Simplifying Form Validation in React with React Hook Form: A Real-Life Use Case
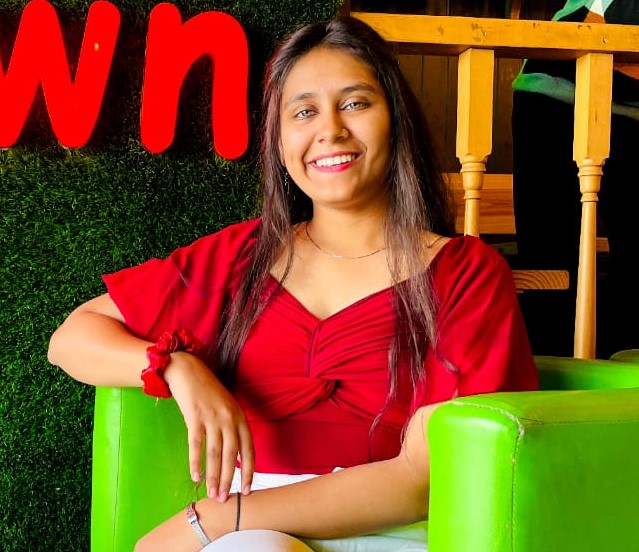
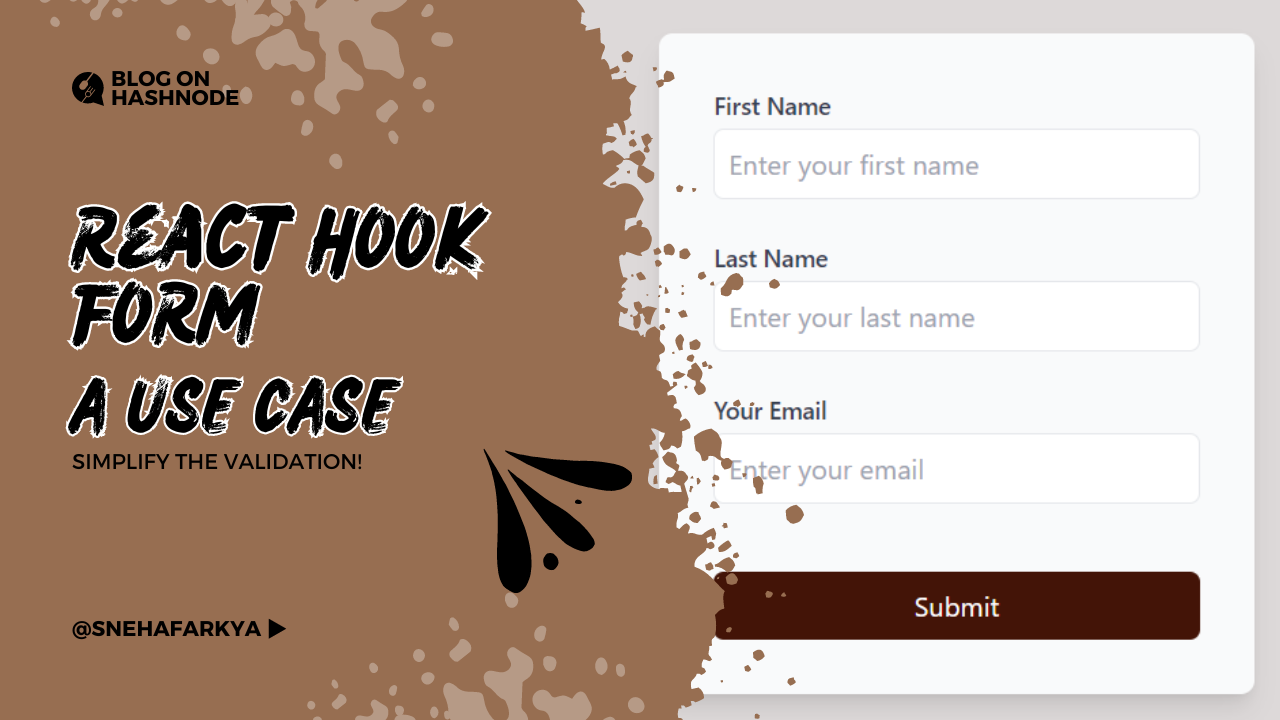
In modern web development, handling forms efficiently is crucial, especially regarding validation and managing user input. React Hook Form is a popular library that simplifies form management in React applications. In this blog, we’ll explore a practical example of using React Hook Form, how it addresses validation challenges, and break down its key features to understand why it’s a go-to solution for developers.
