API 101 : Securing and Documenting Your RESTful API
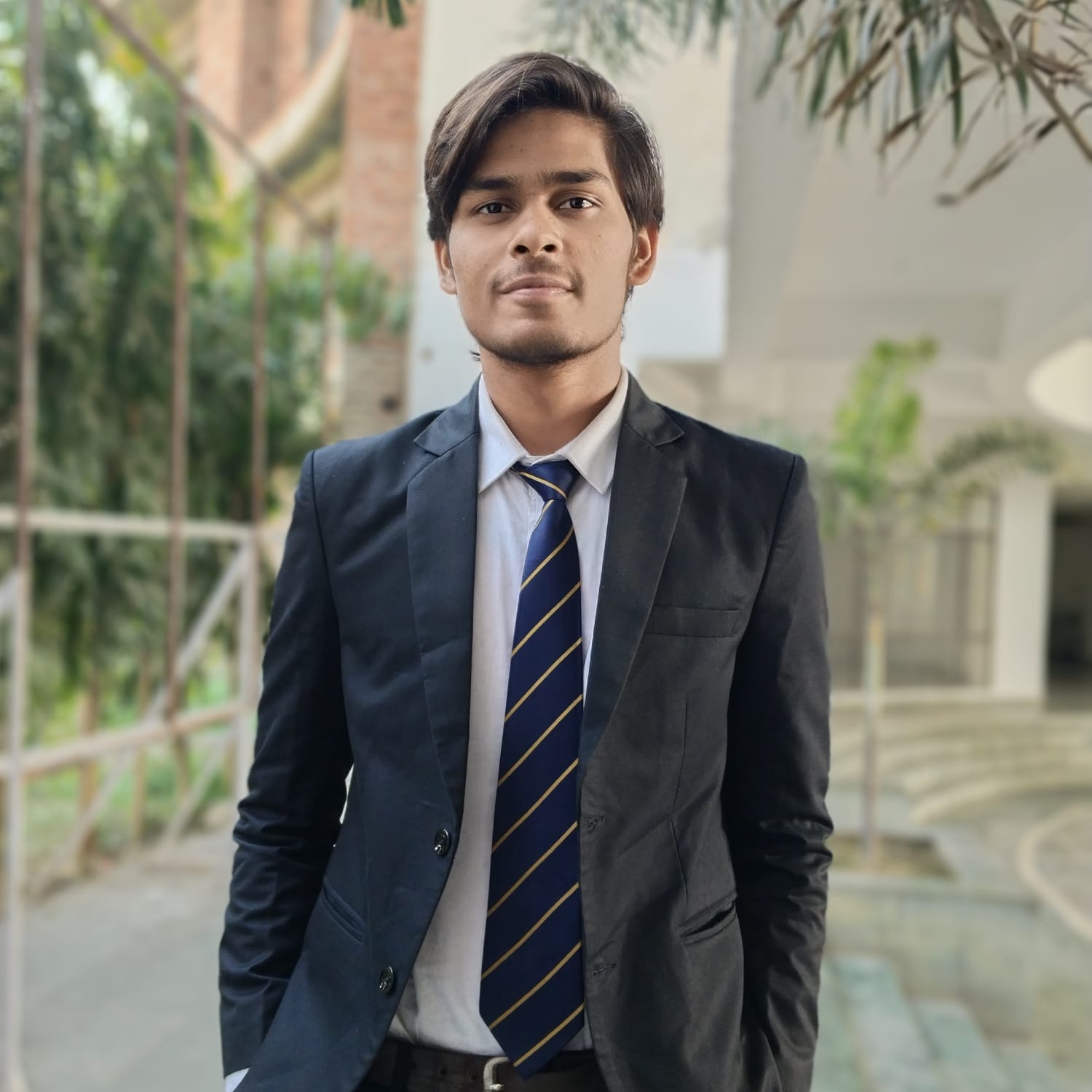
In the previous articles, we walked through building your first RESTful API. Now, it’s time to address two critical aspects of API development: security and documentation. Securing your API ensures that it’s protected from unauthorized access and potential threats, while proper documentation makes it easier for other developers to understand and use your API effectively.
Securing Your RESTful API
In the previous articles, we walked through building your first RESTful API. Now, it’s time to address two critical aspects of API development: security and documentation. Securing your API ensures that it’s protected from unauthorized access and potential threats, while proper documentation makes it easier for other developers to understand and use your API effectively.
Security is a paramount concern when developing APIs, especially if your API handles sensitive data or is publicly accessible. In this section, we’ll explore some common strategies for securing your RESTful API.
1. Authentication and Authorization
Authentication is the process of verifying the identity of a user or client accessing your API. Authorization determines what actions the authenticated user or client is allowed to perform.
Common Authentication Methods:
API Keys: An API key is a unique identifier used to authenticate requests. The client includes the API key in the request header or URL, and the server verifies it before processing the request. While simple, API keys don’t provide fine-grained access control and should be used with other security measures.
