Cloud Messaging Patterns
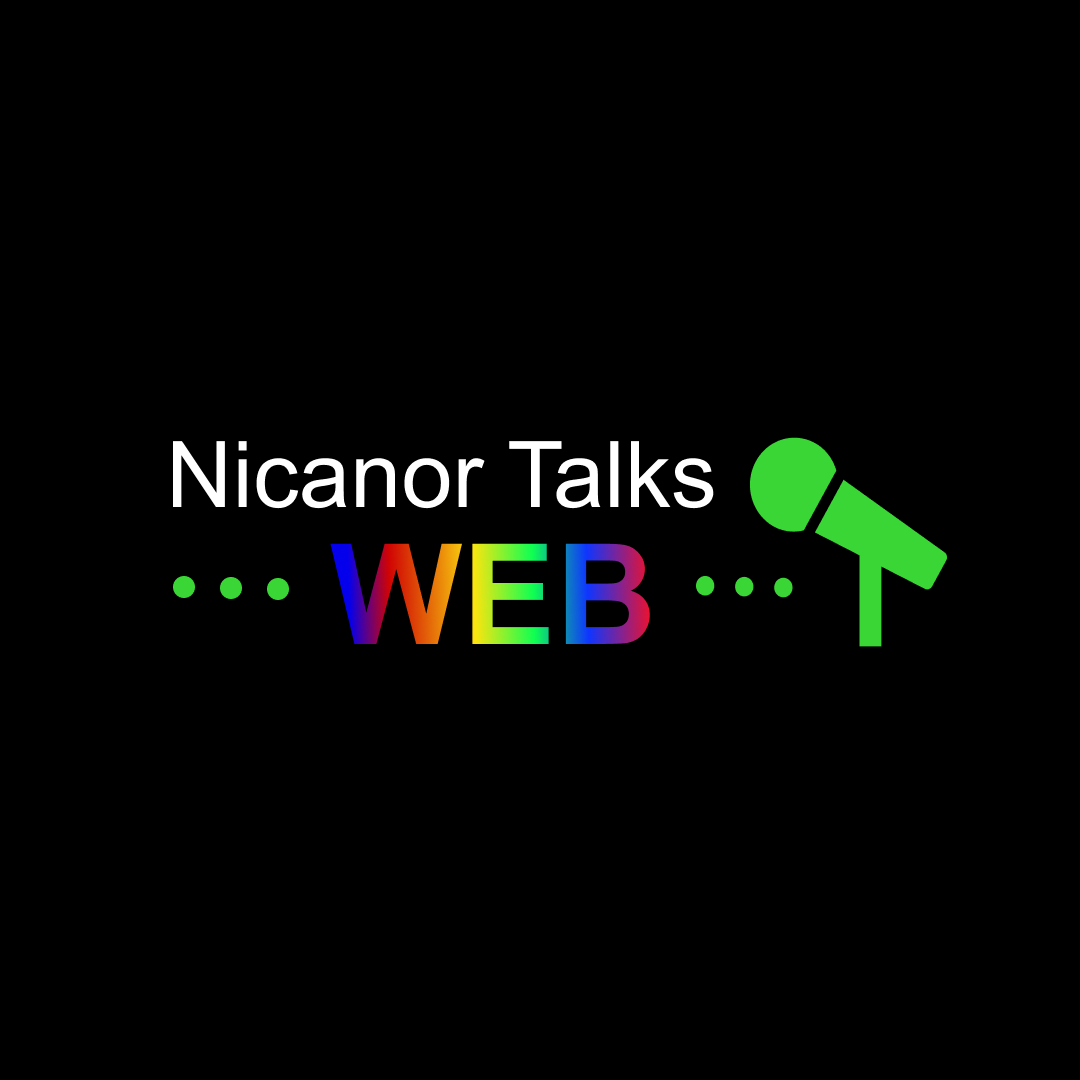
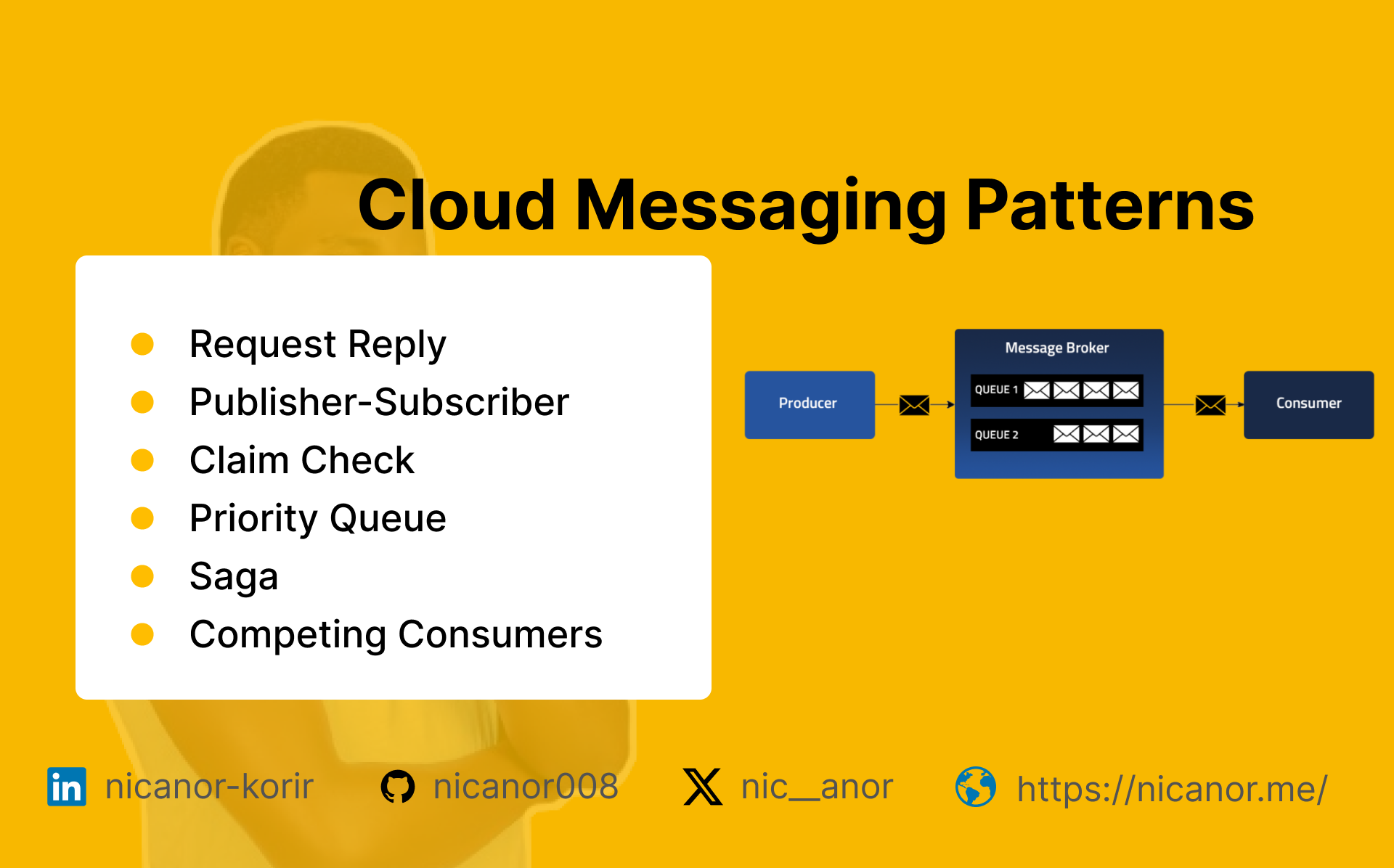
Cloud messaging is a fundamental component of modern distributed systems. It involves the exchange of information between different software components or applications. The core idea is to decouple systems, allowing them to operate independently while still communicating effectively. This is achieved through the use of messaging patterns.
What is a Message?
