Understanding and Implementing the Singleton Pattern in Python: Key Concepts and Challenges

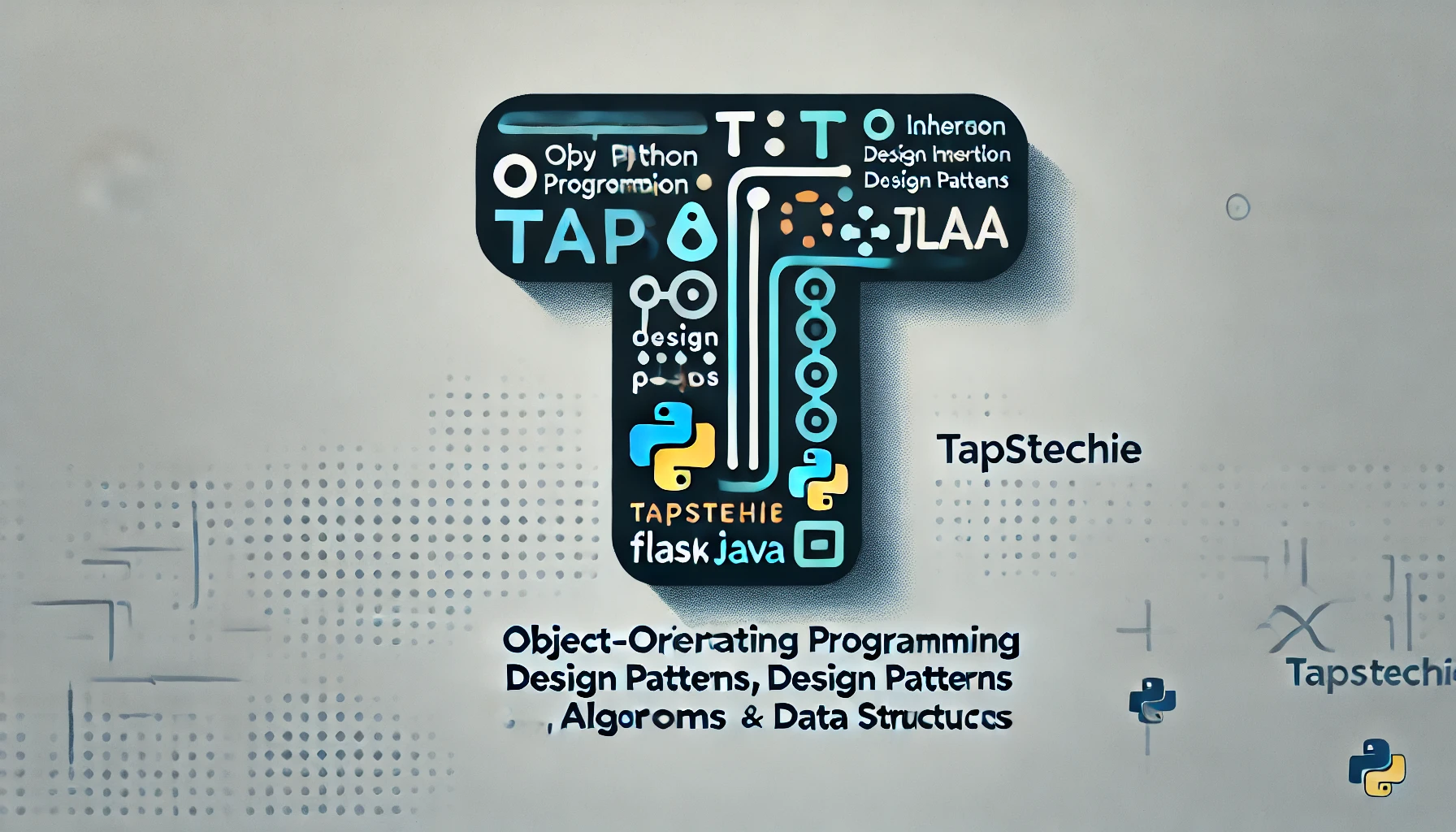
Introduction
The Singleton pattern is one of the most well-known design patterns in software engineering. It ensures that a class has only one instance and provides a global point of access to that instance. This article will guide you through the fundamentals of the Singleton pattern in Python, including its implementation, common pitfalls, advanced variations, and strategies for unit testing.
What is the Singleton Pattern?
The Singleton pattern is a creational design pattern that restricts a class to a single instance while providing a global point of access to this instance. This is particularly useful in scenarios where a single object is needed to coordinate actions, such as in logging, configuration management, or database connections.