Example in Flask:
from flask import request, jsonify API_KEY = "your_api_key_here" @app.before_request def check_api_key(): key = request.headers.get('x-api-key') if key != API_KEY: return jsonify({"error": "Unauthorized"}), 401
OAuth2: OAuth2 is a more robust and flexible authentication framework that provides token-based authentication. It supports different flows (e.g., authorization code, client credentials) depending on the use case. OAuth2 tokens can be scoped to limit access to specific resources or actions.
JWT (JSON Web Tokens): JWTs are compact, URL-safe tokens that can be used to securely transmit information between the client and server. JWTs are often used for stateless authentication, where the server doesn’t need to store session information.
Example in Flask with JWT:
from flask_jwt_extended import JWTManager, create_access_token, jwt_required app.config['JWT_SECRET_KEY'] = 'your_jwt_secret_key' jwt = JWTManager(app) @app.route('/login', methods=['POST']) def login(): username = request.json.get('username') password = request.json.get('password') # Add user verification logic here if username == 'user' and password == 'password': access_token = create_access_token(identity=username) return jsonify(access_token=access_token) return jsonify({"error": "Invalid credentials"}), 401 @app.route('/protected', methods=['GET']) @jwt_required() def protected(): return jsonify({"message": "This is a protected route"})
2. HTTPS and SSL/TLS
Using HTTPS (Hypertext Transfer Protocol Secure) ensures that the data transmitted between the client and server is encrypted. This prevents attackers from intercepting and reading the data.
To secure your API with HTTPS:
Obtain an SSL/TLS certificate from a trusted Certificate Authority (CA).
Configure your web server (e.g., Nginx, Apache) to use HTTPS.
Ensure that all API endpoints are accessible only via HTTPS.
3. Rate Limiting
Rate limiting controls the number of requests a client can make to your API within a specific time frame. This helps protect your API from abuse, such as DDoS attacks or excessive usage.
Implementing rate limiting:
In Flask, you can use the
Flask-Limiter
extension:from flask_limiter import Limiter limiter = Limiter(app, key_func=lambda: request.remote_addr) @app.route('/books', methods=['GET']) @limiter.limit("5 per minute") def get_books(): return jsonify({'books': books})
Consider implementing a more advanced rate limiting strategy on your API gateway or load balancer if you’re handling high traffic.
4. Input Validation and Sanitization
Always validate and sanitize user input to prevent security vulnerabilities like SQL injection, cross-site scripting (XSS), and command injection.
Use parameterized queries when interacting with databases.
Validate JSON payloads against a schema to ensure that only expected data is processed.
Sanitize user input to remove or escape any potentially dangerous characters.
5. CORS (Cross-Origin Resource Sharing)
CORS is a security feature that restricts how resources on a web page can be requested from another domain. By default, browsers block cross-origin requests, but you can configure your API to allow specific domains or methods.
Example in Flask:
from flask_cors import CORS
app = Flask(__name__)
CORS(app, resources={r"/api/*": {"origins": "https://example.com"}})
Documenting Your RESTful API
Good documentation is essential for any API. It helps developers understand how to use your API, what endpoints are available, what data they need to send, and what responses they can expect. Let’s explore some best practices and tools for documenting your API.
1. Writing Clear and Concise Documentation
Overview: Start with an overview that explains what your API does, who it’s for, and how it can be used. Include information about authentication and any prerequisites.
Endpoint Descriptions: For each endpoint, provide the following details:
HTTP Method: GET, POST, PUT, DELETE, etc.
Endpoint URL: The specific URL path, e.g.,
/books/{id}
.Parameters: Query parameters, path variables, and request body data.
Request Example: A sample request with all necessary headers and body data.
Response Example: A sample response with a description of the data returned and possible status codes.
Error Handling: Document the possible errors and status codes that your API might return, along with explanations for each.
Code Samples: Provide code snippets in various languages (e.g., Python, JavaScript, cURL) to help developers get started quickly.
2. Using API Documentation Tools
Several tools can help you automate and standardize your API documentation:
Swagger/OpenAPI: Swagger is one of the most popular tools for generating interactive API documentation. It uses the OpenAPI Specification (OAS) to define your API’s endpoints, parameters, and responses. You can generate a Swagger UI that allows developers to test your API directly from the documentation.
Example Swagger definition:
openapi: 3.0.0 info: title: Book API version: 1.0.0 paths: /books: get: summary: Get a list of books responses: '200': description: A list of books content: application/json: schema: type: array items: type: object properties: id: type: integer title: type: string author: type: string
Postman: Postman offers a tool called Postman Collections, where you can document your API endpoints, organize them into groups, and share them with other developers. Postman also supports automatic generation of API documentation that can be published online.
Redoc: Redoc is another popular tool for generating API documentation from OpenAPI specifications. It provides a clean, customizable UI and supports complex documentation requirements.
