Easy-to-Understand Python Naming Conventions and Coding Best Practices

Table of contents
- Naming Conventions in Python
- General Python Naming Conventions
- Names to Avoid in Python
- Python Programming Naming Styles
- Python Global Variable Naming Conventions
- Python Class Naming Conventions
- Python Class Method Naming Convention
- Python Class Attribute Naming Convention
- Python Class File Naming Convention
- Python Class Instance Naming Convention
- Python Object Naming Conventions
- Python Variable Naming Conventions
- Python Function Naming Convention
- Automating Your Python Code Formatting
- Additional Resources
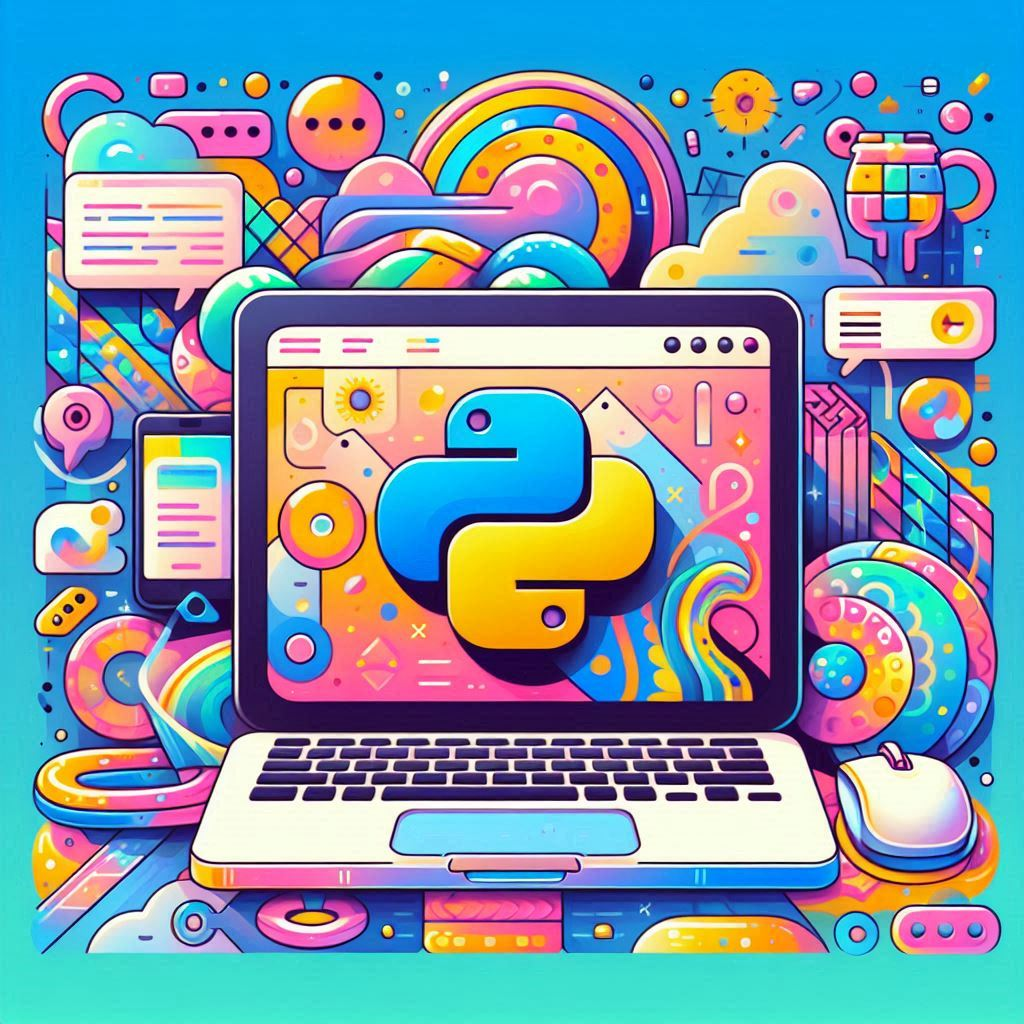
Let's dive into the world of Python naming conventions, but this time with a sprinkle of fun and metaphors. We'll cover everything from naming your variables to creating class names, and we'll do it with practical, easy-to-remember examples. So, grab your favorite snack, and let’s make your code cleaner and more 'Pythonic'!
