React and Tailwind
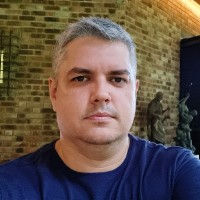
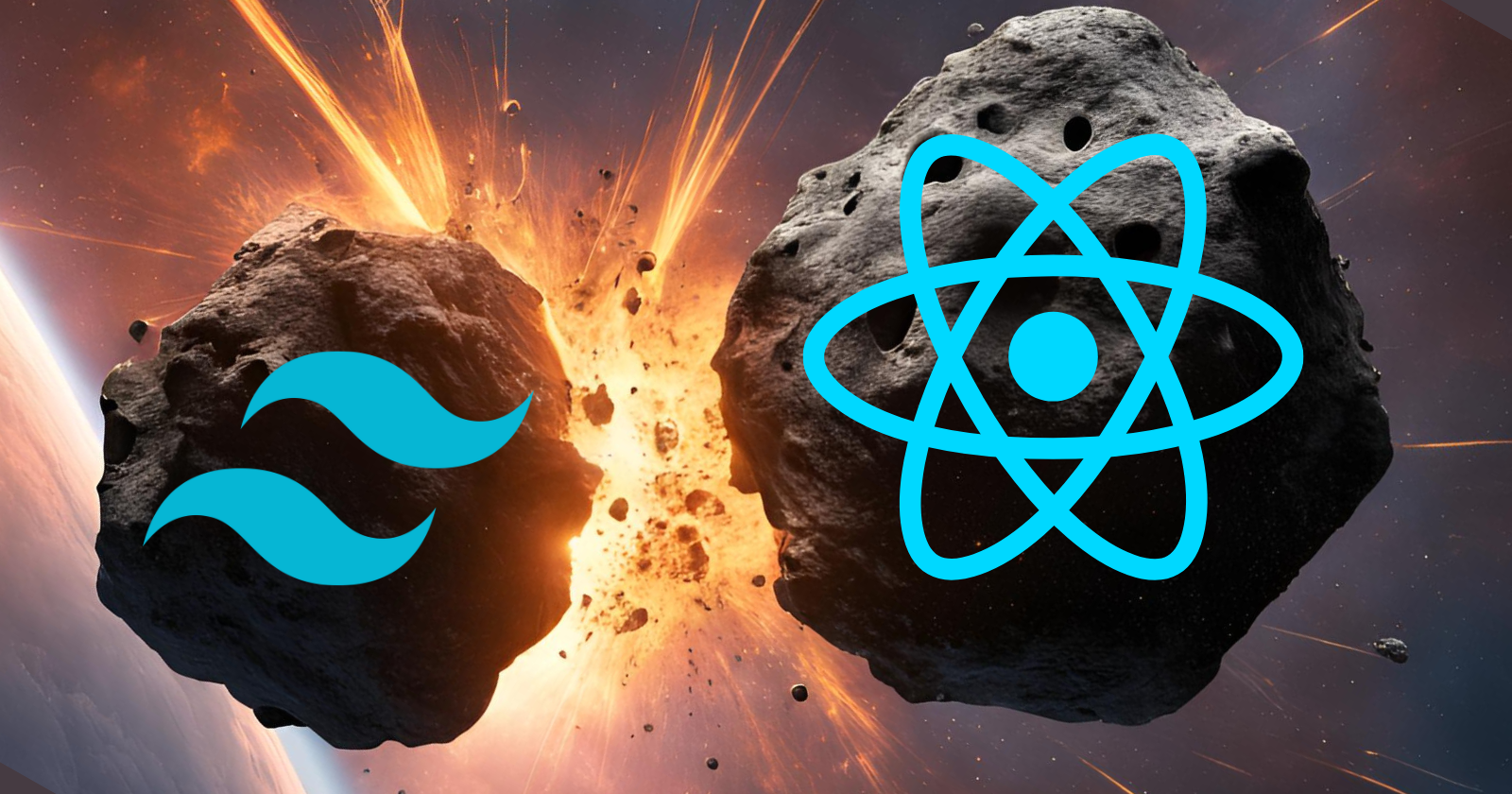
React and Tailwind CSS are a perfect combination for front-end development due to their component-based architecture and utility-first approach, respectively. This article guides you through setting up a React project with Tailwind, explains the advantages of using Tailwind over plain CSS, and provides examples and fun project ideas to help you get started. Dive in to learn how these tools enable rapid prototyping, maintainable code, and consistent, responsive design.
When it comes to front-end development, choosing the right tools can make a world of difference. Enter React and Tailwind CSS: two powerhouses that, when combined, create an efficient and enjoyable development experience. In this article, we'll explore why React and Tailwind are a perfect pair, how to get them up and running, and some fun projects to kickstart your journey.