3. Versioning Your API
As your API evolves, you may need to introduce changes that are not backward compatible. Versioning your API allows you to release new versions without breaking existing clients.
URI Versioning: Include the version number in the URI, e.g.,
/v1/books
.Header Versioning: Specify the version in the request header, e.g.,
Accept: application/vnd.myapi.v1+json
.Query Parameter Versioning: Include the version number as a query parameter, e.g.,
/books?version=1
.
Document the versioning scheme you’re using so that developers know how to access different versions of your API.
Conclusion
Securing and documenting your API are crucial steps in the development process. By implementing robust security measures, you protect your API from potential threats and ensure that only authorized users can access it. Proper documentation, on the other hand, makes your API more accessible and easier to use, fostering better adoption and integration.
In the final part of this series, we’ll explore advanced topics in API development, including performance optimization, error handling, and monitoring. These topics will help you refine your API and ensure it performs well under different conditions.
Subscribe to my newsletter
Read articles from Shivay Dwivedi directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
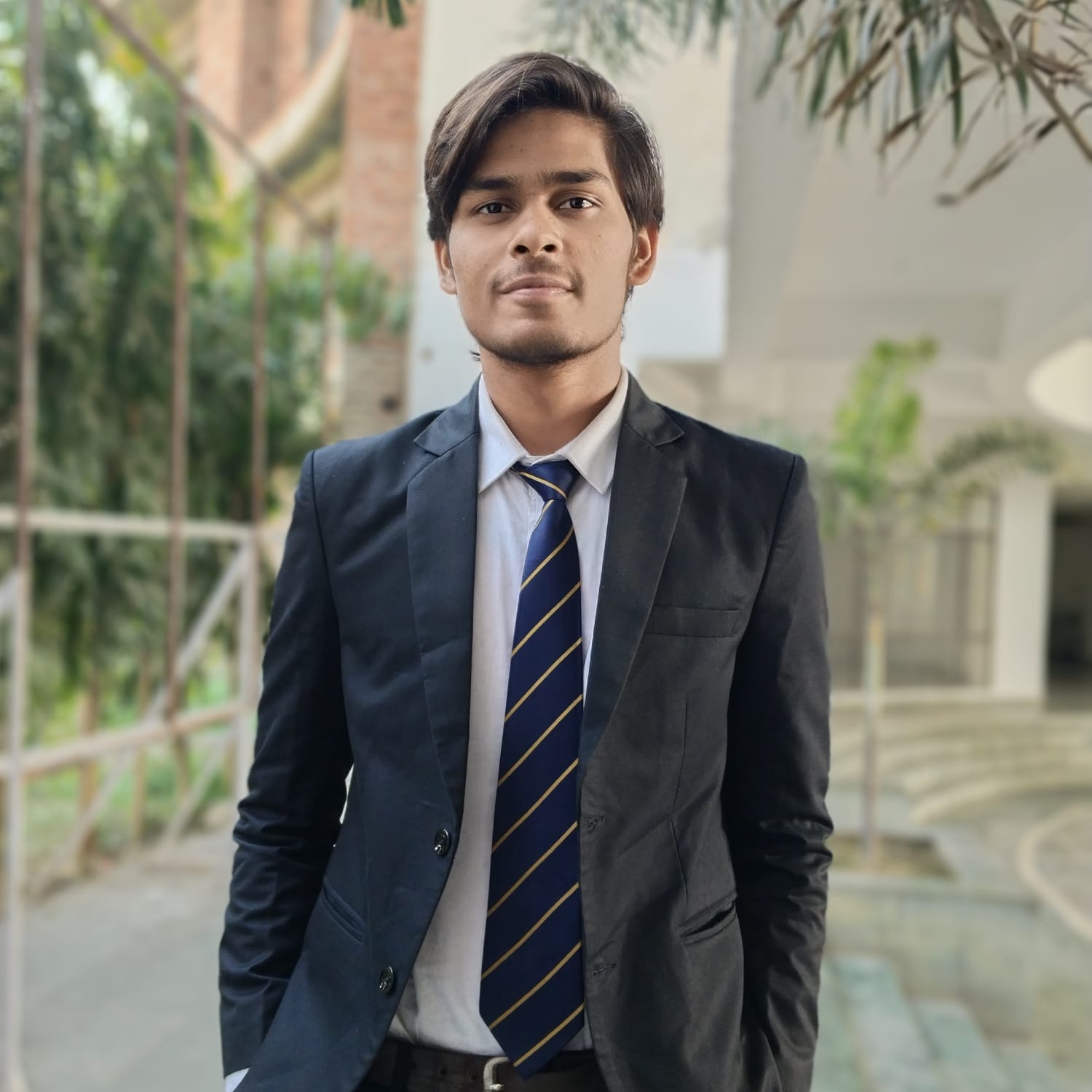