Floating Math Point: The Phantom Menace of Precision

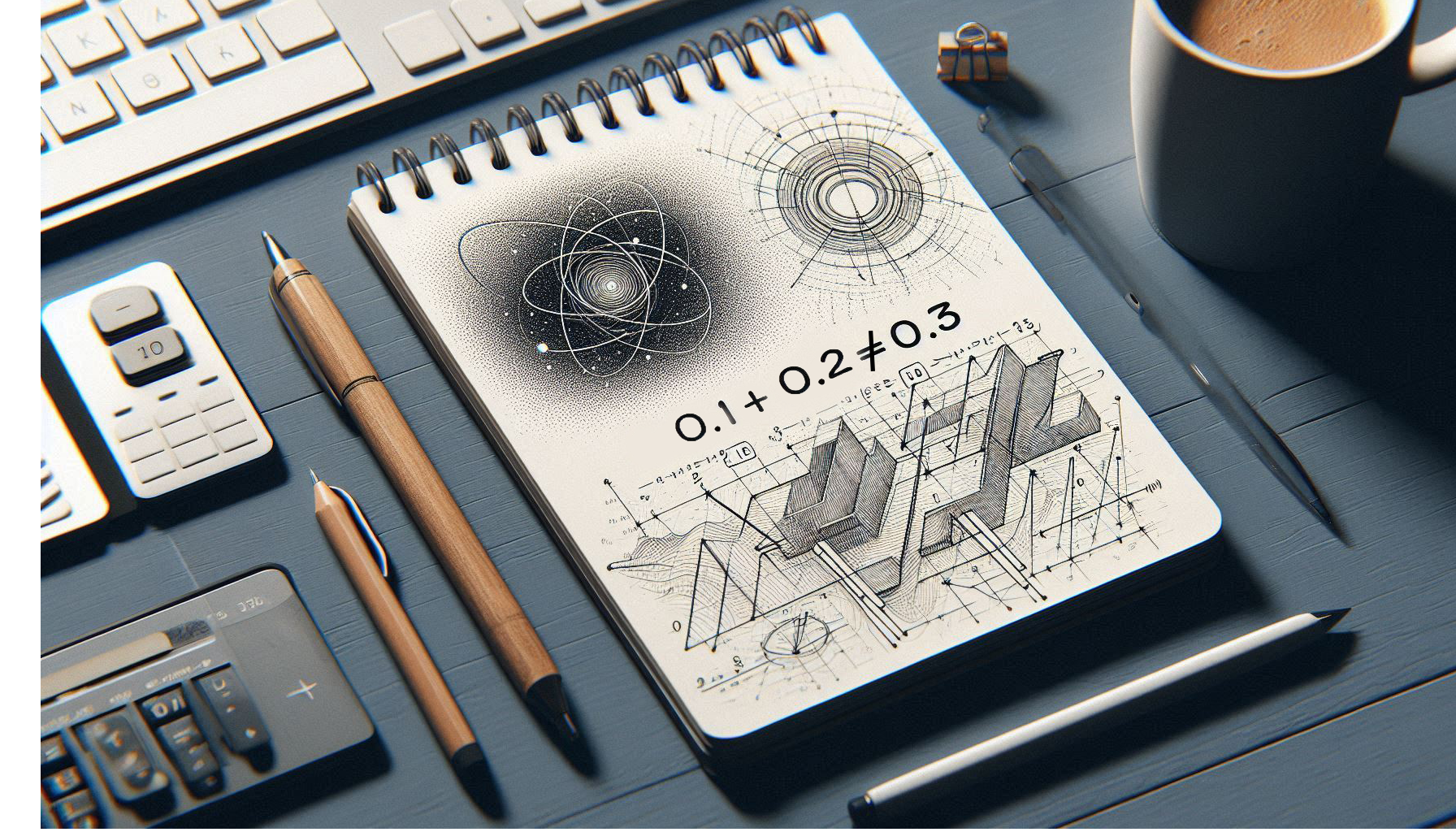
$$0.1 + 0.2 = 0.30000000000000004$$
Hear me out. Try doing 0.1 + 0.2
in your browser console and prepare for the unexpected. Why is the result 0.30000000000000004
? Have I been deceived my entire life? Should I even trust my math teachers anymore? This phenomenon, known as Floating Point Math, is not exclusive to JavaScript but plagues all programming languages. Let's dive into this enigma and uncover why this behavior occurs.
What the Heck? Why Is This Happening?
Alright, let's address the elephant in the room. Why does 0.1 + 0.2
not equal 0.3
? The culprit here is the way computers handle floating-point arithmetic. Computers use a binary system to represent numbers, and floating-point numbers are stored in a format based on the IEEE 754 standard. This standard is a marvel of engineering, but it has its quirks.
The Binary Representation of Floating-Point Numbers
In binary code, some numbers that are simple in decimal (like 0.1) become repeating fractions. For example, 0.1 in binary is an infinite series: 0.00011001100110011...
