Identifying Unutilized Elastic Load Balancers in AWS to Save Costs Using PowerShell

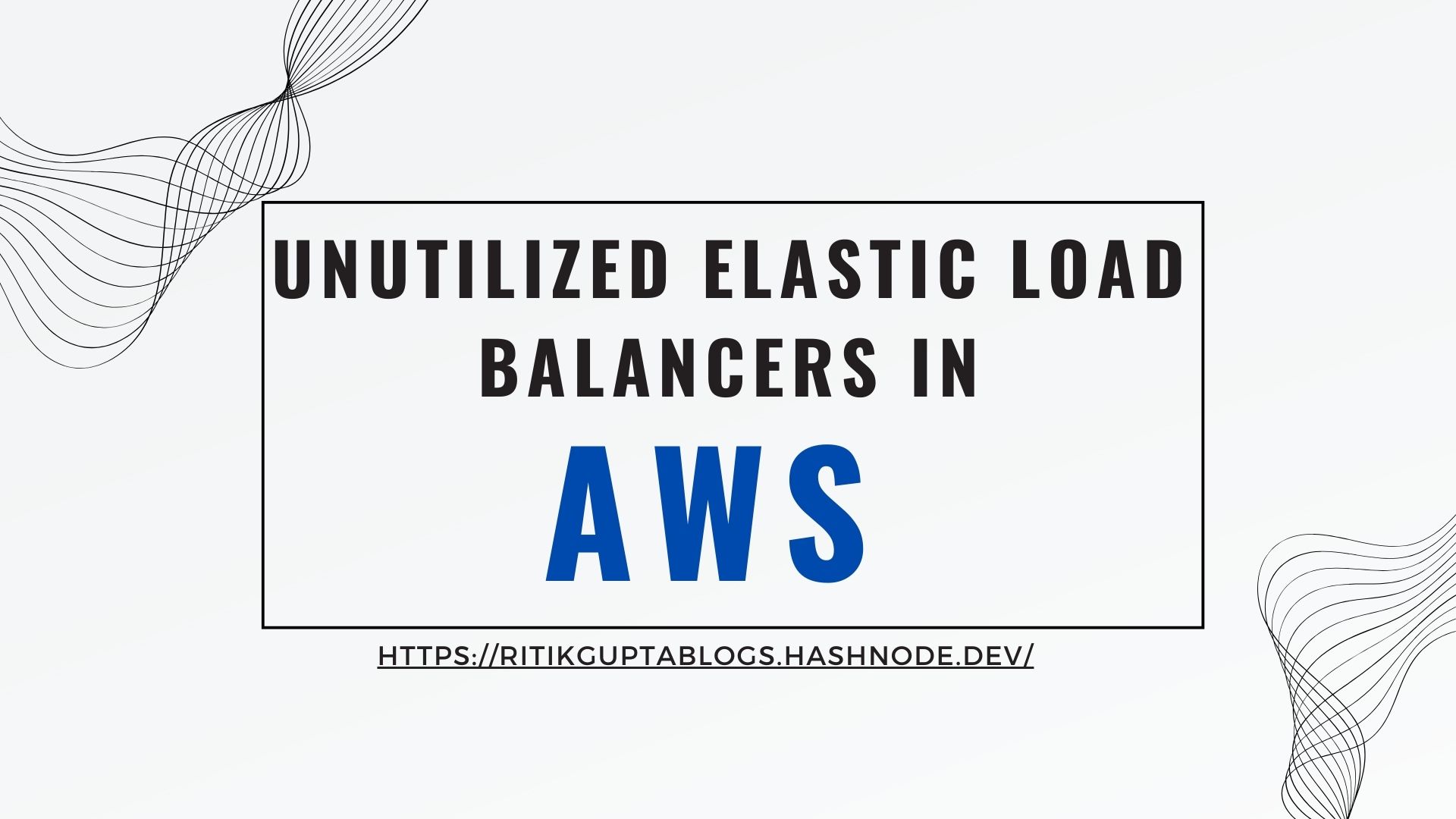
In the dynamic world of cloud computing, managing costs is a key priority for any organization. AWS provides powerful services that can scale and adapt to meet various business needs. However, as these services evolve and infrastructure changes, resources can become orphaned—meaning they no longer serve any purpose but continue to incur costs. One such resource is the Elastic Load Balancer (ELB).
In this blog, the first in a series on AWS orphaned resources, we’ll focus on identifying and removing unutilized elastic load balancers (ELBs) to help you reduce unnecessary expenses.
What is an Elastic Load Balancer?
Elastic Load Balancer is a key AWS service that automatically distributes incoming application traffic across multiple targets, such as Amazon EC2 instances, containers, and IP addresses. It improves application availability and fault tolerance by balancing load across multiple resources. However, as applications and environments change, some ELBs can become detached from their target resources or are left behind after the resources they were supporting are terminated.
Why Orphaned ELBs are Costly
Even if an ELB is no longer associated with any active resources, it still incurs charges. AWS bills you for each hour or partial hour that the ELB is running, along with data processing fees. Therefore, identifying and deleting orphaned ELBs can help you optimize your AWS costs.
How to Identify Orphaned ELBs
Identifying orphaned ELBs involves checking whether the ELB is associated with any active targets or resources. Here’s a step-by-step guide:
Use AWS Console:
Navigate to the Elastic Load Balancing console.
Review the list of ELBs and check their associated instances, targets, or services.
If an ELB has no associated targets or instances, it may be orphaned.
