String Class in Java

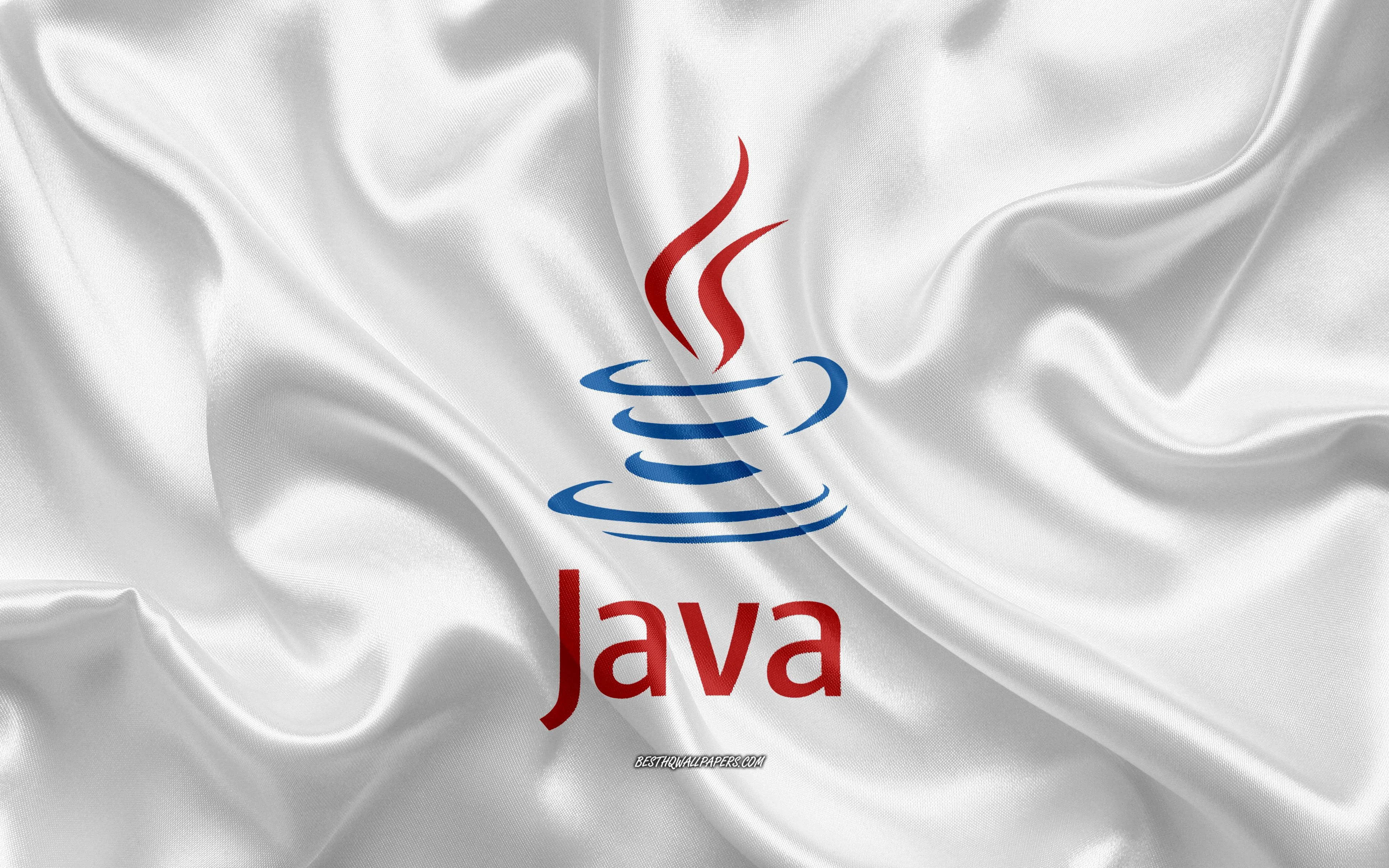
What is a String?
Let's start with the basics. In Java, a string is essentially a sequence of characters. Think of it as a collection of letters, numbers, or symbols enclosed within double quotes. For instance, "Hello, Anubhav" is a string.
The String Class
Behind the scenes, Java represents strings using the String
class. This class belongs to the java.lang
package, which means it's available for use without any additional imports.
Immutability: A Key Concept
One of the most important characteristics of strings in Java is their immutability. This means once a string is created, its content cannot be changed. Any operation that seems to modify a string actually creates a new string object.
String Pool: Where Do Strings Live?
There are two primary places where strings can reside in Java:
String Pool:
When you create a string using string literals (like "Hello"), the JVM checks if this string already exists in the string pool.
If it does, the existing string is reused, saving memory.
If not, a new string is created and added to the pool.
Heap:
- If you create a string using the
new
keyword, it's always placed on the heap, even if it's identical to an existing string in the pool.
- If you create a string using the
Why is this important?
Understanding the string pool and immutability is crucial for efficient memory usage and to avoid unexpected behavior in your Java programs.
Common Methods in the String
Class
Length of a String:
int length = str1.length();
Concatenation (joining two strings):
String str3 = str1 + " How are you?"; // Or using the concat method String str4 = str1.concat(" How are you?");
Character at a specific index:
char ch = str1.charAt(0); // 'H'
Substring (part of the string):
String sub = str1.substring(7, 12); // "World"
Comparing Strings:
Equals method (checks for exact match):
boolean isEqual = str1.equals(str2);
CompareTo method (lexicographical comparison):
int comparison = str1.compareTo(str2);
Checking if a string contains a substring:
boolean contains = str1.contains("World");
Replacing characters or substrings:
String replaced = str1.replace('H', 'h'); // "hello, World!"
Converting to uppercase or lowercase:
String upper = str1.toUpperCase(); // "HELLO, WORLD!" String lower = str1.toLowerCase(); // "hello, world!"
Trimming whitespace from both ends:
String trimmed = str1.trim();
Splitting a string:
String[] words = str1.split(" ");
Conclusion.
This blog post explains how Strings work in Java. It discusses how Strings are stored, their immutability, and the differences between using string literals (which are stored in a special String Pool) and creating Strings with the new
keyword (which are stored in the Heap). Understanding these concepts can help you write better and more efficient Java code.
Stay tuned! Next, we'll explore why strings are immutable and how StringBuffer & StringBuilder come into play.
Subscribe to my newsletter
Read articles from Anubhav Kumar Gupta directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Anubhav Kumar Gupta
Anubhav Kumar Gupta
I'm Anubhav Kumar Gupta, a passionate Data Engineer at Infometry Inc. with expertise in Python, SQL, and Data Engineering. I love solving complex problems, optimizing data pipelines, and integrating back-end technologies.