Why React and Tailwind Are a Perfect Pair
React: The UI Library Extraordinaire
React is a JavaScript library for building user interfaces, developed by Facebook. It's component-based, which means you can build encapsulated components that manage their own state and then compose them to make complex UIs.
Tailwind CSS: The Utility-First CSS Framework
Tailwind CSS is a utility-first CSS framework that provides low-level utility classes to build custom designs without leaving your HTML. It offers a more efficient way to style your application by using predefined classes directly in your markup.
Why They Work Well Together
Component-Based Structure: React's component-based architecture complements Tailwind's utility-first approach perfectly. You can easily apply Tailwind's utility classes to React components, making your code cleaner and more maintainable.
Rapid Prototyping: Both React and Tailwind are excellent for rapid prototyping. React's reusable components and Tailwind's extensive utility classes allow you to build and iterate quickly.
Consistency: Tailwind ensures a consistent design system across your application, while React components ensure consistent behavior and structure.
Setting Up React and Tailwind
Step 1: Create a New React Project
First, you'll need to have Node.js and npm installed. If you haven't already, download and install them from nodejs.org.
To create a new React project, open your terminal and run:
npx create-react-app my-app
cd my-app
Step 2: Install Tailwind CSS
Next, you'll need to install Tailwind CSS and its dependencies:
npm install -D tailwindcss postcss autoprefixer
npx tailwindcss init -p
This will create a tailwind.config.js
file and a postcss.config.js
file in your project.
Step 3: Configure Tailwind
Update the tailwind.config.js
file to include the paths to all of your template files:
module.exports = {
content: [
"./src/**/*.{js,jsx,ts,tsx}",
],
theme: {
extend: {},
},
plugins: [],
}
Next, add Tailwind's directives to your CSS file. Create a new CSS file in the src
directory called index.css
and add the following:
@tailwind base;
@tailwind components;
@tailwind utilities;
Finally, import this CSS file in your src/index.js
:
import './index.css';
Advantages of Using Tailwind Over Plain CSS
1. Utility-First Approach
Tailwind provides low-level utility classes that let you build custom designs without leaving your HTML. This approach eliminates the need for writing custom CSS for each element.
2. Responsive Design Made Easy
With Tailwind, you can easily create responsive designs by adding responsive variants to your utility classes. For example:
<div class="text-center md:text-left lg:text-right">
Responsive Text Alignment
</div>
3. Consistency and Maintainability
Tailwind's utility classes ensure a consistent design language across your application. You can quickly make changes to your design system by updating your Tailwind configuration, which will reflect across your entire application.
4. Rapid Prototyping
Tailwind's extensive set of utility classes allows you to prototype quickly and efficiently, speeding up your development process.
React + Tailwind: The Perfect Match
Let's see a simple example of a React component styled with Tailwind:
Example: Button Component
Button.js
import React from 'react';
const Button = ({ label }) => {
return (
<button className="bg-blue-500 hover:bg-blue-700 text-white font-bold py-2 px-4 rounded">
{label}
</button>
);
};
export default Button;
This example demonstrates how easy it is to style a React component using Tailwind's utility classes. The classes bg-blue-500
, hover:bg-blue-700
, text-white
, font-bold
, py-2
, px-4
, and rounded
are all Tailwind utilities that control the button's appearance.
Fun Projects to Try
1. To-Do List Application
Build a simple to-do list application where you can add, remove, and mark tasks as complete. Use Tailwind to style the application and make it look clean and modern.
2. Weather App
Create a weather application that fetches weather data from an API and displays it beautifully using Tailwind's responsive design utilities.
3. Portfolio Website
Develop your personal portfolio website using React for dynamic content and Tailwind for stunning and consistent design.
Learning Resources
For React
Codecademy: Learn React
Udemy: React - The Complete Guide (incl Hooks, React Router, Redux)
Scrimba: Learn React for free
For Tailwind CSS
Tailwind CSS Documentation: Tailwind CSS
Tailwind Labs YouTube Channel: Tailwind Labs
For Both
Frontend Masters: Complete Intro to React, v6
Coursera: Responsive Website Development and Design Specialization
Conclusion
React and Tailwind CSS together form a powerhouse duo for front-end development. React's component-based structure and Tailwind's utility-first CSS approach complement each other perfectly, allowing for rapid development, consistent design, and maintainable code. By mastering these tools, you can build stunning, responsive web applications with ease. So, dive in, start experimenting with fun projects, and watch your skills soar!
Happy coding! 🎉
Subscribe to my newsletter
Read articles from Hugo Tavares directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
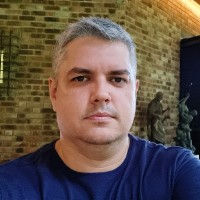
Hugo Tavares
Hugo Tavares
Hello World, my name is Hugo. I’m a Christian, husband, and father. I hold a degree in Administration and have experience in the military as an air traffic controller. Additionally, I’m a frontend developer, fascinated by technology, and constantly seeking to learn new things.