A message is a structured data unit containing information to be communicated. It typically includes a header (metadata like sender, recipient, timestamp) and a body (actual data).
Key Components of a Messaging System
Producer: The entity that creates and sends messages.
Broker: A software component that handles the routing, queuing, and delivery of messages.
Consumer: The entity that receives and processes messages.
Fundamental Messaging Patterns
Publish-Subscribe
Description: A publisher sends messages to a topic, and multiple subscribers can subscribe to that topic to receive messages.
Use Cases: Real-time updates, notifications, log aggregation, and data distribution.
Example: A news website publishes articles on a "news" topic. Multiple users subscribe to receive the latest news.
Point-to-Point
Description: A message is sent from one producer to one specific consumer.
Use Cases: Task queues, work distribution, and reliable messaging.
Example: A job queue where tasks are assigned to available workers.
Advanced Messaging Patterns
Request-Reply
Description: A producer sends a request message and expects a response from a consumer.
Use Cases: Remote procedure calls, service invocation, and synchronous communication.
Example: A web application sends a request to a backend service and waits for a response.
Competing Consumers
Description: Multiple consumers actively compete to process messages from a queue.
Use Cases: High-throughput message processing, load balancing, and fault tolerance.
Example: A queue of customer orders with multiple workers processing them.
Message Broker
Description: A central component responsible for routing, queuing, and delivering messages.
Use Cases: Message queuing, message distribution, and message transformation.
Examples: Apache Kafka, RabbitMQ, and Amazon SQS.
Additional Patterns and Considerations
Dead Letter Queue: A queue for messages that cannot be processed successfully.
Message Transformation: Modifying the content or format of a message before delivery.
Message Routing: Directing messages to specific destinations based on content or other criteria.
Message Filtering: Selecting messages based on specific criteria before delivery.
Message Expiration: Setting a time-to-live for messages.
Message Batching: Combining multiple messages into a single message for efficiency.
Message Deduplication: Ensuring that a message is processed only once.
Message Ordering: Guaranteeing that messages are processed in the order they were sent.
Message Prioritization: Assigning priorities to messages to influence processing order.
Choosing the Right Pattern
Selecting the appropriate messaging pattern depends on factors such as:
Message delivery guarantees: At-least-once, at-most-once, or exactly-once delivery.
Message ordering: Is it important for messages to be processed in order?
Scalability: How well does the pattern handle increasing message volume and number of consumers?
Reliability: How important is it that messages are not lost or corrupted?
Latency: How quickly do messages need to be processed?
Real-World Examples
E-commerce: Order processing, inventory management, fraud detection.
Financial Services: Trade execution, risk management, customer notifications.
IoT: Data collection, device management, and real-time analytics.
Social Media: Feed updates, notifications, and message delivery.
Advanced Topics
Saga Pattern: Coordinating distributed transactions.
Event Sourcing: Storing all changes to an application state as a sequence of events.
Stream Processing: Processing continuous streams of data.
Message Queues vs. Message Brokers: In-depth comparison of these two components.
You can effectively design and implement robust and scalable messaging systems by understanding these patterns and their applications.
Publisher-Subscriber Pattern
The publisher sends messages to a topic, and multiple subscribers can subscribe to that topic to receive notifications. This pattern is ideal for decoupling systems and enabling scalable, asynchronous communication.
Using AWS SNS and SQS
For this example, we'll use AWS Simple Notification Service (SNS) as the topic and Simple Queue Service (SQS) as the subscriber.
