API 101 : Building Your First RESTful API
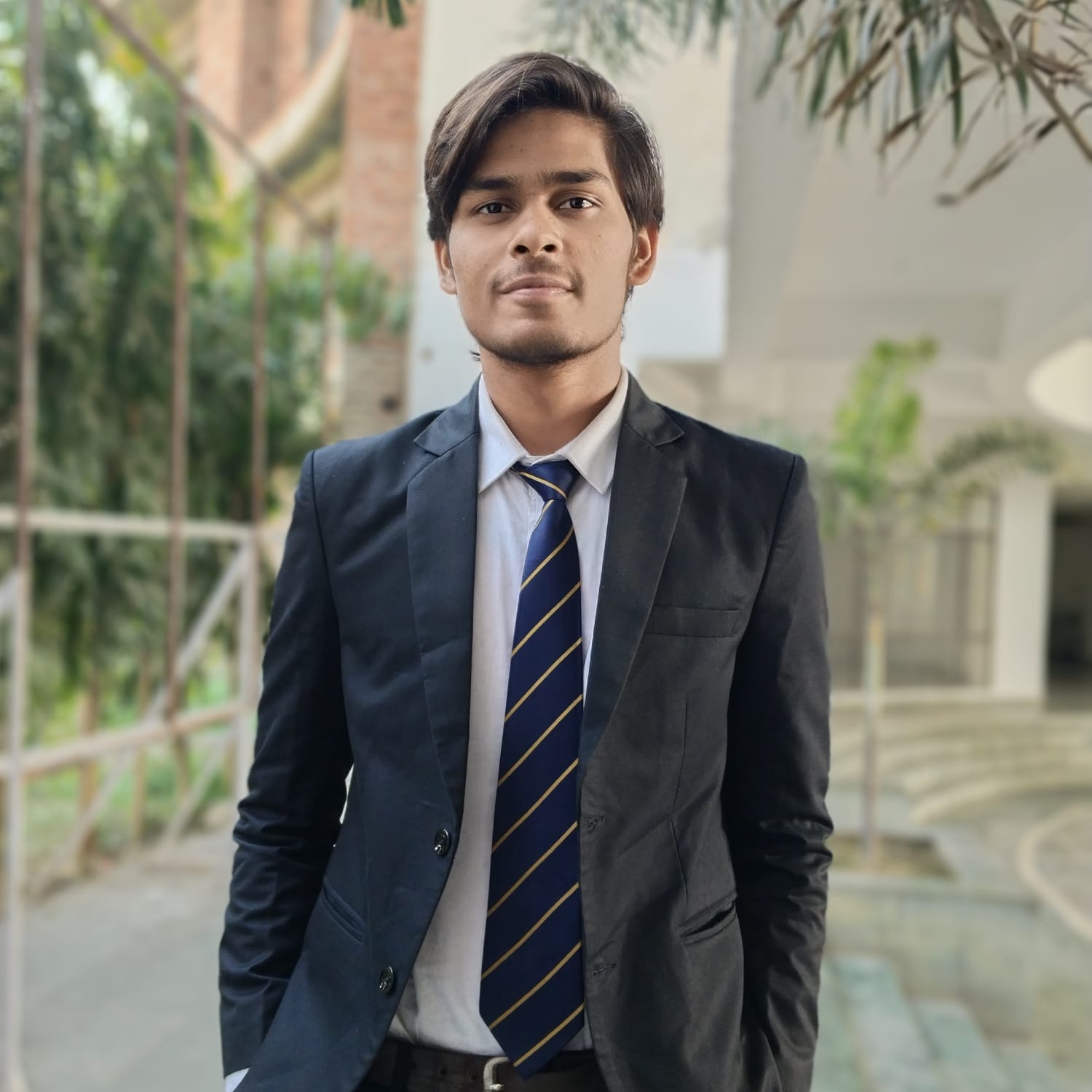
In the previous articles, we covered the basics of APIs and explored the principles of RESTful APIs. Now, it's time to put theory into practice by building your first RESTful API. This hands-on guide will walk you through the process of setting up your development environment, creating basic endpoints, handling requests and responses, and testing your API.
Setting Up Your Environment
Before you start building your API, you'll need to set up your development environment. We'll use Python and Flask, a lightweight web framework, to create our API. However, you can use other languages and frameworks like Node.js with Express if you prefer.
Step 1: Install Python and Flask
If you don't have Python installed on your machine, download it from python.org and follow the installation instructions. Once Python is installed, you can install Flask using pip
, Python's package manager.
Open your terminal and run:
pip install Flask
Step 2: Create a Project Directory
Create a new directory for your project and navigate into it:
mkdir my_first_api
cd my_first_api
Step 3: Create a Python File
In your project directory, create a new file called app.py
. This file will contain the code for your API.
