Bill Management using C (Terminal based)
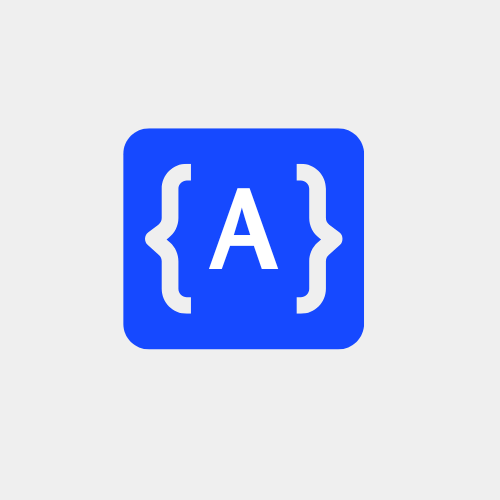
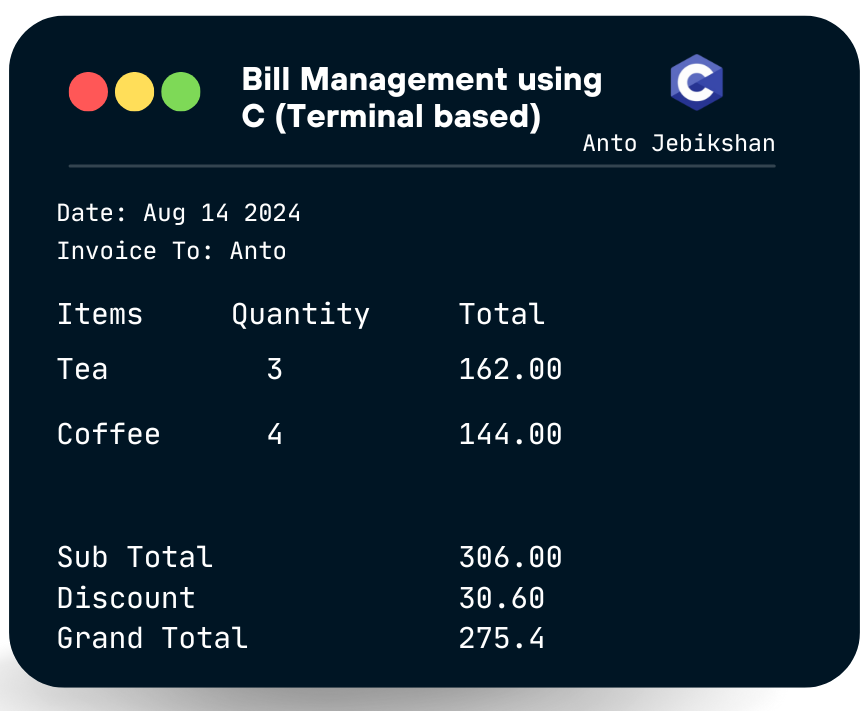
Building a Simple Terminal-Based Billing System in C: A Step-by-Step Guide
Introduction
In this article, we'll delve into creating a simple yet functional terminal-based billing system using the C programming language. This project is perfect for those looking to enhance their understanding of file handling, data structures, and basic arithmetic operations in C.
Project Overview
The goal of this project is to develop a billing system that handles customer transactions, generates invoices, and stores bill records in a file. Our system will support basic operations such as generating a new bill, viewing historical bills, and retrieving recent bills based on customer names.
Key Features
Bill Generation: Create and display a bill with customer details, itemized entries, and calculated totals.
Bill Storage: Save bill records to a file for future reference.
Bill Retrieval: Retrieve and display historical bills based on customer names.
Implementation Details
Let's walk through the implementation step-by-step.
Define Data Structures
In C, structuring data efficiently is crucial for managing related information. We define two structures: entries
and users
. The entries
structure holds details about individual items on the bill, including the item name, unit price, and quantity. This allows us to represent each product sold. The users
structure contains customer information, including their name, the date of the transaction, the number of products purchased, and an array of entries
to store all items associated with the bill. This organization ensures that we can manage and access all relevant information in a structured manner.
struct entries {
char item[20];
float price;
int qty;
};
struct users {
char name[50];
char date[30];
int nop;
struct entries ent[50];
};
We define two primary structures: entries
for individual items and users
for customer records.