Prerequisites
An AWS account with the necessary permissions
Node.js and the AWS SDK installed
const AWS = require('aws-sdk');
const sns = new AWS.SNS();
const sqs = new AWS.SQS();
// Create a SNS topic (replace with your desired topic name)
const createTopicParams = {
Name: 'myTopic'
};
sns.createTopic(createTopicParams, (err, data) => {
if (err) {
console.error('Error creating topic:', err);
} else {
console.log('Topic created:', data);
const topicArn = data.TopicArn;
// Create an SQS queue (replace with your desired queue name)
const createQueueParams = {
QueueName: 'myQueue'
};
sqs.createQueue(createQueueParams, (err, data) => {
if (err) {
console.error('Error creating queue:', err);
} else {
console.log('Queue created:', data);
const queueUrl = data.QueueUrl;
// Subscribe the SQS queue to the SNS topic
const subscribeParams = {
TopicArn: topicArn,
Protocol: 'sqs',
Endpoint: queueUrl
};
sns.subscribe(subscribeParams, (err, data) => {
if (err) {
console.error('Error subscribing queue:', err);
} else {
console.log('Subscription created:', data);
}
});
}
});
}
});
// Publish a message to the SNS topic
const publishParams = {
Message: 'Hello from SNS!',
TopicArn: topicArn
};
sns.publish(publishParams, (err, data) => {
if (err) {
console.error('Error publishing message:', err);
} else {
console.log('Message published:', data);
}
});
Explanation
Create an SNS Topic: Create a new SNS topic.
Create SQS Queue: Create a new SQS queue.
Subscribe SQS to SNS: Subscribes the created SQS queue to the SNS topic.
Publish Message: Publishes a message to the SNS topic.
Note: This is a basic example. In a real-world application, you typically use asynchronous functions, error handling, and more robust configuration.
Using GCP Pub/Sub
While the core concept remains the same, the implementation differs between AWS and GCP. For GCP Pub/Sub, you would use the @google-cloud/pubsub
library.
JavaScript
const { PubSub } = require('@google-cloud/pubsub');
// Create a Pub/Sub client
const pubsub = new PubSub();
// Create a topic
const topicName = 'my-topic';
const topic = pubsub.topic(topicName);
// Publish a message
async function publishMessage() {
const dataBuffer = Buffer.from('Hello, world!');
const messageId = await topic.publish(dataBuffer);
console.log(`Message ${messageId} published.`);
}
publishMessage();
Note:
This is a simplified example. For more complex scenarios, you would typically create subscriptions, handle message acknowledgements, and implement error handling.
Both AWS and GCP offer robust Publish-Subscribe services.
Choose the platform based on your existing infrastructure and preferences.
Consider factors like scalability, reliability, and cost when selecting a service.
Explore advanced features like message ordering, dead-letter queues, and message filtering.
Use Case: Real-time Analytics with Pub/Sub
Problem: Process and analyze large volumes of data in real-time for insights and decision-making.
Solution:
Data Ingestion: Use Pub/Sub as a data ingestion pipeline to collect data from various sources (IoT devices, web applications, databases).
Data Processing: Utilize serverless functions or streaming platforms (like Apache Beam, and Apache Flink) to process data as it arrives.
Data Analysis: Apply machine learning models or statistical analysis to extract insights from the processed data.
Real-time Dashboards: Visualize results on interactive dashboards.
Example: A telecommunications company uses Pub/Sub to collect network data from IoT devices. By processing this data in real time, they can identify network congestion, optimize resource allocation, and detect anomalies.
Advanced Topic: Message Durability and Ordering
Durability: Guaranteeing message delivery even in case of system failures.
Ordering: Ensuring messages are delivered to subscribers in the same order they were published.
Considerations:
At least once delivery: Message is delivered at least once, but possibly multiple times.
At-most-once delivery: Message is delivered at most once, but might be lost.
Exactly-once delivery: The message is delivered exactly once, ensuring data consistency.
Trade-offs: Durability and ordering often come at the cost of performance and complexity.
Challenges and Best Practices
Scalability: Handle increasing message volumes and subscriber numbers.
Reliability: Ensure high availability and fault tolerance.
Security: Protect sensitive data with encryption and access controls.
Cost Optimization: Manage costs effectively based on usage patterns.
Best Practices:
Use appropriate message formats (JSON, Avro, Protobuf) for efficiency.
Implement retry mechanisms for failed message deliveries.
Monitor system performance and optimize as needed.
Consider using dead-letter queues for undeliverable messages.
