API 101 : Advanced Topics in API Development
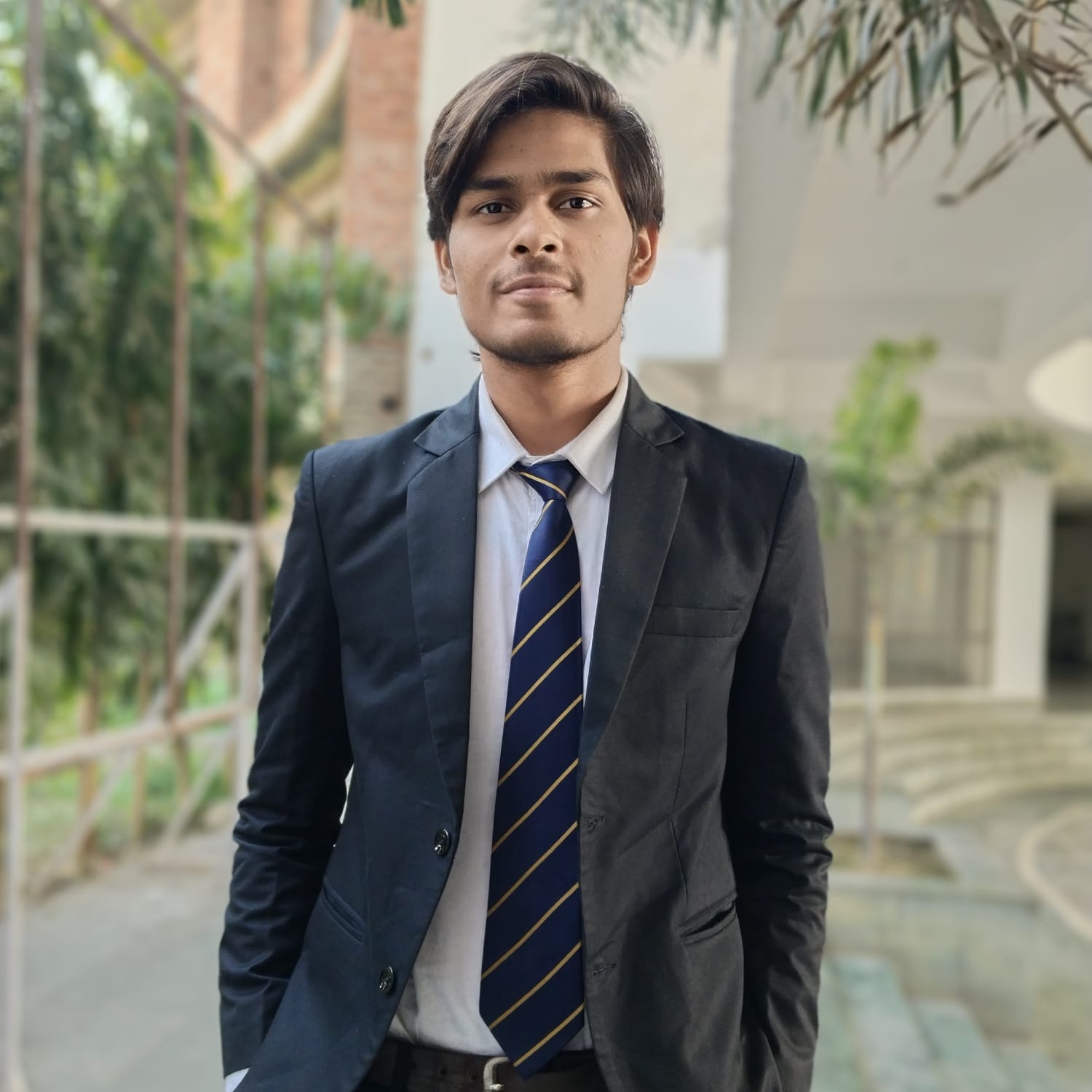
In this final part of our API 101 series, we’ll explore advanced topics in API development that go beyond the basics. These topics include performance optimization, error handling, monitoring, and versioning strategies. Mastering these concepts will help you build APIs that are not only functional but also scalable, reliable, and maintainable.
Performance Optimization
As your API grows and handles more traffic, optimizing performance becomes crucial. Here are some strategies to ensure your API remains responsive and efficient:
1. Caching
Caching is a technique that stores a copy of the data or response so that subsequent requests can be served faster without recomputing or fetching the data again.
Client-Side Caching: Use HTTP headers like
Cache-Control
,Expires
, andETag
to instruct clients to cache responses. This reduces the load on your server by allowing the client to reuse cached data.Example of setting cache headers in Flask:
@app.route('/books/<int:book_id>') def get_book(book_id): book = next((b for b in books if b['id'] == book_id), None) if book is None: return jsonify({'error': 'Book not found'}), 404 response = jsonify(book) response.headers['Cache-Control'] = 'public, max-age=3600' return response
Server-Side Caching: Store frequently accessed data or responses in a cache (e.g., Redis, Memcached) on the server-side. This reduces the need to query the database or perform expensive computations on every request.
