The Foundation of Efficient Code: Understanding Big O Notation and Complexity
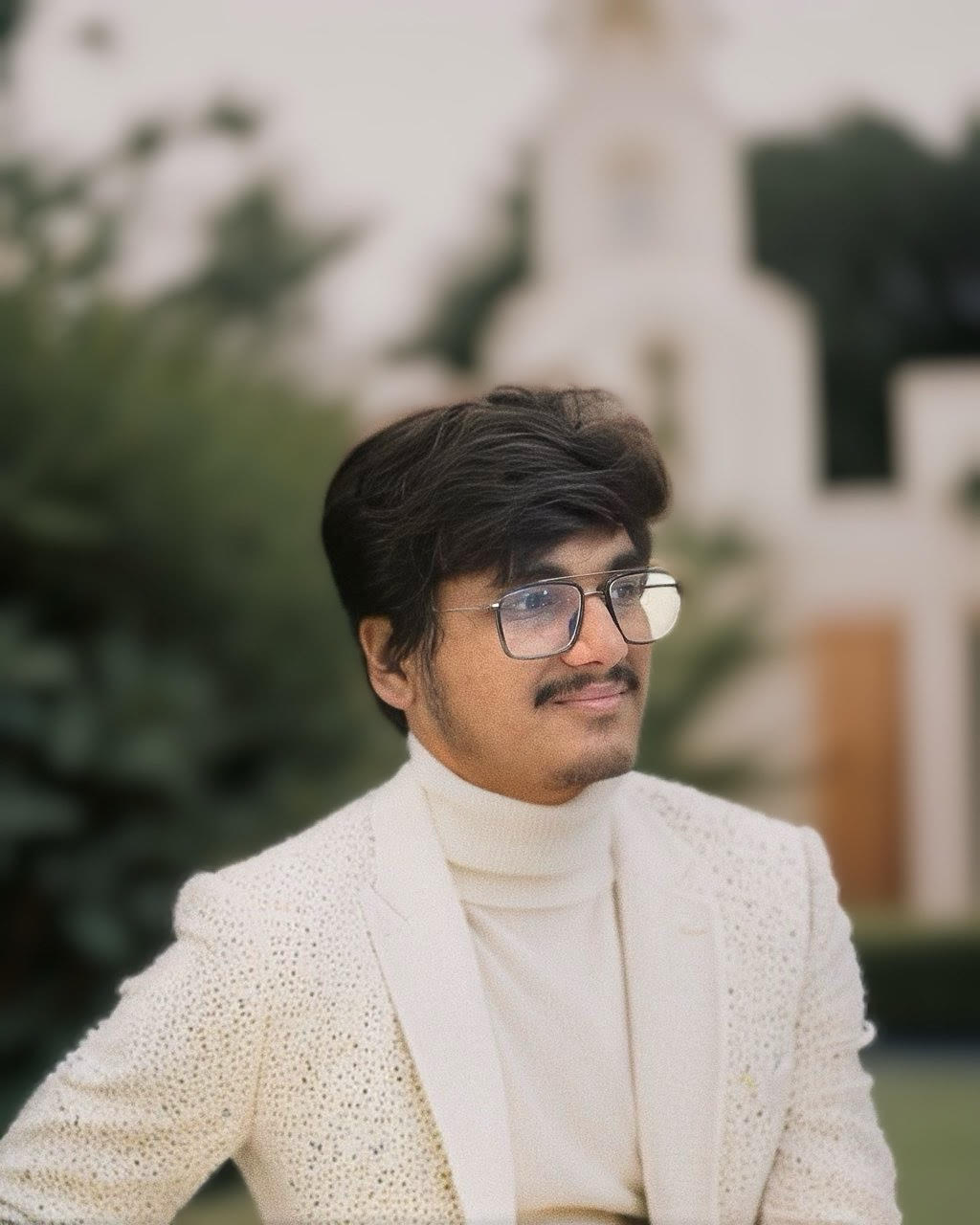
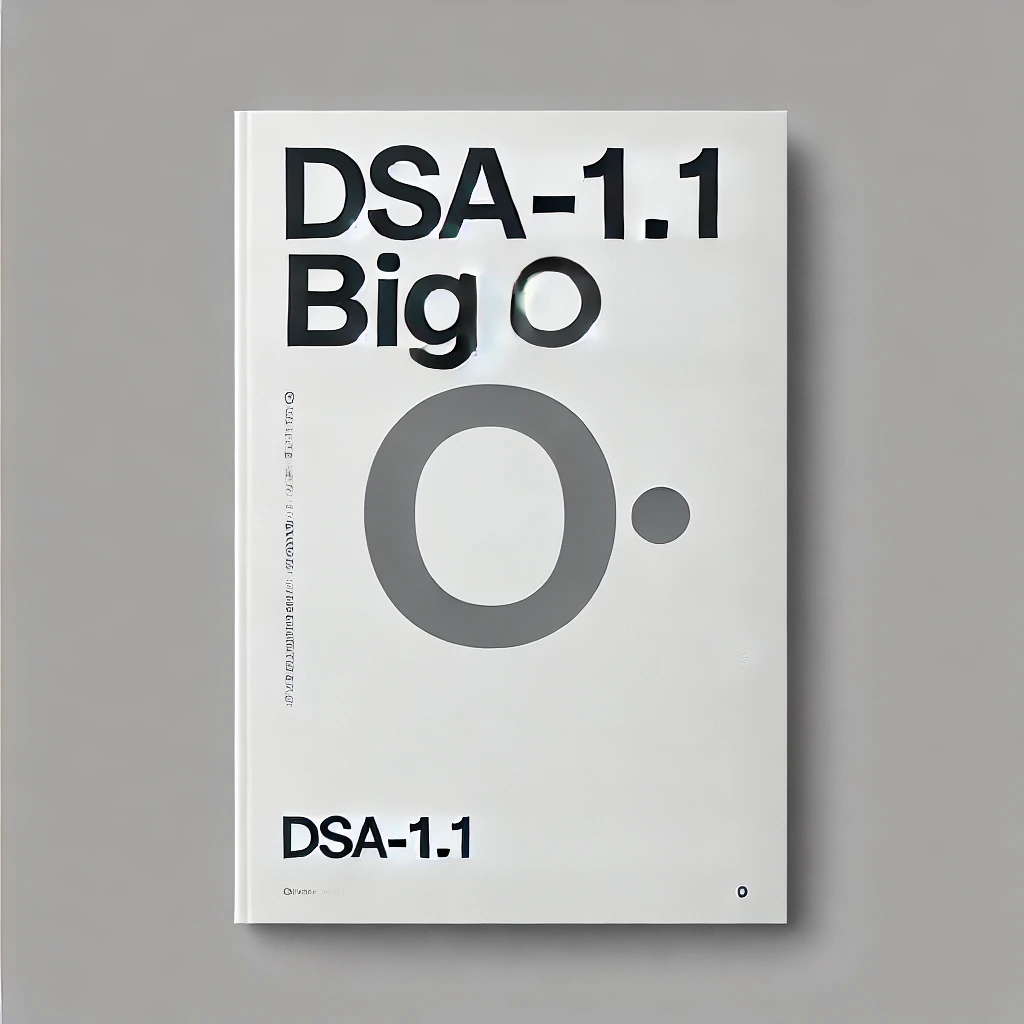
Welcome to Week 1 of our series, where we dive into the essentials of writing efficient and scalable code. In this series, I’ll be using Python as my primary language, but don’t worry—this content is language-agnostic. Whether you’re a seasoned developer or just starting, you’ll find value in understanding the principles we discuss.
Why Good Code Matters
Good code is more than just functional—
It’s maintainable ⚙️
Scalable 📈
Efficient 🎖️.
As developers, we aim to write code that works and performs well under varying conditions. Efficient code is especially crucial in large systems where performance bottlenecks can lead to significant issues, such as slower response times, higher resource consumption, and increased operational costs.
Introducing Big O Notation
To measure the efficiency of our code, we use Big O notation. Big O is a mathematical notation that describes the upper bound of an algorithm’s runtime as a function of the input size. It gives us a way to express how an algorithm's execution time or space requirements grow as the input size increases.
In simple terms, Big O helps us answer the question: “How does the performance of my code scale as the amount of data it processes grows?”
The Rules of Big O Notation
Understanding the rules of Big O notation is crucial to evaluating and optimizing your algorithms. Here are the key principles:
Constant Time - O(1): The execution time remains constant, regardless of the input size.
Linear Time - O(n): The execution time grows linearly with the input size.
Logarithmic Time - O(log n): The execution time grows logarithmically as the input size increases.
Quadratic Time - O(n^2): The execution time grows proportionally to the square of the input size.
These are just a few examples; there are other complexities like O(n log n), O(n!), and so on. The key is to understand the behavior of your algorithms under different input conditions.
Calculating Big O Notation
To calculate Big O, we focus on the most significant factor in an algorithm's execution time. Consider the following example:
def example_function(arr):
for i in arr: # This loop runs n times where n is the size of arr
for j in arr: # This nested loop also runs n times
print(i, j) # A simple operation
Here, the outer loop runs n
times, and for each iteration of the outer loop, the inner loop runs n
times, leading to an O(n^2) complexity. Understanding this helps us identify and optimize performance bottlenecks.
Time and Space Complexity
Big O is not just about time; it’s also about space. Time complexity refers to the amount of time an algorithm takes to complete, while space complexity refers to the amount of memory it requires. Both are crucial in determining the overall efficiency of an algorithm.
For instance, sorting algorithms like QuickSort are often preferred because they offer a good balance of time (O(n log n)) and space complexity. On the other hand, algorithms with high time or space complexity might not be suitable for large datasets or systems with limited resources.