Subscribe to my newsletter
Read articles from Nicanor Talks Web directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
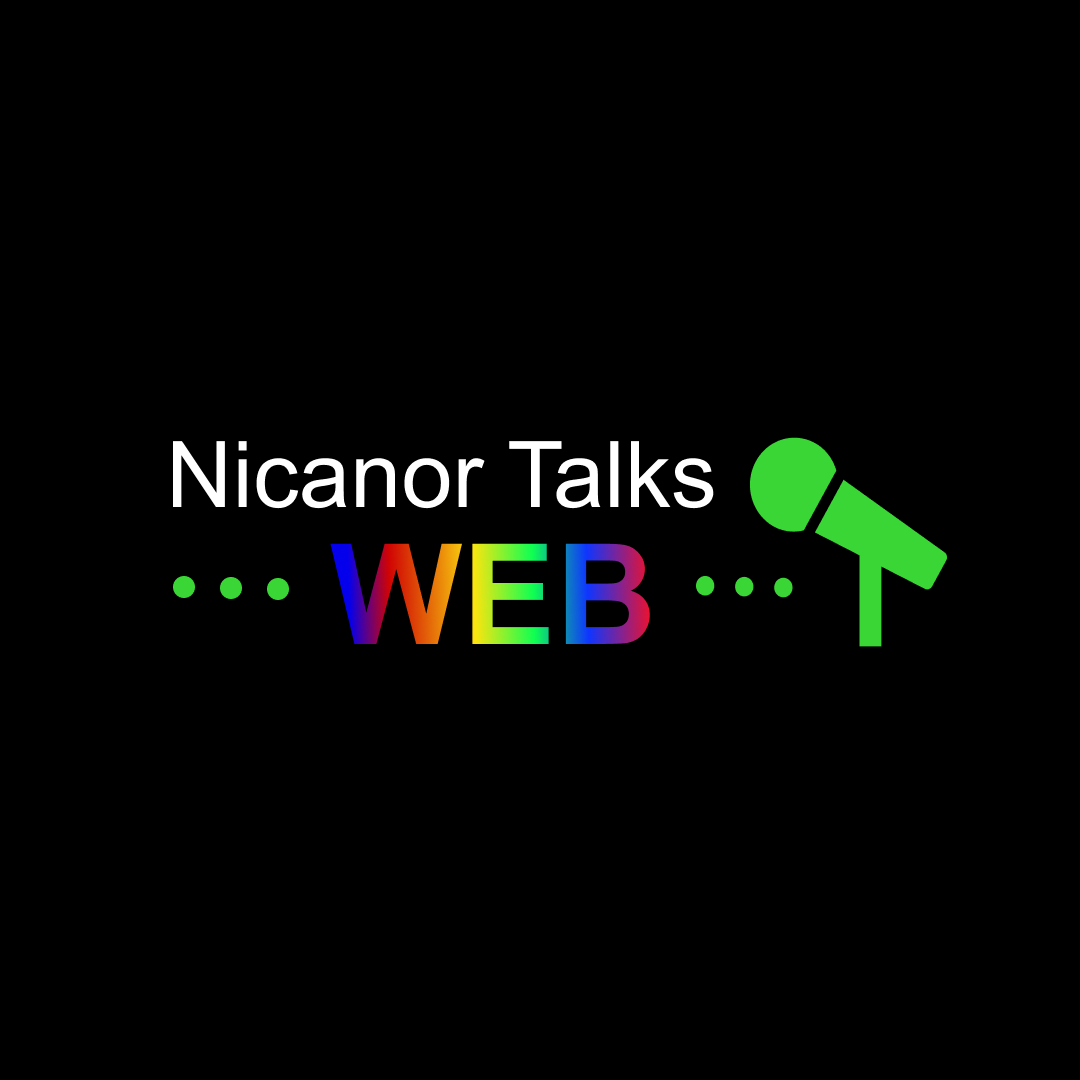
Nicanor Talks Web
Nicanor Talks Web
Hi there! I'm Nicanor, and I'm a Software Engineer. I'm passionate about solving humanitarian problems through tech, and I love sharing my knowledge and insights with others.