Updating Global Choice Options in CRM with HTML Web Resources & JavaScript Web API

Table of contents
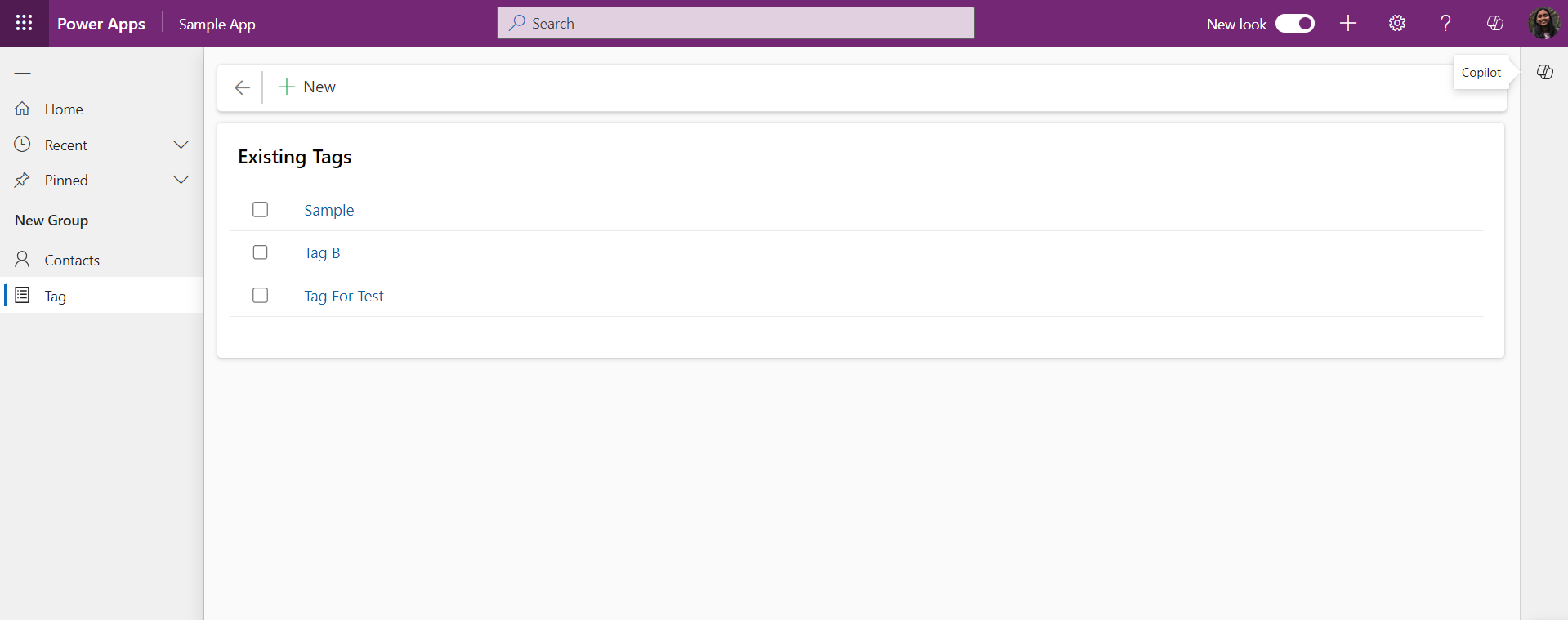
Dynamics 365 CRM is a powerful tool for managing business processes, but sometimes you need to customize it to meet your specific needs. In this blog post, we’ll explore how to manage option set values in a global option set using JavaScript. We'll cover how to add, update, and delete option set values using HTTP requests to the Dynamics 365 Web API.
Overview
Option sets in Dynamics 365 CRM are used to provide a set of predefined options to users. Sometimes, you need to dynamically manage these options based on user input or other conditions. The following JavaScript functions demonstrate how to interact with a global option set by adding, updating, and deleting values.