(and so on). When the computer tries to store this number, it has to truncate it to fit into a finite amount of memory, leading to a tiny error. When you add 0.1
and 0.2
, these small errors accumulate, resulting in the infamous 0.30000000000000004
.
To understand this better, let's delve into the IEEE 754 standard. Floating-point numbers are represented in a form similar to scientific notation, but in binary. They consist of three parts:
Sign bit: Determines whether the number is positive or negative.
Exponent: Determines the scale or magnitude of the number.
Mantissa (or significand): Represents the precision bits of the number.
For example, the number 0.1
is represented in binary as:
Sign bit: 0 (positive)
Exponent: -4 (in binary:
01111011
)Mantissa:
1100110011001100110011001100110011001100110011001101
The mantissa is an approximation because the binary representation of 0.1
is infinitely repeating. This approximation leads to the small error we observe.
Accumulation of Errors
When performing arithmetic operations, these small errors can accumulate. For instance, adding 0.1
and 0.2
involves adding their binary representations. The small errors in each number combine, resulting in a slightly inaccurate result. This is why 0.1 + 0.2
yields 0.30000000000000004
instead of the expected 0.3
.
Is This Happening to All Languages?
Yes, indeed. This floating-point fiasco is not a JavaScript-exclusive party trick. It affects all programming languages that adhere to the IEEE 754 standard, which is virtually all of them. Whether you're coding in Python, C++, Java, or even the venerable FORTRAN, you'll encounter the same issue. The underlying hardware and the IEEE standard are the common denominators here.
Examples from Different Languages
Let's take a look at how this issue manifests in different programming languages:
Python
print(0.1 + 0.2) # Output: 0.30000000000000004
JavaScript
console.log(0.1 + 0.2) # Output: 0.30000000000000004
Java
public class Main {
public static void main(String[] args) {
System.out.println(0.1 + 0.2); // Output: 0.30000000000000004
}
}
C++
#include <iostream>
using namespace std;
int main() {
cout << 0.1 + 0.2 << endl; // Output: 0.30000000000000004
return 0;
}
As you can see, the issue is consistent across different languages due to their reliance on the IEEE 754 standard for floating-point arithmetic.
Is My Language Broken?
No, your language is not broken. It's just that floating-point arithmetic is inherently imprecise due to the limitations of binary representation. Think of it as trying to fit a round peg into a square hole. The peg will go in, but there will be gaps. These gaps are the tiny errors we see in floating-point calculations.
Understanding Limitations
The limitations of floating-point arithmetic stem from the fact that not all decimal numbers can be represented exactly in binary form. This is similar to how the fraction 1/3
cannot be represented exactly in decimal form (it becomes 0.3333
... with repeating threes). The IEEE 754 standard does its best to approximate these numbers, but the approximations are not always perfect.
How Can I Fix It?
Here's the kicker: you can't fix it. Floating-point arithmetic is fundamentally flawed in this regard. However, you can mitigate its effects by being aware of it and using appropriate techniques. For instance, when comparing floating-point numbers, instead of checking for equality, you can check if the numbers are "close enough" within a small tolerance.
Using Tolerance for Comparisons
When comparing floating-point numbers, it's essential to allow for a small margin of error. This can be done using a tolerance value. Here's an example in JavaScript:
function isCloseEnough(a, b, tolerance = 1e-9) {
return Math.abs(a - b) < tolerance;
}
const a = 0.1 + 0.2;
const b = 0.3;
if (isCloseEnough(a, b)) {
console.log("Close enough!");
} else {
console.log("Not close enough!");
}
In this example, isCloseEnough
is a function that compares two numbers with a specified tolerance (default is 1e-9
). The function returns true
if the absolute difference between the two numbers is less than the tolerance, indicating that the numbers are "close enough."
Arbitrary-Precision Libraries
For applications requiring high precision, such as scientific computations or financial calculations, using arbitrary-precision libraries can be a solution. These libraries provide data types that can represent numbers with a high degree of accuracy, avoiding the pitfalls of floating-point arithmetic. Each language has it's own approach but all of them relies in the same principle.
What Happens When Dealing with Money?
Ah, money—the root of all precision problems. When dealing with financial calculations, the tiny errors in floating-point arithmetic can lead to significant discrepancies. Imagine a bank miscalculating interest rates due to floating-point errors. Chaos would ensue!
Using Integers for Money
One common approach to avoid floating-point errors in financial applications is to use integers to represent monetary values. For example, instead of representing dollars and cents as floating-point numbers, you can represent them as integers (e.g., 100 cents instead of 1 dollar).
Example in Python
price_in_cents = 1000 # $10.00
tax_rate = 0.075 # 7.5%
tax_in_cents = int(price_in_cents * tax_rate)
total_price_in_cents = price_in_cents + tax_in_cents
print(f"Total price: ${total_price_in_cents / 100:.2f}")
This approach ensures that calculations are precise, as integer arithmetic does not suffer from the same issues as floating-point arithmetic.