Prerequisites
Before diving into the implementation, you should be familiar with the following concepts:
React Understanding of functional components, state, and props.
JavaScript ES6: Familiarity with ES6+ syntax and features.
React Hook Form: Basic knowledge of what it is and its purpose.
CSS (Tailwind CSS): Knowledge of CSS and how to apply styles, particularly with utility-first frameworks like Tailwind CSS.
The Problem: Form Validation
Form validation is a common but often tedious task in web development. Manually managing form state, tracking input values, and handling validation errors can lead to bulky and error-prone code. For example, if you have a form with multiple input fields, each requiring specific validation, the traditional approach would involve a lot of state management and conditional rendering based on validation results.
Let’s say you’re building a simple contact form with fields for the first name, last name, and email. Each of these fields should be required, and the email field should follow a valid email format. Managing this with plain React would require several useState
hooks, event handlers, and conditional logic to display error messages.
This is where React Hook Form shines by drastically reducing the amount of code needed while providing a more declarative way to handle forms. Here’s how you can implement this form using React Hook Form:
1. Install React Hook Form:
First, you need to install React Hook Form in your project.
npm install react-hook-form
2. Create the Form Component:
In your ReactHookForm.js
file, you’ll define the form component using React Hook Form:
import { useForm } from "react-hook-form";
export default function ReactHookForm() {
const {
register,
handleSubmit,
formState: { errors },
} = useForm();
const onSubmit = (data) => {
console.log(data);
alert("First name: " + data.firstname + ", email: " + data.email);
};
return (
<>
<form
className="flex flex-col gap-6 justify-center max-w-sm w-[350px] mx-auto bg-gray-50 p-8 rounded-lg shadow-lg"
onSubmit={handleSubmit(onSubmit)}
>
{/* First Name Field */}
<div className="flex flex-col gap-1">
<label
htmlFor="firstname"
className="text-sm font-semibold text-gray-700"
>
First Name
</label>
<input
className="p-2 border rounded-md focus:outline-none focus:ring-2 focus:ring-orange-900"
placeholder="Enter your first name"
{...register("firstname", { required: "First name is required" })}
id="firstname"
/>
{errors.firstname && (
<span className="text-red-500 text-xs mt-1">
{errors.firstname.message}
</span>
)}
</div>
{/* Last Name Field */}
<div className="flex flex-col gap-1">
<label
htmlFor="lastname"
className="text-sm font-semibold text-gray-700"
>
Last Name
</label>
<input
className="p-2 border rounded-md focus:outline-none focus:ring-2 focus:ring-orange-900"
placeholder="Enter your last name"
{...register("lastname")}
id="lastname"
/>
</div>
{/* Email Field */}
<div className="flex flex-col gap-1">
<label
htmlFor="email"
className="text-sm font-semibold text-gray-700"
>
Your Email
</label>
<input
type="email"
className="p-2 border rounded-md focus:outline-none focus:ring-2 focus:ring-orange-900"
placeholder="Enter your email"
id="email"
{...register("email", { required: "Email is required" })}
/>
{errors.email && (
<span className="text-red-500 text-xs mt-1">
{errors.email.message}
</span>
)}
</div>
{/* Submit Button */}
<input
type="submit"
value="Submit"
className="bg-orange-950 text-white py-2 px-4 rounded-md cursor-pointer hover:bg-orange-900 transition-colors duration-200 ease-in-out w-full mt-4"
/>
</form>
</>
);
}
3. Integrate the Form in Your App:
In your App.js
file, import the ReactHookForm
component and render it:
import './App.css';
import ReactHookForm from './components/ReactHookForm';
export default function App() {
return (
<main className='flex items-center flex-col gap-8 my-6'>
<h2 className='text-3xl text-orange-950 font-bold underline underline-offset-2'>React Hook Form UseCase</h2>
<ReactHookForm/>
</main>
);
}
Understanding the Code
useForm
This is the hook provided by React Hook Form that manages the form state and validation. It returns several methods and properties that are essential for form handling.
