[PYTHON] Add or Remove Image Background in PowerPoint Slides without Effort

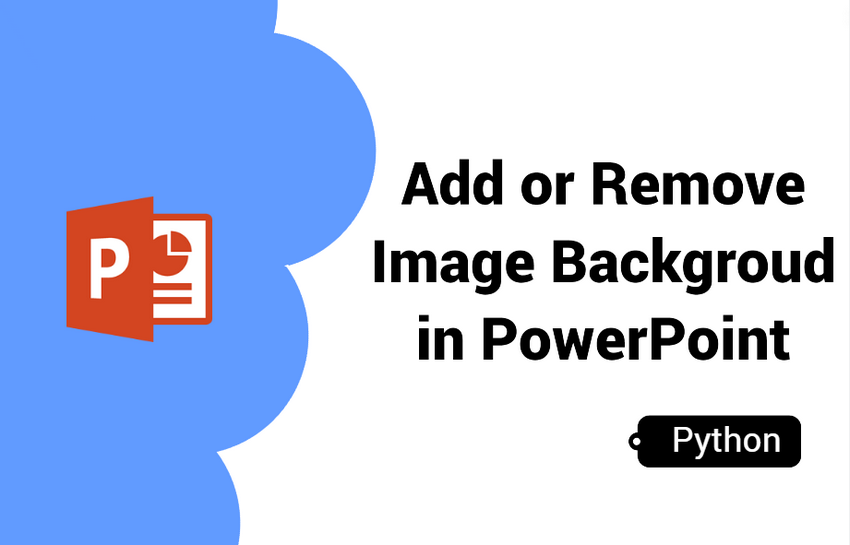
When handling PowerPoint presentations, people always encounter situations where they need to add or remove a background image in PowerPoint. A well-chosen image background can give your slides a more cohesive look and enhance their visual impact, making it easier to capture and maintain the audience's attention. And Python offers powerful tools to finish tasks effortlessly.
Today’s blog will introduce how to add or remove image backgrounds in PowerPoint with Python, including step-by-step guides and code examples. Read on!
Prepare for task
In this guide, we need to use Spire.Presentation for Python to add background images in PowerPoint. It is a powerful Python library that helps you accomplish many PowerPoint presentation tasks, including removing a background image in PowerPoint.
You can install Spire.Presentation for Python from PyPI using the pip command below:
pip install Spire.Presentation
If you have already installed it and would like to upgrade it to the newest version, please use the following command:
pip install - upgrade Spire.Presenation
Add Background Images in PowerPoint with Python
When creating eye-catching PowerPoint presentations, adding a background image in PowerPoint can significantly enhance the visual appeal. You can automate this process with Python to increase efficiency and reduce manual errors.
In this part, we will guide you through how to add background images in PowerPoint with Python.
Steps to add an image to the background in PowerPoint:
Create an object of Presentation and load the document to be operated from the disk.
Get the slide that needs background with Presentation.Slides[] method and using ISlide.SlideBackground property to access its background.
Set the background type as
Custom
then set its fill type to picture fill.Add the background image to the stream for the next step.
Add the background image in PowerPoint, and set the fill type to stretch to make it fit the size of the slide.
Save the resulting presentation by calling Presentation.SaveToFile() method.
The following is a code example of adding a background image to the fourth slide:
from spire.presentation import *
# Create a Presentation object
ppt = Presentation()
# Load a PowerPoint presentation
ppt.LoadFromFile("presentation.pptx")
# Get the fourth slide
slide = ppt.Slides[3]
# Access the background of the slide
background = slide.SlideBackground
# Set the type of the slide's background as a custom type
background.Type = BackgroundType.Custom
# Set the fill mode of the slide's background as a picture fill
background.Fill.FillType = FillFormatType.Picture
# Add an image to the image collection of the presentation
stream = Stream("bg.jpg")
imageData = ppt.Images.AppendStream(stream)
# Set the image as the slide's background
background.Fill.PictureFill.FillType = PictureFillType.Stretch
background.Fill.PictureFill.Picture.EmbedImage = imageData
# Save the result presentation
ppt.SaveToFile("imagebackground.pptx", FileFormat.Pptx2013)
# Release resources
ppt.Dispose()
Stretch
alters the aspect ratio of the original image to cover the entire slide. If you prefer to maintain the image's original size, you can use the Tile
method for filling.You may need >> How to Add or Remove Images to Slides
How to Set Background Color in PowerPoint with Python [Solide & Gradient]
Sometimes, you might find that background images are too distracting and take away from your audience's focus. However, setting a background is still important for enhancing the slide's visual appeal. In such cases, a color background might be the best option.
