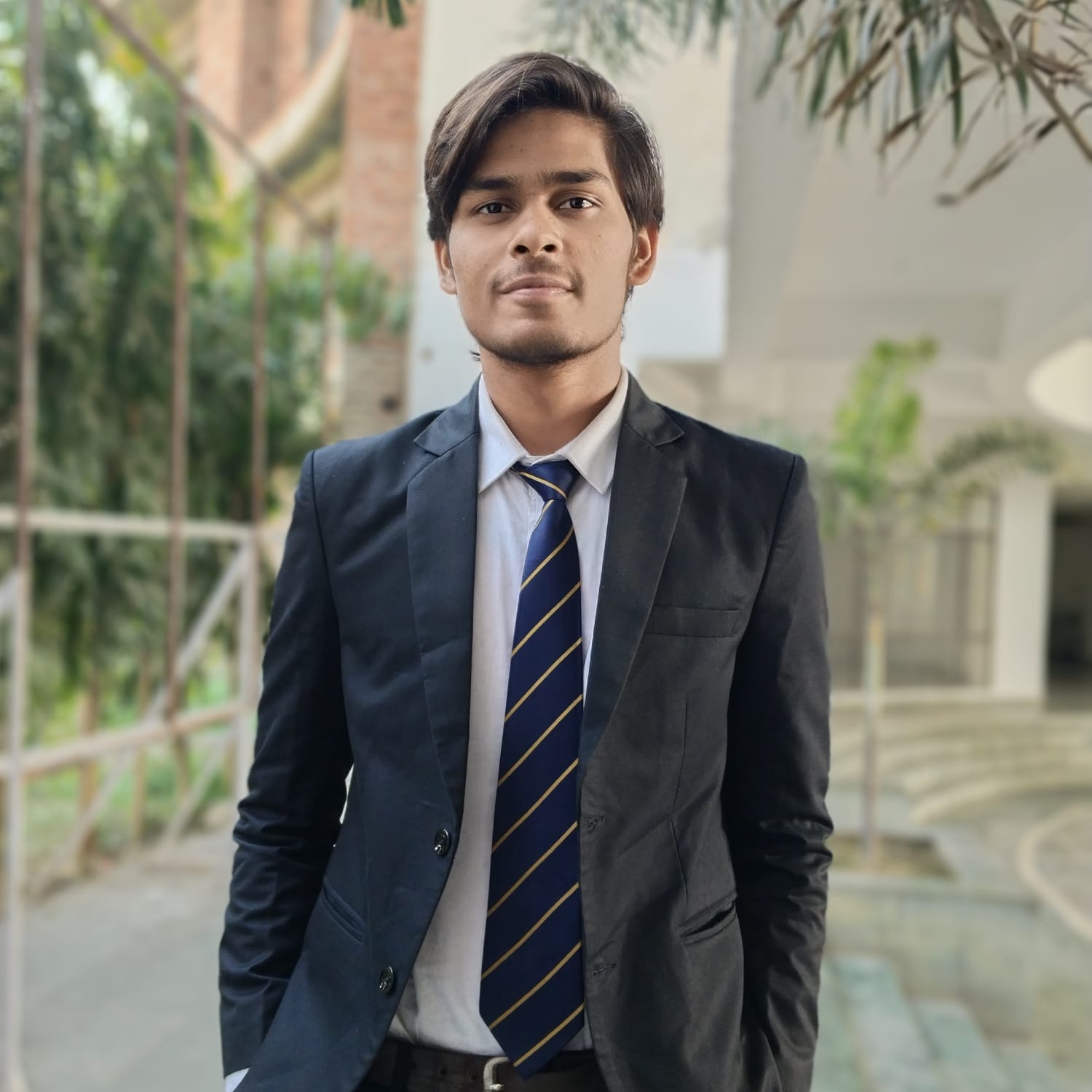
In the first part of this series, we introduced the concept of APIs and their importance in modern software development. Now, let’s delve deeper into one of the most widely used types of APIs: RESTful APIs. REST, or Representational State Transfer, is an architectural style that defines a set of constraints for creating web services. In this article, we'll explore the principles of REST, the common HTTP methods used in RESTful APIs, how endpoints and resources are structured, and the concept of statelessness.
