Setting up a Local Server and Making an RPC Call: A Step-by-Step Guide (Using Python)
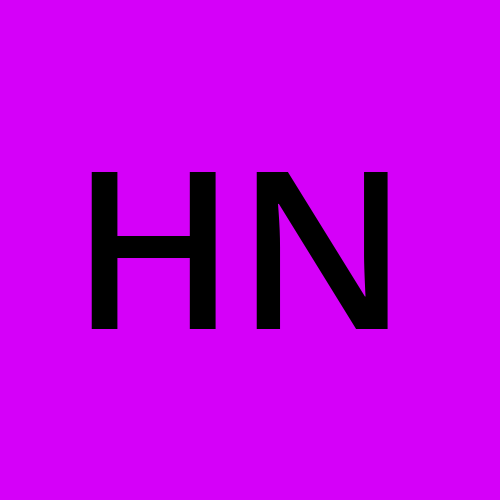
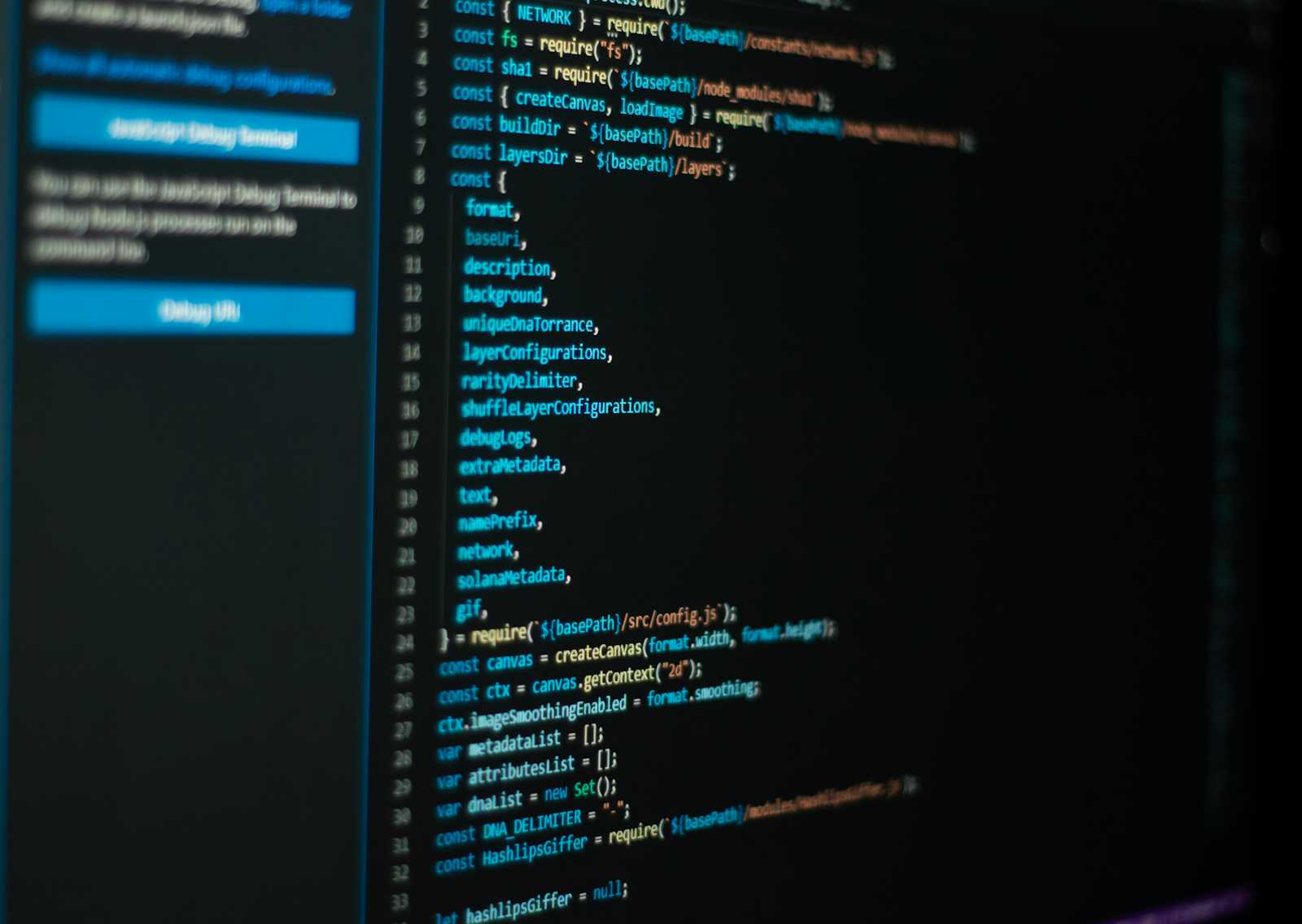
Introduction
This guide will walk you through the process of setting up a local server and making a Remote Procedure Call (RPC) using Python. We will cover everything from installing the required tools to running the server and making the RPC call. By the end of this guide, you will have a working server and client that can communicate with each other through an RPC.