Creating Basic Endpoints
With your environment set up, it's time to create some basic endpoints. We'll start with a simple API that allows users to manage a list of books. Our API will support the following operations:
GET: Retrieve the list of books or a specific book by ID.
POST: Add a new book to the list.
PUT: Update an existing book by ID.
DELETE: Remove a book from the list by ID.
Step 1: Initialize Flask
In app.py
, start by importing Flask and initializing the app:
from flask import Flask, jsonify, request
app = Flask(__name__)
# Sample data
books = [
{'id': 1, 'title': '1984', 'author': 'George Orwell'},
{'id': 2, 'title': 'To Kill a Mockingbird', 'author': 'Harper Lee'},
{'id': 3, 'title': 'The Great Gatsby', 'author': 'F. Scott Fitzgerald'}
]
Step 2: Create the GET Endpoint
Let's create a GET
endpoint to retrieve the list of books:
@app.route('/books', methods=['GET'])
def get_books():
return jsonify({'books': books})
This endpoint returns a JSON response containing the list of books.
Step 3: Create the POST Endpoint
Next, create a POST
endpoint to add a new book:
@app.route('/books', methods=['POST'])
def add_book():
new_book = request.get_json()
books.append(new_book)
return jsonify(new_book), 201
This endpoint accepts a JSON payload containing the book details, adds it to the list, and returns the newly added book with a 201 Created
status code.
Step 4: Create the PUT Endpoint
Now, let's create a PUT
endpoint to update an existing book by its ID:
@app.route('/books/<int:book_id>', methods=['PUT'])
def update_book(book_id):
book = next((b for b in books if b['id'] == book_id), None)
if book is None:
return jsonify({'error': 'Book not found'}), 404
updated_data = request.get_json()
book.update(updated_data)
return jsonify(book)
This endpoint searches for the book by its ID, updates it with the provided data, and returns the updated book. If the book is not found, it returns a 404 Not Found
error.
Step 5: Create the DELETE Endpoint
Finally, create a DELETE
endpoint to remove a book by its ID:
@app.route('/books/<int:book_id>', methods=['DELETE'])
def delete_book(book_id):
global books
books = [b for b in books if b['id'] != book_id]
return '', 204
This endpoint removes the book with the specified ID from the list and returns a 204 No Content
status code.
Step 6: Run the Application
At the end of app.py
, add the following code to run the application:
if __name__ == '__main__':
app.run(debug=True)
Now, you can start the API by running:
python app.py
Your API will be running locally at http://127.0.0.1:5000
.
Handling Requests and Responses
In our API, we're using Flask's request
object to handle incoming requests and jsonify
to send JSON responses. Let's break down how this works:
GET Request: When a client sends a
GET
request to/books
, Flask handles the request and returns the list of books as a JSON object.POST Request: When a client sends a
POST
request with a JSON payload to/books
, Flask parses the JSON data, adds it to the list, and sends back the newly added book as a JSON response.PUT Request: When a client sends a
PUT
request to/books/<book_id>
with updated data, Flask searches for the book, updates it, and sends back the updated book as a JSON response.DELETE Request: When a client sends a
DELETE
request to/books/<book_id>
, Flask removes the book from the list and sends back a204 No Content
status code.
Each of these endpoints follows the principles of RESTful design, ensuring that the API is intuitive and adheres to standard HTTP methods.
Testing Your API
Now that your API is up and running, it's time to test it. You can use tools like Postman, cURL, or even your browser to send requests to your API and see the responses.
Testing with Postman
GET Request:
Open Postman and create a new request.
Set the request type to
GET
.Enter the URL
http://127.0.0.1:5000/books
and clickSend
.You should see the list of books returned as a JSON response.
POST Request:
Create a new request and set the request type to
POST
.Enter the URL
http://127.0.0.1:5000/books
.In the
Body
tab, selectraw
and set the format toJSON
.Enter the JSON payload for a new book, e.g.:
{ "id": 4, "title": "Moby Dick", "author": "Herman Melville" }
Click
Send
and verify that the new book is added.
PUT Request:
Create a
PUT
request to update an existing book.Use the URL
http://127.0.0.1:5000/books/4
.In the
Body
tab, enter the updated JSON data, e.g.:{ "title": "Moby Dick (Updated)", "author": "Herman Melville" }
Click
Send
and check the updated book details.
DELETE Request:
Create a
DELETE
request to remove a book.Use the URL
http://127.0.0.1:5000/books/4
.Click
Send
and verify that the book is removed.