What is REST?
REST (Representational State Transfer) is an architectural style that guides the design and development of network-based applications. It was introduced by Roy Fielding in his 2000 doctoral dissertation as a set of constraints that, when applied to web services, make them scalable, flexible, and easy to maintain.
The key idea behind REST is the concept of resources, which are identified by URIs (Uniform Resource Identifiers). Clients interact with these resources using a standard set of HTTP methods. RESTful APIs, therefore, are APIs that adhere to the principles of REST.
Core Principles of REST
Client-Server Architecture: In a RESTful system, the client and server are separate entities that communicate over a network. The client is responsible for the user interface and user experience, while the server handles the data and business logic. This separation allows for independent development and scaling of both the client and server.
Statelessness: Each request from a client to a server must contain all the information needed to understand and process the request. The server does not store any context about the client between requests. This stateless nature of REST makes the system more scalable, as each request is independent and can be handled in isolation.
Cacheability: Responses from the server can be marked as cacheable or non-cacheable. If a response is cacheable, the client can store the response and reuse it for subsequent requests, reducing the load on the server and improving performance.
Uniform Interface: RESTful APIs adhere to a uniform interface, meaning that the same set of rules and conventions are used across the entire API. This uniformity makes the API easier to understand and use.
Layered System: RESTful APIs can be designed with a layered architecture, where different layers (e.g., security, caching, load balancing) can be added between the client and server without affecting the communication between them.
Code on Demand (Optional): Although not always used, REST allows for the server to send executable code (e.g., JavaScript) to the client, which can then be executed on the client’s side.
HTTP Methods in RESTful APIs
RESTful APIs rely on standard HTTP methods to perform operations on resources. The most common methods are:
GET: The GET method is used to retrieve a resource from the server. For example, a GET request to
/users/123
would retrieve the user with the ID 123. GET requests are idempotent, meaning that making the same request multiple times will produce the same result without any side effects.POST: The POST method is used to create a new resource on the server. For example, a POST request to
/users
with a JSON payload containing user details would create a new user. POST requests are not idempotent; multiple POST requests can result in multiple resources being created.PUT: The PUT method is used to update an existing resource or create a resource if it doesn’t exist. For example, a PUT request to
/users/123
with a JSON payload containing updated user details would update the user with the ID 123.DELETE: The DELETE method is used to remove a resource from the server. For example, a DELETE request to
/users/123
would delete the user with the ID 123.PATCH: The PATCH method is used to partially update a resource. Unlike PUT, which replaces the entire resource, PATCH applies partial modifications to the resource.
Endpoints and Resources
In a RESTful API, a resource is any piece of information that can be named and addressed. Resources are identified by URIs (Uniform Resource Identifiers), and each resource is accessed via an endpoint.
For example, consider an API for managing a collection of books:
Resource: A book is a resource.
Endpoint: The URI to access the book resource could be
/books
.
Endpoints in RESTful APIs are usually designed to be intuitive and hierarchical. For example:
GET /books: Retrieve a list of all books.
GET /books/{id}: Retrieve a specific book by its ID.
POST /books: Create a new book.
PUT /books/{id}: Update an existing book by its ID.
DELETE /books/{id}: Delete a specific book by its ID.
Statelessness in REST
One of the defining characteristics of RESTful APIs is their statelessness. In a stateless system, each request from the client to the server must contain all the information needed to process the request. The server does not store any information about the client’s previous requests.
This stateless nature offers several advantages:
Scalability: Since each request is independent, the server can scale horizontally by adding more servers to handle incoming requests without worrying about maintaining session state.
Reliability: Stateless systems are more resilient to failures. If a server goes down, another server can take over without any loss of information, as each request is self-contained.
Simplicity: Stateless APIs are easier to design and maintain because there’s no need to manage session state on the server side.
However, statelessness also means that clients may need to include additional information (e.g., authentication tokens, request context) in each request, which can increase the size of the requests.
