Why Strings Are Immutable in Java.

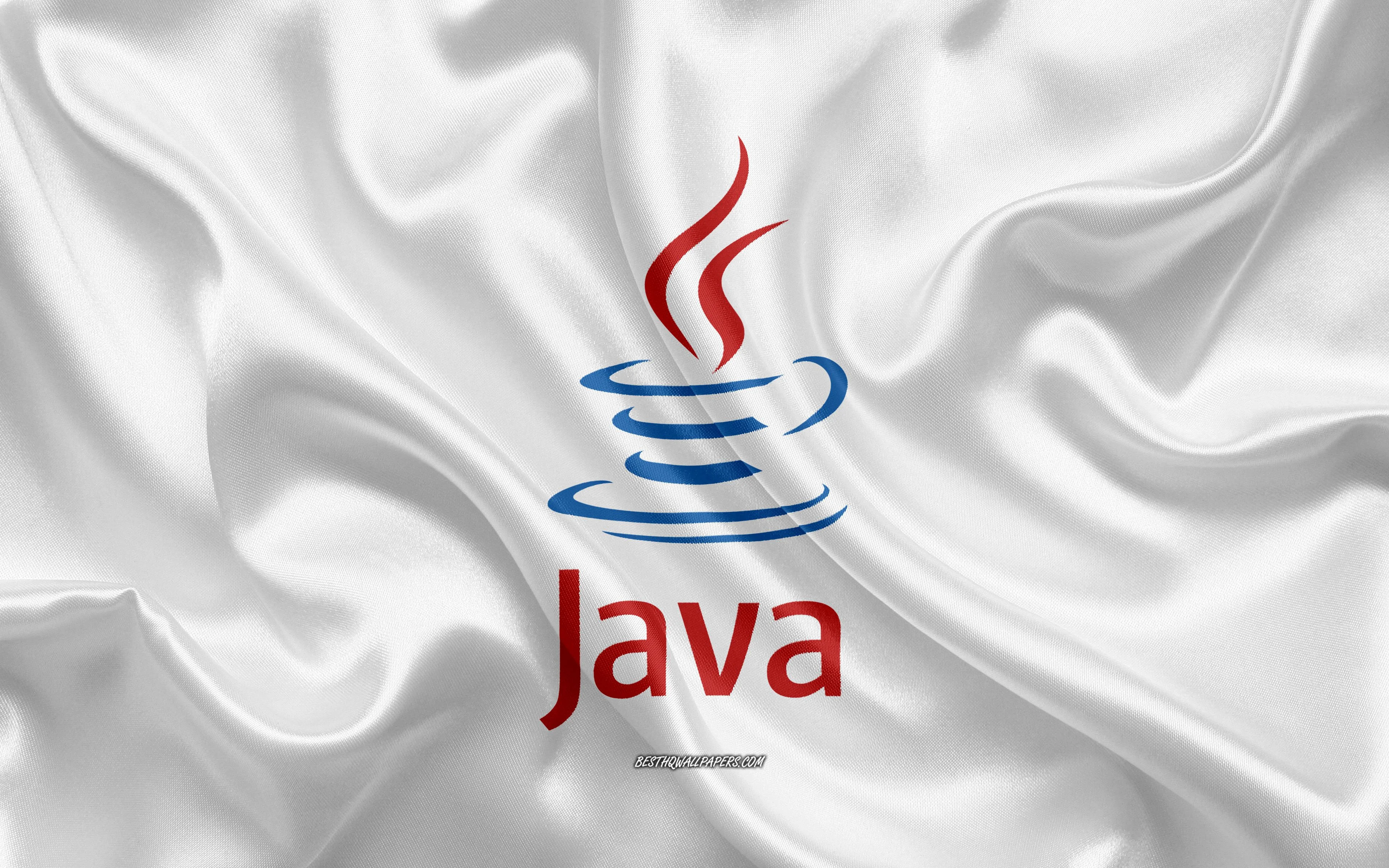
Introduction
So, you've started your Java journey, huh? Welcome to the club! One of the first things you'll encounter is the concept of Strings. And while they might seem simple at first, there's a crucial aspect that sets them apart from other objects: immutability.
What Does Immutable Even Mean?
Imagine a string as a frozen block of ice. Once it's created, you can't change its shape or size. Similarly, a String in Java is unchangeable after it's been created. You can't modify its characters. Trying to do so will actually create a new String object.
Why This Weird Behavior?
You might be wondering why Java designers chose this peculiar approach. Well, there are some solid reasons:
Security:
Strings often hold sensitive information like passwords or URLs.
If they were mutable, a malicious program could potentially alter these values, leading to serious security breaches.
Efficiency:
Java maintains a pool of String objects.
If Strings were mutable, this pool would become complex to manage, impacting performance.
Multithreading:
Since Strings are immutable, they're inherently thread-safe.
Multiple threads can access the same String without worrying about data corruption.
Caching:
- Immutability allows for efficient caching of String objects, improving performance.
Real-World Implications
Understanding immutability is essential for effective Java programming. For instance, when you concatenate Strings using the +
operator, a new String object is created. This might seem inefficient, but it's a trade-off for the benefits mentioned above.
So, How Do I Modify Strings?
You might be wondering, "If Strings are immutable, how do I change them?" The answer lies in using classes like StringBuffer
and StringBuilder
.
These classes provide mutable alternatives for manipulating character sequences.
StringBuffer vs StringBuilder: Which One to Choose?
Understanding the Basics
Both StringBuffer and StringBuilder are used to manipulate character sequences efficiently.
They provide methods for appending, inserting, deleting, and replacing characters within a string. However, their underlying implementations differ significantly.
StringBuffer: The Thread-Safe Option
StringBuffer is synchronized, meaning it's thread-safe. This implies that multiple threads can access and modify a StringBuffer object simultaneously without causing data corruption. While this safety comes at a performance cost, it's essential in multi-threaded environments where string manipulation occurs concurrently.
StringBuilder: The Performance Champion
On the other hand, StringBuilder is not synchronized, making it faster than StringBuffer. If you're working in a single-threaded environment or don't require thread safety, StringBuilder is the preferred choice for optimal performance.
When to Use Which?
StringBuffer: Use StringBuffer when you need to modify strings in a multi-threaded environment.
StringBuilder: Opt for StringBuilder when performance is critical and thread safety is not a concern.
Subscribe to my newsletter
Read articles from Anubhav Kumar Gupta directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Anubhav Kumar Gupta
Anubhav Kumar Gupta
I'm Anubhav Kumar Gupta, a passionate Data Engineer at Infometry Inc. with expertise in Python, SQL, and Data Engineering. I love solving complex problems, optimizing data pipelines, and integrating back-end technologies.