CDN (Content Delivery Network): For APIs that serve static content (e.g., images, files), use a CDN to distribute the load across multiple servers worldwide. This improves response times for clients located far from your main server.
2. Database Optimization
If your API interacts with a database, optimizing your database queries is essential for performance.
Indexing: Ensure that your database tables are properly indexed, especially on columns used in
WHERE
,JOIN
, andORDER BY
clauses. Indexing improves query performance by allowing the database to find rows faster.Query Optimization: Avoid inefficient queries that fetch more data than needed or perform unnecessary calculations. Use database tools (e.g., query planners, analyzers) to identify and optimize slow queries.
Connection Pooling: Use a connection pool to manage database connections efficiently. This reduces the overhead of opening and closing connections for each request.
Pagination: For endpoints that return large datasets, implement pagination to limit the amount of data returned in each response. This reduces memory usage and improves response times.
Example of pagination in Flask:
@app.route('/books', methods=['GET']) def get_books(): page = int(request.args.get('page', 1)) per_page = int(request.args.get('per_page', 10)) start = (page - 1) * per_page end = start + per_page return jsonify({'books': books[start:end]})
3. Asynchronous Processing
Asynchronous processing allows your API to handle long-running tasks (e.g., file uploads, complex computations) without blocking the main request/response cycle.
Async/Await: In modern programming languages like Python, JavaScript, and C#, you can use async/await to perform non-blocking I/O operations. This is especially useful for APIs that interact with external services or databases.
Example of an async function in Flask (requires
Quart
, an async variant of Flask):from quart import Quart, jsonify app = Quart(__name__) @app.route('/async-books') async def async_get_books(): # Simulate a non-blocking I/O operation await some_async_operation() return jsonify({'books': books})
Background Jobs: Offload long-running tasks to a background job queue (e.g., Celery, Sidekiq) and return an immediate response to the client. The client can poll the API or receive a webhook notification when the task is complete.
Error Handling
Proper error handling is crucial for a robust API. It ensures that errors are communicated clearly to the client and that your API can gracefully recover from unexpected situations.
1. Standardized Error Responses
Return consistent and standardized error responses that include:
HTTP Status Code: Use appropriate status codes (e.g., 400 for bad requests, 401 for unauthorized access, 404 for not found, 500 for server errors) to indicate the type of error.
Error Message: Provide a clear and concise error message that describes what went wrong.
Error Code (Optional): Include a custom error code that helps developers identify specific errors.
Example of standardized error responses in Flask:
@app.errorhandler(404) def not_found_error(error): return jsonify({'error': 'Resource not found', 'code': 404}), 404 @app.errorhandler(500) def internal_error(error): return jsonify({'error': 'Internal server error', 'code': 500}), 500
2. Logging
Implement comprehensive logging to capture errors, warnings, and important events. This helps with debugging and provides insights into the health of your API.
Log Levels: Use different log levels (e.g., DEBUG, INFO, WARNING, ERROR, CRITICAL) to categorize log messages based on their importance.
Structured Logging: Log messages in a structured format (e.g., JSON) that makes it easier to parse and analyze logs using tools like ELK Stack (Elasticsearch, Logstash, Kibana) or Splunk.
Error Reporting: Set up automated error reporting tools (e.g., Sentry, Rollbar) to track and notify you of errors in real-time.
Monitoring and Analytics
Monitoring and analytics provide insights into how your API is performing and how it’s being used. This helps you detect issues early and make data-driven decisions.
1. API Monitoring
Set up monitoring tools to track the availability, performance, and error rates of your API.
APM (Application Performance Monitoring): Tools like New Relic, Datadog, or Prometheus help monitor the performance of your API, including response times, database queries, and external service calls.
Health Checks: Implement health check endpoints (e.g.,
/health
,/status
) that return the status of your API and its dependencies. This allows you to integrate with monitoring systems that regularly check the health of your API.Example of a simple health check endpoint:
@app.route('/health', methods=['GET']) def health_check(): return jsonify({'status': 'OK'}), 200
2. API Analytics
Analytics tools provide insights into how your API is being used, which endpoints are most popular, and where performance bottlenecks may exist.
API Gateway Analytics: If you’re using an API gateway (e.g., AWS API Gateway, Kong, Apigee), leverage its built-in analytics to track API usage, response times, and errors.
Custom Analytics: Implement custom analytics by logging key metrics (e.g., request count, response time) and analyzing them using tools like Google Analytics, Mixpanel, or custom dashboards built with Grafana.
3. Rate Limiting and Throttling
To protect your API from abuse and ensure fair usage, implement rate limiting and throttling.
Rate Limiting: Limit the number of requests a client can make within a specific time frame (e.g., 100 requests per minute).
Throttling: Gradually reduce the client’s allowed request rate if they exceed the rate limit, instead of blocking them outright.
Implement these mechanisms at the API gateway level or within your application using middleware.
API Versioning Strategies
As your API evolves, you may need to introduce breaking changes. API versioning allows you to manage these changes without disrupting existing clients.