Why Use the Singleton Pattern?
Controlled Access to Resources: Ensures that only one instance of a resource-heavy object, such as a database connection, is created.
Global Access Point: Allows consistent access to a shared instance across different parts of an application.
Lazy Initialization: The Singleton instance can be created only when it is needed, saving resources.
Basic Implementation of the Singleton Pattern
In Python, the Singleton pattern is typically implemented by overriding the __new__
method to ensure that only one instance of the class is created.
class Singleton:
_instance = None
def __new__(cls, *args, **kwargs):
if cls._instance is None:
cls._instance = super(Singleton, cls).__new__(cls)
return cls._instance
# Usage
singleton1 = Singleton()
singleton2 = Singleton()
print(singleton1 is singleton2) # Output: True
Advanced Concepts: Variations and Challenges
1. Handling Inheritance
One challenge with Singletons arises when dealing with inheritance. By default, subclasses may end up sharing the Singleton instance of the parent class, which may not be desired. To ensure that each subclass has its own Singleton instance, you can use a dictionary to store instances per class:
class Singleton:
_instances = {}
def __new__(cls, *args, **kwargs):
if cls not in cls._instances:
cls._instances[cls] = super(Singleton, cls).__new__(cls)
return cls._instances[cls]
# Subclass example
class SubSingleton(Singleton):
pass
singleton1 = Singleton()
singleton2 = SubSingleton()
print(singleton1 is singleton2) # Output: False
2. Thread Safety
In a multi-threaded environment, race conditions can lead to multiple instances being created. To prevent this, the Singleton pattern can be made thread-safe using a lock:
import threading
class Singleton:
_instance = None
_lock = threading.Lock()
def __new__(cls, *args, **kwargs):
with cls._lock:
if cls._instance is None:
cls._instance = super(Singleton, cls).__new__(cls)
return cls._instance
3. Borg Pattern (Monostate Pattern)
The Borg pattern is a variation where multiple instances share the same state, rather than ensuring only one instance:
class Borg:
_shared_state = {}
def __init__(self):
self.__dict__ = self._shared_state
# Usage
b1 = Borg()
b2 = Borg()
b1.state = "State 1"
print(b2.state) # Output: "State 1"
Testing Singleton Patterns
Singletons can create challenges in unit testing due to their shared state. Here are strategies to mitigate these issues:
1. Resetting Singleton State
Add a method to reset the Singleton instance between tests to ensure isolation:
class Logger:
_instance = None
def __new__(cls):
if cls._instance is None:
cls._instance = super(Logger, cls).__new__(cls)
cls._instance.logs = []
return cls._instance
@classmethod
def reset_instance(cls):
cls._instance = None
2. Dependency Injection
Refactor your code to use dependency injection, allowing you to inject mock instances for testing instead of relying on the Singleton:
class Application:
def __init__(self, logger=None):
self.logger = logger or Logger()
def run(self):
self.logger.log("Application started")
3. Avoid Singletons in Tests
In some cases, it's best to avoid using Singletons in tests altogether. Use factory methods or mock objects to create instances as needed.
Common Pitfalls of the Singleton Pattern
Global State Issues: Singletons introduce global state, which can make debugging difficult and lead to unintended side effects.
Hidden Dependencies: The Singleton pattern can obscure dependencies, making the code harder to maintain and test.
Limited Scalability: Singletons can become a bottleneck in high-load applications if not carefully managed.
Conclusion
The Singleton pattern is a powerful tool in software design, but it comes with challenges that need to be carefully managed. Understanding how to implement Singletons, handle edge cases like inheritance and thread safety, and mitigate issues in unit testing will help you use this pattern effectively. By mastering these concepts, you can make informed decisions about when and how to apply the Singleton pattern in your projects.
Subscribe to my newsletter
Read articles from Tarun Sharma directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Tarun Sharma
Tarun Sharma
Hi there! I’m Tarun, a Senior Software Engineer with a passion for technology and coding. With experience in Python, Java, and various backend development practices, I’ve spent years honing my skills and working on exciting projects. On this blog, you’ll find insights, tips, and tutorials on topics ranging from object-oriented programming to tech trends and interview prep. My goal is to share valuable knowledge and practical advice to help fellow developers grow and succeed. When I’m not coding, you can find me exploring new tech trends, working on personal projects, or enjoying a good cup of coffee. Thanks for stopping by, and I hope you find my content helpful!