Code Explanation
Let's dive into the code:
1. Adding a Tag
The addTag
function is used to add a new option to the global option set. Here's how it works:
javascriptCopy codefunction addTag(tagName) {
showSpinner(); // Show loading spinner
const tagValue = Math.floor(Math.random() * 100000000); // Generate a unique value
const data = {
"OptionSetName": "bincyroy_tag", // Name of the global option set
"Label": {
"LocalizedLabels": [
{
"Label": tagName, // Display label
"LanguageCode": 1033 // Language code (English)
}
]
},
"Value": tagValue // Numeric value for the new option
};
fetch(`${organizationUri}/api/data/v9.2/InsertOptionValue`, {
method: 'POST',
headers: {
'OData-MaxVersion': '4.0',
'OData-Version': '4.0',
'Accept': 'application/json',
'Content-Type': 'application/json; charset=utf-8',
'Prefer': 'return=representation'
},
body: JSON.stringify(data)
})
.then(response => {
if (!response.ok) {
return response.text().then(text => { throw new Error(text); });
}
return response.json().catch(() => ({})); // Handle non-JSON response
})
.then(data => {
document.getElementById('result').innerText = 'Tag added successfully!';
setTimeout(() => {
location.reload(); // Refresh the page
}, 1000);
})
.catch(error => {
console.error('Error inserting option value:', error);
document.getElementById('result').innerText = 'Error adding tag: ' + error.message;
})
.finally(() => hideSpinner()); // Hide loading spinner
}
2. Updating a Tag
The updateTag
function updates an existing option in the global option set:
javascriptCopy codefunction updateTag(tagValue, tagName) {
const data = {
"OptionSetName": "bincyroy_tag",
"Value": parseInt(tagValue, 10),
"Label": {
"LocalizedLabels": [
{
"Label": tagName,
"LanguageCode": 1033
}
]
},
"MergeLabels": true
};
console.log('Request payload:', JSON.stringify(data));
fetch(`${organizationUri}/api/data/v9.2/UpdateOptionValue`, {
method: 'POST',
headers: {
'OData-MaxVersion': '4.0',
'OData-Version': '4.0',
'Accept': 'application/json',
'Content-Type': 'application/json; charset=utf-8',
'Prefer': 'return=representation'
},
body: JSON.stringify(data)
})
.then(response => {
console.log('Response status:', response.status);
console.log('Response headers:', response.headers);
if (!response.ok) {
return response.text().then(text => {
console.error('Error response text:', text);
throw new Error(text);
});
}
return response.json().catch(() => ({}));
})
.then(data => {
console.log('Response data:', data);
document.getElementById('editResult').innerText = 'Tag updated successfully!';
closeModal();
document.getElementById('edit').style.display = 'none';
document.getElementById('deletebutton').style.display = 'none';
document.getElementById('btnnew').style.display = 'inline';
loadTags();
})
.catch(error => {
console.error('Error updating option value:', error);
document.getElementById('editResult').innerText = 'Error updating tag: ' + error.message;
});
}
3. Deleting a Tag
The deleteTag
function removes an option from the global option set:
javascriptCopy codefunction deleteTag(tagValue) {
const data = {
"OptionSetName": "bincyroy_tag",
"Value": tagValue
};
fetch(`${organizationUri}/api/data/v9.2/DeleteOptionValue`, {
method: 'POST',
headers: {
'OData-MaxVersion': '4.0',
'OData-Version': '4.0',
'Accept': 'application/json',
'Content-Type': 'application/json; charset=utf-8'
},
body: JSON.stringify(data)
})
.then(response => {
if (!response.ok) {
return response.text().then(text => { throw new Error(text); });
}
document.getElementById('result').innerText = 'Tag deleted successfully!';
document.getElementById('edit').style.display = 'none';
document.getElementById('deletebutton').style.display = 'none';
document.getElementById('btnnew').style.display = 'inline';
document.getElementById('result').innerText = '';
loadTags();
})
.catch(error => {
console.error('Error deleting tag:', error);
document.getElementById('result').innerText = 'Error deleting tag: ' + error.message;
});
}
Key Points
API Endpoints: We use different endpoints (
InsertOptionValue
,UpdateOptionValue
,DeleteOptionValue
) to manage option set values.Error Handling: Proper error handling ensures that users receive meaningful error messages.
Page Refresh: The
addTag
function includes a page refresh to update the UI after adding a new tag.
Video Tutorial
For a visual demonstration of managing option set values in Dynamics 365 CRM, check out this YouTube video.
Conclusion
Managing option set values in Dynamics 365 CRM through JavaScript and the Web API allows for dynamic customization of your CRM instance. By integrating these functions into your CRM solutions, you can provide more flexibility and control over your data.
For more details on working with Dynamics 365 Web API, you can refer to the Microsoft Learn documentation.
Feel free to adapt these functions to fit your specific requirements and enhance the functionality of your Dynamics 365 CRM instance!
Subscribe to my newsletter
Read articles from Bincy Roy directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Bincy Roy
Bincy Roy
I specialize in customizing and configuring Dynamics 365 modules like Sales, Service, and Customer Insights. My work involves creating effective solutions with Power Automate and developing dynamic web resources using HTML, CSS, and JavaScript. This blog is where I share insights and practical tips on software development and business automation.