Conclusion
RESTful APIs are the backbone of modern web services, providing a flexible, scalable, and easy-to-use interface for interacting with resources over a network. By adhering to the principles of REST, developers can create APIs that are intuitive, reliable, and capable of handling large-scale operations. In the next part of this series, we will walk through the process of building your first RESTful API, covering everything from setting up your development environment to creating and testing basic endpoints.
Subscribe to my newsletter
Read articles from Shivay Dwivedi directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
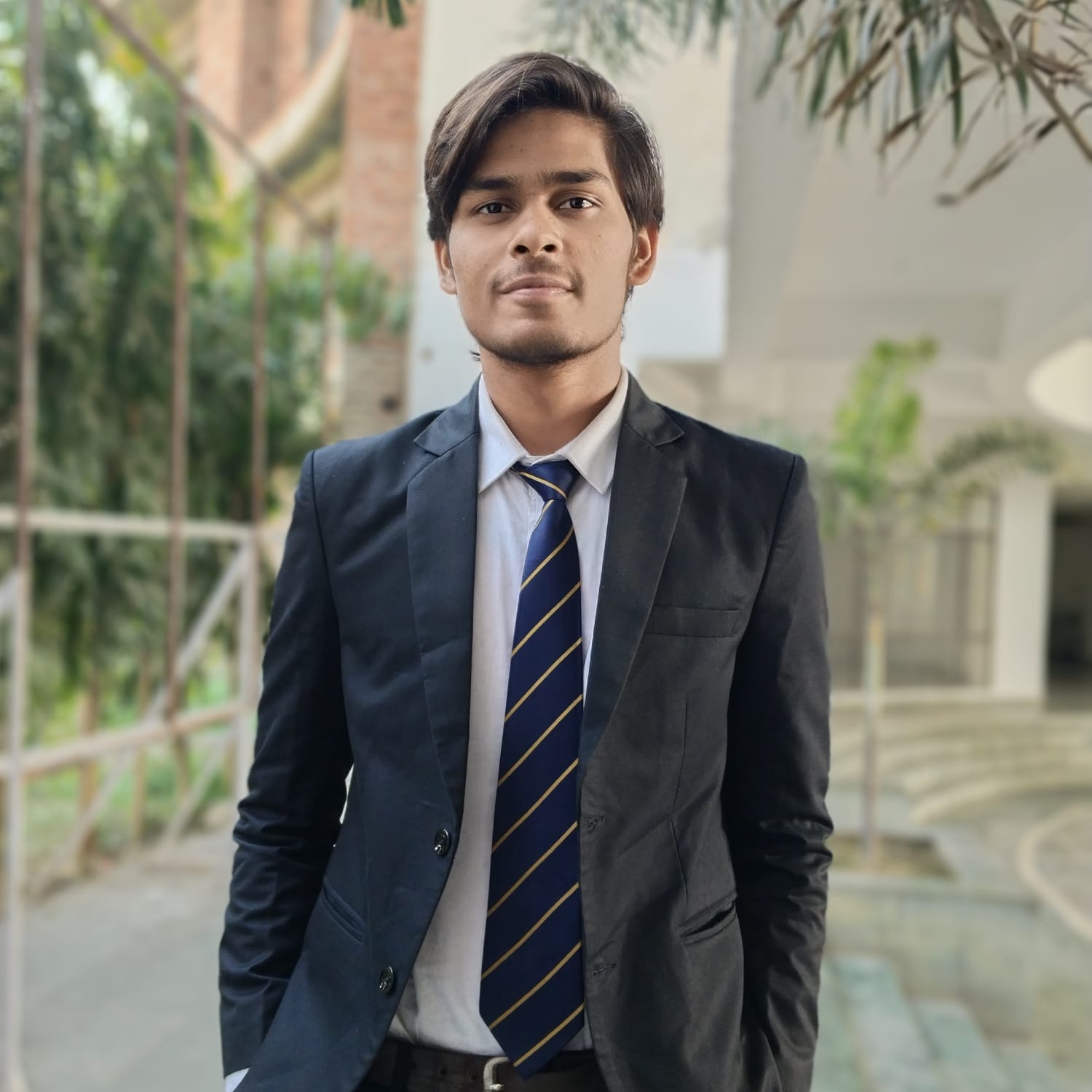