Using AWS CLI: You can use AWS CLI commands to list ELBs and check their status. Here's a basic approach:
aws elb describe-load-balancers --query "LoadBalancerDescriptions[*].[LoadBalancerName,Instances]" --output table
This command lists all the ELBs and their associated instances. An ELB with no instances listed is likely orphaned.
Using AWS SDKs (e.g., Boto3 for Python): If you prefer automation, you can use AWS SDKs to script the process. For example, in Python using Boto3:
import boto3 client = boto3.client('elb') elbs = client.describe_load_balancers() for elb in elbs['LoadBalancerDescriptions']: if not elb['Instances']: print(f"Orphaned ELB found: {elb['LoadBalancerName']}")
This script will print the names of any ELBs that have no associated instances.
CloudWatch Alarms: You can also create CloudWatch alarms to monitor ELB activity. If an ELB has no traffic over a certain period, it might be worth investigating to see if it’s still needed.
Deleting Orphaned ELBs
Once you’ve identified orphaned ELBs, the next step is to delete them to stop incurring costs. This can be done easily via the AWS Management Console, CLI, or SDKs.
Via Console:
Select the orphaned ELB.
Choose Actions > Delete.
Confirm the deletion.
Via CLI:
aws elb delete-load-balancer --load-balancer-name <your-elb-name>
Via SDK: In Boto3:
client.delete_load_balancer(LoadBalancerName='your-elb-name')
Best Practices for Preventing Orphaned Resources
To minimize the occurrence of orphaned resources, consider the following practices:
Regular Audits: Schedule regular audits of your AWS resources to identify and clean up unused or orphaned resources.
Automation: Use AWS Config rules and automation tools like AWS Lambda to automatically detect and clean up orphaned resources.
Powershell Script to Identify the unutilized Load Balancer
PowerShell script that identifies orphaned Classic Load Balancers (CLBs) and Application/Network Load Balancers (ALBs/NLBs) using the AWS Tools for PowerShell
# Import AWS Tools for PowerShell module
Import-Module AWSPowerShell
# Set AWS credentials and region if not already set
Initialize-AWSDefaultConfiguration -ProfileName 'your-aws-profile' -Region 'your-region'
# Initialize arrays to hold orphaned ELBs
$orphanedClassicElbs = @()
$orphanedAlbsNlBs = @()
# Check Classic Load Balancers (CLBs)
$classicElbs = Get-ELBLoadBalancer
foreach ($elb in $classicElbs) {
$instances = (Get-ELBInstance -LoadBalancerName $elb.LoadBalancerName).Instances
if ($instances.Count -eq 0) {
$orphanedClassicElbs += $elb.LoadBalancerName
}
}
# Check Application/Network Load Balancers (ALBs/NLBs)
$albs = Get-ELB2LoadBalancer
foreach ($alb in $albs) {
$targetGroups = Get-ELB2TargetGroup -LoadBalancerArn $alb.LoadBalancerArn
$orphaned = $true
foreach ($tg in $targetGroups) {
$targets = Get-ELB2TargetHealth -TargetGroupArn $tg.TargetGroupArn
if ($targets.TargetHealthDescriptions.Count -gt 0) {
$orphaned = $false
break
}
}
if ($orphaned) {
$orphanedAlbsNlBs += $alb.LoadBalancerName
}
}
# Print the list of orphaned ELBs
Write-Output "Orphaned Classic ELBs:"
$orphanedClassicElbs
Write-Output "Orphaned ALBs/NLBs:"
$orphanedAlbsNlBs
Explanation:
Classic Load Balancers (CLBs):
Retrieve all CLBs using
Get-ELBLoadBalancer
.Check each CLB for associated instances using
Get-ELBInstance
.If no instances are found, add the CLB to the
$orphanedClassicElbs
array.
Application/Network Load Balancers (ALBs/NLBs):
Retrieve all ALBs and NLBs using
Get-ELB2LoadBalancer
.Check each load balancer's target groups using
Get-ELB2TargetGroup
.For each target group, check for associated targets using
Get-ELB2TargetHealth
.If no targets are found for all target groups, add the ALB/NLB to the
$orphanedAlbsNlBs
array.
Print the lists of orphaned ELBs:
- The script prints the names of orphaned Classic ELBs and orphaned ALBs/NLBs.
Conclusion
Orphaned Elastic Load Balancers can silently inflate your AWS bill, but with a bit of regular maintenance, you can easily identify and remove them. This not only helps in cost optimization but also keeps your AWS environment clean and efficient. In the next blog of this series, we’ll explore another commonly orphaned resource and how to manage it effectively.
Stay tuned!
By focusing on these orphaned resources and cleaning them up regularly, you can significantly reduce unnecessary costs in your AWS environment. Keep an eye out for the next post in this series!
Thanks for reading! I hope you understood these concepts and learned something.
If you have any queries, feel free to reach out to me on LinkedIn.
Subscribe to my newsletter
Read articles from Ritik Gupta directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Ritik Gupta
Ritik Gupta
Hi there! 👋 I'm Ritik Gupta, a passionate tech enthusiast and lifelong learner dedicated to exploring the vast world of technology. From untangling complex data structures and designing robust system architectures to navigating the dynamic landscape of DevOps, I aim to make challenging concepts easy to understand. With hands-on experience in building scalable solutions and optimizing workflows, I share insights from my journey through coding, problem-solving, and system design. Whether you're here for interview tips, tutorials, or my take on real-world tech challenges, you're in the right place! When I’m not blogging or coding, you can find me contributing to open-source projects, exploring new tools, or sipping on a good cup of coffee ☕. Let’s learn, grow, and innovate together!