Model-View-Controller Architecture in Rails.

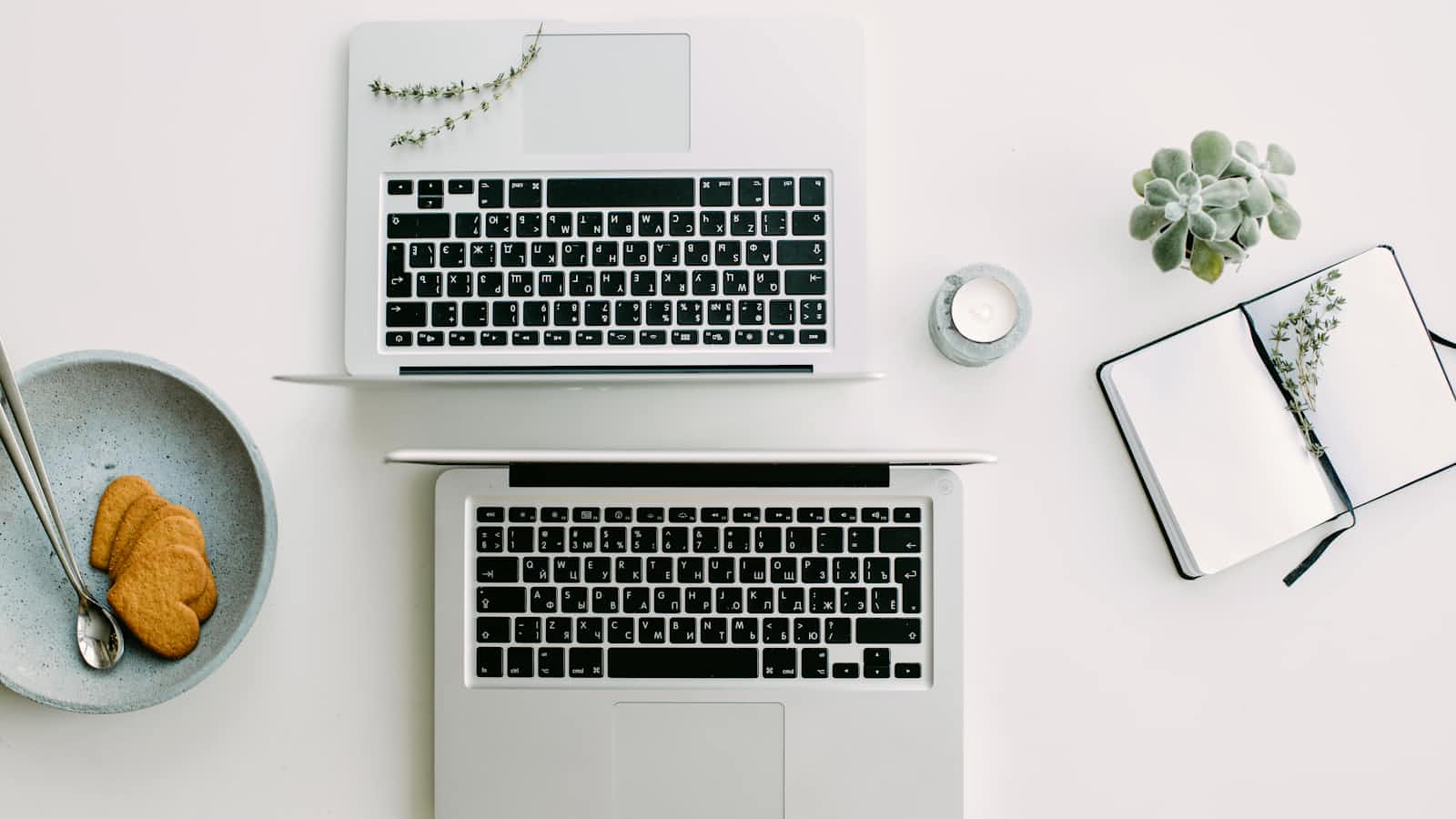
In M-V-C architecture, a software is modeled into three main components: model, views, and controllers
Models -> Acts as the medium for data interaction and representation
Views -> Handles the presentation of information to the user.
Controllers -> Handles logic for the website functionality, it is the glue between models and views.
These three components work seamless together with the router to provide both front-end and back-end website functionality.
Ruby on Rails Way With MVC Architecture.
In Rails the three components are mapped as ActionView(View), ActionController(Controller), and ActiveRecord or ActiveModel (model). A request is made from the user's browser. The request is sent to the server. At t server, the request is first handled by the router. In rails, the router determines which controller action gets to respond based on the url path(route).
This is what you typically see when checking your rails server log, when a request is made from the browser
Started GET "/events" for 127.0.0.1 at 2024-08-11 14:18:30 +0300
Processing by EventsController#index as HTML
Rendering layout layouts/application.html.erb
Rendering events/index.html.erb within layouts/application
Load (0.8ms) SELECT "events".* FROM "events"
↳ app/views/events/index.html.erb:4
Rendered events/index.html.erb within layouts/application (Duration: 3.6ms | Allocations: 501)
User Load (0.4ms) SELECT "users".* FROM "users" WHERE "users"."id" = $1 ORDER BY "users"."id" ASC LIMIT $2 [["id", 1], ["LIMIT", 1]]
↳ app/views/layouts/application.html.erb:17
Rendered layout layouts/application.html.erb (Duration: 13.0ms | Allocations: 2669)
Completed 200 OK in 15ms (Views: 12.8ms | ActiveRecord: 1.1ms | Allocations: 2925)
Requests gets passed to the router (router.rb). The router determines the controller action appropriate for this case EventsController#index
. A controller is responsible for handling the logic for processing user requests, interacting with the database, and rendering the appropriate views. Using the above example, the browser sends a GET request to the server for the events
.
# ../app/contollers/events_controller.rb
class EventsController < ApplicationController
def index
@events = Event.all
end
end
Rails uses .erb
templates for its views, the appropriate view based on the controller action is rendered, in this case events/index.html.erb
- index page for our events controller and layouts/application.html.erb
- this page generally specifies the general structure of the website.
# ../app/views/index.html.erb
<h1>Community Events</h1>
<ul>
<% @events.each do |event| %>
<li>
<%= link_to "#{event.title}", event %>
</li>
<% end %>
</ul>
<%= link_to "Create An Event", new_event_path %>
Events objects from our database through the controller get loaded into our page at this point. ActiveRecord (Model) is responsible for interaction between the database and our rails application, it maps our ruby code into SQL like statement to carry out database transactions. Once all the relevant data is populated in our page, a response is sent back with a complete information.
In Rails, the model sits between our rails code and the database. The model often is left to do the heavy lifting (fat model) concerning the data that goes into the the database as well as those retrieved. My scenerio doesn't allow me to talk much about it. I will get to talk much about it in an upcoming blog.
References
Subscribe to my newsletter
Read articles from Gaetano Osur directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