Conclusion
This week, we’ve laid the groundwork for understanding the importance of writing good code through the lens of Big O notation. We’ve touched on what Big O is, the various rules, and how to calculate it. As we progress through this series, we’ll dive deeper into more complex topics, all while reinforcing the importance of writing efficient, maintainable code.
In next post I will explain how Big O is calculated in a detailed manner!!
Stay tuned, and happy coding!
Subscribe to my newsletter
Read articles from SAI GOUTHAM directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
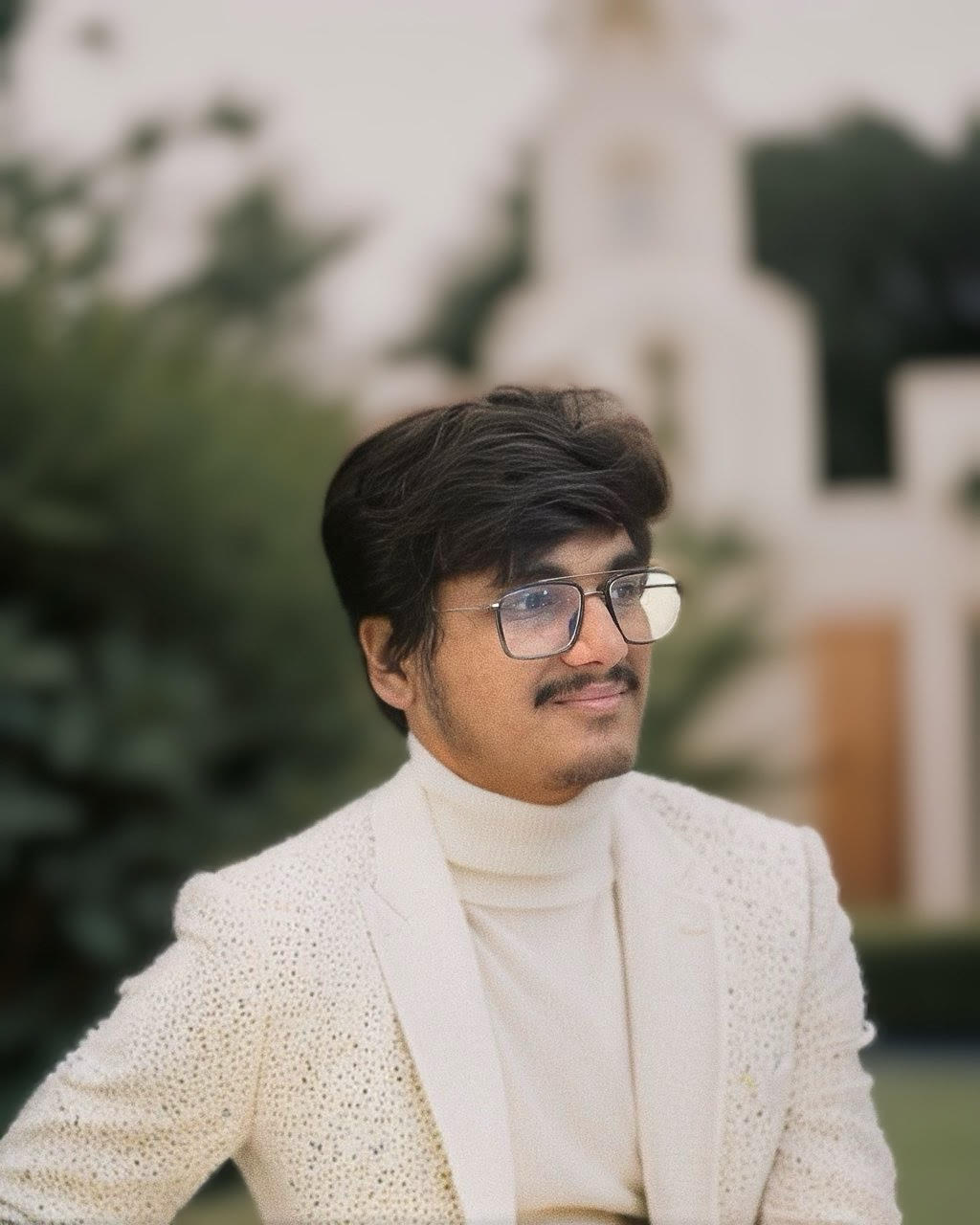
SAI GOUTHAM
SAI GOUTHAM
💻 Experienced Computer Science graduate with 3+ years in software engineering, specializing in full-stack web development and cloud solutions. 🥇 Proficient in Python, JavaScript, and SQL, with expertise in React.js, Node.js, Django, and Flask. 🎖️ Skilled in optimizing system performance and deploying scalable applications using AWS. Strong background in agile methodologies and DevOps practices. 🥅 Committed to delivering high-quality, efficient, and scalable software solutions.