Generate Unique Bill IDs
(while real-world systems often require more robust unique ID generation techniques, our implementation with random numbers is practical and effective for this small-scale project)
To uniquely identify each bill, we use a simple random number generation technique. The csid()
function generates a unique bill ID by adding a random number between 0 and 89999 to a base value of 10000. This approach ensures that each bill ID is unique and falls within a specific range, helping to prevent duplicate IDs and making it easier to track individual transactions.
int csid() {
return 10000 + rand() % 90000;
}
Print Bill Header
The billTop
function is responsible for displaying the bill's header, which includes the billing system’s name, the date of the transaction, and the customer's name. It uses formatted printing to create a clear and organized output, which helps users easily identify the bill's context. The header also sets up the table format for displaying item details, including item names, quantities, and total costs. This structured presentation is crucial for readability and professional appearance.
void billTop(char name[50], char date[30]) {
printf("\n\n");
printf("\t AJ Billing System");
printf("\n\t -----------------");
printf("\nDate: %s", date);
printf("\nInvoice To: %s", name);
printf("\n");
printf("---------------------------------------\n");
printf("Items\t\t");
printf("Quantity\t\t");
printf("Total\t\t");
printf("\n---------------------------------------");
printf("\n\n");
}
Print Bill Details
The billBody
function formats and prints each item on the bill. It takes the item’s name, quantity, and unit price as inputs, calculates the total price for each item, and outputs this information in a tabulated format. This function ensures that the bill accurately reflects the quantities and prices of all purchased items, making it easy for the customer to review their purchases. By handling each item individually, the function maintains clarity and precision in the billing process.
void billBody(char item[30], int qty, float price) {
printf("%s\t\t", item);
printf("\t%d\t\t", qty);
printf("%.2f\t", qty * price);
printf("\n");
}
Print Total and Taxes
The billDetails
function handles the calculation and presentation of the final amounts on the bill. It computes the subtotal, applies a discount, and calculates the net total. Additionally, it calculates the Central Goods and Services Tax (CGST) and State Goods and Services Tax (SGST) at specified rates. These values are then formatted and printed, providing a comprehensive summary of the transaction. This step ensures that all financial aspects of the bill are accurately represented and easily understandable for the customer.
void billDetails(float total) {
printf("\n");
float dis = 0.1 * total;
float netTotal = total - dis;
float cgst = 0.09 * netTotal, grandTotal = netTotal + 2 * cgst;
printf("---------------------------------------\n");
printf("Total\t\t\t%.2f", total);
printf("\nDiscount @10%%\t\t%.2f", dis);
printf("\n\t\t\t\t\t-------");
printf("\nNet Total\t\t\t%.2f", netTotal);
printf("\nCGST \t\t\t%.2f", cgst);
printf("\nSGST \t\t\t%.2f", cgst);
printf("\n---------------------------------------");
printf("\nGrand Total\t\t\t%.2f", grandTotal);
printf("\n---------------------------------------\n");
}
Main Function Logic
The main
function orchestrates the billing process by presenting a menu of options to the user, including starting a new bill, retrieving historical bills, or viewing recent bills. It handles user input, manages file operations for storing and retrieving bills, and ensures the system's operation based on user choices. For example, when starting a new bill, it collects customer and item information, calculates totals, and prints the bill. When retrieving bills, it reads from the file, formats the output, and displays the requested information. This central function integrates all components of the system, providing a user-friendly interface and maintaining the application's overall functionality.