Testing with cURL
You can also use cURL in the terminal to test your API:
GET:
curl http://127.0.0.1:5000/books
POST:
curl -X POST -H "Content-Type: application/json" -d '{"id":4,"title":"Moby Dick","author":"Herman Melville"}' http://127.0.0.1:5000/books
PUT:
curl -X PUT -H "Content-Type: application/json" -d '{"title":"Moby Dick (Updated)"}' http://127.0.0.1:5000/books/4
DELETE:
curl -X DELETE http://127.0.0.1:5000/books/4
Conclusion
Congratulations! You’ve just built and tested your first RESTful API. In this article, you learned how to set up a development environment, create basic endpoints, handle requests and responses, and test your API using tools like Postman and cURL. This foundation will serve you well as you continue to explore and develop more complex APIs.
In the next part of this series, we’ll dive into the crucial topics of securing and documenting your APIs. You'll learn how to protect your API from unauthorized access and create documentation that makes it easy for others to use your API effectively.
Subscribe to my newsletter
Read articles from Shivay Dwivedi directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
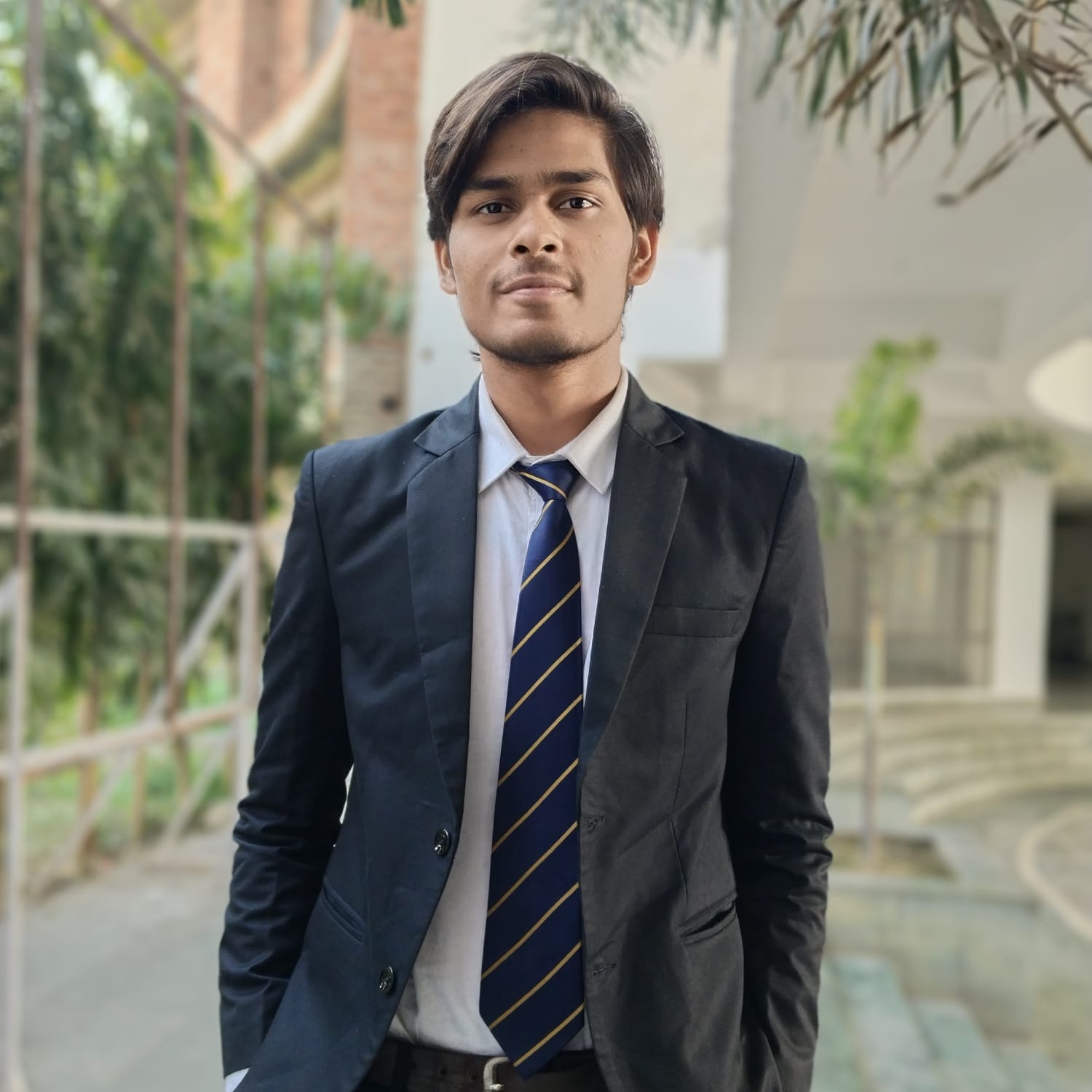