Prerequisites
Before we begin, you'll need to have the following installed on your machine:
Python 3.x
A text editor or an Integrated Development Environment (IDE) like Visual Studio Code
Step 1: Installing Python
If you don't have Python installed on your machine, you can download it from the official Python website (https://www.python.org/downloads/). Follow the instructions provided on the website to install Python for your operating system.
Step 2: Opening Visual Studio Code
Launch Visual Studio Code (VS Code) on your machine.
If you're opening VS Code for the first time, you'll be prompted to select the desired settings and theme. You can either choose the recommended settings or customize them according to your preferences.
Step 3: Creating a New Project Folder
In VS Code, go to the "File" menu and click on "Open Folder" (or "Open..." on Windows).
Choose an appropriate location on your machine where you want to create a new folder for your project.
Create a new folder with a meaningful name (e.g., "rpc-project").
Click "Select Folder" (or "Open" on Windows) to open the newly created folder in VS Code.
Step 4: Creating the Files
In the VS Code explorer pane (usually on the left-hand side), right-click on the project folder and select "New File".
Name the file
interface.py
and press Enter.Repeat step 1, and create two more files named
server.py
andclient.py
.
Step 5: Writing the Code
Now that we have the necessary files, let's write the code for our server and client.
interface.py
This file contains a shared function that both the server and client will use for adding two numbers.
def add_numbers(a, b):
return a + b
This function takes two numbers a
and b
as input and returns their sum.
server.py
This file sets up a server that listens for incoming connections and handles RPC requests.
import socket
import json
from interface import add_numbers
# Server setup
server_socket = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
server_socket.bind(('localhost', 8000))
server_socket.listen(5)
print("Server is listening on localhost:8000")
while True:
client_socket, addr = server_socket.accept()
data = client_socket.recv(1024)
request = json.loads(data.decode())
# Execute the requested function
if request['function'] == 'add_numbers':
result = add_numbers(request['args'][0], request['args'][1])
response = json.dumps({'result': result})
client_socket.sendall(response.encode())
client_socket.close()
Let's break down this code line by line:
import socket
andimport json
import the necessary modules for creating a socket connection and working with JSON data.from interface import add_numbers
imports theadd_numbers
function from theinterface.py
file.server_socket = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
creates a new socket object for the server using theAF_INET
(Internet Address Family) andSOCK_STREAM
(TCP) protocols.server_socket.bind(('localhost', 8000))
binds the server socket to the local host (localhost
) and port8000
.server_socket.listen(5)
sets the maximum number of queued connections to 5.print("Server is listening on localhost:8000")
prints a message indicating that the server is listening onlocalhost:8000
.while True:
starts an infinite loop to accept incoming connections continuously.client_socket, addr = server_socket.accept()
accepts an incoming connection and creates a new socket object (client_socket
) to communicate with the client.data = client_socket.recv(1024)
receives data (up to 1024 bytes) from the client.request = json.loads(data.decode())
decodes the received data from bytes to a string and then loads it as a JSON object.if request['function'] == 'add_numbers':
checks if the requested function is'add_numbers'
.result = add_numbers(request['args'][0], request['args'][1])
calls theadd_numbers
function with the arguments provided in the request and stores the result.response = json.dumps({'result': result})
creates a JSON response object with the result.client_socket.sendall(response.encode())
encodes the response as bytes and sends it back to the client.client_socket.close()
closes the connection with the client.