int main() {
// Initialization
int option, cid, n;
struct users usr;
struct users user;
char store_entries = 'y', contFlag = 'y';
char name[50];
FILE *flp;
while (contFlag == 'y') {
system("clear");
float total = 0;
int bill_found = 0;
printf("\t AJ Billing System ");
printf("\n\nOptions:");
printf("\n\n1.Start Bill");
printf("\n2.Get History");
printf("\n3.Show Recent Bill");
printf("\n4.Close");
printf("\n\nPick the Action You Want to Take : \t");
scanf("%d", &option);
fgetc(stdin); // Clear newline character left by scanf
switch (option) {
case 1:
// Start new bill
// Collect customer and product details
// Generate and print bill
break;
case 2:
// Retrieve and display all historical bills
break;
case 3:
// Retrieve and display a recent bill by customer name
break;
case 4:
printf("\n\t\t Closed...");
exit(0);
break;
default:
printf("Invalid action!\n");
break;
}
printf("Do you want to continue? [y/n]\t");
scanf(" %c", &contFlag);
fgetc(stdin);
}
printf("\n\t\t Closed...");
printf("\n\n");
return 0;
}
Output :
Explore or Clone repository on GitHub
The billing system is designed with a focus on functionality and efficiency, operating exclusively within a terminal environment. While it does not incorporate a graphical user interface (GUI) or advanced visual elements, this choice allows for streamlined performance and simplicity in execution.
In this text-based interface, users interact directly with the system through command-line inputs and outputs. The absence of a GUI emphasizes the core functional aspects of the billing system, ensuring a straightforward and resource-efficient experience. The output is meticulously formatted to provide clear and concise information, including invoice details, itemized billing, and summary calculations.
This approach not only minimizes system resource usage but also facilitates easy deployment and use in environments where graphical interfaces are impractical or unnecessary. By focusing on efficient data processing and clear textual presentation, the system delivers a robust solution for billing management within the constraints of a terminal-based framework.
Final Remarks
In developing this terminal-based billing system, we’ve explored fundamental concepts in C programming, such as data structures, file handling, and user interaction. By utilizing simple random number generation for unique IDs, we’ve created a functional and straightforward system that meets the needs of a small-scale application.
While more sophisticated methods like UUIDs or database auto-increment features are essential for handling large-scale or distributed systems, our approach is sufficient for this prototype. This project demonstrates the core principles of creating a billing system and provides a solid foundation for more advanced development.
As you continue to build and refine your programming skills, consider exploring these advanced techniques and integrating them into more complex systems. This hands-on experience with basic and practical programming concepts will be invaluable as you tackle larger and more intricate projects in the future.
As an undergraduate student, this project has been a valuable learning experience, enhancing my understanding and skills.
Subscribe to my newsletter
Read articles from Anto Jebikshan directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
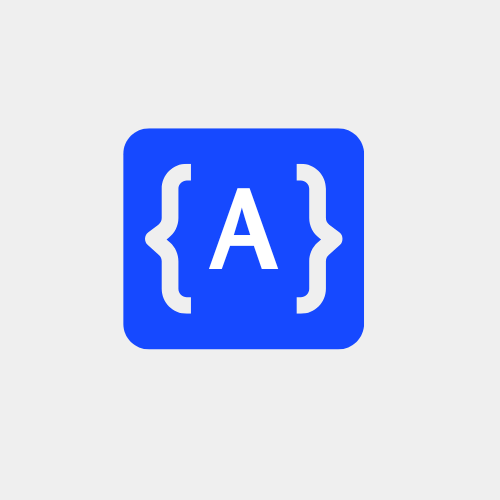
Anto Jebikshan
Anto Jebikshan
As a passionate and driven third-year Computer Science and Engineering undergraduate, I have developed a comprehensive skill set in both technical skills and web development. My technical proficiency encompasses multiple programming languages, including C, C++, Java, and Python, which I utilize to address a wide range of computational challenges. I am also proficient in full-stack web development, proficient in front-end technologies such as React and JavaScript ,Frameworks like Bootstrap, as well as backend frameworks like Express.js and Node.js. My experience includes database management with SQL, PostgreSQL, and PHP, enabling me to design and implement efficient data storage solutions. Additionally, I have practical knowledge in RESTful API integration, facilitating seamless communication between systems. Through academic projects and personal endeavors, I continually seek to enhance my expertise, driven by a deep-seated passion for technology and innovation.