1. URI Versioning
Include the version number in the URI path.
Pros: Simple and clear.
Cons: Requires updating all client requests when changing the version.
Example:
/v1/books /v2/books
2. Header Versioning
Include the version number in the request header.
Pros: Keeps the URI clean.
Cons: Less discoverable and requires clients to modify headers.
Example:
GET /books Accept: application/vnd.myapi.v1+json
3. Query Parameter Versioning
Include the version number as a query parameter.
Pros: Easy to implement and modify.
Cons: Adds complexity to the query string.
Example:
/books?version=1 /books?version=2
4. Backward Compatibility
When introducing a new version, strive to maintain backward compatibility with older versions whenever possible. This reduces the impact on existing clients.
Conclusion
In this article, we explored advanced topics in API development, including performance optimization, error handling, monitoring, analytics, and versioning strategies. These concepts are essential for building APIs that are not only functional but also scalable, reliable, and easy to maintain.
With the knowledge you’ve gained throughout this API101 series, you’re now equipped to design, build, secure, and optimize robust APIs. Whether you’re working on a small personal project or a large-scale enterprise API, these best practices will help you deliver high-quality
Subscribe to my newsletter
Read articles from Shivay Dwivedi directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
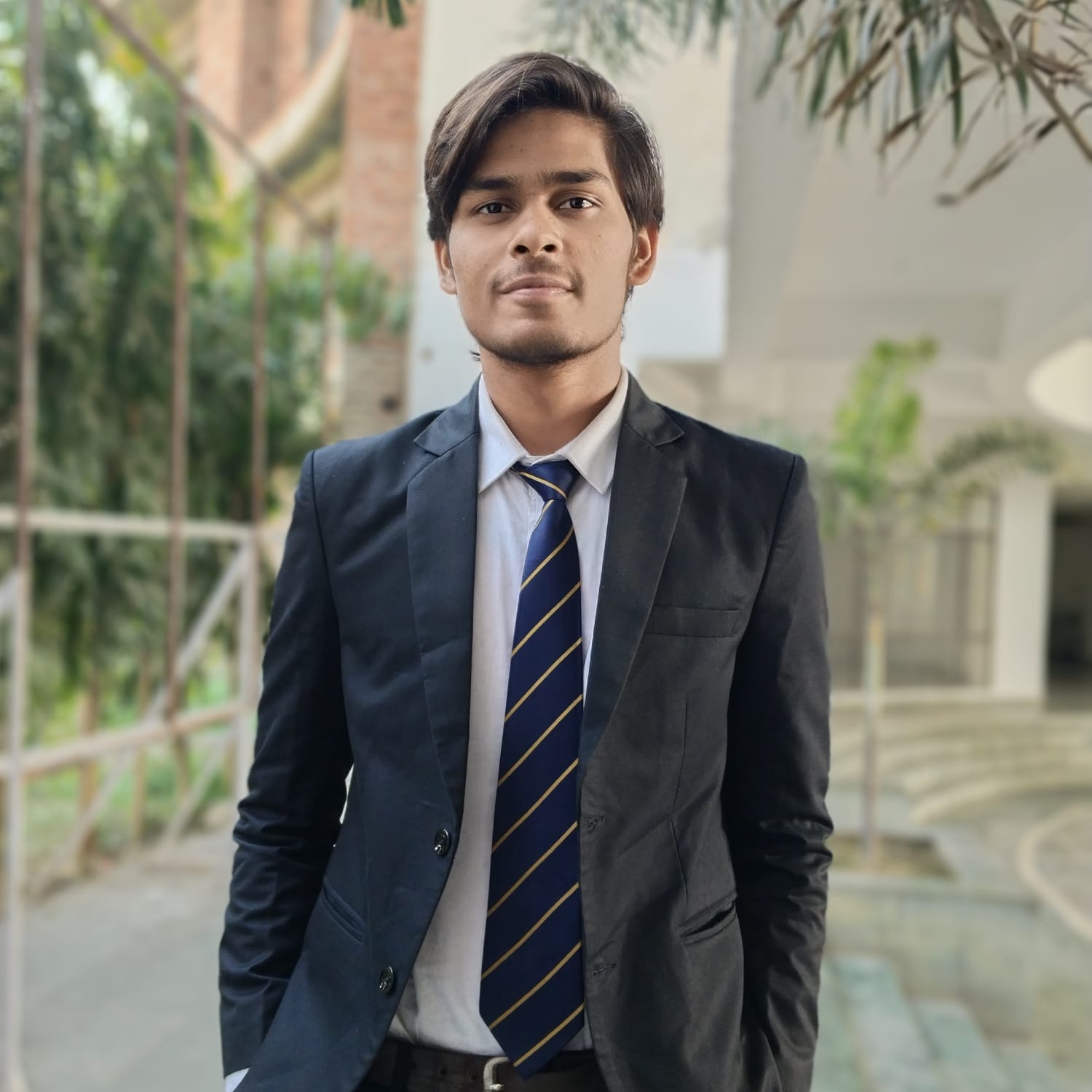