Naming Conventions in Python
Think of Python naming conventions like the dress code for your code. It’s not like anyone’s going to arrest you if you wear flip-flops to a black-tie event, but you’ll definitely stand out—and not in a good way. Python's official style guide, PEP8, is your go-to resource for these guidelines, and following them is like showing up to the party in a sharp suit. It’s a mark of good taste and helps make your code easier to read, maintain, and share with others.
Let’s break down the basics with some fun examples!
General Python Naming Conventions
Before we dive into the nitty-gritty, let’s get the basics down:
Names are case-sensitive: So
myVariable
andmyvariable
are different. Think of them as identical twins with very different personalities.Names can’t start with a digit: No starting your variables with
1
or2
. Python likes to think of names like old-school usernames—letters first, please!Names can contain letters, numbers, and underscores: Feel free to mix it up, but keep it stylish. Use
var1
,var_name
, or evensuper_var_3000
.
Here’s a quick cheat sheet:
Acceptable | Not Acceptable |
var_name , myVar1 | 1var , var-name |
super_duper_var | var name |
Names to Avoid in Python
Avoid using characters like ‘l’ (lowercase L), ‘O’ (uppercase O), or ‘I’ (uppercase I) for single-character variable names. They’re the pranksters of the coding world, often getting mistaken for 1
(one), 0
(zero), and |
(pipe). Also, don’t try to be a rebel by using Python’s reserved words like for
, while
, or if
—those are off-limits.
Python Programming Naming Styles
Python’s naming styles are like different dress codes for different occasions. Here’s how you can dress up your code:
Snake Case: Words are all lowercase and separated by underscores. It’s like your comfy jeans—perfect for variable and function names.
- Example:
user_name
,get_total
- Example:
Pascal Case: Each word starts with a capital letter, no underscores. Think of this as your formal attire—great for class names.
- Example:
MyClass
,CarModel
- Example:
Camel Case: Similar to Pascal Case, but the first word starts with a lowercase letter. This one’s less common in Python—think of it as a style that’s a bit out of fashion.
- Example:
myVariable
,isInstance
- Example:
Upper Case with Underscores: This is your all-caps shouting style, used for constants that never change. Think of it as the code equivalent of bold, underlined text.
- Example:
PI_VALUE
,MAX_SPEED
- Example:
Here’s how these styles look side by side:
Case Type | Description | Example |
Snake Case | All words lowercase, separated by underscores | my_function , user_input |
Pascal Case | Capitalizes each word, no underscores | MyClass , CarModel |
Camel Case | Capitalizes each word except the first, no underscores | myVariable , isInstance |
Upper Case with Underscores | All uppercase letters, words separated by underscores | MAX_SPEED , TOTAL_VALUE |
Python Global Variable Naming Conventions
Global variables are like the VIPs at a party—they can go anywhere and do anything. But just like VIPs, they need to be handled with care. Use snake_case
for naming global variables, and try not to have too many of them running around.
Example:
# Correct way
global_variable = "I'm a global variable"
# For constants
PI_VALUE = 3.14159
MAX_SIZE = 100
Keep in mind that while global variables have their perks, too many of them can lead to a code that’s a bit of a wild party—fun, but hard to control.
Python Class Naming Conventions
Classes are the VIP sections of your code, and they deserve a name that reflects their status. Use PascalCase
for class names, with each word starting with a capital letter.
Example:
# Correct way
class MyClass:
pass
# Incorrect way
class my_class:
pass
Python Class Method Naming Convention
When it comes to methods (those are functions inside your classes), think of snake_case
again. Keep it casual, lowercase, and use underscores between words.
Example:
class MyClass:
def my_method(self):
pass
Python Class Attribute Naming Convention
Class attributes, the variables that belong to the class, should also be named using snake_case
. Let’s keep things consistent!
Example:
class MyClass:
my_attribute = 10
Python Class File Naming Convention
When you save your class in a file, use snake_case
again. It’s like labeling a folder neatly so you can find it later.
Example:
my_class_file.py
Python Class Instance Naming Convention
When you create an instance of your class (think of it as making a new VIP member), name it with snake_case
to keep it in line with the rest of your variables.