register
The register
function is used to connect each input field to React Hook Form's state. It tracks the value, validation, and error messages for the respective input.
handleSubmit
This function wraps the form submission logic, ensuring the form is validated before it's submitted. If there are any validation errors, the form will not be submitted, and the errors will be displayed instead.
formState: { errors }
This object holds all the validation errors for the form. Each error is associated with a specific field, and you can use it to display error messages.
How React Hook Form Simplifies Validation
React Hook Form streamlines form validation by reducing complexity and boilerplate code. By using the register
function, you can easily connect input fields to the form state, with the library automatically tracking values and validation status.
Key benefits include:
Reduced Boilerplate: React Hook Form allows you to manage form state and validation with minimal code, as the
useForm
hook provides all necessary tools out of the box.Declarative Validation: Validation rules can be defined directly within the input fields using the
register
method, making the code cleaner and easier to maintain. Errors are automatically captured and can be displayed with minimal effort.Enhanced Performance: React Hook Form avoids unnecessary re-renders by not relying on external state management libraries, resulting in better performance.
This approach simplifies form handling in React, making it a powerful tool for developers looking to build efficient and maintainable forms.
Conclusion
React Hook Form is a game-changer for form handling in React. It streamlines the process of managing form state and validation, reducing the amount of code you need to write while improving performance. By integrating React Hook Form into your projects, you can create forms that are easy to maintain, user-friendly, and robust.
In this blog, we explored a real-life example of how React Hook Form can be used to create a simple yet effective user registration form. Whether you’re working on a small project or a complex application, React Hook Form is a tool that should be in your toolkit.
Check out the whole source code in this repository: here.
You can just experiment with this example and explore in more depth.
Want to read more?
Find me on this socials, I would love to connect with you:
Have a wonderful day🌻!
Subscribe to my newsletter
Read articles from Sneha Farkya directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
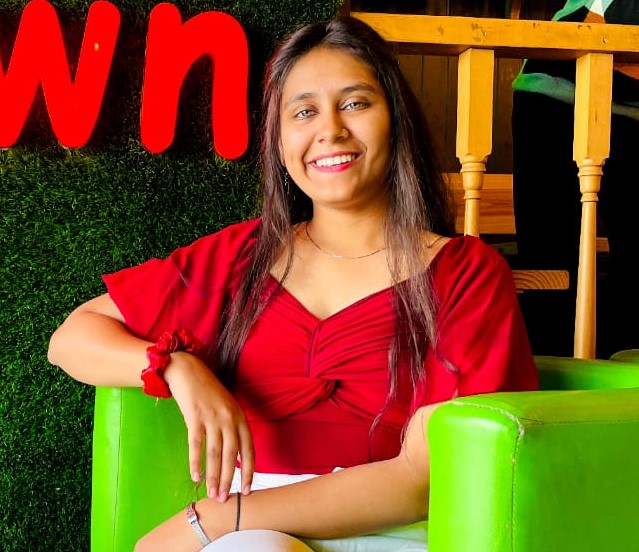
Sneha Farkya
Sneha Farkya
This is Sneha Farkya from India. I am a front-end developer and technical writer with a strong interest in creating interactive and visually appealing web interfaces. I am constantly exploring new technologies and keeping up with the latest trends. I like to solve people's problems and believe in giving bac to community