client.py
This file sets up a client that connects to the server and makes an RPC call.
import socket
import json
from interface import add_numbers
# Client setup
client_socket = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
client_socket.connect(('localhost', 8000))
# Make the RPC call
request = {
'function': 'add_numbers',
'args': [3, 5]
}
data = json.dumps(request)
client_socket.sendall(data.encode())
response = client_socket.recv(1024)
result = json.loads(response.decode())['result']
print(f"Result: {result}")
# Result: 8
client_socket.close()
Let's break down this code line by line:
import socket
andimport json
import the necessary modules for creating a socket connection and working with JSON data.from interface import add_numbers
imports theadd_numbers
function from theinterface.py
file.client_socket = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
creates a new socket object for the client using theAF_INET
(Internet Address Family) andSOCK_STREAM
(TCP) protocols.client_socket.connect(('localhost', 8000))
connects the client socket to the server running onlocalhost:8000
.request = { 'function': 'add_numbers', 'args': [3, 5] }
creates a dictionary representing the RPC request, where'function'
is the name of the function to be called ('add_numbers'
), and'args'
is a list containing the arguments (3
and5
) to be passed to the function.data = json.dumps(request)
converts the request dictionary into a JSON string.client_socket.sendall(data.encode())
encodes the JSON request as bytes and sends it to the server.response = client_socket.recv(1024)
receives the response from the server (up to 1024 bytes).result = json.loads(response.decode())['result']
decodes the received response from bytes to a string, loads it as a JSON object, and extracts the'result'
value.print(f"Result: {result}")
prints the result of the RPC call.client_socket.close()
closes the connection with the server.
Step 6: Running the Server
Open a new terminal or command prompt window.
Navigate to the project folder containing the
server.py
file using thecd
command (e.g.,cd /path/to/rpc-project
).Run the server by executing the following command:
python server.py
You should see the message "Server is listening on localhost:8000" printed in the terminal, indicating that the server is up and running.
Step 7: Running the Client
Open a new terminal or command prompt window.
Navigate to the project folder containing the
client.py
file using thecd
command (e.g.,cd /path/to/rpc-project
).Run the client by executing the following command:
python client.py
You should see the result of the RPC call printed in the terminal (e.g., "Result: 8").
Congratulations! You have successfully set up a local server, made an RPC call, and received the expected result.
Conclusion
In this guide, we covered the steps required to set up a local server and make an RPC call using Python. We explained the code line by line, ensuring that even someone with no prior programming experience can understand and follow along. By completing this guide, you have gained hands-on experience in creating a client-server application and making remote procedure calls.
Subscribe to my newsletter
Read articles from Harshit Nagpal directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
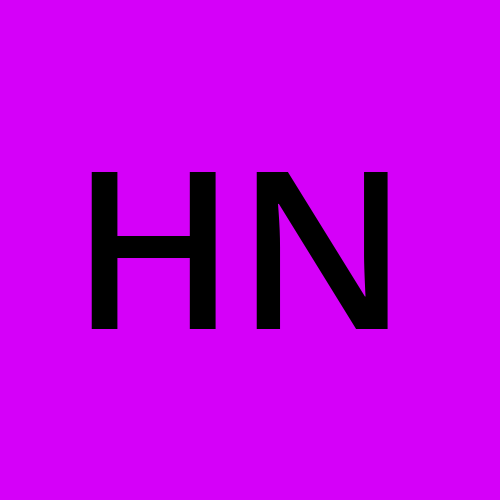