Example:
my_car = Car('red', 'Toyota', 'Corolla')
Python Object Naming Conventions
Naming your objects (instances of classes) is just like naming your variables. Use snake_case
and make it descriptive enough that anyone can tell what the object is at a glance.
Example:
# Correct way
my_car = Car('red', 'Toyota', 'Corolla')
# Incorrect way
MyCar = Car('red', 'Toyota', 'Corolla')
Python Variable Naming Conventions
Variables are like the everyday essentials of your code. Keep them in snake_case
to maintain consistency.
Example:
# Correct way
user_age = 25
# Incorrect way
userAge = 25
Remember, variable names should be like a good book title—clear, descriptive, and giving you an idea of what’s inside. Avoid single characters unless you’re in a loop (and even then, keep it simple).
Python Function Naming Convention
Functions are like the verbs of your code—they do the action. Name them in snake_case
, and start with a verb to make it clear what action they’re performing.
Example:
# Correct way
def calculate_average():
pass
# Incorrect way
def CalculateAverage():
pass
So, there you have it—a fun, friendly guide to Python naming conventions. Stick to these rules, and your code will be the talk of the town (or at least your team).
Automating Your Python Code Formatting
After mastering the art of naming your variables and classes, let's take it a step further by automating code formatting. Think of this as your personal stylist, making sure you always look sharp without lifting a finger.
VSCode Options
1. Automate with "Black"
Black is like your "black tie" for your black-tie event—it ensures your code is always dressed to impress. Just as a black tie makes you look sharp and ready for any formal occasion, Black keeps your code polished and consistent, no matter who's reviewing it. With Black handling the dress code, your code will always be in style, perfectly formatted, and ready to shine!
To add the line of code that sets
black
as the default formatter in VSCode, you’ll need to modify yoursettings.json
file. Here’s how to do it:Open VSCode and go to the settings by pressing
Cmd + ,
(macOS) orCtrl + ,
(Windows/Linux).In the search bar, type "settings.json" and click on "Edit in settings.json" when it appears.
Add the following line to your
settings.json
file:"python.formatting.provider": "black"
Save the file.
This line ensures that every time you format your code in VSCode, it will use black
.To run it manually:
black your_script.py
2. VSCode Extensions to Make Your Life Easier
Prettier: Although famous for JavaScript, Prettier supports Python too.
isort: Automatically sorts your imports, so they’re always in order.
By adding these tools and shortcuts to your arsenal, you'll keep your code looking sharp with minimal effort—just like showing up to a black-tie event already dressed to impress!
Built in Black Magic in JetBrains PyCharm
JetBrains PyCharm already comes with Black built-in, so you don’t even have to lift a finger! It’s like showing up to a black-tie event and finding out the bow tie is already pre-tied for you—effortless and elegant. With PyCharm, your code is always dressed to the nines without any extra hassle. All you need to do is activate it, and you're ready to code in style!
Reformat Code: Hit
Cmd + Option + L
on macOS orCtrl + Alt + L
on Windows/Linux to tidy up your code instantly.Optimize Imports: Clean up those imports with
Cmd + Option + O
(macOS) orCtrl + Alt + O
(Windows/Linux).
Additional Resources
Here are some useful links and resources for Python naming and style conventions according to the PEP 8 standard:
PEP 8: The Style Guide for Python Code - The official documentation of PEP 8, covering all aspects of writing clean, readable Python code, including naming conventions, indentation, line length, and more.
Real Python: Writing Clean Python Code with PEP 8 - This guide provides a practical overview of PEP 8, including examples and explanations that help in understanding the rules and applying them in real-world scenarios.
CS Department at UT Austin: PEP 8 Summary - A PDF summary of PEP 8, provided by the University of Texas, Austin. This resource is ideal for students or developers looking for a comprehensive overview of Python's style guidelines oai_citation:2,www.cs.utexas.edu.
Subscribe to my newsletter
Read articles from Derek Armstrong directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Derek Armstrong
Derek Armstrong
I share my thoughts on software development and systems engineering, along with practical soft skills and friendly advice. My goal is to inspire others, spark ideas, and discover new passions.