Using decimal.js
for Decimal Precision in JavaScript
To handle high-precision decimal arithmetic in JavaScript, you can use the decimal.js
library. If you are using Node.js, you can install it using your preferred package manager.
// If you are using Node.js, first require the library
// const Decimal = require('decimal.js');
// If you are in a browser environment, the library will be available globally as Decimal
const a = new Decimal('0.1').plus(new Decimal('0.2'));
const b = new Decimal('0.3');
if (a.equals(b)) {
console.log("Exact match!");
} else {
console.log("Mismatch!");
}
In this example, Decimal
is a class provided by decimal.js
that allows for high-precision arithmetic operations. We create instances of Decimal
for the numbers 0.1
and 0.2
, and then use the plus
method to add them. Finally, we use the equals
method to compare the result with 0.3
.
Java's BigDecimal
Class
We all love Java because looks like that special friend of ours.
In Java, the built-in BigDecimal
class (part of the standard) provides arbitrary-precision decimal arithmetic, making it suitable for financial calculations:
import java.math.BigDecimal;
public class Main {
public static void main(String[] args) {
BigDecimal a = new BigDecimal("0.1");
BigDecimal b = new BigDecimal("0.2");
BigDecimal c = new BigDecimal("0.3");
if (a.add(b).compareTo(c) == 0) {
System.out.println("Exact match!");
} else {
System.out.println("Mismatch!");
}
}
}
The BigDecimal
class ensures that 0.1 + 0.2
equals 0.3
exactly, avoiding the pitfalls of floating-point arithmetic.
The Floating-Point Hall of Shame
Unexpected Results in Real-World Applications
Floating-point errors are not just academic curiosities; they have real-world implications. One infamous example is the Patriot missile failure during the Gulf War in 1991. A small error in the floating-point arithmetic used to track the missile's target led to a significant miscalculation, resulting in the failure to intercept an incoming missile and the tragic loss of 28 lives.
The Tale of the Pentium FDIV Bug
In 1994, Intel's Pentium processor was found to have a flaw in its floating-point division (FDIV) instruction. This bug caused incorrect results for certain division operations, leading to a massive recall and costing Intel nearly $500 million. The incident highlighted the importance of accuracy in floating-point arithmetic and the potential consequences of even minor errors.
Floating-Point Arithmetic in Machine Learning
The Impact on Training Models
Machine learning algorithms often involve extensive numerical computations, and floating-point errors can propagate through these calculations, affecting the accuracy of the models. For instance, when training neural networks, the small errors in floating-point arithmetic can accumulate over many iterations, potentially leading to suboptimal models.
Mitigating Floating-Point Errors in ML
To mitigate the impact of floating-point errors in machine learning, researchers and practitioners use techniques such as:
Normalization: Scaling input data to a smaller range to reduce the risk of large numerical errors.
Higher Precision: Using higher-precision floating-point formats (e.g., 64-bit instead of 32-bit) to reduce the magnitude of errors.
Regularization: Adding constraints to the model to prevent overfitting and reduce the sensitivity to numerical errors.
The Future of Floating-Point Arithmetic
Emerging Standards and Technologies
As computational demands increase, new standards and technologies are being developed to address the limitations of floating-point arithmetic. For example, the IEEE 754-2008 standard introduced new formats and operations to improve precision and performance. Additionally, emerging technologies such as quantum computing may offer alternative approaches to numerical computations, potentially reducing the reliance on traditional floating-point arithmetic.
Research Directions
Researchers are exploring various approaches to improve the accuracy and reliability of numerical computations. Some promising directions include:
Interval Arithmetic: Representing numbers as intervals with upper and lower bounds to capture the uncertainty in calculations.
Unum Arithmetic: A novel approach proposed by John Gustafson that combines the benefits of floating-point and fixed-point arithmetic to achieve higher precision and efficiency.
Conclusion
In conclusion, the floating-point math conundrum is a fascinating quirk of computer science that affects all programming languages. While it might seem like a betrayal of basic arithmetic, it's a consequence of the limitations of binary representation and the IEEE 754 standard. By understanding and acknowledging these limitations, you can write more robust and reliable code. So, next time you see 0.30000000000000004
, don't panic. Embrace it as a reminder of the beautifully imperfect world of floating-point arithmetic.
Subscribe to my newsletter
Read articles from Ferran Buireu directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Ferran Buireu
Ferran Buireu
I’m a restless developer with 10+ years of full-time experience. I enjoy new challenges and varied projects that keep me on my toes. I draw inspiration from my Master's degree background in geophysics to break down complex problems and find clean and effective solutions. I’ve been praised before for going the extra mile and take pride in delivering neat code that is optimized for maximum readability and scalability. I thrive in the workplace when I can find a dynamic environment that fosters teamwork and encourages me to learn and grow every day both personally and professionally. My passions, besides programming, are playing the guitar, playing football and training for my next marathon.