How JavaScript Execution Context Works: A Simple Guide

Table of contents
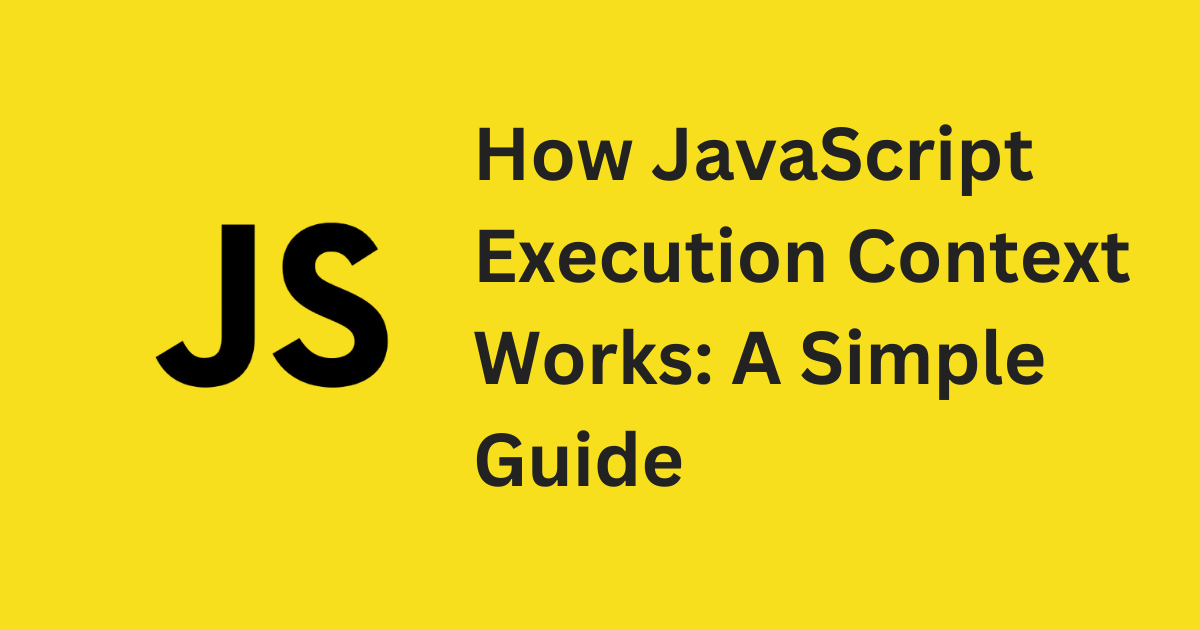
Introduction
JavaScript is one of the most influential and flexible programming languages in existence, thus forming the base for modern web development. Being among the core technologies of the web—like HTML and CSS—this language helps developers create rich, interactive user experiences.
Execution Context is a very important concept that governs the environment in which your code will be executed. It defines which variables, objects, and functions are accessible at any point in the code. In this way, execution context is necessary for managing scope, avoiding variable hoisting and memory usage optimization.
Moreover, understanding the way JavaScript runs code in a cycle from parsing and compilation to the actual execution phase enables a developer to write more predictable and optimized code. It becomes instrumental in the debugging phase and also helps in improving the performance of the code.
Execution Context
"Everything in JavaScript happens inside the execution context." For one to understand how exactly the JavaScript operates, they must have an idea of what an execution context is. Imagine the execution context like a big box containing two important components inside it:
(a) Memory Component (Variable Environment): This is where all variables and functions are stored as key-value pairs. For example, if you declare a variable, let a = 10 , it is stored in memory with 'a' as the key and 10 as its value. Equally, if you declare a function, say, function hello() { console.log("Hello, JavaScript"); }, this entire body of the function is also stored within memory with 'hello' as the key. This component basically acts as the record keeper for all the things that need to be remembered during the execution of your code.
(b) Code Component: This is where the actual execution of your code takes place. JavaScript, in executing your code, goes through the instructions line by line and executes each one in the sequence they are written. It's basically like the "engine" that keeps your program flowing.
How JavaScript Code is Executed ?
lets understand this with the help of an example.....
let n = 2;
function sum(num) {
let ans = num + num;
return ans;
}
let sum2 = sum(n);
Memory Creation Phase (Also known as the "Creation Phase"): It's during this phase that the JavaScript engine goes through the entire code but doesn't execute it; instead, it puts all variables and functions in memory. Let's walk through it step by step here:
Step 1: Let's start with the very first statement a variable declaration let n. The engine creates memory for n and initializes it with undefined.
Step 2: The engine encounters the sum function declaration. Whenever a function is declared in JavaScript, the entire function is stored in memory. So here too, the function sum gets stored with its entire code block itself.
Step 3: Declaration of variables let sum2 encountered. Similar to n, sum2 get memory allocations and get initialized with undefined.
n: undefined
sum: function sum(num) {
let ans = num + num;
return ans;
}
sum2: undefined
Code Execution Phase (Also known as the "Execution Phase"): In this phase, the code gets executed line after line and the values get assigned to variables. Here is what happens:
Step 4: The engine executes let n = 2;. Variable n will carry the value 2.
Memory environment:
n: 2
sum: function sum(num)
{
let ans = num + num;
return ans;
}
sum2: undefined
Step 5: Again, the engine reaches the definition of the sum function. Since it is already stored within memory, nothing happens.
Step 6: The engine executes let sum2 = sum(n);. It calls the sum function passing n holding the value 2 as its argument.
Inside sum function:
A new execution context for this function call is created.
Declares num and initializes it with 2.
let ans = num + num;
executes 2 + 2, assigning ans the value 4.
return ans; returns the value 4 back up into the calling context.
Back in the global context, sum2 becomes the returned value
Final memory environment:
n: 2
sum: function sum(num)
{
let ans = num + num;
return ans;
}
sum2: 4
Subscribe to my newsletter
Read articles from Siddhanth Gupta directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