This section will demonstrate how to set the background color in PowerPoint using Python, complete with step-by-step guides and examples.
Set Background Color in PowerPoint Slide: Solid
To set the background color of a PowerPoint slide, you still need to load the presentation, get the desired slide, and use the ISlide.SlideBackground property to retrieve the background. After that, you can customize the slide background as you want.
Steps to add a background color in PowerPoint:
Create an object for the Presentation class and read the document according to the file path with Presentation.LoadFromFile() method.
Get the slide and access the background.
Set the background type as
Custom
.Set the fill format type to solid, and set the background color in the PowerPoint slide as you need.
Store the modified document with Presentation.SaveToFile() method and release the resource.
Here is an example of adding Alice Blue as the color background of the 4th slide:
from spire.presentation import *
# Create a Presentation object
ppt = Presentation()
# Load a PowerPoint presentation
ppt.LoadFromFile("presentation.pptx")
# Get the fourth slide
slide = ppt.Slides[3]
# Access the background of the slide
background = slide.SlideBackground
# Set the type of the slide's background as a custom type
background.Type = BackgroundType.Custom
# Set the fill mode of the slide's background as a solid fill
background.Fill.FillType = FillFormatType.Solid
# Set a color for the slide's background
background.Fill.SolidColor.Color = Color.get_AliceBlue()
# Save the result presentation
ppt.SaveToFile("Solidbackground.pptx", FileFormat.Pptx2013)
# Release resources
ppt.Dispose()
Set Background Color in PowerPoint Slide: Gradient
Setting up a gradient background is similar to setting a solid color initially, but it diverges from the "FillFormatType" step onwards. The detailed steps are as follows:
InstaInstantiate a Presentation object and open the document from the disk with Presentation.LoadFromFile() method.
Get the slide to be modified and access the background.
Set the background type as
Custom
.Select the fill format type as gradient, then set the gradient stops and colors.
Define the shape type and angle of the gradient background.
Save the modified presentation with Presentation.SaveToFile() method and release the resource.
The example shows how to set Alice Blue and Dark Blue as the gradient color background:
from spire.presentation import *
# Create a Presentation object
ppt = Presentation()
# Load a PowerPoint presentation
ppt.LoadFromFile("presentation.pptx")
# Get the fourth slide
slide = ppt.Slides[3]
# Access the background of the slide
background = slide.SlideBackground
# Set the type of the slide's background as a custom type
background.Type = BackgroundType.Custom
# Set the fill mode of the slide's background as a gradient fill
background.Fill.FillType = FillFormatType.Gradient
# Set gradient stops and colors
background.Fill.Gradient.GradientStops.AppendByColor(0.1, Color.get_AliceBlue())
background.Fill.Gradient.GradientStops.AppendByColor(0.7, Color.get_DarkBlue())
# Set the shape type of the gradient fill
background.Fill.Gradient.GradientShape = GradientShapeType.Linear
# Set the angle of the gradient fill
background.Fill.Gradient.LinearGradientFill.Angle = 45
# Save the result presentation
ppt.SaveToFile("Gradientbackground.pptx", FileFormat.Pptx2013)
# Release resources
ppt.Dispose()
How to Remove a Background in PowerPoint with Python [Image & Color]
After learning how to add background images and color in PowerPoint, it’s time to check out how to remove backgrounds in PowerPoint.
When the current image or color background doesn't align with the overall style of your PowerPoint presentation, it's time to remove it. The process for removing picture and color backgrounds in PowerPoint is identical, with the key step being to set the background type to none. For detailed instructions, please refer to the steps below.
How to remove a background in PowerPoint:
Create an object of the Presentation class and load the document.
Get the slide that needs to be modified with Presentation.Slides[] method.
Define the background type to
none
.Save the resulting document with Presentation.SaveToFile() method and release the resource.
Here is an example of removing the background on the 4th slide in PowerPoint:
from spire.presentation import *
# Create a Presentation document object
presentation = Presentation()
# Read the presentation document from file
presentation.LoadFromFile(“imagebackground.pptx”)
# Get the fourth slide
slide = presentation.Slides[3]
# Remove the background image by setting FillType to Solid with a transparent color
slide.SlideBackground.Type = BackgroundType.none
# Save the modified presentation
presentation.SaveToFile(“RemoveBackground_out.pptx”, FileFormat.Pptx2010)
# Release resource
presentation.Dispose()
The Conclusion
This blog demonstrates how to add or remove background in PowerPoint using Python. Each section offers detailed steps and code examples to enhance your understanding of how Spire.Presentation completes these tasks. We hope you find it helpful!
Subscribe to my newsletter
Read articles from Casie Liu directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
